This paper introduces the characteristics and kernel structure of the real-time operating system μC/OS-II, and implements the migration of μC/OS-II to the Motorola embedded processor MCF5272 for the first time.
As a real-time kernel, μC/OS has been familiar to people since 1992, and has now developed into μC/OS-II. ΜC/OS-II supports up to 56 tasks. Its kernel is preemptive and always executes the highest priority task in the ready state. It also supports a variety of commonly used inter-process communication mechanisms such as Semaphore, Mailbox, Message Queue, etc. Unlike most commercial RTOS, μC/OS-II makes all source codes public and available for free, and charges a small license fee for commercial applications . General commercial operating systems such as VxWorks, pSOS, and WinCE cost tens of thousands of dollars to purchase, and each product needs to pay operating fees, which is expensive to develop and use.
At present, MCF5272 is the most integrated ColdFire processor of Motorola. It adopts ColdFire V2 variable-length RISC processor core and DigitalDNA technology, and can reach 63Dhrystone2.1MIPS at 66MHz clock. Its internal SIM (System Integrated Module) unit integrates a variety of general modules, such as 10/100MHz Fast Ethernet controller, USB1.1 interface, etc., and can achieve seamless connection with commonly used peripheral devices (such as SDRAM, ISDN transceiver), thereby simplifying the peripheral circuit design and reducing product cost, volume and power consumption.
Use GNU tool chain (including cross compiler GCC , assembler AS, etc.) to compile μC/OS-II kernel, and the host environment is 16MB SDRAM. Compile the executable code of MCF5272 processor on the host, and download it to the target board for debugging and running through MCF5272's BDM debugging tool.
1 μC/OS-II system structure
Figure 1 illustrates the software and hardware architecture of μC/OS-II. The application is at the top level of the entire system. Each task can think that it has exclusive use of the CPU, so it can be designed into an infinite loop. μC/OS-II processor-independent code provides μC/OS-II system services. Applications can use these API functions for memory management, inter-task communication, and task creation and deletion.
Most of the μC/OS-II code is written in ANSI C, so μC/OS-II is highly portable. However, some processor-related code still needs to be written in C and assembly language. The porting of μC/OS-II needs to meet the following requirements:
(1) The processor's C compiler can generate reentrant code;
(2) C calls can be used to enter and exit critical code;
(3) The processor must support hardware interrupts and require a timer interrupt source;
(4) The processor needs a hardware stack that can accommodate a certain amount of data;
(5) The processor needs instructions that can exchange data between CPU registers and memory and stack.
The main work of porting μC/OS-II is to write processor and compiler-related code and BSP.
2 μC/OS-II DSP Writing
BSP (board support package) is a software layer between the underlying hardware and the operating system. It completes the initial hardware and software initialization after the system is powered on, and encapsulates the underlying hardware so that the operating system no longer faces specific operations.
Write a simple BSP for μC/OS-II. It first sets the CPU internal registers and system stack, initializes the stack pointer, and establishes the program's running and calling environment; then you can easily use C language to set the MCF5272 chip select address (CS0~CS7), GPIO and SDRAM controller, initialize the serial port ( UART 0) as the default print port, and provide the operating system with some hardware-related routines and functions such as dprintf() to facilitate debugging; after the CPU, board level and program initialization are completed, the control of the CPU can be handed over to the operating system.
The MCF5272 processor treats system power-on as exception No. 2, so the physical address of the first command needs to be filled in the corresponding position in the exception vector table, which can be automatically completed during compilation. The vector table must be stored in the FLASH corresponding to CS0 for automatic reading when the CPU is powered on. Such as:
_vectors: //starting address of vector table.long
0x0,_start,_fault,_fault,… //Initialize 1K byte vector table
...
_start: nop //first instruction
move.w #0x2700,%sr //shield all interrupts
move.1 #_vectors,%d0
move.c %d0,%VBR //#vectors->VBR
move.1 #0x10000001,%d0
move.c %d0,%MBAR //SIM unit base address 0x10000000
move.1 #0x20000001,%a0 //SRAM starting address 0x20000000
move.c %a0,%RAMBAR0 //Initialize internal SRAM
move.1 #0x20001001,%a7 //Set stack pointer
...
jsr cpu_init //Call cpu_init to initialize SIM unit
jsr ucos_start //Start μC/OS-II
……
Among them, the cpu_init function is used to initialize the CPU's internal SIM unit, SDRAM controller, and UART serial port. It is worth noting that the SDRAM initialization has different initialization timings for different manufacturers.
After completing the chip-level and board-level initialization, the BSP is also responsible for initializing the program itself, such as copying the contents of the .data segment from the read-only ROM to the SDRAM and establishing the runtime environment. The following is the code to establish the program data segment:
m EMC py(&_sdata,&_etext,(&_ EDA ta-&_sdata)); //Copy the .data segment
MEMS et(&_sbss,0,(&_ebss - &_sbss)); //Clear the .bss segment
Four simple assembly functions need to be written for μC/OS-II. After each hardware clock arrives, μC/OS-II will call OSI NTC txSw() in the interrupt service routine to schedule tasks; in addition, when a task is suspended due to waiting for resources, it is not necessary to wait until its own time slice is used up. It can actively give up the CPU, which can be achieved by calling a task-level task scheduling function OSCtxSw(). The relatively complex one is OSIntCtxSw(). Since OSTickISR() calls OSIntExit(), and OSIntExit() calls OSIntCtxSw() again, if the task is switched, neither call will return, and different C compilers and different compilation options use the stack differently when processing C calls. Therefore, OSIntCtxSw() is compiler-related. When GCC uses level 2 to 4 optimization, there will be a jsr jump instruction in the main calling function, and the called function starts with linkw % FP , #
OSIntCtxSw:
adda.1 #16,%a7 //Stack compensation, GCC-O2->-04 optimization
move.1 (OSTCBCur), %a1
move.1 %a7,(%a1) //OSTCBCur->OSTCBStkPtr=SP
jsr OSTaskSwHook //Reconcile Hook hook function
/*OSTCBCur->OSTCBStkPtr=OSTCBHighRdy->OSTCBStkPtr*/
move.1 (OSTCBHighRdy),%a1
move.1 %a1,(OSTCBCur)
move.b (OSPrioHighRdy),%d0
move.b %d0,(OSPrioCur) //OSPrioCur=OSPrioHighRdy
move.1 (%a1),%a7 //SP=OSTCBCur->OSTCBStkPtr
movem.1 (%a7),%d0-%d7/%a0-%a6 //Restore CPU registers and switch to new task
lea 60(%a7),%a7
Due to the limited space of rte
, the other three functions will not be described.
3 μC/OS-II task stack initialization
Each task in μC/OS-II has its own task stack, which is initialized by OSTaskStkInit() at the beginning of task creation. The purpose of initializing the stack is to simulate an interrupt. The task stack saves the starting address of the task code and some CPU registers (the initial value is irrelevant), so that once the conditions are met, the task can be executed. When an interrupt occurs, MCF5272 will automatically save the program pointer PC, status register SR and some other information in a four-word frame structure. In addition, %d0-%d7 and %a0-%a6 must also be pushed into the stack in a certain order. After completing the stack initialization, OSTaskStkInit() also returns the top of the stack pointer for the initialization of the task control block TCB structure. The program is written in C language.
4 μC/OS-II system clock
The MCF5272 processor has 4 built-in timers, and uses TIMER0 to generate a 10ms periodic interrupt as the system clock . When the PIVR register is set to 0x40, TIMER0 is interrupt No. 69. The entry address of the clock service program OSTickISR() needs to be filled in the corresponding position of the vector table, and the clock needs to be initialized:
volatile unsigned short*pTimer;
pTimer=(unsigned short*)(0x10000000+0x200); //Point to TIMER0
/*Reset clock*/
*pTimer &=0xFFF9; //Timer is in STOP state
*pTimer=(*pTimer & 0x00FF)|0xFA00;//Prescaler = 250
*pTimer |=0x0018; //Count is automatically cleared when full, interrupt mode
pTimer[2]=165; //Set TRR=165
*pTimer=(*pTimer & 0xFFF9) |0x0004; //CLK=Master/16, start
The clock beat cycle of the above program segment is: (1/66MHz)×250×164×16=0.01 seconds. A more precise clock can be used in situations with high real-time requirements. Once TIMER0 is initialized, it starts working. However, in order for an interrupt to occur, the corresponding field of the ICR register must be set to assign an IPL (Interrupt Priority Level) to the interrupt, and ensure that the interrupt is not masked by the initial state register SR.
The clock initialization code can be placed in the first μC/OS-II task and executed after OSStart(). Once the kernel can perform normal task switching, the porting work is basically completed.
5 Kernel compilation and download
All C and assembly source files are compiled and linked to form a binary image file. Since μC/OS-II uses custom data types, they must be converted into types that can be recognized by GCC (GNU C Compiler), such as INT8U can be defined as unsigned char. In addition, an LD (link script) file must be written to control the compilation and locate the program in the actual ROM and RAM resources. For debugging convenience, the kernel is usually downloaded to the target board SDRAM through the BDM tool for running, and then solidified into FLASH after debugging.
RTOS is a feature of current embedded applications. Applying RTOS can make products more reliable, more powerful and with a shorter development cycle. μC/OS-II has good real-time performance and a small amount of code, and has been widely ported to many processors such as x86, 68K, ColdFire, MPC 8xx, ARM, MIPS, C5409, etc. Hundreds of successful commercial application examples show that μC/OS-II is a stable and reliable kernel, so porting μC/OS-II to MCF5272 has a strong practical prospect.
Previous article:Simplify the principles and design of portable medical equipment based on high-performance analog devices
Next article:Floating-point digital signal processing based on the SHARC 2147x processor
Recommended ReadingLatest update time:2024-11-16 22:38
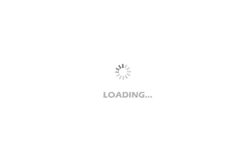
![[Lianshengde W806 Hands-on Notes] VII. I2C](https://6.eewimg.cn/news/statics/images/loading.gif)
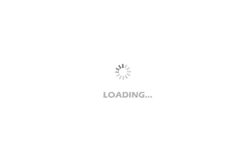
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- SMT32 is no longer available, let's discuss alternative models
- Robotics (Stanford University Open Course)
- [Anxinke UWB indoor positioning module NodeMCU-BU01] 01: Module appearance interface, onboard resources and power supply characteristics
- ESD204 surge protection for HDMI interface
- Last day! Pre-register to win a JD card! 60 cards in total! Rohm live broadcast: How to use laser diodes well
- Based in Chengdu, job opening: RF chip test engineer
- CircuitPython 4.0.2 released
- Vicor engineers take you through agricultural drone power solutions
- Analysis of previous "Instrumentation" competition topics
- Hard work pays off? TMS320F28379D debugging experience