Instantaneous water heater program MCU AT89C51 XAL 12MHz
//#pragma SRC
#include
#include
#include
void delay(unsigned int); //Delay function
void display(void); //display functionunsigned
char keysCAN(void); //key scan processing functionvoid
heatCTRl(void); //heating control functionvoid
temptest(void); //temperature measurement functionsbit
swkey=P1^0; //switch keysbit
upkey=P1^1; //heating gear "+" keysbit
downkey=P1^2; //heating gear "-" keysbit
buzz=P1^05; //buzzer output
terminalsbit triac=P1^6; //thyristor trigger signal output terminalsbit
relay=P1^7; //relay control signal output terminalsbit
LED1=P2^5; //heating gear indicator light 1
sbit led2=P2^6; //heating gear indicator light 2
sbit led3=P2^7; //heating gear indicator light 3
signed char data ctemp; //current measured water temperature
registerunsigned char data dispram[2]={0x10,0x10}; //display area
cacheunsigned char data heatpower,px0count; //Heating gear register, external interrupt 0 counter
bit tempov,t0tst,testok; //Over-temperature flag, temperature measurement start flag, temperature measurement completion flag
/*----------------------------------------------
Main function void main(void)
No parameters, no return valueCyclic
call display, key scan, temperature detection, heating control function
----------------------------------------------*/
void main(void)
{
unsigned char i,j;
ctemp=15; //Initialize water temperature register
heatpower=5; //Initialize heating gear to 5 when
tempov=0; //Clear over-temperature flag
swkey=0; //Default switch key is pressed, enter standby state
TMOD=0x11; //Set T0 and T1 working mode as 16-bit timer
TCON=0x05; //Set external interrupt 0 and 1 to falling edge trigger
IP=0x01; //Set external interrupt 0 priority
IE=0x80; //Open total interruptwhile
(1)
{
i=1;
do{
for (j=0;j<100;j++) //Loop 100 times, about 0.5s
{
if (keyscan()) i=6; //If a key is pressed, display the current gear for 3s
display(); //Call the display function once for about 4ms
heatctrl(); //Call the heating control function
}//end for (b=0;b<100;b++)
temptest(); //Measure the temperature every 0.5s
} while (--i); //Determine whether to refresh the display by changing the size of the number of loops i
j=abs(ctemp); //Get the absolute value of the temperature
dispram[1]=j%10; //Get the ones digit and send it to the display
j/=10; //Get the tens digit
dispram[0]=j?j:0x11; //Send it to the display (with zero extinguishing)
}//end while (1)
}
/*--------------------------------------
Delay function void delay(unsigned int dt)
Parameter: dt, no return value
Delay time = dt*500 machine cycles
--------------------------------------*/
void delay(unsigned int dt)
{
register unsigned char bt; //define register variable
for (; dt; dt--)
for (bt=250; --bt; ); //This sentence is implemented as "DJNZ" when compiled, 250*2=500 machine cycles
}
/*--------------------------------------
Display function void display(void)
no parameters, no return value
two-digit common anode digital tube scanning display
--------------------------------------*/
void display(void)
{
unsigned char code table[]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90,\
0x88,0x83,0xc6,0xa1,0x86,0x8e,0xbf,0xff};
unsigned char i,a;
a=0xfe; //bit selection initial value
for (i=0; i<2; i++) //loop scan two-digit digital tube
{
P2|=0x1f; //clear bit selection
P0=table[dispram[i]]; //send display segment code
P2&=a; //select one bit
delay(4); //delay 2ms
a=_crol_(a,1); //Change the bit selection word
P0=0xff; //Eliminate
}
}
/*----------------------------------------------------------
Key scan processing function unsigned char keyscan(void)
No parameters, return value: unsigned character type, 0 if no key is pressed, other if a key is pressed
Affects global variables: heatpower
----------------------------------------------------------*/
unsigned char keyscan(void)
{
unsigned char i,ch;
if (upkey==0) //"+" key
{
buzz=0; //Turn on the buzzer (make a key sound)
for (i=0;i<5;i++) display(); //Delay debounce
buzz=1; //Turn off the buzzerif
(heatpower<9) heatpower++; //Increase the gear position
dispram[0]=0;
dispram[1]=heatpower; //Display the current gearwhile
(upkey==0) display(); //Wait for the key to be releasedreturn
(1); //Return if a key is pressed
}
else if (downkey==0) //"-" key
{
buzz=0; //Turn on the buzzer (sound of a button press)
for (i=0;i<5;i++) display(); //Delay to eliminate bounce
buzz=1; //Turn off the buzzer
if (heatpower>0) heatpower--; //Minus one gear
dispram[0]=0;
dispram[1]=heatpower; //Display the current gear
while (downkey==0) display(); //Wait for the key to be released
return (2); //Return that a key has been pressed
}
else if (swkey==0) //Switch key
{
buzz=0; //Turn on the buzzer (sound of a button press)
for (i=0;i<30;i++) display(); //Delay to eliminate bounce
buzz=1; //Turn off the buzzer
swkey=1; //Set the switch key
while (swkey==0) display(); //Wait for the key to be released
ch=IE; //Temporarily store the interrupt control word IE
IE=0x00; //Disable interrupts
P0=0xff;
P1=0xff;
P2=0xff; //Clear port output
dispram[0]=0x10;
dispram[1]=0x10; //Display "--"
display();
while (1)
{
while (swkey) display(); //Wait for the key to be pressedbuzz
=0; //Turn on the buzzer (make a key sound)
for (i=0;i<10;i++) display();//Delay debouncebuzz
=1; //Turn off the buzzerif
(swkey==0) break; //Confirm that the key is pressed
}
while (swkey==0) display(); //Wait for the key to be releasedIE
=ch; //Restore the interrupt control word IE
return (0); //Return that no key is pressed
}
else return (0); //Return when no key is pressed
}
/*--------------------------------------
Heating control function void heatctrl(void)
No parameters, no return valueJudge
whether to heat, heating power and gear indicator light processing
--------------------------------------*/
void heatctrl(void)
{
if (!tempov) //When there is no over-temperature mark
{
relay=0; //Turn on the relaybuzz
=1; //Turn off the buzzerswitch
(heatpower) //Judge the heating gear
{
case 0: {EX1=0;ET1=0;triac=1;led1=1;led2=1;led3=1;break;}//0 gear does not heat, indicator light is not on
case 1:
case 2:
case 3:
case 4: {led1=0;led2=1;led3=1;EX1=1;break;} //1~4 gear No. 1 indicator is on
case 5:
case 6:
case 7:
case 8: {led1=0;led2=0;led3=1;EX1=1;break;} //5~8 gear No. 1, No. 2 indicator lights are on
case 9: {EX1=0;ET1=0;led1=0;led2=0;led3=0;triac=0;break;} //9 gear full power, indicator lights are all on
}
}
else //When there is an over-temperature mark
{
relay=1; //Disconnect the relayEX1
=0; ET1=0; triac=1; //Turn off the thyristorbuzz
=0; //Buzz alarm
}
}
/*--------------------------------------
Temperature measurement function void temptest(void)
has no parameters, no return value,
affects global variables: ctemp, tempov
measures and calculates the temperature through table lookup to determine whether it is over-temperature
--------------------------------------*/
void temptest(void)
{
signed char temp,tempmin,tempmax;
unsigned int t0rig;
unsigned int code temptab[]={0x6262,0x61eb,0x6171,0x60f7,0x6047,0x5ff7,0x5f6e,0x5eef,0x5e53,0x5dbe,0x5d4b,0x5ca5,0x5c17,\
0x5b6b,0x5ada,0x5a5c,0x599b,0x58ff,0x5869,0x57b0,0x570d,0x5663,0x55c6,0x550e,0x5444,0x5396,\
0x52dd,0x5240,0x5189,0x50b0,0x500 5,0x4f20,0x4e69,0x4db1,0x4cef,0x4c42,0x4b64,0x4aaa,0x49e1,\
0x48fc,0x4847,0x476c,0x46b1,0x4604,0x4503,0x4449,0x4356,0x4299,0x41c0,0x40ce,0x3ff0,0x3f2b,\
0x3e33,0x3d86,0x3ca6,0x3bd2,0x3b 26,0x3a39,0x3973,0x38a6,0x37ef,0x373f,0x3687,0x35c3,0x3507,\
0x3487,0x33bc,0x32ed,0x324f,0x319e,0x3106,0x3053,0x2fa6,0x2f2a,0x2e88,0x2e00,0x2d63,0x2cd6,\
0x2c65,0x2bae,0x2b28,0x2a97,0x2a 07,0x298e,0x2914,0x287a,0x280d,0x278a,0x2703,0x2687 ,0x2626,\
0x25e5,0x256d,0x24ee,0x2489,0x2414,0x23bc,0x2356,0x22d9,0x2278,0x2203}; //Temperature frequency table
px0count=2; //Frequency measurement interrupt function parameter
t0tst=1; //Set frequency measurement program start flag
EX0 =1; //Open the frequency measurement external interrupt
testok=0; //Clear the frequency measurement program completion flag
while (!testok) display(); //Wait for the test to complete
t0rig=(unsigned int)TH0<<8|TL0; / /byte synthesis word
tempmin=0; //The following is the binary table lookup method to calculate the temperature value
tempmax=100; //tempmin and tempmax are the range of the temperature table
while (1)
{
temp=(tempmax+tempmin)/2; / /Assume that the current temperature is the midpoint between the maximum and minimum values
if (t0rig==temptab[temp]) break; //If the actual value is equal to the assumed value, end the search
else if (t0rig>temptab[temp]) tempmax=temp; //If the actual value is greater than the assumed value, reduce the maximum value of the search range
else tempmin=temp; //If the actual value If the search range is smaller than the assumed value, increase the minimum value of the search range
if (tempmax-tempmin<=1) //If the search range has been narrowed to 1 degree,
{ //Judge which endpoint the actual value is closer to
if (temptab[tempmax]+ temptab[tempmin]>2*t0rig) temp=tempmax;//Close to the maximum value, take the maximum value
else temp=tempmin; //Close to the minimum value, take the minimum value
break; //End the search
}
}
ctemp=temp; //Refresh the current Temperature register
if (temp>65) tempov=1; //If the temperature exceeds 65 degrees, set the over-temperature flag
else if (temp<45) tempov=0; //When the temperature drops below 45 degrees, clear the over-temperature flag
}
/*------------------------------------------
Temperature measurement frequency test function void tempFrequency(void)
uses external X0 interrupt and register bank 1
to measure the temperature - the frequency of the frequency conversion circuit
---------------------------- --------------*/
void tempfrequency(void) interrupt 0 using 1
{
if (--px0count) return; //Find the starting point or count
if (t0tst) //If it is the starting point
{
t0tst=0; //Clear the frequency measurement start flag
px0count=100; //Take 100 square waves as one frequency measurement
TH0=0;
TL0=0; //Clear timer T0
TR0=1; //Start timing
}
else //If it is the end point
{
TR0=0; //Stop timing
EX0=0; //Stop frequency measurement external interrupt
testok=1; //Set frequency measurement completion flag
}
}
/*--------- -----------------------------
Heating control over-zero detection function void pass0(void)
uses external X1 interrupt, register group 2
detects over 0 o'clock, assign initial value to timer T1
--------------------------------------*/
void pass0(void) interrupt 2 using 2
{
unsigned char code powertab[]={0xd8,0xf0,0xe2,0x63,0xe5,0x25,0xe8,0x3e,0xeb,0x16,0xed,0xda,0xf0,0xb2,0xf3,0xcb,0xf7,0x8d,0xf7,0x8d}; //10 power levels of thyristor conduction angle delay parameter table
TH1=powertab[2*heatpower]-1;
TL1=powertab[2*heatpower+1]; //After the mains passes zero, assign delay parameters to timer T1 according to the currently set gear
ET1=1; //Allow timer T1 interrupt
TR1=1; //Turn on timer T1
}
/*------------------------------------------
thyristor trigger signal control function void tria CC trl(void)
uses timer T1 interrupt, register group 3
sends trigger signal to thyristor
------------------------------------------*/
void triacctrl(void) interrupt 3 using 3
{
register unsigned char i;
triac=0; //output thyristor conduction signal
ET1=0; //turn off timer T1 interrupt
TR1=0; //stop timer operation
for (i=0;i<2;i++); //delay to ensure that the conduction signal has enough width
triac=1; //complete thyristor conduction signal
}
Previous article:AT89C52 single chip microcomputer controls BH1415F FM station C program
Next article:AT89C2051 simple sine wave, triangle wave, square wave generator
Recommended ReadingLatest update time:2024-11-16 12:49
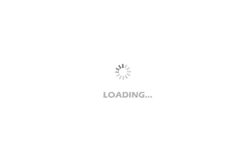
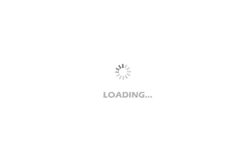
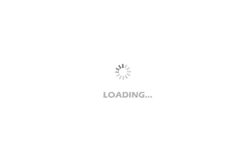
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Recommended development directory for CH579M
- Free gifts! 11 popular Maxim development boards are waiting for you!
- Have you been affected by the price increase? Share the best pin-compatible alternative solution under the wave of price increase#, a certain brand?
- Request: DC-DC small package chip similar to LM2596
- What is the reason! Another wind turbine fire accident! And caused a ground wildfire
- 【Iprober 520 current probe】Practical use in motor control
- Can you please help me find out where is the problem with the program?
- How to add c files in esp32 idf components to compile
- Have you noticed the LPDSP32 audio codec inside RSL10? Does your project use this resource?
- Will the push-pull circuit be straight through?