1. ATMEGA128 Data Sheet
Watchdog Timer
The watchdog timer is driven by an independent 1 MHz on-chip oscillator. This is a typical value at VCC = 5V. See the characteristic data for typical values at other VCC levels. The time interval of the watchdog reset can be adjusted by setting the prescaler of the watchdog timer, as shown in Table 22 on P53. The watchdog reset instruction WDR is used to reset the watchdog timer. In addition, the timer is reset when the watchdog timer is disabled or a reset occurs. There are 8 options for the reset time. If the timer is not reset in time, once the time exceeds the reset period, the ATmega128 is reset and executes the program pointed to by the reset vector. The specific watchdog reset timing is described on P50.
To prevent the watchdog timer from being accidentally disabled or the reset time changed, the chip provides three different protection levels based on the fuse bits M103C and WDTON, as shown in Table 21. Security level 0 corresponds to the ATmega103 settings. Enabling the watchdog timer has no restrictions. Please refer to P 54 “Changing the timing sequence of the watchdog timer configuration”.
Watchdog Timer Control Register - WDTCR
• Bits 7..5 – Res: Reserved.
Reserved bits. A read operation returns a value of zero.
• Bit 4 – WDCE: Watchdog Modification Enable
When clearing WDE, WDCE must be set first, otherwise the watchdog cannot be disabled. Once set, the hardware will clear it after the next 4 clock cycles. Please refer to the description of WDE to disable the watchdog. WDCE must also be set to modify the prescaler data when operating in security levels 1 and 2, as shown in P 54 “Time sequence for changing the watchdog timer configuration”.
• Bit 3 – WDE: Watchdog Enable
When WDE is "1", the watchdog is enabled, otherwise the watchdog will be disabled. WDE can only be cleared when WDCE is "1". The following are the steps to turn off the watchdog:
1. Write "1" to WDCE and WDE in the same instruction, even if WDE is already "1".
2. Write "0" to WDE within the next 4 clock cycles.
The watchdog timer can never be disabled when working at security level 2. Refer to P 54 "Timing sequence for changing the watchdog timer configuration".
• Bits 2..0 – WDP2, WDP1, WDP0: Watchdog Timer Prescaler 2, 1, and 0
WDP2, WDP1, and WDP0 determine the prescaler for the Watchdog Timer as shown in Table 22.
The following examples implement the operation of turning off the WDT in assembly and C. It is assumed that the interrupt is under user control (for example, global interrupt is disabled), so the interrupt will not occur when executing the following program.
Assembly code examples |
WDT_off: ; Set WDCE and WDE ldi r16, (1< ; Turn off WDT ldi r16, (0< ret |
C code example |
void WDT_off(void) |
Watchdog initialization routine
C code example |
void WDT_Init(void) { _WDR();//reset watchdog timer } |
Changing the watchdog timer configuration timing sequence
The sequence for changing the configuration varies slightly depending on the security level. We will explain each one below.
Security Level 0
This mode is compatible with the watchdog operation of the ATmega103. The initial state of the watchdog is disabled. You can enable it without restriction by setting WDE and changing the timer overflow period. When disabling the watchdog timer, you need to follow the instructions about WDE.
Security Level 1
In this mode, the watchdog timer is initially disabled and can be enabled without restriction by setting WDE (e.g., WDTCR |= (1<
2. Writing "0" to WDE and appropriate data to WDP simultaneously within the next 4 clock cycles, while writing "0" to WDCE.
Security Level 2
In this mode, the watchdog timer is always enabled and the read return value of WDE is "1". Changing the timer overflow period requires executing a specific timing sequence:
1. Write "1" to WDCE and WDE in the same instruction. Although WDE is always set, "1" must be written to start the timing.
2. Write "0" to WDCE and write appropriate data to WDP simultaneously within the next 4 clock cycles. The value of WDE can be arbitrary.
2. Watchdog AVR-GCC routine
Header file: #include, including the watchdog reset command _WDR();
avr-libc provides three APIs to support the operation of the internal Watchdog of the device, namely:
wdt_reset() // Watchdog reset
wdt_enable(timeout) // Watchdog enable
wdt_disable() // Watchdog disable
Before calling the above functions, you must include the header file wdt.h. wdt.h also defines the Watchdog timer timeout symbol constants, which are used to provide timeout values for the wdt_enable function. The symbolic constants are as follows:
Symbolic constant Value Meaning
WDTO_15MS Watchdog timer 15 milliseconds timeout
WDTO_30MS Watchdog timer 30 milliseconds timeout
WDTO_60MS Watchdog timer 60 milliseconds timeout
WDTO_120MS Watchdog timer 120 milliseconds timeout
WDTO_250MS Watchdog timer 250 milliseconds timeout
WDTO_500MS Watchdog timer 500 milliseconds timeout
WDTO_1S Watchdog timer 1 second timeout
WDTO_2S Watchdog timer 2 second timeout
Watchdog test program:
When executing this program on CA-M8, S1-8 (LEDY) should be turned on to connect the yellow LED to the PB0 pin.
#include
#include
#include
#define uchar unsigned char
#define uint unsigned int
#define SET_LED PORTB&=0XFE //PB0 connected to the yellow LED
#define CLR_LED PORTB|=0X01
//1ms delay so the error will not be too big function
void DelayMs(uint ms)
{
uint i;
for(i=0;i
_delay_loop_2(4 *250);
}
int main(void)
{
DDRB=_BV(PB0);
PORTB=_BV(PB0); //CLR_LED
//WDT counter synchronization is one second
wdt_enable(WDTO_1S);
wdt_reset();//Feed the dog
DelayMs(500);
SET_LED;
//Wait for the dog to starve to death
DelayMs(5000);
SET_LED;
while(1)
wdt_reset();
}
Execution result:
The yellow LED on CA-M8 keeps flashing, proving that Watchdog makes the MCU reset continuously.
3. Notes
If the time of each cycle in the loop body does not exceed the reset time of the watchdog, it is sufficient to feed the dog once, otherwise it needs to be fed multiple times.
Previous article:12864 LCD display picture-CVAVR program
Next article:Questions about using internal pull-up resistors in AVR microcontrollers
Recommended ReadingLatest update time:2024-11-16 13:01
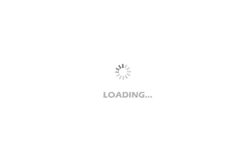
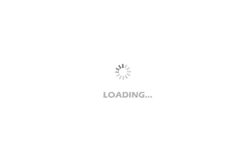
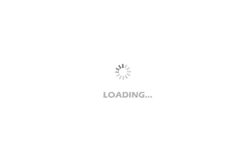
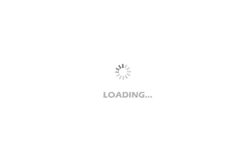
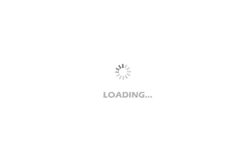
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- RISC-V MCU Application Development Series Tutorial CH32V103
- Let's take a look
- 【TI Wireless】Micro Dual-Mode Wireless Receiver
- Let’s talk about whether Huawei can survive in the end.
- Can the network cable be directly soldered to the PCB without a crystal plug or can the network cable be plugged into the circuit board with terminals?
- [Revenge RVB2601 creative application development] helloworld_beginner's guide to debugging methods and processes
- What changes will occur if RFID technology is applied to clothing production?
- EEWORLD University Hall----30 Case Studies of MATLAB Intelligent Algorithms
- Flyback Power Supply Magnetic Core Calculation Method
- Is there any way to directly give the calculation equation and related solutions based on the electrical parameters of a given circuit and related components?