3. ATMEGA16 drives 16*2 dot matrix character LCD
3. (01) ATMEGA16 drives 16*2 dot matrix character LCD
This article is just a simple driver to make 1602 display, and does not use the part of reading data and reading status, nor does it use read and write detection (if you are interested, you can still write it here)
1. What kind of LCD should be used and how to drive it? Now I will post some pictures of the datasheet. It is so easy~~
1) Its pin description (I personally feel that if you want to know more, you can search it on the Internet~~ I just have a simple understanding here~)
There are only three pins that need attention here, RS, R/W, and E. These three pins are necessary for transmitting data. There is no need to introduce the others in detail~~ (The following program is written based on the timing diagram of these three pins!
2) (To be honest, I really don't want to go into detail, because many people think driving this 1602 is too simple~~ so I try to be concise) Basic operation timing
1 Read status: Input: RS=L, RW=H, E=H Output: D0~D7=status word
2 Write command: Input: RS=L, RW=L, D0~D7=command code, E=H Output: None
3 Read data: Input: RS=H, RW=H, E=OK Output: D0~D7=data
4 Write data: Input: RS=H, RW=L, D0~D7=data, E=high pulse Output: None
Note that since we don’t focus on reading, the important thing to look at above is the write timing and the timing parameters! ! ! !
(I don't need to explain too much here, but please note that 1602 is used to display data. Those functions such as read status and read data are not used for the time being, so I will not introduce them here for the time being. So, let's just use write instructions and write data directly. If you look closely, you will find that there is only one difference between write instructions and write data~~~~ that is, RS is low for write instructions; otherwise~~~)
3) Status word description
The read/write detection mentioned later is not needed for the time being, that's because we give it a delay, but if you want to use it, then take a good look at the datasheet~~~~ (To be honest: I don't know how to use the status word yet, if I do, I'll post back later~~haha)
4) RAM address map
5) Instruction description (this is the key point~~ you can find the above as long as you find the datasheet~~~ ah~~ so annoying )
5.1 Initialization settings:
5.1.1 Display mode settings:
Just write instruction 0x38
5.1.2 Display switch and cursor setting
This is very simple. I have also watched teacher Guo Tianxiang's teaching video~~He used this to explain~~Hehe
5.2 Data Control (I’m so tired, I really don’t want to write anymore, just post the pictures~~)
The read data and write data in the picture are the read timing and write timing. They are all above, so I won’t post them~~
Okay, I simulated the picture
Finally, the program~~
//------------------------------------------------------------------------------
//LCD1602 display program
#include "ioavr.h"
#include "intrinsics.h"
//------------------------------------------------------------------------------
typedef unsigned char uchar;
typedef unsigned int uint;
//------------------------------------------------------------------------------
//RS, RW, EN pin output high and low level macro definition
#define lcd_rs_1 PORTB|=1
#define lcd_rs_0 PORTB&=~1
#define lcd_rw_1 PORTB|=2
#define lcd_rw_0 PORTB&=~2
#define lcd_en_1 PORTB|=4
#define lcd_en_0 PORTB&=~4
//------------------------------------------------------------------------------
#define data_port PORTA
#define busy 0x80
#define xtal 8
//------------------------------------------------------------------------------
uchar __flash str0[]={"This is a LCD-!"};
uchar __flash str1[]={"Designed by ME"};
//------------------------------------------------------------------------------
//Delay 1ms function
void delay_1ms()
{
uint i;
for(i=1;i<(uint)(xtal*143-2);i++);
}
//------------------------------------------------------------------------------
//Delay nms function
void delay_nms(uint n)
{
uint i=0;
while(i
delay_1ms();
i++;
}
}
//------------------------------------------------------------------------------
//LCD write data function
void lcd_write_data(uchar dat)
{
lcd_rs_1;
lcd_rw_0;
data_port=dat;
delay_1ms();
lcd_en_1;
delay_1ms();
lcd_en_0;
}
//------------------------------------------------------------------------------
//LCD write command function
void lcd_write_command(uchar com)
{
lcd_rs_0;
lcd_rw_0;
data_port=com;
delay_1ms();
lcd_en_1;
delay_1ms();
lcd_en_0;
}
//------------------------------------------------------------------------------
//LCD initialization function
void lcd_init()
{
lcd_write_command(0x01);
delay_1ms();
lcd_write_command(0x38);
delay_1ms();
lcd_write_command(0x0c);
delay_1ms();
lcd_write_command(0x06);
delay_1ms();
}
//------------------------------------------------------------------------------
//LCD display function
void lcd_display()
{
uint num;
lcd_write_command(0x80);
for(num=0;num<16;num++)
{
lcd_write_data(str0[num]);
delay_1ms();
}
lcd_write_command(0x80+0x40);
for(num=0;num<16;num++)
{
lcd_write_data(str1[num]);
delay_1ms();
}
}
//------------------------------------------------------------------------------
//main
void main()
{
delay_nms(100);
DDRA=0XFF;
PORTA=0X00;
DDRB=0XFF;
PORTB=0X00;
lcd_init();
lcd_display();
while(1);
}
Previous article:AVR microcontroller (learning) - (IV), ATMEGA16 timer/counter - 01
Next article:AVR microcontroller (learning) - (II), ATMEGA16 interrupt system - 02
Recommended ReadingLatest update time:2024-11-16 18:09
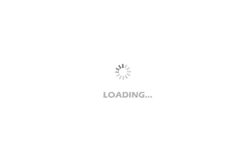
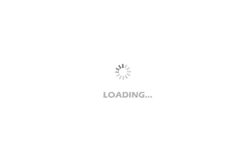
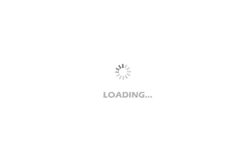
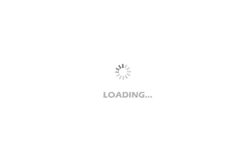
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Verilog007
- The future belongs to ultra-wideband (UWB)
- Zhongke Bluesun (AB32VG1) development board (based on RT-Thread system) --- Music player -- Sharing
- What exactly is a sine filter?
- Pingtouge RVB2601 board-GPIO
- [TI recommended course] #DC/DC switching regulator packaging innovation#
- 【AT-START-F425 Review】Reading USB files to achieve image reproduction
- MSP430 MCU Program Upgrade Example
- Looking for open source enthusiasts to improve the BabyOS open source project
- Can experienced friends recommend some useful power modules?