To correctly execute the external interrupt of 2440, generally two parts need to be completed: interrupt initialization and interrupt processing function.
Before executing the interrupt, the interrupt to be used must be initialized. The external interrupt pin EINT of 2440 is multiplexed with the general IO pins F and G. To use the interrupt function, the corresponding pin must be configured to interrupt mode. For example, if we want to set port F0 to external interrupt, while the functions of other pins remain unchanged, then GPFCON=(GPFCON & ~0x3) | 0x2. After configuring the pin, you also need to configure the specific interrupt function. We need to open the mask of a certain interrupt so that we can respond to the interrupt. The corresponding register is INTMSK; we also need to set the trigger mode of the external interrupt, such as low level, high level, rising edge, falling edge, etc. The corresponding register is EXTINTn. In addition, since EINT4 to EINT7 share an interrupt vector, EINT8 to EINT23 also share an interrupt vector, and INTMSK is only responsible for the mask of the total interrupt vector, to specifically open a specific interrupt mask, you also need to set EINTMASK. The above is the most basic initialization. Of course, there are some other configurations, such as when fast interrupts are needed, INTMOD should be used, and when interrupt priority needs to be configured, PRIORITY should be used. The
interrupt handling function is responsible for executing specific interrupt instructions. In addition, the corresponding bits in SRCPND and INTPND need to be cleared (cleared by setting 1), because when an interrupt occurs, 2440 will automatically set the corresponding positions in these two registers to 1 to indicate that an interrupt has occurred. If they are not cleared in the interrupt handling function, the system will always execute the interrupt function. In addition, as mentioned earlier, some interrupts share an interrupt vector, and an interrupt vector can only have one interrupt execution function. Therefore, EINTPEND is needed to determine which external interrupt it is, and the corresponding bits need to be cleared by setting 1. Generally speaking, the keyword __irq is used to define the interrupt handling function, so that the system will automatically save some necessary variables for us and can return correctly after the interrupt handling function is executed. It should also be noted that the interrupt handling function cannot have a return value or pass any parameters.
In order to match this interrupt handling function with the interrupt vector table defined in the 2440 startup file, it is necessary to define the interrupt entry address variable first. The interrupt entry address must be consistent with the address in the interrupt vector table, and then pass the first address of the interrupt handling function to the variable, that is, the interrupt entry address.
The following is an example of an external interrupt. There are four buttons on the development board, connected to EINT0, EINT1, EINT2 and EINT4 respectively. We let these four buttons control the four LEDs connected to the B5~B8 pins respectively: press the button once to turn on the LED, press it again to turn it off:
#define _ISR_STARTADDRESS 0x33ffff00
#define U32 unsigned int
#define pISR_EINT0 (*(unsigned *)(_ISR_STARTADDRESS+0x20))
#define pISR_EINT1 (*(unsigned *)(_ISR_STARTADDRESS+0x24))
#define pISR_EINT2 (*(unsigned *)(_ISR_STARTADDRESS+0x28))
#define pISR_EINT4_7 (*(unsigned *)(_ISR_STARTADDRESS+0x30))
#define rSRCPND (*(volatile unsigned *)0x4a000000) //Interrupt request status
#define rINTMSK (*(volatile unsigned *)0x4a000008) //Interrupt mask control
#define rINTPND (*(volatile unsigned *)0x4a000010) //Interrupt request status
#define rGPBCON (*(volatile unsigned *)0x56000010) //Port B control
#define r GPBDAT (*(volatile unsigned *)0x56000014) //Port B data
#define rGPBUP (*(volatile unsigned *)0x56000018) //Pull-up control B
#define rGPFCON (*(volatile unsigned *)0x56000050) //Port F control
#define rEXTINT0 (*(volatile unsigned *)0x56000 088) //External interrupt control register 0
#define rEINTMASK (*(volatile unsigned *)0x560000a4) //External interrupt mask
#define rEINTPEND (*(volatile unsigned *)0x560000a8) //External interrupt pending
static void __irq Key1_ISR(void) //EINT1
{
int led;
rSRCPND = rSRCPND | (0x1<<1);
rINTPND = rINT PND | (0x1<<1);
led = rGPBDAT & (0x1<<5);
if (led ==0)
rGPBDAT = rGPBDAT | (0x1<<5);
else
rGPBDAT = rGPBDAT & ~(0x1<<5);
}
static void __irq Key2_ISR(void) //EINT4
{
int led;
rSRCPND = rSRCPND | (0x1<<4);
rINTPND = rINTPND | (0x1<<4);
if(rEINTPEND&(1<<4))
{
rEINTPEND = rEINTPEND | (0x1<<4);
led = rGPBDAT & (0x1<<6);
if (led ==0 )
rGPBDAT = rGPBDAT | (0x1<<6);
else
rGPBDAT = rGPBDAT & ~(0x1<<6);
}
}
static void __irq Key3_ISR(void) //EINT2
{
int led;
rSRCPND = rSRCPND | (0x1<<2 );
rINTPND = rINTPND | (0x1<<2);
led = rGPBDAT & (0x1<<7);
if (led ==0)
rGPBDAT = rGPBDAT | (0x1<<7);
else
rGPBDAT = rGPBDAT & ~(0x1 <<7);
}
void __irq Key4_ISR(void) //EINT0
{
int led;
rSRCPND = rSRCPND | 0x1;
rINTPND = rINTPND | 0x1;
led = rGPBDAT & (0x1<<8);
if (led ==0)
rGPBDAT = rGPBDAT | (0x1<<8 );
else
rGPBDAT = rGPBDAT & ~(0x1<<8);
}
void Main(void)
{
int light;
rGPBCON = 0x015550;
rGPBUP = 0x7ff;
rGPFCON = 0xaaaa;
rSRCPND = 0x17;
rINTMSK = ~0x17;
rINTPND =0x17;
rEINTPEND = (1<<4);
rEINTMASK = ~(1<<4);
rEXTINT0 = 0x20222;
light = 0x0;
rGPBDAT = ~light;
pISR_EINT0 = (U32)Key4_ISR;
pISR_EINT1 = (U32)Key1_ISR; pISR_EINT2
= (U32)Key3_ISR;
pISR_EINT4_7 = (U32)Key2_ISR;
while(1)
;
}
Previous article:Detailed explanation of LCD application of s3c2440
Next article:ARM interrupt function definition
Recommended ReadingLatest update time:2024-11-16 16:32
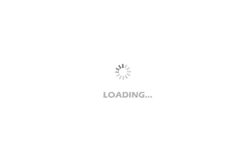
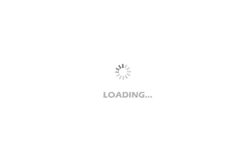
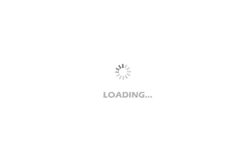
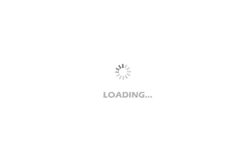
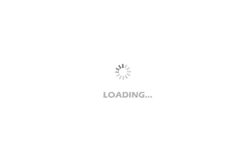
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- ST MEMS waterproof pressure sensor LPS27HHW review summary
- The problem of not receiving data when building lorawan gateway with Raspberry Pi
- Two questions about registers
- UWB, Bluetooth, RFID, WIFI and indoor positioning technology
- 【micropython】NUCLEO_L073RZ and NUCLEO_L452RE have been added to the firmware library
- [NUCLEO-L552ZE Review] + Low Power Measurement Tool
- Lithium battery charging and discharging integrated chip
- Ethernet LED is not on
- AM57x Processors for Smart Grid Applications
- The pinout has changed, where should I modify?