There are 8 banks in S3C2440, each with an address space of 128MB, for a total of 1GB. The data width of bank0 is selected by hardware, and the others can be changed by setting the corresponding registers. Bank0-bank5 supports external ROM and SRAM, and bank6 and bank7 also support SDRAM (i.e. the memory on the development board), and the address space size of bank6 and bank7 is variable.
S3C2440 leads out 27 address lines ADDR0-ADDR26, accessing 128MB of space. It also leads out nGCS0-nGCS7, corresponding to bank0-bank7. When accessing the corresponding bank, the corresponding nGCSx outputs a low level to select the peripheral. The address distribution diagram is as follows.
Steppingstone is the 4KB RAM inside the CPU. When the CPU is powered on and the hardware chooses to boot from Nand flash, the hardware will copy the first 4KB of data in Nand flash to Steppingstone, starting at address 0.
Using SDRAM:
SDRAM needs to be connected to bank6 or bank7. The SDRAM used on the development board is HY57V561620, which consists of 4 logical banks (L-banks), each bank has 4M, and the data width is 16 bits. So its capacity is 4*4MB*2B=32MB. The development board has two SDRAMs. The data width is 32 bits, so the capacity is 64MB. The connection is as shown:
Because the total capacity of the SDRAM on the board is 64MB, 26 address lines are used. The last two bits LADDR24 and LADDR25 are connected to BA0 and BA1 of the SDRAM to select the logical bank of the SDRAM. Because the data width is 32 bits, the lower two bits LADDR0 and LADDR1 are not used. The chip select signal nSCS of the SDRAM is connected to nGCS6:nSCS0 of the CPU, so Bank6 is used.
The steps for accessing SDRAM are roughly as follows:
1. The CPU sends a chip select signal nSCS that is valid and SDRAM is selected.
2. LADDR24 and LADDR25, select the L-bank in SDRAM.
3. Perform row and column addressing on the selected chip.
4. The data of the found storage unit is transferred to the data bus.
These operations are all performed automatically by the CPU when the corresponding registers are set and the memory is accessed. The CPU can automatically separate the L-bank, row, and column addresses according to the column address bits and memory size information set in the registers, and send them to the SDRAM in the corresponding timing.
Register introduction:
The memory controller has 13 registers in total. Only BWSCON and BANKCONx need to be set for bank0-bank5. Other registers need to be set when bank6 and bank7 are connected to external SDRAM. The following is an introduction to each register.
BWSCON (Bus width & Wait CONtrol register) is a bit width and wait register. This register sets the bit width of each bank, where each 4 bits controls one bank. According to the data sheet, each 4 bits
DWx: occupies two bits to set the bit width of bankx, 00 corresponds to 8 bits, 01 corresponds to 16 bits, and 10 corresponds to 32 bits
WSx: occupies one bit, whether to use the WAIT signal, usually 0 is not used
STx: occupies one bit. Whether to enable the SDRAM data mask pin. This bit of SDRAM is 0.
Among them, the more special one is bank0, whose bit width is determined by the hardware pins OM0 and OM1.
BANKCONx (BANK CONtrol register). In the 8 banks, bank 6 and bank 7 can be connected to SDRAM, so BANKCON6 and BANKCON7 are different from 0-5. 0-5 mainly control the access timing of external devices. 6 and 7 have additional,
MT: Used to set this bank to be an external memory type. SRAM/ROM is 00, SDRAM is 11
Trcd: delay from RAS to CAS
SCAN: SDRAM column address number, 00 is 8 bits, 01 is 9 bits, 10 is 10 bits,
REFRESH (refresh control register) Refresh control register, where REFEN 0 is to disable the refresh function, 1 is to enable the refresh function. TREFMD refresh mode, Trp precharge time, Tsrc half line cycle. The remaining 0-10 bits are refresh counters, calculated as 2^11+1-SDRAM clock frequency (MHz)*SDRAM refresh cycle (us). When the 2440 development board does not use PLL, the clock frequency is equal to the crystal frequency of 12MHz, and the calculated value is equal to 1955. The entire register is 0x008C07A3 in this development board
BANKSIZE bank size register, where BURST_EN 0 is to disable burst transfer 1 is to support burst transfer. SCKE_EN enables power-down mode or not, SCLK_EN only sends SCLK signal during access to SDRAM. BK76MAP sets BANK6/7 size. 010 is 128MB 001 is 64MB. In this example, it is 0xb1.
MRSRBx (SDRAM mode register set register) x is 6 and 7. Only CL[6:4] CAS wait time can be set. The entire register is set to 0x30
Experimental code introduction:
@****************************************
@ Set SDRAM to download to nandflash and transfer to internal
@ The program in SRAM is copied to SDRAM and then jumps to SDRAM
@ implement
@****************************************
.equ MEM_CTL_BASE, 0x48000000
.equ SDRAM_BASE, 0x30000000
.equ WTCON, 0x53000000
.text
.global _start
_start:
bl disable_watchdog
bl init_sdram
bl move_to_sdram
ldr pc, =jump_to_main
disable_watchdog:
ldr r1, =WTCON
mov r2, #0x0
str r2, [r1]
mov pc, lr
init_sdram:
ldr r1, =MEM_CTL_BASE
adrl r2, mem_cfg_val
add r3, r1,#52 @4*13 = 52
0: @Number labels are local labels, which can appear multiple times in different areas.
ldr r4, [r2],#4
str r4, [r1],#4
cmp r1, r3
bne 0b
mov pc, lr
move_to_sdram:
mov r1, #0;
ldr r2, =SDRAM_BASE
add r3, r1,#4*1024
0:
ldr r4, [r1],#4
pg r4, [r2],#4
cmp r2,r3
bne 0b
mov pc, lr
jump_to_main:
ldr sp, =0x34000000
bl main
.align 4
mem_cfg_val:
.word 0x22011110 @BWSCON
.word 0x00000700 @BANKCON0
.word 0x00000700 @BANKCON1
.word 0x00000700 @BANKCON2
.word 0x00000700 @BANKCON3
.word 0x00000700 @BANKCON4
.word 0x00000700 @BANKCON5
.word 0x00018005 @BANKCON6
.word 0x00018005 @BANKCON7
.word 0x008C07A3 @REFRESH
.word 0x000000b1 @BANKSIZE
.word 0x00000030 @MRSRB6
.word 0x00000030 @MRSRB7
The Makefile is as follows:
sdram.bin : head.S leds.c
arm-linux-gcc -c -o head.o head.S
arm-linux-gcc -c -o leds.o leds.c
arm-linux-ld -Ttext 0x30000000 head.o leds.o -o sdram_elf
arm-linux-objcopy -O binary -S sdram_elf sdram.bin
arm-linux-objdump -D -m arm sdram_elf > sdram.dis
clean:
rm -f sdram.dis sdram.bin sdram_elf *.o
Previous article:ARM Notes: SDRAM Memory Driver
Next article:ARM Notes: Detailed explanation of assembly and C language programs 1
Recommended ReadingLatest update time:2024-11-16 13:54
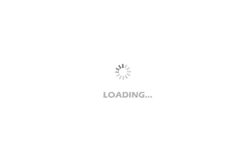
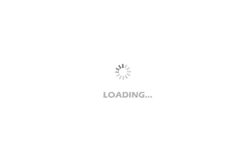
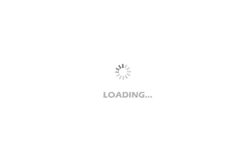
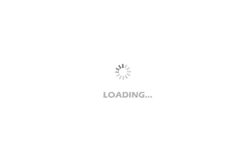
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- The meaning and measurement method of noise
- The experience and IQ of the elderly are not proportional
- Summary of filter reliability test items, a must for designers!
- [GD32E231 DIY Contest] Part 3: Submit videos and documents
- Please make ST's hal library more rigorous!!!
- FeatherSnow can run CircuitPython or Arduino
- Passive high-pass filter circuit has no output
- Will there be any impact if the amplifier circuit is not zeroed? @【Analog Electronics】
- What is the difference between automotive electronics and other electronics?
- Allwinner V853 heterogeneous core impression