1. Read half word (16 bits) function
//Read the half word (16-bit data) of the specified address
//faddr: read address (this address must be a multiple of 2!!)
//Return value: corresponding data.
u16 STMFLASH_ReadHalfWord(u32 faddr)
{
return *(vu16*)faddr;
}
2. Direct writing without checking
// Write without checking
//WriteAddr: starting address
//pBuffer: data pointer
//NumToWrite: half-word (16-bit) number
void STMFLASH_Write_NoCheck(u32 WriteAddr,u16 *pBuffer,u16 NumToWrite)
{
u16 i;
for(i=0;i
{
FLASH_ProgramHalfWord(WriteAddr,pBuffer[i]);
WriteAddr+=2; //Address increases by 2. Write one half word at a time (16-bit number)
}
}
3. Read data of specified length from specified address
//Read data of specified length starting from the specified address
//ReadAddr: starting address
//pBuffer: data pointer
//NumToWrite: half-word (16-bit) number
void STMFLASH_Read(u32 ReadAddr,u16 *pBuffer,u16 NumToRead)
{
u16 i;
for(i=0;i
{
pBuffer[i]=STMFLASH_ReadHalfWord(ReadAddr); //Read 2 bytes.
ReadAddr+=2; //Offset 2 bytes.
}
}
4. Write data of specified length starting from the specified address
Program ideas
//Write data of specified length starting from the specified address
//WriteAddr: starting address (this address must be a multiple of 2!!)
//pBuffer: data pointer
//NumToWrite: half-word (16-bit) number (that is, the number of 16-bit data to be written.)
#if STM32_FLASH_SIZE<256
#define STM_SECTOR_SIZE 1024 // bytes
#else
#define STM_SECTOR_SIZE 2048
#endif
u16 STMFLASH_BUF[STM_SECTOR_SIZE/2]; // up to 2K bytes
void STMFLASH_Write(u32 WriteAddr,u16 *pBuffer,u16 NumToWrite)
{
u32 secpos; //sector address
u16 secoff; //Inside sector offset address (16-bit word calculation)
u16 secremain; // Remaining addresses in the sector (16-bit word calculation)
u16 i;
u32 offaddr; // remove the address after 0X08000000
if(WriteAddr=(STM32_FLASH_BASE+1024*STM32_FLASH_SIZE)))return;//Illegal address, first determine whether the address to be written is an illegal address
FLASH_Unlock(); //Unlock
offaddr=WriteAddr-STM32_FLASH_BASE; //actual offset address.
secpos=offaddr/STM_SECTOR_SIZE; //sector address 0~127 for STM32F103RBT6, determine which page or sector
secoff=(offaddr%STM_SECTOR_SIZE)/2; //Offset within the sector (2 bytes as the basic unit.)
secremain=STM_SECTOR_SIZE/2-secoff; //Sector remaining space size
if(NumToWrite<=secremain)secremain=NumToWrite; //Not larger than the sector range
while(1)
{
STMFLASH_Read(secpos*STM_SECTOR_SIZE+STM32_FLASH_BASE,STMFLASH_BUF,STM_SECTOR_SIZE/2); //Read the contents of the entire sector and read it into STMFLASH_BUF
for(i=0;i
{
if(STMFLASH_BUF[secoff+i]!=0XFFFF)break;//need to erase
}
If (i) the check above is aborted midway, i must be less than secretmain, otherwise if all checks are successful, i should be equal to secretmain.
{
FLASH_ErasePage(secpos*STM_SECTOR_SIZE+STM32_FLASH_BASE);//Erase this sector
for(i=0;i
{
STMFLASH_BUF[i+secoff]=pBuffer[i]; //Write the data to be updated to the array
}
STMFLASH_Write_NoCheck(secpos*STM_SECTOR_SIZE+STM32_FLASH_BASE,STMFLASH_BUF,STM_SECTOR_SIZE/2);//Write the entire sector
}
else STMFLASH_Write_NoCheck(WriteAddr,pBuffer,secremain);//Write what has been erased, directly write to the remaining area of the sector.
if(NumToWrite==secremain)break;//Writing is finished
else //Writing is not finished
{
secpos++; //Increase the sector address by 1
secoff=0; //Offset position is 0
pBuffer+=secremain; //Pointer offset
WriteAddr+=secremain; //write address offset
NumToWrite-=secremain; //Number of bytes (16 bits) decreases
if(NumToWrite>(STM_SECTOR_SIZE/2))secremain=STM_SECTOR_SIZE/2; //The next sector cannot be written completely
else secremain=NumToWrite; //The next sector can be written
}
};
FLASH_Lock(); //Lock
}
Previous article:42.485 Communication Experiment
Next article:43. Flash memory programming principles and steps
Recommended ReadingLatest update time:2024-11-16 22:24
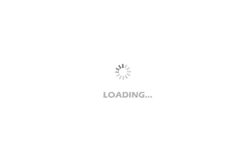
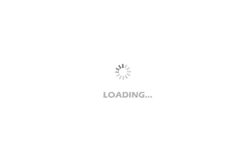
- Popular Resources
- Popular amplifiers
-
Automotive Electronics S32K Series Microcontrollers: Based on ARM Cortex-M4F Core
-
EDA Technology Practical Tutorial--Verilog HDL Edition (Sixth Edition) (Pan Song, Huang Jiye)
-
ARM Embedded System Principles and Applications (Wang Xiaofeng)
-
Deep Understanding of Linux Driver Design (Tsinghua Developer Library) (Wu Guowei, Yao Lin, Bi Chenglong)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 1
- Wireless Earbud Battery Ultra-Low Standby Power Consumption Reference Design
- Fake news and exaggerated hype are hurting China's IC industry. Silence is not the solution (Reprinted)
- TA0CCR0 interrupt of msp430
- What are the 9 most important applications of the Internet of Things (IoT)?
- MOS tube burnt out
- About CS1256 Analog Chip
- At the same frequency, the impedance of a large capacitor is smaller than that of a small capacitor, and it has better filtering performance for high frequencies. Why do we need to connect a small capacitor in parallel?
- [GD32E503 Review] Part 5: FreeRTOS Project Creation
- I heard that ST has released a LoRa development board, NUCLEO-WL55JC2. Why can't I find any information about it anywhere in the world?