1. Experimental Purpose
1) Familiar with STM32 general timer;
2) Use the timer interrupt to flip the LED light.
2. Introduction to STM32 general timer
The general purpose timer is a 16-bit auto-reload counter driven by a programmable prescaler. It is suitable for many applications, including measuring the pulse length of input signals (input capture) or generating output waveforms (output compare and
PWM). Using the timer prescaler and the RCC clock controller prescaler, the pulse length and waveform period can be adjusted from a few microseconds to a few milliseconds. Each timer is completely independent and does not share any resources with each other. They
Can be operated synchronously together.
The STM32F103 has four general-purpose TIMx (TIM2, TIM3, TIM4 and TIM5). Their functions include:
● 16-bit up, down, up/down auto-load counter
● 16-bit programmable (can be modified in real time) prescaler, the counter clock frequency division factor is any value between 1 and 65536
● 4 independent channels:
─ Input capture
─ Output comparison
─ PWM generation (edge or center aligned mode)
─ Single pulse mode output
● Synchronous circuits that use external signals to control timers and interconnect timers
● Interrupt/DMA is generated when the following events occur:
─ Update: counter overflow/underflow, counter initialization (by software or internal/external trigger)
─ Trigger event (counter starts, stops, initializes, or counts by internal/external trigger)
─ Input capture
─ Output comparison
● Supports incremental (quadrature) encoder and Hall sensor circuits for positioning
● Trigger input as external clock or cycle-by-cycle current management
The functions of the STM32 general-purpose timer are very complex. The ones we generally use more are input capture, output comparison, PWM generation and corresponding interrupts.
Main registers: control register 1 (TIMx_CR1), control register 2 (TIMx_CR2), slave mode control register (TIMx_SMCR), DMA/interrupt enable register (TIMx_DIER), status register (TIMx_SR), event generation register (TIMx_EGR), capture/compare mode register 1 (TIMx_CCMR1), capture/compare mode register 2 (TIMx_CCMR2), capture/compare enable register (TIMx_CCER), capture/compare register 1 (TIMx_CCR1), capture/compare register 2 (TIMx_CCR2), capture/compare register 3 (TIMx_CCR3), capture/compare register 4 (TIMx_CCR4), DMA control register (TIMx_DCR), DMA address of continuous mode (TIMx_DMAR), counter register (TIMx_CNT), prescaler register (TIMx_PSC), automatic load register (TIMx_ARR).The time base unit contains:
● Counter register (TIMx_CNT)
● Prescaler register (TIMx_PSC)
● Automatic reload register (TIMx_ARR)
Configuration steps:
1) Turn on TIM3 clock and multiplexed function clock, and configure PB5 as multiplexed output.
2) Set TIM3_CH2 to remap to PB5.
3) Initialize TIM3, set ARR and PSC of TIM3.
4) Set PWM mode of TIM3_CH2, and enable CH2 output of TIM3.
5) Enable TIM3.
6) Modify TIM3_CCR2 to control the duty cycle.
3. Hardware Design
Indicator lamp, TIM3
4. Software Design
void TIM3_Int_Init(u16 arr,u16 psc)
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM3, ENABLE); //Clock enable
//Timer TIM3 initialization
TIM_TimeBaseStructure.TIM_Period = arr; //Set the value of the auto-reload register period to load the activity at the next update event
TIM_TimeBaseStructure.TIM_Prescaler =psc; //Set the prescaler value used as the TIMx clock frequency divisor
TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1; //Set clock division: TDTS = Tck_tim
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up; //TIM up counting mode
TIM_TimeBaseInit(TIM3, &TIM_TimeBaseStructure); //Initialize the time base unit of TIMx according to the specified parameters
TIM_ITConfig(TIM3,TIM_IT_Update,ENABLE); //Enable the specified TIM3 interrupt and allow update interrupt
//Interrupt priority NVIC setting
NVIC_InitStructure.NVIC_IRQChannel = TIM3_IRQn; //TIM3 interrupt
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0; //Preempt priority level 0
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 3; //From priority level 3
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; //IRQ channel is enabled
NVIC_Init(&NVIC_InitStructure); //Initialize NVIC registers
TIM_Cmd(TIM3, ENABLE); //Enable TIMx
}
//Timer 3 interrupt service routine
void TIM3_IRQHandler(void) //TIM3 interrupt
{
if (TIM_GetITStatus(TIM3, TIM_IT_Update) != RESET) //Check whether TIM3 update interrupt occurs
{
TIM_ClearITPendingBit(TIM3, TIM_IT_Update ); //Clear TIMx update interrupt flag
LED1=!LED1;
}
}
V. Experimental Results
success!
Previous article:STM32F103ZET6 general timer single pulse mode experiment
Next article:[Experiment 5] Window watchdog experiment
Recommended ReadingLatest update time:2024-11-17 00:50
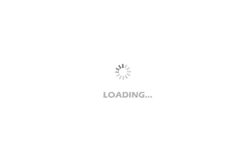
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- EEPROM address problem
- The matching problem between op amp and ADC
- ZigBee-WSN temperature and humidity monitoring system
- Help: After selecting the package in AD09, no corresponding description appears
- TI HVI Power Technology Detailed 2019
- When the op amp is used as a voltage follower, what is the role of the resistor and capacitor connected to the negative feedback terminal?
- Can I install Windows 7 on a computer with this configuration?
- What is Ethernet? What is Industrial Ethernet?
- Has anyone used the official PIC development board? PIC32MZ curiosity board
- Terminal software that supports automatic serial port search