Hardware flow control
uses nCTS input and nRTS output to control the serial data flow between two devices. The figure shows how to connect two devices in this mode:
Write 1 to the RTSE bit and CTSE bit in the USART_CR3 register to enable RTS and CTS flow control respectively.
RTS Flow Control
If RTS flow control is enabled (RTSE=1), the USART receiver asserts nRTS (tied to low) whenever it is ready to receive new data. When the receive register is full, nRTS is deasserted, indicating that the transmission process will stop after the end of the current frame. The following figure shows an example of communication with RTS flow control enabled.
CTS Flow Control
If CTS flow control is enabled (CTSE=1), the transmitter checks nCTS before sending the next frame. If nCTS is valid (tied to low), the next data is sent (assuming data is ready to send, that is, TXE=0); otherwise, no transmission is performed. If nCTS becomes invalid during transmission, the transmitter stops after the current transmission is completed.
When CTSE=1, the CTSIF status bit is automatically set to 1 by hardware whenever nCTS changes. This indicates whether the receiver is ready for communication. If the CTSIE bit in the USART_CR3 register is set to 1, an interrupt is generated. The following figure shows an example of communication with CTS flow control enabled.
Note: Special behavior of stop frame: When CTS flow is enabled, the transmitter will not check the nCTS input status when sending the stop signal.
USART Interrupts
The USART interrupt events are connected to the same interrupt vectors (see Figure 270).
● During transmission: Transmit Complete, Clear to Send or Transmit Data Register Empty interrupts.
● During reception: Idle Line Detect, Overrun Error, Receive Data Register Not Empty, Parity Error, LIN Break Detect, Noise Flag (Multi-buffer communication only) and Framing Error (Multi-buffer communication only)
These events generate interrupts if the corresponding enable control bits are set.
USART Configuration Mode
Continuous Communication Using DMA
Transmitting Using DMA
Enable DMA mode for transmission by setting the DMAT bit in the USART_CR3 register. When the TXE bit is set, data can be loaded from the SRAM area (configured by DMA, see the DMA section) to the USART_DR register. To map a DMA channel for USART transmission, follow these steps (x represents the channel number): 1. Write the
USART_DR register address in the DMA control register to configure it as the destination address of the transfer. After each TXE event, the data is moved from the memory to this address.
2. Write the memory address in the DMA control register to configure it as the source address of the transfer. After each TXE event, the data is loaded from this memory area to the USART_DR register.
3. Configure the total number of bytes to be transferred in the DMA control register. 4. Configure
the channel priority in the DMA register
5. Generate a DMA interrupt after half or all of the transfer is completed, depending on the application requirements.
6. Write 0 to the TC bit in the SR register to clear it.
7. Activate the channel in the DMA registers.
When the amount of data transfer set in the DMA controller is reached, the DMA controller generates an interrupt on the interrupt vector of the DMA channel.
In transmit mode, after the DMA has written all the data to be transmitted (the TCIF flag in the DMA_ISR register is set to 1), the TC flag can be monitored to ensure that the USART communication is complete. This step must be performed before disabling the USART or entering stop mode to avoid corruption of the last transmission. The software must wait until TC=1. The TC flag must remain clear during all data transmissions and then be set to 1 by hardware after the last frame transmission is completed.
Reception using DMA
Setting the DMAR bit in the USART_CR3 register enables DMA mode for reception. When a data byte is received, the data is loaded from the USART_DR register into the SRAM area (configured by DMA, see DMA specification). To map a DMA channel for USART reception, follow these steps:
1. Write the USART_DR register address in the DMA control register to configure it as the source address of the transfer. After each RXNE event, the data is moved from this address to the memory.
2. Write the memory address in the DMA control register to configure it as the destination address of the transfer. After each RXNE event, the data is loaded from the USART_DR register to this memory area.
3. Configure the total number of bytes to be transferred in the DMA control register.
4. Configure the channel priority in the DMA control register.
5. Generate an interrupt after half or all of the transfer is completed, depending on the application requirements.
6. Activate the channel in the DMA control register.
When the amount of data transfer set in the DMA controller is reached, the DMA controller generates an interrupt on the interrupt vector of the DMA channel. In the interrupt subroutine, the DMAR bit in the USART_CR3 register should be cleared by software.
Note: If DMA is used for reception, do not enable the RXNEIE bit.
Error Flags and Interrupt Generation in Multi-Buffer Communication
In multi-buffer communication, if any error occurs in the transaction, an error flag is set after the current byte. If the interrupt enable is set, an interrupt is generated. During single-byte reception, the framing error, overflow error, and noise flags that are set along with RXNE have a separate error flag interrupt enable bit (EIE bit in the USART_CR3 register); if this bit is set, an interrupt is generated after the current byte for any of these errors.
STM32F4 library
serial port configuration process
Enable USART clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USARTx, ENABLE); //1 and 6 USART
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USARTx, ENABLE); //2, 3, 4, 5 USART
Enable GPIO
RCC_AHB1PeriphClockCmd(); //IO can be TX, RX, CTS and SCLK
Set the multiplexing function of the peripheral
1. GPIO_PinAFConfig(GPIOB, GPIO_PinSource6, GPIO_AF_USART1); //Connect the pin to the corresponding multiplexing function
2. Configure the pin to the corresponding multiplexing function through GPIO_InitStruct->GPIO_Mode_AF
3. Select the IO pull-up and pull-down, IO type and IO speed through GPIO_PuPd, GPIO_OType and GPIO_Speed
4. (Asynchronous) Configure Baud Rate, Word Length, Stop Bit, Parity, hardware data, mode (send and receive) through USART_Init().
USART_InitStructure.USART_BaudRate = 115200; /* Baud rate*/
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1; USART_InitStructure.USART_Parity
= USART_Parity_No;
USART_InitStructure.USART_Har dwareFlowControl = USART_HardwareFlowControl_None; USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx; USART_Init(USART1,&USART_InitStructure);
5. For synchronous mode, enable clock and polarity, phase and last bit
void USART_StructInit(USART_InitTypeDef* US ART_InitStruct);
void 6. If interrupts need to be enabled, enable
NVIC and enable the corresponding interrupt bits through the USART_ITConfig function 7. When using DMA mode - configure DMA through the DMA_Init() function ——Activate the required channels through the function USART_Cmd() 8. Enable USART through USART_Cmd() 9. When using DMA, enable DMA through DMA_Cmd() 10. For details about Multi-Processor, LIN, half-duplex, Smartcard, IrDA, please refer to the M4 Authoritative Guide
In order to obtain a higher baud rate, the function USART_OverSampling8Cmd() can be used to set 8 times oversampling (the default is 16 times oversampling). This function needs to be called after the clock RCC_APBxPeriphClockCmd() and before USART_Init().
void USART_DeInit(USART_TypeDef* USARTx) is implemented by resetting the clock
The data transmission function
uses the function USART_ReceiveData() to read the value of the register USART_DR. This data comes from the RDR buffer. The function USART_SendData() is used to write to the register USART_DR. The data will be stored in the TDR buffer.
void USART_SendData(USART_TypeDef* USARTx, uint16_t Data)
{
/* Check the parameters */ assert_param(IS_USART_ALL_PERIPH(USARTx)); assert_param(IS_USART_DATA(Data));
/* Transmit Data */
USARTx->DR = (Data & (uint16_t)0x01FF);
}
uint16_t USART_ReceiveData(USART_TypeDef* USARTx)
{
/* Check the parameters */ assert_param(IS_USART_ALL_PERIPH(USARTx));
/* Receive Data */
return (uint16_t)(USARTx->DR & (uint16_t)0x01FF);
}
DMA transfer and management functions
The function USART_DMACmd is used to enable DMA send and receive requests
void USART_DMACmd(USART_TypeDef* USARTx, uint16_t USART_DMAReq, FunctionalState NewState)
{
/* Check the parameters */ assert_param(IS_USART_ALL_PERIPH(USARTx)); assert_param(IS_USART_DMAREQ(USART_DMAReq)); assert_param(IS_FUNCTIONAL_STATE(NewState));
if (NewState != DISABLE)
{
/* Enable the DMA transfer for selected requests by setting the DMAT and/or DMAR bits in the USART CR3 register */
USARTx->CR3 |= USART_DMAReq;
}
else
{
/* Disable the DMA transfer for selected requests by clearing the DMAT and/or DMAR bits in the USART CR3 register */
USARTx->CR3 &= (uint16_t)~USART_DMAReq;
}
}
中断标志管理函数
查询模式
(#) USART_FLAG_TXE : to indicate the status of the transmit buffer register
(#) USART_FLAG_RXNE : to indicate the status of the receive buffer register
(#) USART_FLAG_TC : to indicate the status of the transmit operation (#) USART_FLAG_IDLE : to indicate the status of the Idle Line
(#) USART_FLAG_CTS : to indicate the status of the nCTS input
(#) USART_FLAG_LBD : to indicate the status of the LIN break detection (#) USART_FLAG_NE : to indicate if a noise error occur
(#) USART_FLAG_FE : to indicate if a frame error occur
(#) USART_FLAG_PE : to indicate if a parity error occur
(#) USART_FLAG_ORE : to indicate if an Overrun error occur
在查询模式,建议使用下面两个函数:
(+) FlagStatus USART_GetFlagStatus(USART_TypeDef* USARTx, uint16_t USART_FLAG);
(+) void USART_ClearFlag(USART_TypeDef* USARTx, uint16_t USART_FLAG);
中断模式
挂起标志Pending Bits:
(##) USART_IT_TXE : to indicate the status of the transmit buffer register
(##) USART_IT_RXNE : to indicate the status of the receive buffer register
(##) USART_IT_TC : to indicate the status of the transmit operation (##) USART_IT_IDLE : to indicate the status of the Idle Line
(##) USART_IT_LBD : to indicate the status of the LIN break detection (##) USART_IT_NE : to indicate if a noise error occur
(##) USART_IT_FE : to indicate if a frame error occur
(##) USART_IT_PE : to indicate if a parity error occur
(##) USART_IT_ORE : to indicate if an Overrun error occur
中断源Interrupt Source:
(##) USART_IT_TXE : specifies the interrupt source for the Tx buffer empty interrupt.
(##) USART_IT_RXNE : specifies the interrupt source for the Rx buffer not empty interrupt.
(##) USART_IT_TC : specifies the interrupt source for the Transmit complete interrupt.
(##) USART_IT_IDLE : specifies the interrupt source for the Idle Line interrupt.
(##) USART_IT_CTS : specifies the interrupt source for the CTS interrupt.
(##) USART_IT_LBD : specifies the interrupt source for the LIN break detection interrupt.
(##) USART_IT_PE : specifies the interrupt source for the parity error interrupt.
(##) USART_IT_ERR : specifies the interrupt source for the errors interrupt.
上面的标志和中断源可以在函数组合使用
在这个模式下,建议使用下面三个函数
(+) void USART_ITConfig(USART_TypeDef* USARTx, uint16_t USART_IT, FunctionalState NewState);
(+) ITStatus USART_GetITStatus(USART_TypeDef* USARTx, uint16_t USART_IT);
(+) void USART_ClearITPendingBit(USART_TypeDef* USARTx, uint16_t USART_IT);
DMA mode
USART has two requests to manage in DMA mode, one is the send request and the other is the receive request
(#) USART_DMAReq_Tx: specifies the Tx buffer DMA transfer request (#) USART_DMAReq_Rx: specifies the Rx buffer DMA transfer request
It is recommended to use the surface function in this mode
(+) void USART_DMACmd(USART_TypeDef* USARTx, uint16_t USART_DMAReq, FunctionalState NewState);
The void USART_ITConfig()
function is used to enable the 8 related interrupt sources, which are the 8 introduced earlier.
FlagStatus USART_GetFlagStatus()
void USART_ClearFlag()
According to the previous introduction, these two are used together, mainly in the query mode for interrupts. There are two other functions
ITStatus USART_GetITStatus()
void USART_ClearITPendingBit()
Previous article:STM32F4 study notes 8——NIVC vector interrupt
Next article:STM32F4 Study Notes 6——USART Part 1
Recommended ReadingLatest update time:2024-11-16 16:34
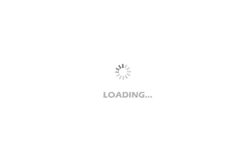
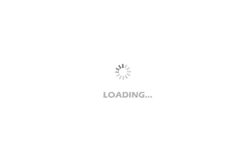
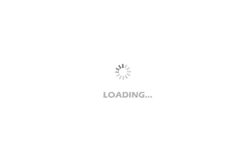
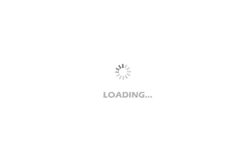
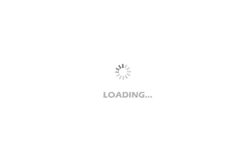
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Who remembers a video about the principles of network communication?
- [Sipeed LicheeRV 86 Panel Review] 7 - lvgl Solution to the problem of incorrect image color display
- Wireless communication technology-NB-IoT
- SMT32 is no longer available, let's discuss alternative models
- Robotics (Stanford University Open Course)
- [Anxinke UWB indoor positioning module NodeMCU-BU01] 01: Module appearance interface, onboard resources and power supply characteristics
- ESD204 surge protection for HDMI interface
- Last day! Pre-register to win a JD card! 60 cards in total! Rohm live broadcast: How to use laser diodes well
- Based in Chengdu, job opening: RF chip test engineer
- CircuitPython 4.0.2 released