The Universal Synchronous Asynchronous Receiver/Transmitter (USART) provides a flexible method for full-duplex data exchange with external devices using the industry standard NR asynchronous serial data format. The USART provides a wide range of baud rate selections using a fractional baud rate generator, supporting both synchronous unidirectional communication and half-duplex single-wire communication.
1. The main idea of using peripheral devices in the STM32 firmware library
In STM32, the configuration ideas of peripheral devices are relatively fixed. First, the relevant clocks are enabled. On the one hand, the clock of the device itself is enabled. On the other hand, if the device outputs through the IO port, the clock of the IO port also needs to be enabled; finally, if the corresponding IO port is an IO port with multiplexing function, the clock of AFIO must also be enabled.
The second step is to configure GPIO. The various properties of GPIO are detailed in the AFIO chapter of the hardware manual and are relatively simple.
Next, if the relevant device needs to use the interrupt function, the interrupt priority must be configured first, which will be described in detail later.
Then configure the related properties of the peripheral device, depending on the specific device. If the device needs to use the interrupt mode, the interrupt of the corresponding device must be enabled, and then the related devices need to be enabled.
Finally, if the device uses the interrupt function, you also need to fill in the corresponding interrupt service program and perform corresponding operations in the service program.
2. UART configuration steps (query method)
2.1. Open the clock
Since the TX, RX and AFIO of UART are all hung on the APB2 bridge, the firmware library function RCC_APB2PeriphClockCmd() is used for initialization. UARTx needs to be discussed separately. If it is UART1, it is hung on the APB2 bridge, so RCC_APB2PeriphClockCmd() is used for initialization, and the rest of UART2~5 are hung on APB1.
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
2.2 GPIO initialization
The properties of GPIO are contained in the structure GPIO_InitTypeDef, where for the TX pin, the GPIO_Mode field is set to GPIO_Mode_AF_PP (multiplexed push-pull output), and the GPIO_Speed switching rate is set to GPIO_Speed_50MHz; for the RX pin, the GPIO_Mode field is set to GPIO_Mode_IN_FLOATING (floating input), and the switching rate does not need to be set. Finally, enable the IO port through GPIO_Init().
The following is the example code for GPIO settings:
GPIO_InitTypeDef GPIO_InitStructure; //USART1 Tx(PA.09) GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP; GPIO_Init(GPIOA, &GPIO_InitStructure); //USART1 Rx(PA.10) GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING; GPIO_Init(GPIOA, &GPIO_InitStructure);
2.3. Configure UART related properties
Determined by the USART_InitTypeDef structure. The fields in UART mode are as follows
USART_BaudRate: baud rate, depending on the specific device
USART_WordLength: word length
USART_StopBits: Stop bits
USART_Parity: verification method
USART_HardwareFlowControl: Hardware flow control
USART_Mode: Single/Duplex
Finally set up. The example code is:
//USART1 configuration USART_InitTypeDef USART_InitStructure; USART_InitStructure.USART_BaudRate = 9600; USART_InitStructure.USART_WordLength = USART_WordLength_8b; USART_InitStructure.USART_StopBits = USART_StopBits_1; USART_InitStructure.USART_Parity = USART_Parity_No; USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None; USART_InitStructure.USART_Mode = USART_Mode_Tx | USART_Mode_Rx; USART_Init(USART1, &USART_InitStructure); USART_Cmd(USART1, ENABLE);
Don't forget to use USART_Cmd() at the end to start the device UART1.
2.4. Redirect the print() function.
int fputc(int ch,FILE *f) { USART1->SR; //USART_GetFlagStatus(USART1, USART_FLAG_TC) Solve the problem of failure to send the first character //Send characters one by one USART_SendData(USART1, (unsigned char) ch); //Wait for sending to complete while(USART_GetFlagStatus(USART1,USART_FLAG_TC)!=SET); return(ch); }
int main(void) { // USART1 config 9600 8-N-1 USART1_Config(); printf("hello world!"); }
3. UART configuration steps (interrupt mode)
Turning on the clock, initializing GPIO, configuring UART related properties, and redirecting the print() function are the same as above.
3.1、Configuration of interrupt priority
This is a strange thing about STM32. When there is only one interrupt, the priority still needs to be configured to enable the trigger channel of a certain interrupt. STM32 interrupts have at most two levels, namely preemptive priority and slave priority. The length of the entire priority setting parameter is 4 bits, so it is necessary to first divide the preemptive priority bit and the slave priority bit, which is implemented through NVIC_PriorityGroupConfig();
The interrupt priority NVIC property of a specific device is contained in the structure NVIC_InitTypeDef, where the field NVIC_IRQChannel contains the interrupt vector of the device, which is saved in the startup code; the field NVIC_IRQChannelPreemptionPriority is the primary priority, and NVIC_IRQChannelSubPriority is the secondary priority. The value range should be determined according to the bit division situation; finally, the NVIC_IRQChannelCmd field is whether to enable, generally located at ENABLE. Finally, NVIC_Init() is used to enable this interrupt vector. The example code is as follows:
//Configure UART1 receive interrupt void NVIC_Configuration(void) { NVIC_InitTypeDef NVIC_InitStructure; /* Configure the NVIC Preemption Priority Bits */ NVIC_PriorityGroupConfig(NVIC_PriorityGroup_0); /* Enable the USARTy Interrupt */ NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn; NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0; NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1; NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; NVIC_Init(&NVIC_InitStructure); }
3.2 Design of interrupt service program
Currently, two interrupts of UART are used: USART_IT_RXNE (receive buffer fill interrupt) and USART_IT_TXE (transmit buffer empty interrupt). The former interrupt ensures that once data is received, an interrupt is entered to receive data of a specific length. The latter interrupt means that once a data is sent, an interrupt function is entered to ensure continuous transmission of a segment of data. All interrupts of a device are included in an interrupt service routine, so it is necessary to first distinguish which interrupt is being responded to this time, and use the USART_GetITStatus() function to determine it; use the USART_ReceiveData() function to receive a byte of data, and use the USART_SendData() function to send a byte of data. When the interrupt is turned off, use USART_ITConfig() to disable the interrupt response. Example program:
All programs in this experiment "STM32 serial port USART1 query and interrupt mode program"
Supplement: There has always been a question about receiving and sending data: for a string like "hello", are they received one by one or displayed as a whole? The following experiment can verify whether it is done one by one.
Previous article:STM32 debug interface SWD connection
Next article:STM32 USART usage notes
Recommended ReadingLatest update time:2024-11-16 13:34
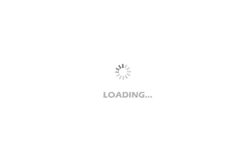
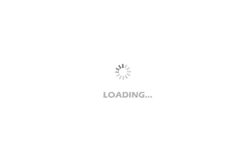
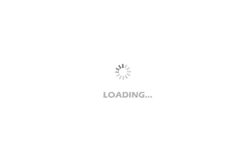
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- OSAL operating system issues
- Want to get better service? TE customer service said: "I can"
- Is the '*' symbol in Verilog considered a multiplier?
- 【Qinheng RISC-V core CH582】Evaluation summary
- Cache Coherence
- Draw a process flow chart for the production of a DC regulated power supply.
- Problems with batch modification of silk screen printing on PCB
- Keep up with the latest news in 2018: fast charging and wireless charging, Bluetooth and smart home and GaN
- Application of LOTO oscilloscope with VI curve tester in circuit board maintenance
- About PCB board to make a set of playing cards