The internal EEPROM of the STC microcontroller is simulated by DATAFLASH, not a real EEPROM memory, and cannot be operated by ordinary methods.
Here are some points to note:
1. Before writing a byte, read the other valid data of the sector where the byte is located into RAM for temporary storage (this step is not necessary).
2. After temporary storage, erase the entire sector (512 bytes). After erasing, the data in each address of the entire sector becomes 0xFF.
3. Write the N bytes of data to be written into EEPROM using the byte write function
. 4. Write other useful EEPROM values temporarily stored in RAM back to EEPROM using the byte write function.
5. The byte write function of the EEPROM simulated by STC using FLASH can only change 1 to 0, but not 0 to 1.
The data is all 1 only after the sector is erased.
For example: at address 0x21f0, write 11010110 for the first time, and write 111010 for the second time. The read result is the AND of these two values 10010.
Therefore, it is wrong to write new data when the value at an address is not 0xff. The sector must be erased first to change it to 0xff.
For writing a single byte, we can first check whether the data at the address is 0xff. If it is, there is no need to erase the sector.
----------------------------------------------------------------------
The reading and writing process of the internal EEPROM of the STC89C52 microcontroller
1. Configure the ISP_CONTR register, enable the 7th bit ISPEN, make the ISP_IAP function effective, and configure the waiting time of the lower 3 bits
2. Write instructions: the 3 commands of read/write/erase sector 3. Assignment
: the address value of ISP_ADDRH and ISP_ADDRL
4. Turn off the total interrupt EA, because the 2 trigger instructions to be written below must be operated continuously and cannot be interrupted
5. Execute the common ISP_IAP trigger instruction. The read and write operations can be performed after the trigger
6. Turn on the interrupt EA, turn off the ISP_IAP function: clear the relevant registers
#include "my51.h"
/******************Define command bytes******************/
#define read_cmd 0x01 //Byte read data command
#define wirte_cmd 0x02 //byte programming data command
#define erase_cmd 0x03 // sector erase data command
/****************Special function register declaration****************/
sfr ISP_DATA = 0xe2;
sfr ISP_ADDRH = 0xe3;
sfr ISP_ADDRL = 0xe4;
sfr ISP_CMD = 0xe5;
sfr ISP_TRIG = 0xe6;
sfr ISP_CONTR = 0xe7;
/*Define Flash operation waiting time and constants to allow IAP/ISP/EEPROM operations******************/
//#define enable_waitTime 0x80 //When the system operating clock is <30MHz, set this value to the IAP_CONTR register
//#define enable_waitTime 0x81 //When the system operating clock is <24MHz, set this value to the IAP_CONTR register
//#define enable_waitTime 0x82 //When the system operating clock is <20MHz, set this value for the IAP_CONTR register
#define enable_waitTime 0x83 //When the system working clock is <12MHz, set this value to the IAP_CONTR register
//#define enable_waitTime 0x84 //When the system operating clock is <6MHz, set this value for the IAP_CONTR register
void ISP_IAP_disable(void) //Close ISP_IAP
{
EA=1; //Resume interrupt
ISP_CONTR = 0x00;
ISP_CMD = 0x00;
ISP_TRIG = 0x00;
}
void ISP_IAP_trigger() //trigger
{
EA=0; //The following two instructions must be executed continuously, so the interrupt is disabled
ISP_TRIG = 0x46; //Send trigger command word 0x46
ISP_TRIG = 0xB9; //Send trigger command word 0xB9
}
void ISP_IAP_readData(u16 beginAddr, u8* pBuf, u16 dataSize) //Read data
{
ISP_DATA=0; //clear to zero, or not clear
ISP_CMD = read_cmd; //Command: read
ISP_CONTR = enable_waitTime; // Enable ISP_IAP and send the waiting time
while(dataSize--) //Loop reading
{
ISP_ADDRH = (u8)(beginAddr >> 8); //Send address high byte
ISP_ADDRL = (u8)(beginAddr & 0x00ff); //Send address low byte
ISP_IAP_trigger(); //Trigger
beginAddr++; //Address++
*pBuf++ = ISP_DATA; //Save data to the receiving buffer
}
ISP_IAP_disable(); //Disable ISP_IAP function
}
void ISP_IAP_writeData(u16 beginAddr,u8* pDat,u16 dataSize) //write data
{
ISP_CONTR = enable_waitTime; // Enable ISP_IAP and send the waiting time
ISP_CMD = wirte_cmd; //Send byte programming command word
while(dataSize--)
{
ISP_ADDRH = (u8)(beginAddr >> 8); //Send address high byte
ISP_ADDRL = (u8)(beginAddr & 0x00ff); //Send address low byte
ISP_DATA = *pDat++; //Send data
beginAddr++;
ISP_IAP_trigger(); //Trigger
}
ISP_IAP_disable(); //Disable
}
void ISP_IAP_sectorErase(u16 sectorAddr) // sector erase
{
ISP_CONTR = enable_waitTime; // Enable ISP_IAP; and send the waiting time
ISP_CMD = erase_cmd; //Send sector erase command word
ISP_ADDRH = (u8)(sectorAddr >> 8); //Send address high byte
ISP_ADDRL = (u8)(sectorAddr & 0X00FF); //Send address low byte
ISP_IAP_trigger(); //Trigger
ISP_IAP_disable(); //Disable ISP_IAP function
}
void main() //test
{
u8 buf[3]={0}; //Receive data buffer
u8 dat[5]={b(111010),b(1001),b(1),b(1011),b(1110)}; //I wrote it in binary to observe the LED light
ISP_IAP_sectorErase(0x2000); // sector erase, one block of 512 bytes
ISP_IAP_writeData(0x21f0,dat,sizeof(dat)); //Write EEPROM
ISP_IAP_readData(0x21f0,buf,sizeof(buf)); //Read
P1=buf[2]; //Write 11010110 at address 0x21f0 for the first time, and 111010 for the second time. The result is the AND of these two values 10010
while(1); //So if the value at an address is not 0xff, it is wrong to write new data. You must first erase it to 0xff
}
#ifndef _MY51_H
#define _MY51_H
#include
//#include
#include
#include
#include "mytype.h"
/*************Binary Input Macro****************************/
#ifndef _LongToBin_
#define _LongToBin_
#define LongToBin(n) \
( \
((n >> 21) & 0x80) | \
((n >> 18) & 0x40) | \
((n >> 15) & 0x20) | \
((n >> 12) & 0x10) | \
((n >> 9) & 0x08) | \
((n >> 6) & 0x04) | \
((n >> 3) & 0x02) | \
((n) & 0x01) \
)
#define bin(n) LongToBin(0x##n##l)
#define BIN(n) bin(n)
#define B(n) bin(n)
#define b(n) bin(n)
#endif
/*************Setting macro for a single data bit*********************/
#ifndef _BIT_
#define _BIT_
#define BIT(n) (1<
#define bit(n) BIT(n)
#endif
#define high 1 //High level
#define low 0 //Low level
#define led P1 //light bus control
sbit led0=P1^0; //8 LED lights, the cathode sends a low level to light up
sbit led1=P1^1;
sbit led2=P1^2;
sbit led3=P1^3;
sbit led4=P1^4;
sbit led5=P1^5;
sbit led6=P1^6;
sbit led7=P1^7;
sbit ledLock=P2^5; //LED latch status, 0 for lock, 1 for unlock
sbit beep=P2^3; //Buzzer
void delayms(u16 ms);
//void delayXus(u8 us); //The function executes (8+6x) machine cycles, i.e. t=(8+6x)*1.085
///////////////////////////////////////////////////// //////////////////////////////
#endif
Previous article:51 MCU learning notes: stepper motor control, forward and reverse rotation, etc.
Next article:51 MCU Study Notes: Infrared Receiver
Recommended ReadingLatest update time:2024-11-16 12:44
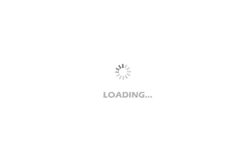
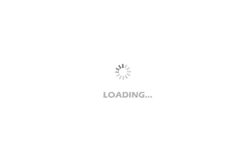
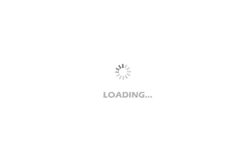
![[MCU] [Learning Log] 51 MCU Learning Log [Day1, 2022.1.09]](https://6.eewimg.cn/news/statics/images/loading.gif)
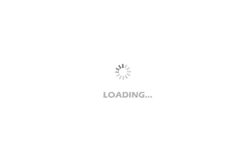
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Check and compare the specified current limit with the actual current limit. Have you used counterfeit LM257x and LM259x?
- I'm soldering a board recently, but I saw that the circuit requires 500k ohm and 5meg resistors. Do you have something similar that I can use? ?
- MSP430 Learning Experience
- Understanding and Utilizing Solar Loads for Augmented Reality Head-Up Displays
- For example, for a 24-bit ADC, we only know the accuracy. How is the sampling speed/sampling rate of the microcontroller calculated?
- #Idle Market#Selling Texas Instruments Tiva C Series TM4C123G Development Board
- A Preliminary Study on the Working Principle of PCIe
- FAQ_ How to set up the allocation of memory to the dynamic memory area
- 【Running posture training shoes】No.003-Data collection and real-time display
- [Open Source] FPGA-based binocular camera adapter board