LPC2478 external interrupt
The external interrupt model of 2478 is as follows
That is to say, port 0 and 2 support external interrupts, EINT0-2 are interrupts for three independent pins, and EINT3 is the vector shared by all interrupts of port 0 and 2.
For port 0 and 2, you do not need to configure the pins to interrupt mode. Just configure them to input mode and enable interrupts. For independent interrupts, the configuration process is as follows
1. Configure the corresponding pin to EINT mode
2. Set the mode to pull up or down according to your needs
3. Configure the interrupt mode and interrupt polarity in the system control register
4. Configure the middle end, match the interrupt function, priority, enable interrupt, etc.
The interrupts of PORT0 and PORT2 are actually similar in usage, mainly the configuration of EINT3
1. Select GPIO for IO port function
2. Enable the GPIO interrupt of the pin
3. Next, configure the eint3 interrupt and you can use it
When interrupt processing, eint0-2 can be processed directly, and port0 and port2 interrupts in eint3 need to be judged once, relying on the following three registers
From these three registers, you can see the interrupt status of each pin and choose the correct processing method
Check the code for details
#ifndef __EXTI_H_
#define __EXTI_H_
#include "common.h"
#include "lpc24xx.h"
extern u8 eint0Count;
extern u8 eint3Count;
void ExtiInit(void);
void GPIOEINT3Init(void);
#endif
#include "exti.h"
u8 eint0Count = 0;
u8 eint3Count = 0;
void __irq EINT0_Handler(void)
{
IENABLE; /* handles nested interrupt */
EXTINT |= (1<<0); // Clear interrupt
//do something here
eint0Count = 1;
IDISABLE;
VICVectAddr = 0; /* Acknowledge Interrupt */
}
void __irq EINT3_Handler(void)
{
IENABLE; /* handles nested interrupt */
//do something here
if((IO_INT_STAT&(1<<0)) == 1)//port0 interrupt
{
if((IO0_INT_STAT_F&(1<<10)) != 0)//Confirm that an interrupt occurs on P0.10
{
eint3Count = 1;
IO0_INT_CLR |= (1<<10);
}
}
EXTINT |= (1<<3); // Clear interrupt
IDISABLE;
VICVectAddr = 0; /* Acknowledge Interrupt */
}
//Use exti0 as an example
void ExtiInit(void)
{
PINSEL4 &= ~(3<<20); //Configure as interrupt
PINSEL4 |= (1<<20);
PINMODE4 &= ~(3<<20); //Configure pull-up resistor
EXTMODE |= (1<<0); //Edge trigger
//
EXTPOLAR |= (1<<0); // rising edge trigger
//Interrupt vector configuration
VICSoftIntClr |= (1<<14); // Clear software interrupt, eint0 interrupt source is 14 0 start
VICIntEnClr |= (1<<14); //Disable interrupt
VICIntSelect &= (1<<14); //Select IRQ interrupt
VICVectAddr14 = (unsigned)EINT0_Handler; //Connect interrupt vector
VICVectPriority14 = 0x01; //Interrupt priority register
VICIntEnable |= (1<<14); //Interrupt vector enable is valid
}
//Use p0.10 as an example
void GPIOEINT3Init(void)
{
PINSEL0 &= ~(3<<20); //Select normal IO function
PINMODE0 &= ~(3<<20); //Select pull-up
IO0_INT_EN_F |= (1<<10); // falling edge interrupt enable
EXTMODE |= (1<<3); //Edge trigger
EXTPOLAR &= ~(1<<3); //Falling edge trigger
//Interrupt vector configuration
VICSoftIntClr |= (1<<17); // Clear software interrupt, eint3 interrupt source is 17 0 start
VICIntEnClr |= (1<<17); //Disable interrupt
VICIntSelect &= (1<<17); //Select IRQ interrupt
VICVectAddr17 = (unsigned)EINT3_Handler; //Connect interrupt vector
VICVectPriority17 = 0x01; //Interrupt priority register
VICIntEnable |= (1<<17); //Interrupt vector enable is valid
}
In the external interrupt handler, remember to clear the corresponding IO port interrupt first
Then clear the EINT0 interrupt. Do not clear EINT first and then clear the IO port interrupt (this will cause repeated interrupts)
Previous article:SPI usage of LPC2478
Next article:Detailed explanation of GPIO usage of LPC2478
Recommended ReadingLatest update time:2024-11-16 12:46
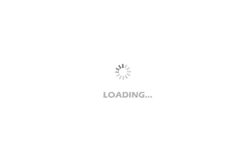
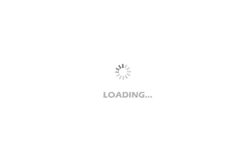
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Project establishment of the JIHAI APM32E103VET6 evaluation (Keil)
- Analysis of key technologies of small base stations in the 5G era
- Allwinner V5 - Building FTP environment --- Lindeni V5 development board
- Chip manual calculation problem
- Business cards that can run Linux
- UC3843 BUCk Buck Circuit Implementation
- [RVB2601 Creative Application Development] Online MP3 Music Playback
- What will happen if you input a non-existent time into the real-time clock DS1302?
- [Shanghai Hangxin ACM32F070 development board + touch function evaluation board] 01. Basic engineering & KEY & LED & BUZZER & SHELL
- Complain about the search engine you currently use...