#include "led.h"
void led_init(void)
{
//p1.14 p0.16 p1.13 p4.27
LPC_SC->PCONP |= (1<<15); //Turn on the clock
//Select the pin mode. 1788 has designed a register for each pin to select the pin mode
LPC_IOCON->P1_14 = 0x00; //Select gpio function, disable hysteresis, non-reverse, normal push-pull
LPC_IOCON->P1_14 |= (2<<3); //Pull up
P1dir(14) = 1; //output
P1low(14) = 1; //Set to low level
LPC_IOCON->P0_16 = 0x00; //Select gpio function, disable hysteresis, non-reverse, normal push-pull
LPC_IOCON->P0_16 |= (2<<3); //Pull up
P0dir(16) = 1; //output
P0low(16) = 1; //Set to low level
LPC_IOCON->P1_13 = 0x00; //Select gpio function, disable hysteresis, non-reverse, normal push-pull
LPC_IOCON->P1_13 |= (2<<3); //Pull up
P1dir(13) = 1; //output
P1low(13) = 1; //Set to low level
LPC_IOCON->P4_27 = 0x00; //Select gpio function, disable hysteresis, non-reverse, normal push-pull
LPC_IOCON->P4_27 |= (2<<3); //Pull up
P4dir(27) = 1; //output
P4low(27) = 1; //Set to low level
}
void led_set(u8 ch)
{
switch(ch)
{
case 0:
P1high(14) = 1;
break;
case 1:
P0high(16) = 1;
break;
case 2:
P1high(13) = 1;
break;
case 3:
P4high(27) = 1;
break;
}
}
void led_clear(u8 ch)
{
switch(ch)
{
case 0:
P1low(14) = 1; //Set to low level
break;
case 1:
P0low(16) = 1; //Set to low level
break;
case 2:
P1low(13) = 1; //Set to low level
break;
case 3:
P4low(27) = 1; //Set to low level
break;
}
}
//Bitband operation to achieve GPIO control function similar to 51
//For specific implementation ideas, please refer to Chapter 5 (pages 87-92) of <
//IO port operation macro definition
#define BITBAND(addr, bitnum) ((addr & 0xF0000000)+0x2000000+((addr &0xFFFFF)<<5)+(bitnum<<2))
#define MEM_ADDR(addr) *((volatile unsigned long *)(addr))
#define BIT_ADDR(addr, bitnum) MEM_ADDR(BITBAND(addr, bitnum))
//IO port address mapping
//Output register
#define GPIO0_ODR_Addr (LPC_GPIO0_BASE+0x18) //0x2009C018
#define GPIO1_ODR_Addr (LPC_GPIO1_BASE+0x18) //0x2009C038
#define GPIO2_ODR_Addr (LPC_GPIO2_BASE+0x18) //0x2009C058
#define GPIO3_ODR_Addr (LPC_GPIO3_BASE+0x18) //0x2009C078
#define GPIO4_ODR_Addr (LPC_GPIO4_BASE+0x18) //0x2009C098
//Input register
#define GPIO0_IDR_Addr (LPC_GPIO0_BASE+0x14) //0x2009C014
#define GPIO1_IDR_Addr (LPC_GPIO1_BASE+0x14) //0x2009C034
#define GPIO2_IDR_Addr (LPC_GPIO2_BASE+0x14) //0x2009C054
#define GPIO3_IDR_Addr (LPC_GPIO3_BASE+0x14) //0x2009C074
#define GPIO4_IDR_Addr (LPC_GPIO4_BASE+0x14) //0x2009C094
//Direction register
#define GPIO0_DIR_Addr (LPC_GPIO0_BASE+0x00) //0x2009C000
#define GPIO1_DIR_Addr (LPC_GPIO1_BASE+0x00) //0x2009C020
#define GPIO2_DIR_Addr (LPC_GPIO2_BASE+0x00) //0x2009C040
#define GPIO3_DIR_Addr (LPC_GPIO3_BASE+0x00) //0x2009C060
#define GPIO4_DIR_Addr (LPC_GPIO4_BASE+0x00) //0x2009C080
// Clear register
#define GPIO0_CLS_Addr (LPC_GPIO0_BASE+0x1C) //0x2009C01C
#define GPIO1_CLS_Addr (LPC_GPIO1_BASE+0x1C) //0x2009C03C
#define GPIO2_CLS_Addr (LPC_GPIO2_BASE+0x1C) //0x2009C05C
#define GPIO3_CLS_Addr (LPC_GPIO3_BASE+0x1C) //0x2009C07C
#define GPIO4_CLS_Addr (LPC_GPIO4_BASE+0x1C) //0x2009C09C
//IO port operation, only for a single IO port!
// Make sure the value of n is less than 32!
#define P0high(n) BIT_ADDR(GPIO0_ODR_Addr,n) //Output 0 Output unchanged 1 Output is 1
#define P0low(n) BIT_ADDR(GPIO0_CLS_Addr,n) // Clear 0 output unchanged 1 output 0
#define P0in(n) BIT_ADDR(GPIO0_IDR_Addr,n) //输入
#define P0dir(n) BIT_ADDR(GPIO0_DIR_Addr,n) //Direction 0 input 1 output
#define P1high(n) BIT_ADDR(GPIO1_ODR_Addr,n) //Output 0 Output unchanged 1 Output is 1
#define P1low(n) BIT_ADDR(GPIO1_CLS_Addr,n) // Clear 0 output unchanged 1 output 0
#define P1in(n) BIT_ADDR(GPIO1_IDR_Addr,n) //Input
#define P1dir(n) BIT_ADDR(GPIO1_DIR_Addr,n) //Direction 0 input 1 output
#define P2high(n) BIT_ADDR(GPIO2_ODR_Addr,n) //Output 0 Output unchanged 1 Output is 1
#define P2low(n) BIT_ADDR(GPIO2_CLS_Addr,n) // Clear 0 output unchanged 1 output 0
#define P2in(n) BIT_ADDR(GPIO2_IDR_Addr,n) //输入
#define P2dir(n) BIT_ADDR(GPIO2_DIR_Addr,n) //Direction 0 input 1 output
#define P3high(n) BIT_ADDR(GPIO3_ODR_Addr,n) //Output 0 Output unchanged 1 Output is 1
#define P3low(n) BIT_ADDR(GPIO3_CLS_Addr,n) // Clear 0 output unchanged 1 output 0
#define P3in(n) BIT_ADDR(GPIO3_IDR_Addr,n) //输入
#define P3dir(n) BIT_ADDR(GPIO3_DIR_Addr,n) //Direction 0 input 1 output
#define P4high(n) BIT_ADDR(GPIO4_ODR_Addr,n) //Output 0 Output unchanged 1 Output is 1
#define P4low(n) BIT_ADDR(GPIO4_CLS_Addr,n) // Clear 0 output unchanged 1 output 0
#define P4in(n) BIT_ADDR(GPIO4_IDR_Addr,n) //输入
#define P4dir(n) BIT_ADDR(GPIO4_DIR_Addr,n) //Direction 0 input 1 output
Previous article:LPC1788 LCD interface drives true color screen
Next article:IIC usage of LPC1788
Recommended ReadingLatest update time:2024-11-17 05:49
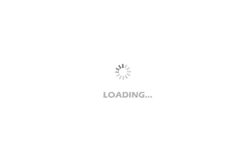
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- How to simulate I2C communication using GPIO on C2000
- Evaluation board quick test - based on TI Sitara Cortex-A9
- Vimicro goes public
- Never forget the teacher's kindness, teachers, happy holidays~~
- ★★ If you don’t read this before you start to learn embedded systems, you will definitely regret it.
- PCB short circuit
- BLE Bluetooth Basic Concepts
- RF PCB Design Tips
- [Essential Tips for Job Hunting] Summary of Written Test Questions for Electronic Engineer Interviews
- Design of full-speed USB interface for CC2531 chip