The principle of buzzer sound is that the current passes through the electromagnetic coil, which makes the electromagnetic coil generate a magnetic field to drive the vibration membrane to make sound. Therefore, a certain current is required to drive it. The current output by the IO pin of the microcontroller is small, and the TTL level output by the microcontroller basically cannot drive the buzzer. Therefore, a current amplification circuit is needed. The role of the transistor is to drive, and the driving current is amplified by the transistor, so that the buzzer can make a sound.
The difference between active buzzer and passive buzzer:
The "source" here does not refer to the power supply. It refers to the oscillation source. In other words, the active buzzer has an oscillation source inside, so it will buzz as soon as it is powered on. The passive buzzer
does not have an oscillation source inside, so it cannot be made to buzz if a DC signal is used. It must be driven by a 2K~5K square wave.
Active buzzers are often more expensive than passive ones because they have multiple oscillation circuits inside.
The advantages of passive buzzers are: 1. Cheap, 2. The sound frequency is controllable, and the "Doremi Fasolaxi" effect can be produced. 3. In some special cases, it can reuse a control port with the LED. The advantages of active buzzers are: convenient program control.
Routine, reference from the Internet.
1 /************************************************ ************************
2 [File Name] C51 Music Program (August Osmanthus)
3 [Function] Playing music through microcontroller
4
5/************************************************ ************************/
6 #include
7 #include
8 //This example uses 89C52, the crystal oscillator is 11.0592MHZ
9 // Regarding how to compile music code, it is actually very simple. You can see the following code.
10 //The frequency constant is the pitch in musical terms, and the beat constant is the number of beats in musical terms;
11 //So take out the music score and try to edit it!
12
13 sbit Beep = P1^5 ;
14
15 unsigned char n=0; //n is the beat constant variable
16 unsigned char code music_tab[] ={
17 0x18, 0x30, 0x1C, 0x10, //Format: frequency constant, beat constant, frequency constant, beat constant,
18 0x20, 0x40, 0x1C , 0x10,
19 0x18, 0x10, 0x20 , 0x10,
20 0x1C, 0x10, 0x18, 0x40,
21 0x1C, 0x20, 0x20 , 0x20,
22 0x1C, 0x20, 0x18, 0x20,
23 0x20, 0x80, 0xFF , 0x20,
24 0x30, 0x1C, 0x10, 0x18,
25 0x20, 0x15, 0x20 , 0x1C,
26 0x20, 0x20, 0x20 , 0x26,
27 0x40, 0x20, 0x20 , 0x2B,
28 0x20, 0x26, 0x20 , 0x20,
29 0x20, 0x30, 0x80, 0xFF,
30 0x20, 0x20, 0x1C , 0x10,
31 0x18, 0x10, 0x20, 0x20,
32 0x26, 0x20, 0x2B , 0x20,
33 0x30, 0x20, 0x2B , 0x40,
34 0x20, 0x20, 0x1C , 0x10,
35 0x18, 0x10, 0x20, 0x20,
36 0x26, 0x20, 0x2B , 0x20,
37 0x30, 0x20, 0x2B , 0x40,
38 0x20, 0x30, 0x1C , 0x10,
39 0x18, 0x20, 0x15 , 0x20,
40 0x1C, 0x20, 0x20 , 0x20,
41 0x26, 0x40, 0x20 , 0x20,
42 0x2B, 0x20, 0x26 , 0x20,
43 0x20, 0x20, 0x30, 0x80,
44 0x20, 0x30, 0x1C , 0x10,
45 0x20, 0x10, 0x1C , 0x10,
46 0x20, 0x20, 0x26 , 0x20,
47 0x2B, 0x20, 0x30, 0x20,
48 0x2B, 0x40, 0x20, 0x15,
49 0x1F, 0x05, 0x20, 0x10,
50 0x1C, 0x10, 0x20, 0x20,
51 0x26, 0x20, 0x2B , 0x20,
52 0x30, 0x20, 0x2B , 0x40,
53 0x20, 0x30, 0x1C , 0x10,
54 0x18, 0x20, 0x15 , 0x20,
55 0x1C, 0x20, 0x20 , 0x20,
56 0x26, 0x40, 0x20 , 0x20,
57 0x2B, 0x20, 0x26 , 0x20,
58 0x20, 0x20, 0x30, 0x30,
59 0x20, 0x30, 0x1C , 0x10,
60 0x18, 0x40, 0x1C , 0x20,
61 0x20, 0x20, 0x26 , 0x40,
62 0x13, 0x60, 0x18, 0x20,
63 0x15, 0x40, 0x13 , 0x40,
64 0x18, 0x80, 0x00
65 };
66
67 void int0() interrupt 1 //Use interrupt 0 to control the beat
68 {TH0=0xd8;
69 TL0=0xef;
70 n--;
71 }
72
73 void delay (unsigned char m) //Control frequency delay
74 {
75 unsigned i=3*m;
76 while(--i);
77 }
78
79 void delayms(unsigned char a) // millisecond delay subroutine
80 {
81 while(--a); //Use while(--a) Do not use while(a--); You can compile and see the assembly result to know!
82 }
83
84 void main()
85 { unsigned char p,m; //m is the frequency constant variable
86 unsigned char i=0;
87 TMOD&=0x0f;
88 TMOD|=0x01;
89 TH0=0xd8;TL0=0xef;
90 IE=0x82;
91 play:
92 while(1)
93 {
94 a: p=music_tab[i];
95 if(p==0x00) { i=0, delayms(1000); goto play;} //If the end character is encountered, delay 1 second and go back to the beginning and play again
96 else if(p==0xff) { i=i+1;delayms(100),TR0=0; goto a;} //If a rest is encountered, delay 100ms and continue to pick the next note
97 else {m=music_tab[i++], n=music_tab[i++];} //Get the frequency constant and beat constant
98 TR0=1; //Start timer 1
99 while(n!=0) Beep=~Beep,delay(m); //Wait for the beat to finish, output audio through P1 port (multi-channel is possible!)
100 TR0=0; //Turn off timer 1
101 }
102 }
Previous article:51 MCU AD conversion
Next article:51 MCU buttons, keyboard detection
Recommended ReadingLatest update time:2024-11-16 17:46
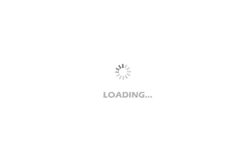
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
Teach you to learn 51 single chip microcomputer-C language version (Second Edition) (Song Xuefeng)
-
Hand-drawn illustrations revealing the secrets of communication circuits and sensor circuits (Mims III)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- NPN transistor saturation conduction conditions VB>VC, VB>VE
- I bought a polymer lithium battery (3.7V10A, 1C) on TB. Can I use TP4056 or IP5305, IP5306 module to charge and discharge it?
- Courseware Collection|Tektronix Semiconductor Materials and Devices Seminar (2019-2018)
- RaspberryPi Pico To-Do Board using Micropython
- TTP223_BA6 uses non-isolated power supply + inverter and the lower button fails
- 【TI Recommended Course】#TI.com Online Purchasing Special: Smart Buildings#
- Filtering and Signal Processing Reference Design for MSP430 FRAM Microcontrollers
- [Chuanglong TLT3-EVM Development Board Review] From Development Board to PLC
- This article explains the process of Keil compiling a program
- Support EEWorld 19 Growth Plan and win wonderful gifts!