Recently, I need to use IIC bus operation when using the light measurement module BH1750FVI, so I spent some time to learn it and basically understood it. So, I used the IO port of 51 to simulate the bus timing and correctly operate a series of modules that need to be accessed by IIC bus. I originally wanted to write an article to briefly introduce my understanding of IIC bus, but I found that I didn’t have time, so I will do it later. Today I will only give a sample program, which can work well on my 51 single-chip computer system. iic.h lists all the operation functions of IIC bus. The source code is given below:
//iic.h - implementation related header file
//Girls Don't Cry 2013-01-18
#ifndef __IIC_H__
#define __IIC_H__
void iic_start(void);
void iic_stop(void);
bit iic_get_ack(void);
void iic_write_byte(uchar dat);
uchar iic_read_byte(void);
void iic_send_ack(bit ack);
#endif//!__IIC_H__
//IIC bus simulation implementation file
//Girls Don't Cry 22:11 2013-1-18
#include "common.h"
#include "iic.h"
sbit SDA = P1^2;
sbit SCL = P1^0;
//Generate START signal
void iic_start(void)
{
SDA = 1; //need to be set before SCL
SCL = 1; //Hardware enters SDA detection state
delay_us(5); //Delay at least 4.7us
SDA = 0; //SDA changes from 1->0, generating a start signal
delay_us(5); //delay at least 4us
SCL = 0; //SCL becomes invalid
}
//Generate STOP signal
void iic_stop(void)
{
SDA = 0; // pull low before SCL
SCL = 1; //Hardware enters SDA detection state
delay_us(5); //Delay at least 4us
SDA = 1; //SDA changes from 0->1, generating an end signal
delay_us(5); //Delay at least 4.7us
}
//Write 1 byte to the IIC bus
void iic_write_byte(uchar dat)
{
uchar loop = 8; //Must be one byte
while(loop--){
SDA = dat&0x80; //Write bit by bit starting from MSB
SCL = 1;
delay_us(5); //delay at least 4us
SCL = 0;
dat <<= 1; //Move from low to high
delay_us(5);
}
}
//Read 1 byte from the IIC bus
uchar iic_read_byte(void)
{
uchar loop = 8; //Must be one byte
uchar ret = 0;
while(loop--){
SDA = 1; //8051 internal resistor pull-up
SCL = 1;
delay_us(5); //delay at least 4us
ret <<= 1;
ret |= SDA; //read 1 bit
SCL = 0 ;
delay_us(5);
}
return ret;
}
//Send response code from master to slave
//0-ACK,1-NAK
void iic_send_ack(bit ack)
{
SDA = ack; //Generate response level
SCL = 1; //Send response signal
delay_us(5); //delay at least 4us
SCL = 0; //Maintain the response signal during the whole period
}
//Get the response code from slave to master
bit iic_get_ack(void)
{
bit ret; // used to receive the return value
SDA = 1; //Resistor pull-up, enter read (8051)
SCL = 1; //Enter response detection
delay_us(5); //Delay at least 4us
ret = SDA; //Save the response signal
SCL = 0;
return ret;
}
The above code gives all the IIC bus programs.
Functions implemented in common.h and related definitions
Previous article:AT93C46 read and write program (C language) through debugging
Next article:8051 MCU Study Notes/Summary/Summary/Memo
Recommended ReadingLatest update time:2024-11-16 16:33
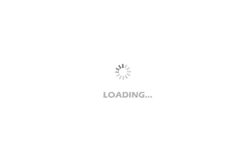
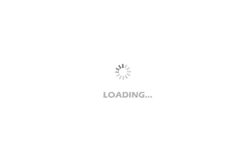
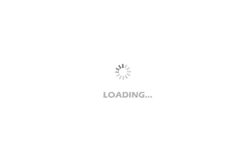
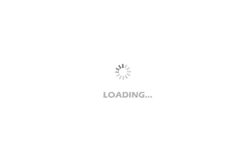
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Fundamentals and Applications of Single Chip Microcomputers (Edited by Zhang Liguang and Chen Zhongxiao)
-
Single chip microcomputer control technology (Li Shuping, Wang Yan, Zhu Yu, Zhang Xiaoyun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- TI DBV package silkscreen details
- MSP430F6638 baud rate setting
- KiCAD released a new version - 5.1.10
- 2020 National College Student Electronics Contest Test Questions
- High voltage and small size: the latest technology trends of Nichicon's drive film capacitors
- [Chip] Interesting chips, interesting engineer souls
- Serial communication
- TMS320C6678DSP SRIO channel and frequency settings
- Development troubles, what to do when you encounter bad teammates
- Should 5G be blamed for cheating in the college entrance examination?