When using KEIL, we are used to writing what we want to write in a .c file in the order of our own thoughts. This is a very common way of writing. When the program is relatively short, such as dozens of lines or more than a hundred lines, there is no problem. But when the program is very long, for example, if you want to use LCD to display data, there are several LCD-related functions, and then you want to display the temperature on the LCD, then there must be DS18B20-related operations, which have several related functions. If you also want to add the time display function of DS1302, then there are many more functions. In this way, it is normal for a program to have hundreds of lines. For the program you wrote, it may be clearer in your mind and not too messy, but when you give the program you wrote to others to read, others often read it in the clouds, and often don't know what you wrote after reading it.
If you have written a relatively long program like an electronic clock, you must have downloaded related programs on the Internet and read them. Have you ever felt that other people's programs look very depressed? Haha. Now let's introduce a module writing method in C language in KEIL. This writing method is actually very useful and is often seen in some slightly longer programs. The structure is shown in the figure below:
Does it look familiar? That's right. In fact, if you have learned the C language for PC and are familiar with compiling multiple files, then this is not a problem, because it is basically the same. If you are an expert and are familiar with it, then please skip this article; if you are not very familiar with it and are a little interested in it, then this article may be helpful to you. If there is anything wrong with this article, please post it in the comments. Or leave me a message on my homepage for communication.
This tutorial is not easy to explain clearly in the form of text. If it is done with a video, the effect should be much better, but I don't have this condition (I am not good at Mandarin and I am afraid of scaring everyone, haha). It may not be finished in one post, and typing is a very painful thing, so please forgive me for this. Let's start officially.
Our main purpose is to learn modular writing, so the function is secondary. After getting familiar with this writing, the function is controlled by everyone. Now we will take the control of LED lights as an example.
So, we first create three .c files, named main.c, delay.c and led_on.c, and try to make the program function visible by looking at the file name when creating the file, so that it is more intuitive and not easy to be confused. Then add all three files to the project. (This is not impossible, right?)
In delay.c, we add the following code:
void delay1s() { unsigned int m,n; for(m=1000;m>0;m--) for(n=20;n>0;n--); }
Of course, the actual delay time of this delay function is not one second, so we don't need to worry about it for now. Just know that it has a delay effect. In the file led_on.c, add the following code:
void led_on() { P0=0x00; delay1s(); P0=0xff; delay1s(); }
Then we add the following code in the main.c function:
void main() { led_on(); }
The function of this program is very simple, which is to realize the flashing of LED.
The next question is how to associate these three C files.
In fact, in a single .c file program, the first thing we do when writing a program is to write #include
Let's talk about it here first. Next time, let's see how to associate the three files.
First, we need a new document. There are two ways to create this document (taking the delay1s function as an example). The first way is to create a delay1s.txt in the project directory and then rename it to delay1s.h. Because they are all in the same encoding, there will be no garbled characters, and then open it in the project. The second way is to directly create a new document in the project, and then save it as delay1s.h. If you need to add a lot of files, it is recommended to use the first method. This is my personal suggestion. Secondly, we need to write the content of the delay1s.h file, the content is as follows:
#ifndef _DELAY1S_H_#define _DELAY1S_H_ void delay1s(); //delay function#endif
This is the definition of the header file, which declares the delay1s() function. If we need to use the delay1s() function in other functions, an error will occur during compilation if it is not declared in advance. For #ifndef...#define...#endif; this structure roughly means that if a string is not defined (macro definition), then we define it and then execute the following statements. If it is defined, then skip and do not execute any statements.
Why do we need to use such a definition method? For example, in the led_on() function, we call the delay1s() function, and then in the main() function, we also call the delay() function. Then, in the led_on() function, we need to include the header file delay1s.h, and then in the main() function, we also need to include delay1s.h. If we have called led_on() in the main function, then when we encounter delay1s() and led_on() during compilation, delay1s.h will be interpreted twice, and an error will occur. If there is the above preprocessing command, then the _DELAY1S_H_ has been defined the second time, so there will be no problem of duplicate definition. This is its function. But please note that the header file does not participate in the compilation when the compiler is compiling.
Again, we create a led_on.h file, and the code content is as follows:
#ifndef _LED_ON_H_#define _LED_ON_H_ void led_on();//light flashes#endif
The function is the same as delay1s.h. If you don't understand, you can look at the explanation above again.
Finally, let’s complete the three functions we talked about last time.
In the led_on() function, we used the definitions of some registers of the 51 MCU, so we need to include reg52.h, and we used the delay1s() function, so we need to include delay1s.h. Therefore, the code of the led_on() function is as follows:
#include#include “delay1s.h” //Note that there is no semicolon here void led_on() { P0=0x00; delay1s(); P0=0xff; delay1s(); }
The code of the Main function is as follows:
#include#include “delay1s.h”void main() { led_on(); delay1s(); //In fact, only the first sentence is enough here, this sentence is unnecessary led_on(); //This is also unnecessary}
In this function, in order to explain again the definition of the #ifndef...#define...#endif structure, you can remove this structure from all .h files and then compile it to see the effect.
I believe that everyone has a general understanding of this modular writing method. If we want to add a new function, such as a running light function, then we only need to add a led_circle.c and led_circle.h, and then add them to the project according to the above steps. The rest of the program does not need to be changed. Obviously, this is very convenient. In fact, the extern keyword can be used in the function declaration. This type is the default in C language, so it is not necessary to write it.
If there is anything that is not clear, please raise it and we will discuss it together. Since these things are entered manually, there will inevitably be errors. If you find any errors or inappropriateness in this tutorial, please point it out and I will correct it in time to avoid misleading others, hehe.
Finally, I attach a reference example, which can be used directly on the CEPARK 51 board to see the experimental results. I hope this can be helpful to everyone.
Previous article:MCU C language modular programming
Next article:C51 Programming Specifications
Recommended ReadingLatest update time:2024-11-16 13:49
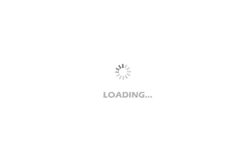
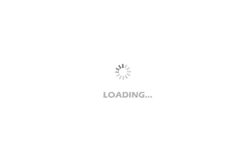
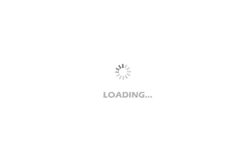
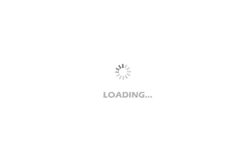
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
Single-chip microcomputer C language programming and simulation
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Single chip microcomputer control technology (Li Shuping, Wang Yan, Zhu Yu, Zhang Xiaoyun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- I called Knowles today and it was hilarious.
- Is there any sensor that can detect whether there is someone in front through the tinted glass?
- Should the filter capacitor and bleeder resistor in the circuit be placed before or after the anti-reverse polarity diode?
- Which teacher can explain this circuit?
- [2022 Digi-Key Innovation Design Competition] 1. STM32F7508-DK Unboxing
- Some predictions about the national competition questions - about power supply questions
- RFID low frequency and high frequency antenna technology
- First look at Texas Instruments' C2000 series
- What is UWB and why is it in my phone? Ultra-wideband technology, explained
- Can the TF card that comes with SensorTile.box be used?