//FileName:delay.h
#ifndef __LZP_DELAY_H_
#define __LZP_DELAY_H_
#define TRUE 1
#define FALSE 0
//Set the baud rate
#define OSC_FREQ 11059200L
#define BAUD_115200 256 - (OSC_FREQ/192L)/115200L // 255
#define BAUD_57600 256 - (OSC_FREQ/192L)/57600L // 254
#define BAUD_38400 256 - (OSC_FREQ/192L)/38400L // 253
#define BAUD_28800 256 - (OSC_FREQ/192L)/28800L // 252
#define BAUD_19200 256 - (OSC_FREQ/192L)/19200L // 250
#define BAUD_14400 256 - (OSC_FREQ/192L)/14400L // 248
#define BAUD_9600 256 - (OSC_FREQ/192L)/9600L // 244
// Timer2
#define RCAP2_50us 65536L - OSC_FREQ/240417L
#define RCAP2_1ms 65536L - OSC_FREQ/12021L
void delay_ms(unsigned int num);
void delay_50us(unsigned char num);
void delay_us(unsigned char num);
#endif
//FileName:delay.c
/********************************************
** start51 study board
** delay function implementation
** author:bluehacker
** QQ:282074921
**********************************************/
#include "delay.h"
#include "reg52.h"
void delay_ms(unsigned int num)
{
RCAP2H = (RCAP2_1ms>>8);
RCAP2L=(RCAP2_1ms&0x00ff);
TH2=(RCAP2_1ms>>8);;
TL2=(RCAP2_1ms&0x00ff);;
ET2 = 0; // Disable timer2 interrupt
T2CON = 0x04; // 16-bit auto-reload, clear TF2, start timer
while (num--)
{
while (!TF2);
TF2 = FALSE;
}
TR2 = FALSE;
}
void delay_50us(unsigned char num)
{
RCAP2H=(RCAP2_50us>>8);
RCAP2L=(RCAP2_50us&0x00ff);
TH2=(RCAP2_50us>>8);
TL2=(RCAP2_50us&0x00ff);
ET2=0;
T2CON=0x04;
while(num--)
{
while(!TF2)
TF2=FALSE;
}
TR2=FALSE;
}
void delay_us(unsigned char num)
{
unsigned char i;
for (i=0;i
}
}
//FileName:lcd.h
#ifndef __LZP_LCD_H_
#define __LZP_LCD_H_
#include "reg52.h"
/////////////////////////////
//定义LCD控制引脚
////////////////////////////
sbit LCDRS="P2"^0;
sbit LCDRW="P2"^1;
sbit LCDE="P2"^2;
void lcd_write_cmd(unsigned char cmd);
void lcd_write_data(unsigned char dat);
void lcd_clear(void);
void lcd_init(void);
unsigned char lcd_status(void);
void lcd_set_mode(unsigned char cursor, unsigned char text);
void lcd_write_str(unsigned char x,unsigned char y,unsigned char *s);
void lcd_write_char(unsigned char x,unsigned char y, unsigned char d);
#endif
//FileName:lcd.c
/************************************************************
**This development board supports 1602 character LCD
**You can find a lot of interface information for this LCD by using Google
** Pin definition:
* 1---GND
* 2---VDD
* 3---VLCD: Contrast adjustment
* 4---RS: Register selection, select data register when high level, select instruction register when low level
* 5---R/W: Read and write signal line, read operation when high level, write operation when low level.
When RS and RW are both low level, instructions can be written or display addresses can be displayed. When RS is low level and
RW is high level, busy signal can be read. When RS is high level and RW is low level, data can be written.
* 6---E: Enable terminal. When E terminal jumps from high level to low level, the LCD module executes the command.
* 7~14---DB0~DB7 data lines,
* 15---A: backlight pin, "A" connects to positive
* 16---K: backlight pin, "K" connects to negative
**************************************************************/
#include "lcd.h"
#include "delay.h"
#include "intrins.h"
/*向LCD写入命令*/
void lcd_write_cmd(unsigned char cmd)
{
unsigned char status;
P0=cmd;
LCDRS="0";
LCDRW="0";
LCDE="0";
delay_us(5);
do{
status="lcd"_status();
}while(status&0x80);
LCDE="1";
}
void lcd_write_data(unsigned char dat)
{
unsigned char status;
P0=dat;
LCDRS="1";
LCDRW="0";
LCDE="0";
delay_us(5);
do{
status="lcd"_status();
}while(status&0x80);
LCDE="1";
}
void lcd_clear(void)
{
lcd_write_cmd(0x01);
}
/*显示屏字符串写入函数*/
void lcd_write_str(unsigned char x,unsigned char y,unsigned char *s)
{
if (y == 0) {
lcd_write_cmd(0x80 + x);
}
else {
lcd_write_cmd(0xC0 + x);
}
while (*s) {
lcd_write_data( *s);
s ++;
}
/*
unsigned char i;
for(i=0;i<16&&s!=0;i++)
{
lcd_write_char(x+i,y,s);
}
*/
}
void lcd_write_char(unsigned char x,unsigned char y, unsigned char d)
{
if(y==0)
{
lcd_write_cmd(0x80+x);
}
else
{
lcd_write_cmd(0xc0+x);
}
lcd_write_data(d);
}
//光标复位
void lcd_reset_cursor(void)
{
lcd_write_cmd(0x02);
}
//Set the display mode
void lcd_set_mode(unsigned char cursor, unsigned char text)
{
unsigned char mode="0x04";
if(cursor){//Cursor moves right
mode|=0x02;
}
else{//Cursor moves left
mode|=0x00;
}
if(text){//Text moves
mode|=0x01;
}
else{//Text does not move
mode|=0;
}
lcd_write_cmd(mode);
}
void lcd_init(void)
{
P0=0;
LCDE="1";
delay_ms(500);
lcd_clear();
lcd_write_cmd(0x38);//Set LCD function: 8-bit bus, dual-line display, 5X7 dot matrix character
lcd_write_cmd(0x0f);//Display switch control: display ON, cursor ON, blink ON
lcd_write_cmd(0x06);//Cursor input mode incremental shift
lcd_write_cmd(0x80);
//lcd_write_cmd(0x0c);
//lcd_clear();
}
//Read status, busy or not
unsigned char lcd_status(void)
{
unsigned char tmp="0";
P0=0xff;
LCDRS="0";
LCDRW="1";
LCDE="0";
_nop_();
LCDE="1";
//_nop_();
tmp="P0";
return tmp;
}
//FileName:test.c
/*******************************
** Start51 study board test software
** test 1602LCD
** author: bluehacker
** QQ:282074921
***********************************/
#include "lcd.h"
#include "delay.h"
#include "intrins.h"
void main(void)
{
//初始化串口
SCON="0x50";/*mode 1,1 start bit ,8 data bit ,1 stop bit,enable receive*/
PCON="0x80";/*SMOD=1, Baud Rate twice*/
TMOD="0x21";/*timer 0--mode 1 and timer 1 ---mode2*/
//set baud rate,use timer 1 as baud rate generator
TH1=BAUD_19200;
TL1=TH1;
TR1=TRUE;
ET1=FALSE;//disable timer 1 interrupt
EA="0";
//delay_ms(400);
lcd_init();
// lcd_set_mode(1,0);
//lcd_reset_cursor();
// lcd_write_char(3,1,'c');
lcd_write_str(2,0,"bluehacker");
lcd_write_str(2,1,"QQ:282074921");
while(1)
{
}
}
Previous article:51 MCU low power working mode
Next article:192*64 LCD driver written by Keil C51
Recommended ReadingLatest update time:2024-11-16 22:22
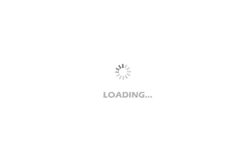
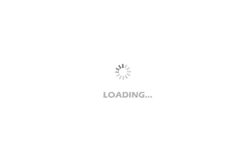
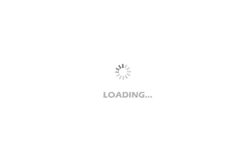
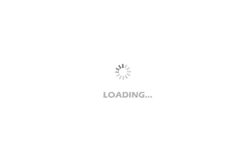
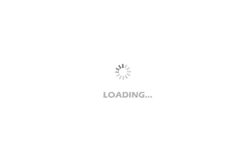
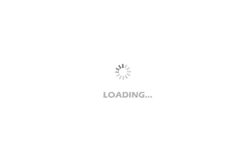
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- First look at TMS320C5410
- [Mill MYB-YT507 development board trial experience] + build QT development environment and test, the most detailed steps to solve all troubles
- [Repost] Advantages and process of dry etching
- Microphone noise problem
- How to ensure IoT security
- LTC2323-12 chip does not work
- A brief analysis of the power amplifier in RF chips
- 【ST NUCLEO-G071RB Review】_04_UART Experiment
- ELT8629 chip
- MicroPython driver for non-volatile memory