MMU: Memory Management Unit. Function:
(1) The mapping of virtual address to physical address makes each process have the same address space.
(2) Check memory access permissions (implemented by the hardware itself). Protect the memory used by each process from being destroyed by other processes.
In a 32-bit CPU, the virtual memory address is 0~0xFFFF_FFFF.
Cache: A high-speed cache memory between the main memory and the CPU.
Detailed code explanation: (refer to Brother Wei Dongshan's code)
(1) head.S
@**************************************************************************** |
(2)init.c
/* |
(3) leds.c
/* |
(4)mmu.lds
SECTIONS { |
(5) Makefile
objs := head.o init.o leds.o |
Previous article:S3C2440 Hardware Part 4: NandFlash (1) Introduction
Next article:S3c2440 Hardware Part 2: SDRAM
Recommended ReadingLatest update time:2024-11-16 22:24
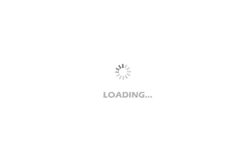
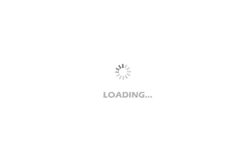
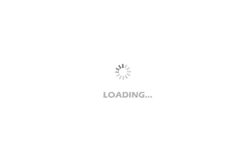
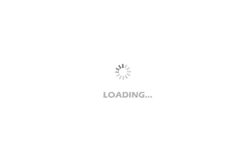
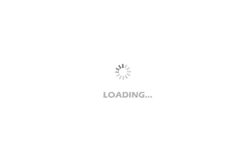
- Popular Resources
- Popular amplifiers
-
Semantic Segmentation for Autonomous Driving: Model Evaluation, Dataset Generation, Viewpoint Comparison, and Real-time Performance
-
Hardware Accelerators in Autonomous Driving
-
Raspberry Pi Development in Action (2nd Edition) ([UK] Simon Monk)
-
A common software/hardware approach for future advanced driver assistance systems
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- CCS Basics Tutorial
- 2021 ST Industrial Summit Tour starts to reserve seats: "Motor Drive, Power Supply, Industrial Automation" + "New Products, Courses, Training"
- Happy Dragon Boat Festival, salty rice dumplings, sweet rice dumplings, which kind do you like?
- [Topmicro Intelligent Display Module Review] 5. Development Board NUCLEO-F746ZG Communication with Intelligent Display Module
- TI MCU has launched a new product! August live broadcast reveals new features video replay summary!
- Please restore the number of invitees to 8. Thank you. Thank you. I invited 8 people before, but there are already 6 people in the list.
- Experts talk about IPC7351
- 5G commercial use is on the way, are you ready?
- Chat with Vicor engineers about efficient power supply and learn about modular power solutions for drones!
- Download the practical guide - Motor Control Basics