#include
#include
#define lcd_bus P0 // data bus
sbit rs =P2^4; // data & instruction selection, H: write data, L: write instruction
sbit rw =P2^3; // read & write selection, H:read, L:write
sbit e =P2^2; // read and write enable
sbit bf =P0^7; // busy and idle status flag, H: internal execution, L: idle
void chk_busy(void); // detect LCD busy and idle
void init_lcd(void); // LCD initialization
void wr_comm(unsigned char comm); // write instruction
void wr_comm_no(unsigned char comm); // write instruction, do not detect busy and idle
void wr_da
void wr_str(unsigned char *p); // Display string
unsigned char rd_lcd(void); // Read LCD data
void delayus(unsigned char us); // Delay subroutine us
void delayms(unsigned int ms); // Delay subroutine ms
void main()
{
delayms(200);
init_lcd(); // LCD initialization
wr_comm(0x80); // The first character address of the first line
wr_str("lcd1602");
wr_comm(0xc0); // The first character address of the second line
wr_str("Tai shan Dizn Zi");
while(1);
}
/*------------------LCD initialization-----------------*/
void init_lcd(void)
{
wr_comm_no(0x38); // Do not detect busy or idle
delayms(5);
wr_comm_no(0x38);
delayms(5);
wr_comm_no(0x38);
delayms(5);
wr_comm_no(0x38);
delayms(5);
wr_comm(0x38); // Set LCD to 16*2 display, 5*7 dot matrix, 8-bit data interface, detect busy signal
delayus(3); // Delay 11us
wr_comm(0x08); // Turn off the display, detect the busy signal
delayus(3);
wr_comm(0x01); // Clear the screen, detect the busy signal
delayus(3);
wr_comm(0x06); // Display cursor automatically moves right, the whole screen does not move, detect the busy signal
delayus(3);
wr_comm(0x0c); // Turn on the display, do not display the cursor, detect the busy signal
delayus(3);
}
/*--------------Detect LCD busy---------------*/
void chk_busy(void)
{
lcd_bus=0xff;
rs=0;
rw=1;
;
e=1;
while(bf==1);
e=0;
}
/*------------Write command to LCD--------------*/
void wr_comm(unsigned char comm)
{
chk_busy();
rs=0; // H: write data, L: write instruction
rw=0;
e=0;
;
lcd_bus=comm; // content
delayus(3);
e=1;
;
e=0;
}
/*------------write command to LCD without detecting busy/idle--------------*/
void wr_comm_no(unsigned char comm)
{
rs=0; // H: write data, L: write instruction
rw=0;
e=0;
;
lcd_bus=comm; // content
delayus(3);
e=1;
;
e=0;
}
/*------------write data to LCD--------------*/
void wr_dat
{
chk_busy();
rs=1; // H: write data, L: write instruction
rw=0;
e=0;
;
lcd_bus=dat; // content
delayus(3);
e=1;
;
e=0;
}
/*--------------read LCD data---------------*/
unsigned char rd_lcd(void)
{
unsigned char rd_da
chk_busy(); // Detect busy
rs=1;
rw=1;
e=1;
;
rd_da
e=0;
return rd_da
}
/*-------------write string----------------*/
void wr_str(unsigned char *s)
{
while(*s>0) // the string ends with 0
{
wr_data
s++;
}
}
/*---------------delay subroutine us----------------*/
void delayus(unsigned char us)
{
while(--us); // a cycle of 2us
}
/*---------------ms delay subroutine----------------*/
void delayms(unsigned int ms) //delay n ms
{
while(ms)
{
int i;
i=110;
while(i--);
ms=ms-1;
}
}
Previous article:12864 (with font library) Display graphics and Chinese characters-51 program
Next article:ATMEGA16 serial port sending and interrupt receiving CVAVR program
Recommended ReadingLatest update time:2024-11-16 12:49
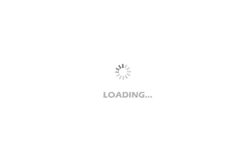
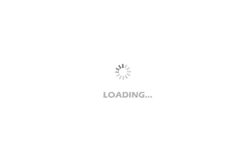
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Single-chip microcomputer C language programming and simulation
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Have you been affected by the price increase? Share the best pin-compatible alternative solution under the wave of price increase#, a certain brand?
- Request: DC-DC small package chip similar to LM2596
- What is the reason! Another wind turbine fire accident! And caused a ground wildfire
- 【Iprober 520 current probe】Practical use in motor control
- Can you please help me find out where is the problem with the program?
- How to add c files in esp32 idf components to compile
- Have you noticed the LPDSP32 audio codec inside RSL10? Does your project use this resource?
- Will the push-pull circuit be straight through?
- Award-winning live broadcast: Third-generation TI C2000 new features resource update
- EEWORLD University - Industrial Internet of Things using CC1310 sub-1 GHz wireless MCU