It consists of the test program
/* ************************************************
* File name: main.c
* Function: AVR's UART data receiving and sending test program
* Description: Forward the data received by the RXD port to the TXD port
* The receiving and sending work is automatically completed by the UART interrupt
* Author & Date: Joshua Chan, 2012/03/28
* ************************************************/
#include
#include
#include
#include
#include
#include "uart_test.h"
/* Test program: Send the data received by the serial port as is*/
void main(void)
{
unsigned char data;
fifo_pos_init(); /* Reset position record*/
uart_init(B9600); /* Initialize UART */
_SEI();
while (1) {
if ((data = rx_byte()) != NONE_DATA)
tx_byte(data);
_WDR(); /* Feed the dog*/
}
}
/* ************************************************
* File name: uart_test.h
* Function: AVR's UART data receiving and sending test program
* Description: Forward the data received by the RXD port to the TXD port
* The receiving and sending work is automatically completed by the UART interrupt
* Author & Date: Joshua Chan, 2012/03/28
* ************************************************/
#ifndef _UART_TEST_H
#define _UART_TEST_H
/* Define the UBRR value when FOSC=16.0MHz*/
__flash enum baudrate {
B2400 = 416,
B4800 = 207,
B9600 = 103,
B14400 = 68,
B19200 = 51,
B28800 = 34,
B38400 = 25,
B57600 = 16,
B76800 = 12,
B115200 = 8,
B230400 = 3,
};
#define NONE_DATA '\4' /* No new data flag*/
#define RX_BUF_SIZE 32 /* Receive buffer size*/
#define TX_BUF_SIZE 32 /* Transmit buffer size*/
/* Reset position record*/
extern void fifo_pos_init(void);
/* UART initialization*/
extern void uart_init(unsigned int baud);
/* Read 1 byte from the receive buffer*/
extern unsigned char rx_byte(void);
/* Send 1 byte of data to the transmit buffer and enable the data register empty interrupt*/
extern void tx_byte(unsigned char data);
#endif
/* ************************************************ * File name: uart_test.c * Function: AVR UART data receiving and sending test program * Description: Forward the data received by the RXD port to the TXD port * Transmission and reception are automatically completed by UART interrupt * Author & Date: Joshua Chan, 2012/03/28 * ************************************************/ #include#include #include #include #include #include "uart_test.h" unsigned char rx_fifo[RX_BUF_SIZE]; /* Receive buffer */ unsigned char tx_fifo[TX_BUF_SIZE]; /* Send buffer */ unsigned char rx_pos; /* The position of the last received data in the buffer*/ unsigned char read_pos; /* The position of the last data read in the buffer*/ unsigned char tx_pos; /* The position of the last transmitted data in the buffer*/ unsigned char write_pos; /* The position of the last written data in the buffer*/ /* UART initialization */ void uart_init(unsigned int baud) { /* Disable USART function first */ UCSR0B = 0x00; /* Set baudrate */ UBRR0H = (unsigned char)(baud>>8); UBRR0L = (unsigned char)baud; /* Set frame format: 8 data bits, 1 stop bit*/ UCSR0C = 3 << UCSZ00; /* Enable: receive end interrupt, receive, send*/ UCSR0B = (1<
Previous article:Programming Tips: 16-bit AVR timer compare match interrupt test program
Next article:Programming Tips: 8-bit AVR timer compare match interrupt test program
Recommended ReadingLatest update time:2024-11-16 13:40
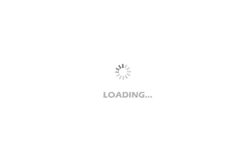
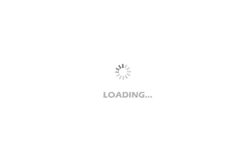
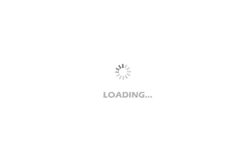
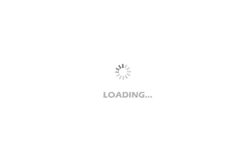
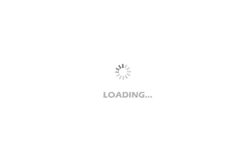
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- FAQ: PolarFire SoC FPGA Secure Boot | Microchip Security Solutions Seminar Series 12
- Some Problems on Measuring AC Current with Current Transformer
- Simulation of staying up late is harmful to health
- [RVB2601 Creative Application Development] + RTC Clock Display Experiment
- From traditional substation to smart substation
- [Raspberry Pi Pico Review] Xiaohui Review
- ST sensor evaluation platform STEVAL_MKI109V3 development board
- Analog Circuit Design Handbook: Advanced Application Guide
- How do you spend the May Day holiday?
- [Chuanglong Technology Allwinner A40i Development Board] Qt performance test