* Version..........: 1.0
* Author..........: Chen Lidong
* Target.......: ATmega128
* File name..........: UART.h
* Compiler.....: IAR for AVR V5.5
* Creation time.....: 2010.10.14
* Last modified.....: 2010.10.14
******************************************/
#ifndef __UART_H__
#define __UART_H__
#include
#include "../main.h" /* F_CPU */
#define UART_BAUD 9600 //波特率
#define UART_TXBUF_LENGTH 500
#define UART_RXBUF_LENGTH 500
struct UART_BUF
{
volatile unsigned char data;
volatile struct UART_BUF *next;
};
extern void UART_Init(void);
extern void UART_TxByte(unsigned char _data);
extern void _enable_uart_interrupt(void);
extern void _disable_uart_interrupt(void);
extern volatile struct UART_BUF UART_TxBuf[UART_TXBUF_LENGTH], UART_RxBuf[UART_RXBUF_LENGTH];
extern volatile struct UART_BUF *p_TxBuf_Head, *p_TxBuf_Tail, *p_RxBuf_Head, *p_RxBuf_Tail;
#endif /* __UART_H__ */
* Version..........: 1.0
* Author..........: Chen Lidong
* Target.......: ATmega128
* File name..........: UART.c
* Compiler.....: IAR for AVR V5.5
* Creation time.....: 2010.10.14
* Last modified.....: 2010.10.14
******************************************/
#include "UART.h"
volatile struct UART_BUF UART_TxBuf[UART_TXBUF_LENGTH], UART_RxBuf[UART_RXBUF_LENGTH];
volatile struct UART_BUF *p_TxBuf_Head, *p_TxBuf_Tail, *p_RxBuf_Head, *p_RxBuf_Tail;
void UART_Init(void)
{
UBRR0L = (F_CPU / 16L / UART_BAUD - 1);
UCSR0B_RXEN0 = 1;
UCSR0B_TXEN0 = 1;
UCSR0B_RXCIE0 = 1;
UCSR0B_TXCIE0 = 1;
unsigned int i = 0;
//
for (i = 0; i < UART_TXBUF_LENGTH - 1; i++)
{
UART_T xBuf[i].next = &UART_TxBuf[i + 1];
}
UART_TxBuf[UART_TXBUF_LENGTH - 1].next = &UART_TxBuf[0];
p_TxBuf_Head = &UART_TxBuf[0];
p_TxBuf_Tail = &UART_TxBuf[0];
//
for (i = 0; i < UART_RXBUF_LENGTH - 1; i++)
{
UART_RxBuf[i].next = &UART_RxBuf[i + 1];
}
UART_RxBuf[UART_RXBUF_LENGTH - 1].next = &UART_RxBuf[0];
p_RxBuf_Head = &UART_RxBuf[0];
p_RxBuf_Tail = &UART_ RxBuf[0];
__enable_interrupt();
}
void UART_TxByte(unsigned char _data)
{
if (UCSR0A_UDRE0 == 1 && p_TxBuf_Head == p_TxBuf_Tail)
{
UDR0 = _data;
}
else
{
if (p_TxBuf_Tail->next != p_TxBuf_Head)
{
p_TxBuf _Tail->data = _data;
p_TxBuf_Tail = p_TxBuf_Tail->next;
}
}
}
void _enable_uart_interrupt(void)
{
UCSR0B_RXCIE0 = 1;
UCSR0B_TXCIE0 = 1;
}
void _disable_uart_interrupt(void)
{
UCSR0B_RXCIE0 = 0;
UCSR0B_TXCIE0 = 0;
}
#pragma vector = USART0_TXC_vect
__interrupt void USART0_TXC(void)
{
if (UCSR0A_UDRE0 == 1 && p_TxBuf_Head != p_TxBuf_Tail)
{
UDR0 = p_TxBuf_Head->data;
p_TxBuf_Head = p_TxBuf_Head->next;
}
}
#pragma vector = USART0_RXC_vect
__interrupt void USART0_RXC(void)
{
if (p_RxBuf_Tail->next != p_RxBuf_Head)
{
p_RxBuf_Tail->data = UDR0;
p_RxBuf_Tail = p_RxBuf_Tail->next;
}
}
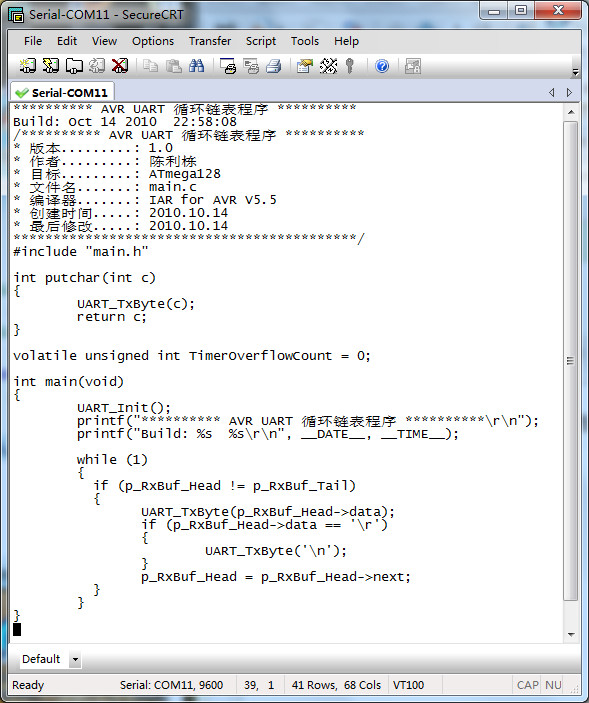
Previous article:AVR serial port transmission and reception using ring buffer program source code
Next article:AVR matrix keyboard program source code V3.5 (with continuous key function and combination key function)
Recommended ReadingLatest update time:2024-11-16 13:41
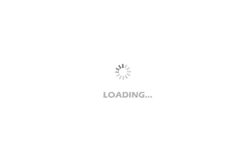
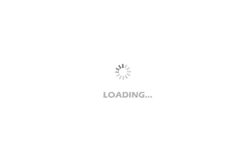
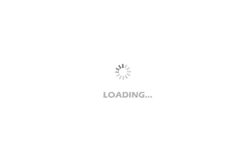
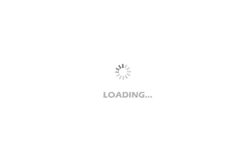
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Metronom Real-Time Operating System RTOS for AVR microcontrollers
-
Learn C language for AVR microcontrollers easily (with video tutorial) (Yan Yu, Li Jia, Qin Wenhai)
-
ATmega16 MCU C language programming classic example (Chen Zhongping)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- dsp DM642 uses timer to light up LED source program
- [2022 Digi-Key Innovation Design Competition] I am just an image porter
- Nengdian Electronics Capacitive Liquid Level Sensor D1CS-D54 Review
- [Zhongke Bluexun AB32VG1 RISC-V board "meets" RTT] GPIO simulation realizes full-color LED lights
- Solution | Application of Feiling FETMX8MP-C core board in AGV car
- What will happen if you connect the input and output of the power bank?
- [Silicon Labs Development Kit Review] TensorFlow Application Code Analysis Based on Deep Learning
- Direction of electrical energy transfer in a circuit
- Detailed explanation of the BQ25618/9 dedicated switch charging chip for TWS true wireless headset charging compartment
- Share a reference implementation of path planning, the key is that there are animated pictures!