//*****************************USART Control and Status Register A (UCSRA)********************************
/*USART Control and Status Register A (UCSRA)
bit7 bit6 bit5 bit4 bit3 bit2 bit1 bit0
RXC TXC UDRE FE DOR PE U2X MPCM
RXC: USART receive end
RXC is set when there is unread data in the receive buffer, otherwise it is cleared.
The RXC flag can be used to generate a receive end interrupt.
TXC: USART transmit end
The data in the transmit shift buffer is sent out and TXC is set when the transmit buffer (UDR) is empty.
The TXC flag is automatically cleared when the interrupt ends, and can also be cleared by writing 0. The TXC flag can be used to generate a transmission
Transmit end interrupt (see the description of the TXCIE bit).
UDRE: USART Data Register Empty
The UDRE flag indicates whether the transmit buffer (UDR) is ready to receive new data. If UDRE is 1, the buffer is empty.
Ready to receive data. The UDRE flag can be used to generate a data register empty interrupt
After reset, UDRE is set, indicating that the transmitter is ready.
FE: Frame Error
If the next character received in the receive buffer has a frame error, the first
If the stop bit is 0, FE is set. This bit is valid until the receive buffer (UDR) is read.
When the stop bit is 1, the FE flag is 0. When writing to UCSRA, this bit should be written as 0.
DOR: Data Overflow
DOR is set when data overflows. When the receive buffer is full (contains two data), and there is data in the receive shift register,
If a new start bit is detected at this time, data overflow occurs. This bit is valid until the receive buffer
(UDR) is read. When writing to UCSRA, this bit should be written as 0.
PE: Parity Error
When parity is enabled (UPM1 1) and the next character received in the receive buffer has a parity error
UPE is set. This bit remains valid until the receive buffer (UDR) is read. This bit is set when UCSRA is written.
Write 0 for each digit.
U2X: Send at twice the speed
This bit has an effect only for asynchronous operation. Clear this bit to 0 when using synchronous operation.
The bit is reduced from 16 to 8, effectively doubling the transfer rate of the asynchronous communication mode.
MPCM: Multiprocessor Communication Mode
Setting this bit enables multiprocessor communication mode. When MPCM is set, the USART receiver receives
Any incoming frames with address information will be ignored. The transmitter is not affected by the MPCM setting.
*/
//***************************USART Control and Status Register B (UCSRB)*******************
/* USART Control and Status Register B (UCSRB)
bit7 bit6 bit5 bit4 bit3 bit2 bit1 bit0
RXCIE TXCIE UDRIE RXEN TXEN UCSZ2 RXB8 TXB8
RXCIE: Receive end interrupt enable
When RXCIE is 1, the global interrupt flag SREG is set, and the RXC in the UCSRA register is
When it is 1, a USART receive end interrupt can be generated.
TXCIE: Transmit end interrupt enable
When TXCIE is 1, the global interrupt flag SREG is set, and the TXC flag of the UCSRA register is set.
When it is 1, a USART transmission end interrupt can be generated.
UDRIE: USART Data Register Empty Interrupt Enable
When UDRIE is 1, the global interrupt flag SREG is set, and the UDRE bit in the UCSRA register is
When it is 1, a USART data register empty interrupt can be generated.
RXEN: Receive Enable
When set, the USART receiver is enabled. The general port function of the RxD pin is replaced by the USART function.
The receiver will flush the receive buffer and invalidate the FE, DOR, and PE flags.
TXEN: Transmit Enable
When set, the USART transmitter is started. The general port function of the TxD pin is replaced by the USART function.
After TXEN is cleared, the transmitter can be disabled only after all data has been sent.
After the transmitter is disabled, the TxD pin returns to its normal state.
I/O functions.
UCSZ2: character length
UCSZ2 and UCSZ1:0 of the UCSRC register can be combined to set the number of data bits (characters) contained in the data frame.
length).
RXB8: Receive data bit 8
When operating on a 9-bit serial frame, RXB8 is the 9th data bit. Before reading the low-order data contained in UDR, first
To read RXB8.
TXB8: Transmit data bit 8
When operating on a 9-bit serial frame, TXB8 is the 9th data bit. Before writing UDR, it must be written first.
*/
//********************************Status register C (UCSRC)********************************
/* Status register C (UCSRC)
bit7 bit6 bit5 bit4 bit3 bit2 bit1 bit0
URSEL UMSEL UPM1 UPM0 USBS UCSZ1 UCSZ0 UCPOL
The UCSRC register shares the same I/O address as the UBRRH register. Access to this register.
URSEL: Register Select
This bit is used to select access to the UCSRC register or the UBRRH register. When reading UCSRC, this bit is 1; when writing UCSRC,
When URSEL is 0, the UBRRH value is updated; if URSEL is 1, the UCSRC setting is updated
UMSEL: USART mode selection
This bit is used to select synchronous or asynchronous operation mode.
UMSEL Settings
UMSEL Mode
0 Asynchronous Operation
1 Synchronous Operation
UPM1:0: Parity mode
These two bits set the parity check mode and enable parity check. If parity check is enabled, then
For each received data, the transmitter will automatically generate and send the parity bit.
Generate a parity value and compare it with the value set in UPM0. If they do not match, then the UCSRA
The PE bit is set.
UPM Settings
UPM1 UPM0 Parity mode
0 0 Prohibited
0 1 Reserved
1 0 Even parity
1 1 Odd parity
USBS: Stop bit selection
This bit can be used to set the number of stop bits. The receiver ignores the setting of this bit.
USBS Settings
USBS stop bits
0 1
1 2
UCSZ1:0: character length
UCSZ1:0 and UCSZ2 of the UCSRB register can be combined to set the number of data bits contained in the data frame (word
character length).
UCSZ Settings
UCSZ2 UCSZ1 UCSZ0 Character length
0 0 0 5
0 0 1 6
0 1 0 7
0 1 1 8
1 0 0 Reserved
1 0 1 Reserved
1 1 0 Reserved
1 1 1 9
UCPOL: Clock polarity
This bit is used only in synchronous mode of operation. When using asynchronous mode, clear this bit to 0. UCPOL sets the output
The relationship between data changes and input data sampling, and the synchronization clock XCK.
UCPOL Settings
UCPOL Transmit data change (output of TxD pin) Receive data sampling (input of RxD pin)
0 XCK rising edge XCK falling edge
1 XCK falling edge XCK rising edge
*///*************************************USART baud rate register (UBRRL and UBRRH)****************************
/* USART baud rate register (UBRRL and UBRRH)
bit15 bit14 bit13 bit12 bit11 bit10 bit9 bit8
URSEL – – – UBRR[11:8]
UBRR[7:0]
The UCSRC register shares the same I/O address as the UBRRH register.
URSEL: Register selection
This bit is used to select access to the UCSRC register or the UBRRH register. When reading UBRRH, this bit is 0; when writing
When UBRRH, URSEL is 0.
Bit 14:12 – Reserved
These bits are reserved for future use. To be compatible with future devices, write UBRRH to clear these bits.
UBRR11:0: USART Baud Rate Register
This 12-bit register contains the baud rate information of the USART. UBRRH contains the high 4 bits of the USART baud rate.
UBRRL contains the lower 8 bits. Changing the baud rate will cause the ongoing data transmission to be disrupted. Writing UBRRL
The baud rate divider will be updated immediately.
*/
/*
Baud rate is defined as the speed of bit transmission per second (bps)
BAUD baud rate (bps)
fOSC system clock frequency
UBRR UBRRH and UBRRL values (0-4095)
Asynchronous normal mode (U2X = 0)
FOSC FOSC
BAUD = -------------- UBRR = ------------ -1
16( UBRR+ 1) 16BAUDAsynchronous
double speed mode (U2X = 1)
fOSC fOSC
BAUD = -------------- UBRR = ------------ -1
8(UBRR+ 1) 8BAUDSynchronous
master mode
fOSC fOSC
BAUD = --------------- UBRR = ------------ -1
2(UBRR+ 1) 2BAUD
*/
#include
#include
#pragma interrupt_handler UDR_empty:iv_USART_UDRE
#pragma interrupt_handler RXC_END:iv_USART_DRE
#pragma interrupt_handler TXC_END:iv_USART_TX
unsigned char UASART_DATA=0; void USART_Init_commonage(unsigned int baud) // common initialization {/* Set baud rate */ SREG&=0x7F; } /* UASART_DATA=UDR; //add your code here void USART_Transmit5_8( unsigned char data )//Use polling method to send data for UDRE flag (send 5-8 bits of data) unsigned char USART_Receive5_8( void )//Receive 5-8 bits of data by polling RXC /* {
void USART_Init( unsigned int baud,unsigned char digit,unsigned char mode,unsigned char checkout) //Detailed initialization mode
{ SREG&=0x7F;
UCSRC&=~(1<
UBRRH = (unsigned char)(baud>>8);
UBRRL = (unsigned char)baud;
/* Receiver and transmitter enable*/
UCSRB = (1<
UCSRB = (1<
switch(mode) //different mode selection
{
case 0:UCSRB&=~(1<
UCSRC|=1<
the 9th bit of the data should be written to TXB8 of register UCSRB first,
and then the lower 8 bits of data should be written to the transmit data register UDR, */
switch(digit) //// Set the frame format: digit data bit 5-9,
{
case 5:UCSRB&=~(1<
switch(checkout)//checkout verification mode
{
case 0:UCSRC&=~(1<
UCSRC|=1<
//UCSRA|=1<
}
/************UBRR baud setting parameter table****************************/
//U2X=0;8mhz crystal oscillator 9600: UBRR=51;Error: 0.2%. 4800: UBRR=103;Error: 0.2%. 2400: UBRR=207;Error: 0.2%
//U2X=1;8mhz crystal oscillator 9600: UBRR=103;Error: 0.2%. 4800: UBRR=207;Error: 0.2%. 2400: UBRR=416;Error: -0.1%
//U2X=0;11.0592mhz crystal oscillator 9600: UBRR=71;Error: 0.0%. 4800: UBRR=143; Error: 0.0%. 2400: UBRR=287; Error: 0.0%
//U2X=1; 11.0592mhz crystal oscillator 9600: UBRR=143; Error: 0.0%. 4800: UBRR=287; Error: 0.0%. 2400: UBRR=575; Error: 0.0%
UBRRH = (unsigned char)(baud>>8);
UBRRL = (unsigned char)baud;
/* Receiver and transmitter enable data register empty enable disable*/
UCSRB = (1<
UCSRC = (1<
SREG|=0x80;//Enable global interrupt
}
USART data register empty flag UDRE and transmission end flag TXC, both flags can generate interrupts.
*/
void UDR_empty()
//Enable requirements: global interrupt enable, data register empty interrupt enable bit UDRIE is set. UDRE is set (automatically).
//Writing to register UDR will clear UDRE
{unsigned char data;
UDR = data;
//add your code here
}
void TXC_END()
{UDR =UASART_DATA;
//add your code here
}
void RXC_END()
{
}
{ /* Wait for the transmit buffer to be empty*/
while ( !( UCSRA & (1<
UDR = data;
}
void USART_Transmit_9( unsigned int data )//Use polling method to send 9-bit data frame
{ /* Wait for the transmit buffer to be empty*/
while ( !( UCSRA & (1<
UCSRB &= ~(1<
UCSRB |= (1<
UDR = data;
}
{
/* Wait for receiving data*/
while ( !(UCSRA & (1<
return UDR;
}
unsigned int USART_Receive_9( void )//Receive 9-bit data frame
{
unsigned char status, resh, resl;
/* Wait for receiving data*/
while ( !(UCSRA & (1<
/* from buffer */
status = UCSRA;
resh = UCSRB;
resl = UDR;
/* Return if error occurs*/
if ( status & (1<
/* Filter the ninth bit of data and return*/
resh = (resh >> 1) & 0x01;
return ((resh << 8) | resl);
}
When the receiver is disabled, the buffer FIFO is flushed and the buffer is cleared. This causes the unread data to be lost. If the buffer must be flushed in normal operation due to an error,
then the UDR needs to be read until the RXC flag is cleared.
*/
void USART_Flush( void )
unsigned char dummy;
while ( UCSRA & (1<
Previous article:AVR microcontroller SPI serial peripheral interface initialization configuration and description
Next article:AVR microcontroller external interrupt 0, 1, 2 initialization configuration and description
Recommended ReadingLatest update time:2024-11-17 02:39
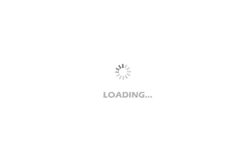
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- Please advise, where can I modify the USB device instance path generated by STM32?
- Msp430f149 microcontroller controls stepper motor C language program
- dsp reports an error after importing a new project!
- [NXP Rapid IoT Review] Adding Bluetooth Capabilities with NXP Rapid IoT
- On the saturation problem of operational amplifier circuit
- sdram_datasheet.pdf
- CC2640R2F hardware RF from design to production
- The book on switching power supplies states that because the capacitor is charged in each cycle, the current decline slope continues to increase. How do you understand this?
- [TI recommended course] #In-depth study of light load high efficiency and low noise power supply reference design for wearable devices and the Internet of Things (TIDA-01566)#
- Channel explanation in wireless communication