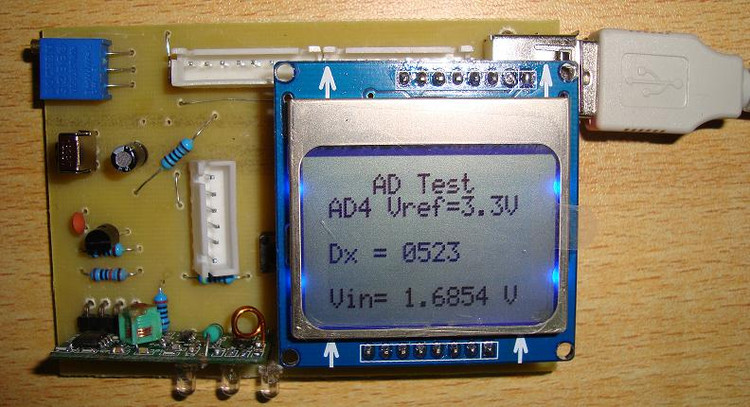
/*************PIC16F917 MCU program******************************/
/************************************************************************/
/*****File Function : AD acquisition, LCD display program *****/
/*****Program Author : ZhengWen(ClimberWin) *****/
/*****MCU : PIC16F917 internal crystal oscillator *****/
/*****Compile Date : 2010/08/18 *****/
/*****Edition Info : V1.0 *****/
/*********************************************************************/
//Measure AD and display it through LCD
//Pin definition: PORTD=8LED, KEY=RB0(INT) RA5(AN4) as AD input
/*Modification Date: */
/************************************/
#include
#include "english_6x8_pixel.h"
#define uchar unsigned char
#define uint unsigned int
void Init(void); //初始化子程序
void LCD_init(void); //LCD初始化程序
void LCD_clear(void);
void LCD_write_char(unsigned char c);
void LCD_set_XY(unsigned char X, unsigned char Y);
void LCD_write_english_string(unsigned char X,unsigned char Y,const char *s);
void LCD_write_byte(unsigned char data, unsigned char command);
void delayms(unsigned int count);
void interrupt ADint(void);
#define KEY RB0
#define SPI_CLK RD0
#define SPI_MOSI RD1
#define LCD_DC RD2
#define LCD_CE RD3
#define LCD_RST RD4
/***********************************************/
const unsigned char mask_table[8]={0x80,0x40,0x20,0x10,0x08,0x04,0x02,0x01};
float ad_data;//AD数据存储地址
uchar temp1,temp2;
uint temp3;
/*********************************************/
void delayms(unsigned int count)
{
uint i,j;
for(i=0;i
}
/*********************************************/
void Init(void)
{
PORTA = 0B00000000;
PORTB = 0B00000000;
PORTD = 0B00000000;
TRISA = 0B00100010; //Set RA5 (AN4) RA1 as input
TRISB = 0B00100001; //Set RB0 as input, as a key port
TRISD = 0B00000000; //Set PORTD as output, as LCD/LED display port
RD5=1; //Turn off LED
RD6=1;
RD7=1;
///////////AD configuration////////////////////////////////////////
ANSEL= 0B00010000; //Select AN4 as AD input (PDF 148)
ADCON0=0B10010001; //AD result format Right justified, select reference voltage VDD-VSS, AN4 input, turn off AD conversion
ADCON1=0B01010000; //AD conversion clock selection
ADIE=1;//AD interrupt enable
PEIE=1;
ADIF=0;//clear interrupt flag
GIE=1; //enable general interrupt
/////////////////////////////////////
LCD_init(); //initialize LCD
}
void interrupt ADint(void)
{
temp1=ADRESL;
temp2=ADRESH;
temp3=temp2*256+temp1; //Dx value
ad_data=(temp3*33000)/1024; //voltage value
ADIF=0; //clear interrupt flag
}
void LCD_init(void)
{
LCD_RST=0; //LCD reset
NOP();
LCD_RST=1;
LCD_CE=0 ; //Disable LCD
NOP();
LCD_CE=1; //Enable LCD
NOP();
LCD_write_byte(0x21, 0); // Use extended commands to set LCD mode
LCD_write_byte(0xc8, 0); // Set bias voltage
LCD_write_byte(0x06, 0); // Temperature correction
LCD_write_byte(0x13, 0); // 1:48
LCD_write_byte(0x20, 0); // Use basic commands
LCD_clear(); // Clear the screen
LCD_write_byte(0x0c, 0); // Set display mode, normal display
LCD_CE=0 ; // Turn off LCD
}
/////////LCD清屏程序/////////////
void LCD_clear(void)
{
uint i;
LCD_write_byte(0x0c, 0);
LCD_write_byte(0x80, 0);
for (i=0; i<504; i++)
LCD_write_byte(0x00, 1);//清零
}
///////////设置LCD坐标///////////////////
void LCD_set_XY(unsigned char X, unsigned char Y)
{
LCD_write_byte(0x40 | Y, 0);
LCD_write_byte(0x80 | X, 0);
}
/////////////////Character display program/////////////////////
void LCD_write_char(unsigned char c)
{
uint line;
c=c-32;
for (line=0; line<6; line++)
LCD_write_byte( font6x8[c][line], 1);
}
/////////////////打印字符串/////////////////////////
void LCD_write_english_string(unsigned char X,unsigned char Y, const unsigned char *s)
{
uchar i = 0;
LCD_set_XY(X,Y);
while(*s) {LCD_write_char(*s++);}
}
////////////Write data to LCD//////////////////////
void LCD_write_byte(unsigned char data, unsigned char command)
{
uchar i;
LCD_CE=0 ; // Enable LCD
if (command == 0)
{LCD_DC=0 ;} // Send commandelse
{
LCD_DC=1 ;} // Send datafor
(i=0;i<8;i++)
{
if(data&mask_table[i])
{SPI_MOSI=1;}
else
{SPI_MOSI=0;}
SPI_CLK=0;
NOP();
SPI_CLK=1;
}
LCD_CE=1; // Turn off LCD
}
/////////////Main program///////////////////////////
void main (void)
{
Init();//初始化程序
LCD_clear(); //LCD清屏
delayms(1000);
LCD_write_english_string(0,0," AD Test " );
LCD_write_english_string(0,1,"AD4 Vref=3.3V" );
ADCON0=ADCON0|0B00000010;//开始AD转换
LCD_set_XY(0,3);
LCD_write_char('D');
LCD_write_char('x');
LCD_write_char(' ');
LCD_write_char('=');
LCD_set_XY(0,5);
LCD_write_char('V');
LCD_write_char('i');
LCD_write_char('n');
LCD_write_char('=');
LCD_set_XY(72,5);
LCD_write_char('V');
while(1)
{
LCD_set_XY(30,3);
LCD_write_char((temp3/1000)+16+32);
LCD_write_char( (temp3%1000)/100+16+32);
LCD_write_char( ((temp3%1000)%100)/10+16+32);
LCD_write_char( ((temp3%1000)%100)%10+16+32);
//ad_data=ad_data*10000;
temp3=(uint)ad_data;
LCD_set_XY(30,5);
LCD_write_char((temp3/10000)+16+32);
LCD_write_char('.');
LCD_write_char( (temp3%10000)/1000+16+32);
LCD_write_char( ((temp3%10000)%1000)/100+16+32);
LCD_write_char( (((temp3%10000)%1000)%100)/10+16+32);
LCD_write_char( (((temp3%10000)%1000)%100)%10+16+32);
delayms(100);
ADCON0=ADCON0|0B00000010; //Start AD conversion
}
}
Previous article:PIC16F917 NOKIA5110 LCD arbitrary dot drawing program
Next article:PIC16F917 NOKIA5110 LCD test program
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Here is the camera information on the national competition list
- RF Components Test Technology Seminar for 5G - You are invited to attend!
- Has anyone designed this power supply?
- Network Control of ROS Melodic
- Let’s take a look at how this overvoltage protection circuit can be optimized?
- Inverter and Motor Control
- 【ST NUCLEO-H743ZI Review】(2) First experience with Ethernet testing
- Disassembling a common fire emergency light
- [NXP Rapid IoT Review] Mobile APP connection finally succeeded
- Buy an oscilloscope and get the essential analysis software 5-PWR for power engineers