**** AVR TWI read and write examples ***
**** ***
**** Author: HJJourAVR ***
**** Compiler: WINAVR20050214 ***
**********************************************/
/*
This program simply demonstrates how to use ATMEGA16's TWI to read and write AT24C02 IIC EEPROM
TWI protocol
(i.e. IIC protocol, please refer to the content of IIC protocol carefully, otherwise you can't master it at all)
one master and multiple slaves application, M16 as the master
(M16 as slave and multiple masters and multiple slaves are not many applications, please refer to relevant documents)
interrupt mode
(because the speed of AVR is very high, and the speed of IIC is relatively low,
the use of query mode will monopolize the CPU for a long time, which will significantly reduce the CPU utilization.
Especially when the speed of IIC is affected by the environment and can only communicate at a low speed, it will have a serious impact on the real-time performance of the system.
The query mode can refer to other documents and software simulation IIC documents)
AT24C02/04/08 operation characteristics
In order to simplify the program, various data are not output to the outside, and it is recommended to use JTAG when learning ICE Hardware Emulator
*/
#include
#include
#include
#include
//The clock is set to an external crystal oscillator 7.3728MHz, F_CPU=7372800
#include
//Defines the status code list for various modes (TWSR has masked the pre-scaling bit), and the Chinese description is attached at the end of this article
//Pin definition
#define pinSCL 0 //PC0 SCL
#define pinSDA 1 //PC1 SDA
//To be on the safe side, it is best to connect an external pull-up resistor of 1~10K to VCC on SCL/SDA.
#define fSCL 100000 //TWI clock is 100KHz
//Pre-scaling factor = 1 (TWPS=0)
#if F_CPU < fSCL*36
#define TWBR_SET 10; //TWBR must be greater than or equal to 10
#else
#define TWBR_SET (F_CPU/fSCL-16)/2; //Calculate TWBR value
#endif
#define TW_ACT (1<
#define SLA_24CXX 0xA0 //24Cxx series manufacturer device address (upper four bits)
#define ADDR_24C02 0x00
// AT24C02 address lines A2/1/0 are all grounded, SLAW=0xA0+0x00<<1+0x00, SLAR=0xA0+0x00<<1+0x01
//TWI_operation status
#define TW_BUSY 0
#define TW_OK 1
#define TW_FAIL 2
//TWI_read and write command status
#define OP_BUSY 0
#define OP_RUN 1
//Common steps for TWI read and write operations
#define ST_FAIL 0 //Error status
#define ST_START 1 //START status check
#define ST_SLAW 2 //SLAW status check
#define ST_WADDR 3 //ADDR status check
//TWI read operation steps
#define ST_RESTART 4 //RESTART status check
#define ST_SLAR 5 //SLAR status check
#define ST_RDATA 6 //Read data status check, loop n bytes
//TWI write operation steps
#define ST_WDATA 7 //Write data status check, loop n bytes
#define FAIL_MAX 20 // Maximum number of retries
//define global variables
unsigned char ORGDATA[8]=
{0xAA,0xA5,0x55,0x5A,0x01,0x02,0x03,0x04}; //original data
unsigned char CMPDATA[8]; //comparison data
unsigned char BUFFER[256]; //buffer, can load the entire AC24C02 data
struct str_TWI //TWI data structure
{
volatile unsigned char STATUS; //TWI_operation statusunsigned
char SLA; //Slave device addressunsigned
int ADDR; //Slave data addressunsigned
char *pBUF; //Data buffer pointerunsigned
int DATALEN; //Data lengthunsigned
char STATE; //TWI read and write operation stepsunsigned
char FAILCNT; //Number of failed retries
};
struct str_TWI strTWI; //TWI data structure variable
// During simulation, monitor these global variables in the watch window.
//AT24C02 read and write functions (including random read, continuous read, byte write, page write)
//Determined by the lowest bit of sla (determined by the interrupt program)
//bit0=1 TW_READ read
//bit0=0 TW_WRITE write
// sla device address (cannot be wrong)
// addr EEPROM address (0~1023)
// *ptr read and write data buffer
// len read data length (1~1024), write data length (1 or 8 or 16)
// Return value whether the current operation can be executed
unsigned char TWI_RW(unsigned char sla,unsigned int addr,unsigned char *ptr,unsigned int len)
{
unsigned char i;
if (strTWI.STATUS==TW_BUSY)
{//TWI is busy, can't perform the operation
return OP_BUSY;
}
strTWI.STATUS=TW_BUSY;
i=(addr>>8)<<1;
i&=0x06; //Considering the high bit of 24C04/08's EEPROM address, place it in SLA
strTWI.SLA=sla+i;
strTWI.ADDR=addr;
strTWI.pBUF=ptr;
strTWI.DATALEN=len
; strTWI.STATE=ST_START;
strTWI.FAILCNT=0;
TWCR=(1<
}
/*
TWI interrupt function
This function flow only considers the IIC device with a byte data (command) address after the device address
(most IIC interface devices are of this type, such as AT24C01/02/04/08/16, DS1307, DS1721, etc.)
For IIC devices with two bytes of data addresses (such as AT24C32/64/128/256 and other large-capacity EEPROMs), please make slight changes
//Determined by the lowest bit of strTWI.SLA
//bit0=1 TW_READ read
//bit0=0 TW_WRITE write
Although the interrupt service program is very long, it only executes one case at a time, so it does not take long.
*/
SIGNAL(SIG_2WIRE_SERIAL)
{//IIC interrupt
unsigned char action,state,status;
action=strTWI.SLA&TW_READ; //Get operation mode
state=strTWI.STATE;
status=TWSR&0xF8; //Shield pre-scaling bit
if ((status>=0x60)||(status==0x00))
{//Bus error or interrupt caused by slave mode, do not handle
return;
}
switch(state)
{
case ST_START: //START status check
if(status==TW_START)
{//Send start signal successfully
TWDR=strTWI.SLA&0xFE; //Send device address and write SLAW
TWCR=TW_ACT; //Trigger the next action and clear the start sending flag at the same time
}
else
{//Error in sending start signal
state=ST_FAIL;
}
break;
case ST_SLAW: //SLAW status check
if(status==TW_MT_SLA_ACK)
{//Send device address successfully
TWDR=strTWI.ADDR; //Send eeprom address
TWCR=TW_ACT; //Trigger the next action
}
else
{//Send device address error
state=ST_FAIL;
}
break;
case ST_WADDR: //ADDR status check
if(status==TW_MT_DATA_ACK)
{//Send eeprom address successfully
if (action==TW_READ)
{//Read operation mode
TWCR=(1<
else
{//Write operation mode
TWDR=*strTWI.pBUF++; //Write the first byte
strTWI.DATALEN--;
state=ST_WDATA-1; //The next step will jump to the WDATA branch
TWCR=TW_ACT; //Trigger the next action
}
}
else
{//Send eeprom address error
state=ST_FAIL;
}
break;
case ST_RESTART: //RESTART status check, only read operation mode can jump hereif
(status==TW_REP_START)
{//Send restart signal successfullyTWDR
=strTWI.SLA; //Send device address and read SLAR
TWCR=TW_ACT; //Trigger the next action and clear the start send flag at the same time
}
else
{//Error in resending start signalstate
=ST_FAIL;
}
break;
case ST_SLAR: //SLAR status check, only read operation mode can jump hereif
(status==TW_MR_SLA_ACK)
{//Send device address successfullyif
(strTWI.DATALEN--)
{//Multiple dataTWCR
=(1<
else
{//There is only one data
TWCR=TW_ACT; //Set NAK to trigger the next action
}
}
else
{//Error in sending device address
state=ST_FAIL;
}
break;
case ST_RDATA: //Read data status check, only read operation mode can jump here
state--; //Loop until the specified length of data is read
if(status==TW_MR_DATA_ACK)
{//Data read successful, but not the last data
*strTWI.pBUF++=TWDR;
if (strTWI.DATALEN--)
{//There are more data
TWCR=(1<
else
{//Prepare to read the last data
TWCR=TW_ACT; //Set NAK to trigger the next action
}
}
else if(status==TW_MR_DATA_NACK)
{//The last data has been read
*strTWI.pBUF++=TWDR;
TWCR=(1<
}
else
{//Error in reading datastate
=ST_FAIL;
}
break;
case ST_WDATA: //Write data status check, only write operation mode can jump herestate--
; //Loop until the specified length of data is writtenif
(status==TW_MT_DATA_ACK)
{//Write data successfullyif
(strTWI.DATALEN)
{//Still need to writeTWDR
=*strTWI.pBUF++;
strTWI.DATALEN--;
TWCR=TW_ACT; //Trigger the next action
}
else
{//Write enoughTWCR
=(1<
//After starting the write command, it takes 10ms (maximum) of programming time to actually record the data
//The device does not respond to any commands during programming
}
}
else
{//Write data failedstate
=ST_FAIL;
}
break;
default:
//error status
state=ST_FAIL;
break;
}
if (state==ST_FAIL)
{//Error handling
strTWI.FAILCNT++;
if (strTWI.FAILCNT
TWCR=(1<
else
{//Otherwise stop
TWCR=(1<
}
}
state++;
strTWI.STATE=state; //Save status
}
int main(void)
{
unsigned char i;
//Power-on default DDRx=0x00, PORTx=0x00 input, no pull-up resistor
PORTA=0xFF; //Unused pins enable internal pull-up resistors.
PORTB=0xFF;
PORTC=0xFF; //SCL, SDA enable internal 10K pull-up resistors
PORTD=0xFF;
//TWI initialization
TWSR=0x00; //Prescaler=0^4=1
TWBR=TWBR_SET;
TWAR=0x00; //Host mode, this address is invalid
TWCR=0x00; //Close TWI module
sei(); //Enable global interrupt
strTWI.STATUS=TW_OK;
TWI_RW(SLA_24CXX+(ADDR_24C02<<1)+TW_WRITE,0x10,&ORGDATA[0],8);
//Write 8 bytes of data starting from address 0x10
while(strTWI.STATUS==TW_BUSY); //Wait for the operation to complete
if (strTWI.STATUS==TW_FAIL)
{
//Operation failed?
}
_delay_ms(10); //Delay to wait for programming to completewhile
(1)
{
i=TWI_RW(SLA_24CXX+(ADDR_24C02<<1)+TW_READ,0x10,&CMPDATA[0],8);
//Read 8 bytes of data starting from address 0x10while
(strTWI.STATUS==TW_BUSY); //Wait for the operation to complete
//If no waiting is added, it is necessary to detect the return value i to know whether the current operation has been executed
// 0 OP_BUSY The previous operation was not completed, and the current operation was not executed
// 1 OP_RUN The current operation is being executedif
(strTWI.STATUS==TW_FAIL)
{
//Operation failed?
}
//Read successfully, compare the data of ORGDATA and CMPDATA
i=TWI_RW(SLA_24CXX+(ADDR_24C02<<1)+TW_READ,0x00,&BUFFER[0],256);
//Read 256 bytes of data (the entire ATC24C02) starting from address 0x00
while(strTWI.STATUS==TW_BUSY); //Wait for the operation to complete
};
}
/*
Two-wire serial interface bus definition
The two-wire interface TWI is very suitable for typical processor applications.
The TWI protocol allows system designers to interconnect 128 different devices using only two bidirectional transmission lines.
The two lines are the clock SCL and the data SDA. The external hardware only needs two pull-up resistors, one on each line.
All devices connected to the bus have their own address.
Note: This means that there cannot be two devices with the same address.
The TWI protocol solves the problem of bus arbitration.
The bus drivers of all TWI compatible devices are open-drain or open-collector. This implements the wired AND function that is critical to interface operation.
When the TWI device outputs "0", the TWI bus will generate a low level.
When all TWI device outputs are tri-stated, the bus will output a high level, allowing the pull-up resistor to pull the voltage higher.
Note: To ensure all bus operations, all AVR devices connected to the TWI bus must be powered on.
The number of devices connected to the bus is limited by the following conditions:
the bus capacitance must be less than 400pF and can be addressed with a 7-bit slave address.
Two different specifications, one for bus speeds below 100 kHz and the other for bus speeds up to 400 kHz.
SCL and SDA pins
SCL and SDA are the TWI interface pins of the MCU.
The output driver of the pin contains a waveform slope limiter to meet the TWI specification.
The input part of the pin includes a spike suppression unit to remove glitches less than 50ns.
When the corresponding port is set to SCL and SDA pins, the 10K pull-up resistor inside the I/O port can be enabled, which can save the external pull-up resistor.
Note: If high-speed communication is required or there are a large number of slaves, it is better to connect a suitable pull-up resistor externally.
Bit rate generator unit
When TWI works in master mode, the bit rate generator controls the period of the clock signal SCL.
It is specifically set by the pre-scaling coefficient of the TWI status register TWSR and the bit rate register TWBR.
When TWI works in slave mode, there is no need to set the bit rate or pre-scaling, but the CPU clock frequency of the slave must be greater than 16 times the frequency of the TWI clock line SCL.
Note that the slave may extend the SCL low level time, thereby reducing the average clock cycle of the TWI bus.
The frequency of SCL is generated according to the following formula:
fSCL=fCPU/((16+2(TWBR)(4^TWPS))
TWBR = the value of the TWI bit rate register
TWPS = the value of the TWI status register prescaler
Note: When TWI works in master mode, the TWBR value should not be less than 10, otherwise the host will generate error outputs on SDA and SCL as prompt signals.
The problem occurs when TWI works in master mode and sends Start + SLA + R/W to the slave (there is no need for a real slave to be connected to the bus).
Control Unit
The control unit monitors the TWI bus and responds accordingly according to the settings of the TWI control register TWCR.
When an event that requires application intervention occurs on the TWI bus, the TWI interrupt flag TWINT is set.
In the next clock cycle, the TWI status register TWSR is updated with the status code word indicating this event.
At other times, the content of TWSR is a special status word indicating that no event has occurred.
Once the TWINT flag is "1", the clock line SCL That is, it is pulled low, suspending data transmission on the TWI bus and allowing the user program to handle events.
When the following conditions occur, the TWINT flag is set:
? After TWI transmits the START/REPEATED START signal
? After TWI transmits the SLA+R/W data
? After TWI transmits the address byte
? After TWI fails to arbitrate the bus
? After TWI is addressed by the host (broadcast mode or slave address match)
? After TWI receives a data byte
? When working as a slave, TWI receives the STOP or REPEATED START signal
? When a bus error occurs due to an illegal START or STOP signal
TWI Register Description
TWI Bit Rate Register - TWBR
? Bits 7..0 - TWI Bit Rate Register
TWBR is the frequency division factor of the bit rate generator.
The bit rate generator is a frequency divider that generates the SCL clock frequency in the master mode. For
the bit rate calculation formula, please refer to the previous [Bit Rate Generator Unit]
TWI Control Register - TWCR
TWCR Used to control TWI operation.
It is used to enable TWI, initiate host access by applying START to the bus, generate receiver response, generate STOP state, and control bus pause when writing data to TWDR register.
This register can also give write conflict information caused by trying to write data to TWDR during the period when TWDR cannot be accessed.
? Bit 7 – TWINT: TWI interrupt flag
TWINT is set when TWI completes the current work and wants the application to intervene.
If the I flag of SREG and the TWIE flag of TWCR register are also set, the MCU executes the TWI interrupt routine.
When TWINT is set, the low level of SCL signal is extended.
The clearing of TWINT flag must be done by software writing "1".
The hardware will not automatically rewrite it to "0" when executing an interrupt.
It should be noted that once this bit is cleared, TWI starts working immediately.
Therefore, before clearing TWINT, you must first complete the access to the address register TWAR, the status register TWSR, and the data register TWDR.
? Bit 6 – TWEA: Enable TWI Acknowledge
The TWEA flag controls the generation of the acknowledge pulse.
If TWEA is set, the interface will send an ACK pulse when the following conditions occur:
1. The slave address of the device matches the address sent by the master
2. A general call is received when TWGCE of TWAR is set
3. A byte of data is received in the master/slave reception mode
Clearing TWEA can temporarily disconnect the device from the bus.
After setting it, the device resumes address recognition.
? Bit 5 – TWSTA: TWI START Status Flag
When the CPU wants to become the master on the bus, it needs to set TWSTA.
The TWI hardware detects whether the bus is available.
If the bus is idle, the interface generates a START state on the bus.
If the bus is busy, the interface waits until a STOP condition is detected and then generates a START to declare that it wishes to become the master.
Software must clear TWSTA after sending a START.
? Bit 4 – TWSTO: TWI STOP Status Flag
In master mode, if TWSTO is set, the TWI interface will generate a STOP condition on the bus and then TWSTO is automatically cleared.
In slave mode, setting TWSTO can restore the interface from an error state to an unaddressed state.
At this time, no STOP condition will be generated on the bus, but TWI returns to a defined unaddressed slave mode and releases SCL and SDA to a high impedance state.
? Bit 3 – TWWC: TWI Write Collision Flag
When TWINT is low, writing to the data register TWDR will set TWWC.
When TWINT is high, each write access to TWDR will update this flag.
? Bit 2 – TWEN: TWI Enable
The TWEN bit is used to enable TWI operation and activate the TWI interface.
When the TWEN bit is written to "1", the TWI pin switches the I/O pin to the SCL and SDA pins, enabling the waveform slope limiter and spike filter.
If this bit is cleared to 0, the TWI interface module will be disabled and all TWI transfers will be terminated.
? Bit 0 – TWIE: Enable TWI interrupt
When the I of SREG and TWIE are set, the TWI interrupt is activated as long as TWINT is "1".
TWI Status Register - TWSR
? Bits 7..3 – TWS: TWI Status
These 5 bits are used to reflect the status of the TWI logic and bus.
The different status codes will be described in the following sections.
Note that the value read from the TWSR includes a 5-bit status value and a 2-bit prescaler value.
The designer should mask the prescaler bit to "0" when detecting the status bit. This makes the status detection independent of the prescaler setting.
? Bits 1..0 – TWPS: TWI prescaler bits
These two bits are readable/writable and are used to control the bit rate prescaler factor.
The prescaler factor is 4 raised to the power
of n. The formula for calculating the bit rate is given in the previous [Bit Rate Generator Unit]
TWI Data Register – TWDR
In transmit mode, TWDR contains the byte to be transmitted;
in receive mode, TWDR contains the received data.
This register is writable when the TWI interface is not shifting (TWINT is set).
The user cannot initialize the data register before the first interrupt occurs.
As long as TWINT is set, the data in TWDR is stable.
As data is shifted out, the data on the bus is simultaneously shifted into the register.
TWDR always contains the last byte present on the bus, unless the MCU is woken up by a TWI interrupt from power-down or power-save mode. In this case, the content of TWDR is undefined.
In case of bus arbitration failure, the master will switch to slave, but the data present on the bus will not be lost.
The processing of ACK is automatically managed by the TWI logic and the CPU cannot directly access ACK.
? Bits 7..0 – TWD: TWI data register,
depending on the state, its content is the next byte to be sent, or the received data.
TWI (slave) address register - TWAR
The upper 7 bits of TWAR are the slave address.
When working in slave mode, TWI will respond according to this address.
This address is not required in master mode.
In a multi-master system, TWAR needs to be set so that other masters can access it.
The LSB of TWAR is used to identify the broadcast address (0x00).
There is an address comparator inside the device. Once the received address matches the local address, the chip requests an interrupt.
? Bits 7..1 – TWA: TWI slave address register,
its value is the slave address.
? Bit 0 – TWGCE: Enable TWI broadcast recognition
When set, the MCU can recognize TWI bus broadcasts.
Using TWI
The TWI interface of AVR is byte-oriented and interrupt-based.
All bus events, such as receiving a byte or sending a START signal, will generate a TWI interrupt.
Since the TWI interface is interrupt-based, the TWI interface does not require application intervention during byte sending and receiving. The
TWI interrupt enable bit [TWIE] and the global interrupt enable bit [I] of the TWCR register together determine whether the application responds to the interrupt request generated by the TWINT flag.
If TWIE is cleared, the application can only detect the TWI bus status by polling the TWINT flag.
When the TWINT flag is "1", it means that the TWI interface has completed the current operation and is waiting for the application's response.
In this case, the TWI status register TWSR contains the value indicating the current TWI bus status.
The application can read the status code of TWCR to determine whether the current status is correct, and determine how the TWI interface should work in the next TWI bus cycle by setting the TWCR and TWDR registers.
List of status codes in various modes (TWSR has masked the pre-scaling bit)
There are definitions in twi.h, and the Chinese description is attached
Host sends status code
#define TW_START 0x08 //START has been sent
#define TW_REP_START 0x10 //Repeat START has been sent
#define TW_MT_SLA_ACK 0x18 //SLA+W has been sent and received ACK
#define TW_MT_SLA_NACK 0x20 //SLA+W has been sent and received NOT ACK
#define TW_MT_DATA_ACK 0x28 //Data has been sent and received ACK
#define TW_MT_DATA_NACK 0x30 //Data has been sent and received NOT ACK
#define TW_MT_ARB_LOST 0x38 //SLA+W or data arbitration failed
Host receives status code
//#define TW_START 0x08 //START has been sent
//#define TW_REP_START 0x10 //Repeated START has been sent
#define TW_MR_ARB_LOST 0x38 //Arbitration failure of SLA+R or NOT ACK
#define TW_MR_SLA_ACK 0x40 //SLA+R has been sent and ACK has been received
#define TW_MR_SLA_NACK 0x48 //SLA+R has been sent and NOT ACK
has been received #define TW_MR_DATA_ACK 0x50 //Data received ACK has been returned
#define TW_MR_DATA_NACK 0x58 //Data received NOT ACK has been returned
Slave receiving status code
#define TW_SR_SLA_ACK 0x60 //Own SLA+W has been received ACK has been returned
#define TW_SR_ARB_LOST_SLA_ACK 0x68 //SLA+R/W arbitration as the host has failed; own SLA+W has been received ACK has been returned
#define TW_SR_GCALL_ACK 0x70 //Received broadcast address ACK has been returned
#define TW_SR_ARB_LOST_GCALL_ACK 0x78 //SLA+R/W arbitration as the host failed; received broadcast address ACK has been returned
#define TW_SR_DATA_ACK 0x80 //Previously addressed with its own SLA+W; data has been received ACK has been returned
#define TW_SR_DATA_NACK 0x88 //Previously addressed with its own SLA+W; data has been received NOT ACK has been returned
#define TW_SR_GCALL_DATA_ACK 0x90 //Previously addressed in broadcast mode; data has been received ACK has been returned
#define TW_SR_GCALL_DATA_NACK 0x98 //Previously addressed in broadcast mode; data has been received NOT ACK has been returned #define TW_SR_STOP 0xA0 //
Send status code
when receiving STOP or repeated START while working as a slave
#define TW_ST_SLA_ACK 0xA8 //Own SLA+R has been received and ACK has been returned
#define TW_ST_ARB_LOST_SLA_ACK 0xB0 //SLA+R/W as the host arbitration failed; own SLA+R has been received and ACK has been returned
#define TW_ST_DATA_ACK 0xB8 //Data in TWDR has been sent and ACK has been received
#define TW_ST_DATA_NACK 0xC0 //Data in TWDR has been sent and NOT ACK has been received #define TW_ST_LAST_DATA 0xC8 //One byte of data in TWDR has been sent (TWAE = "0"); ACK
other status codes
have been received
#define TW_NO_INFO 0xF8 //No relevant status information; TWINT = "0"
#define TW_BUS_ERROR 0x00 //Bus error caused by illegal START or STOP
AT24C02/04/08 Characteristics of IIC interface EEPROM
(the characteristics of 24 series EEPROMs from different companies are partially different, please refer to the data sheet)
1 AT24C02/04/08 is a 2K/4K/8K-bit serial CMOS E2PROM containing 256/512/1024 8-bit bytes inside
2 AT24C02 has an 8-byte page write buffer, AT24C04/08/16 has a 16-byte page write buffer
3 Through the device address input terminals A0, A1, and A2, up to
8 24C02 devices,
4 24C04 devices
, and 2 24C08 devices can be
connected to the bus at the same time
4 Write operation
1 byte write
2 page write AT24C02 is 8 bytes/page AT24C04/08 is 16 bytes/page
Note: The address of the page write is automatically accumulated only in the current page and circulates within the page address range.
After starting the write command, it takes 10ms (maximum) programming time to actually record the data. During programming, the device does not respond to any command.
5 Read operation
1 Immediate address read The address is automatically accumulated, that is, the address of the last read/write operation + 1 (this program does not support this operation)
2 Random read Read a byte at the specified address
3 Continuous read The continuous read operation can be started by immediate read or random read operation, and the host sends NAK and STOP to stop the read operation.
During the read operation, the address counter increases within the entire address of AT24C02/04/08, so that the entire register area can be read out in one read operation
. After reading the last address, continue to read from the first address.
6 Write protection function, controlled by the WP pin. When WP=VCC, the upper 1K bits of 24C02, the upper 2K bits of 24C04, and all 8K bits of 24C08 become read-only and cannot be written.
Previous article:ATmega8 clock selection fuse settings
Next article:AVR microcontroller work experience summary
Recommended ReadingLatest update time:2024-11-16 14:29
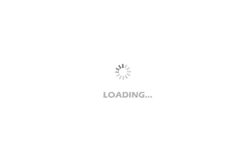
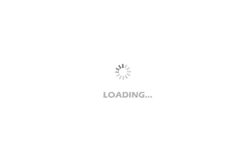
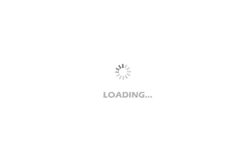
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Metronom Real-Time Operating System RTOS for AVR microcontrollers
-
Learn C language for AVR microcontrollers easily (with video tutorial) (Yan Yu, Li Jia, Qin Wenhai)
-
ATmega16 MCU C language programming classic example (Chen Zhongping)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- EEWORLD University Hall----Design and optimization of buck-boost charging chip compatible with USB-PD protocol
- Self-wound coil inductance calculation assistant
- Showing goods + Qualcomm original QCA4020 development board
- How to enable TI 15.4-Stack to support 470M frequency band
- EEWORLD University - SimpleLink Wi-Fi CC3120 Project Introduction
- OC5501-OC5502-LED automotive high and low beam solutions (no diode design, applied to automotive lights, MR16, architectural lighting)
- I would like to ask you guys, do I need to draw PCB in electronic competitions?
- stm32f407 driver enc28j60
- LM3478 step-down problem
- Does anyone understand the PD power supply protocol? USB Type-C PD power supply problem!