SYNOPSIS Overview
#include
#include
int tcgetattr(int
int tcsetattr(int fd, int optional_actions, struct termios *termios_p);
int tcsendbreak(int fd, int duration);
int tcdrain(int fd);
int tcflush(int fd, int queue_selector);
int tcflow(int fd, int act
int cfmakeraw(struct termios *termios_p);
speed_t cfgetispeed(struct termios *termios_p);
speed_t cfgetospeed(struct termios *termios_p);
int cfsetispeed(struct termios *termios_p, speed_t speed);
int cfsetospeed(struct termios *termios_p, speed_t speed);
DESCRIPTION
The termios family of functions provides a general terminal interface for controlling asynchronous communications ports.
Most of the properties described here have an argument of type termios_p, which is a pointer to a termios structure. This structure contains at least the following members:
tcflag_t c_iflag; /* input mode*/
tcflag_t c_oflag; /* output mode*/
tcflag_t c_cflag; /* control mode*/
tcflag_t c_lflag; /* local mode*/
cc_t c_cc[NCCS]; /* control character*/
c_iflag Flag constant:
IGNBRK
Ignore BREAK status on input.
BRKINT
If IGNBRK is set, BREAK is ignored. If not set, but BRKINT is set, then BREAK causes the input and output queues to be flushed, and if the terminal is the controlling terminal of a foreground process group, all processes in that process group will receive a SIGINT signal. If neither IGNBRK nor BRKINT is set, BREAK is treated as a synonym for the NUL character, unless PARMRK is set, in which case it is treated as the sequence \377 \0 \0.
IGNPAR
Ignore frame and parity errors.
PARMRK
If IGNPAR is not set, insert \377 \0 before characters with parity or frame errors. If neither IGNPAR nor PARMRK is set, treat characters with parity or frame errors as \0.
INPCK
Enable input parity checking.
ISTRIP
Strip the eighth bit.
INLCR
Translate NL on input into CR.
IGNCR
Ignore carriage returns on input.
ICRNL
Translate carriage returns on input into new lines (unless IGNCR is set).
IUCLC
(not in POSIX) Map uppercase letters on input to lowercase.
IXON
Enable XON/XOFF flow control on output.
IXANY
(not in POSIX.1; XSI) Allow any character to restart output. (?)
IXOFF
Enable XON/XOFF flow control on input.
IMAXBEL
(not in POSIX) Zero when the input queue is full. Linux does not implement this bit and always treats it as set.
c_oflag flag constants defined in POSIX.1:
OPOST
enables implementation-defined output processing.
The remaining c_oflag flag constants are as defined in POSIX 1003.1-2001 unless otherwise noted.
OLCUC
(not in POSIX) Map lowercase letters to uppercase letters on output.
ON
(XSI) Map newline characters to carriage return-linefeed on output.
OCRNL
Map carriage returns to newline characters on output .
ON
does not output carriage returns in column 0.
ON
does not output carriage returns.
OFILL
sends fill characters as delays instead of using timers to delay.
OFDEL
(not in POSIX) The fill character is ASCII DEL (0177). If not set, the fill character is ASCII NUL.
NLDLY
Newline delay mask. Valid values are NL0 and NL1.
CRDLY
Carriage return delay mask. Valid values are CR0, CR1, CR2, or CR3.
TABDLY
Horizontal tab delay mask. Valid values are TAB0, TAB1, TAB2, TAB3 (or XTABS). Valid values are TAB3, which is XTABS, which expands tabs to spaces (8 spaces per tab). (?)
BSDLY
fallback delay mask. Value is BS0 or BS1. (Never implemented)
VTDLY
vertical tab delay mask. Value is VT0 or VT1.
FFDLY
table entry delay mask. Value is FF0 or FF1.
c_cflag flag constants:
CBAUD
(not in POSIX) Baud rate mask (4+1 bits).
CBAUDEX
(not in POSIX) Extended baud rate mask (1 bit), included in CBAUD.
(POSIX specifies that the baud rate is stored in the termios structure, without specifying its exact location, but providing the functions cfgetispeed() and cfsetispeed() to access it. Some systems use the bits selected by CBAUD in c_cflag, others use separate variables such as sg_ispeed and sg_ospeed.)
CSIZE
Character length mask. Can be CS5, CS6, CS7, or CS8.
CSTOPB
Set two stop bits instead of one.
CREAD
Open the receiver.
PARENB
Enable parity information for output and even/odd parity for input.
PARODD
Input and output are odd parity.
HUPCL
Lower the modem control line (hangup) after the last process closes the device. (?)
CLOCAL
Ignore the modem control line.
LOBLK
(not in POSIX) Block output from non-current shells (for shl). (?)
CIBAUD
(not in POSIX) Mask for input speed. CIBAUD bits have the same values as CBAUD bits, shifted left by IBSHIFT bits.
CRTSCTS
(not in POSIX) Enable RTS/CTS (hardware) flow control.
c_lflag Flag constants:
ISIG
Generates the corresponding signal when the characters INTR, QUIT, SUSP, or DSUSP are received.
ICANON
Enables canonical mode. The special characters EOF, EOL, EOL2, ERASE, KILL, LNEXT, REPRINT, STATUS, and WERASE are allowed, as well as line buffering.
XCASE
(not part of POSIX; not supported under Linux) If ICANON is also set, the terminal is uppercase only. Input is converted to lowercase, except for characters prefixed with \. On output, uppercase characters are prefixed with \, and lowercase characters are converted to uppercase.
ECHO
Echoes the input characters.
ECHOE
If ICANON is also set, the characters ERASE erase the previous input character and WERASE erase the previous word.
ECHOK
If ICANON is also set, the characters KILL delete the current line.
ECHONL
If ICANON is also set, the character NL is echoed, even if ECHO is not set.
ECHOCTL
(not in POSIX) If ECHO is also set, ASCII control signals except TAB, NL, START, and STOP are echoed as ^X, where X is the ASCII code 0x40 greater than the control signal. For example, the character 0x08 (BS) is echoed as ^H.
ECHOPRT
(not in POSIX) If ICANON and IECHO are both set, characters are printed as they are deleted.
ECHOKE
(not in POSIX) If ICANON is also set, echoing KILL deletes every character on a line, as if ECHOE and ECHOPRT were specified.
DEFECHO
(not in POSIX) Echo only when a process is reading.
FLUSHO
(not in POSIX; not supported under Linux) Output is flushed. This flag can be toggled on and off by typing the characters DISCARD.
NOFLSH
disables flushing of the input and output queues on SIGINT, SIGQUIT, and SIGSUSP.
TOSTOP
sends SIGTTOU to the background process group that attempts to write to the controlling terminal.
PENDIN
(not part of POSIX; not supported under Linux) All characters in the input queue are re-output when the next character is read. (bash uses this to handle typeahead)
IEXTEN
enables implementation-defined input processing. This flag must be used with ICANON to interpret the special characters EOL2, LNEXT, REPRINT and WERASE, and the IUCLC flag is valid.
The c_cc array defines special control characters. The symbol subscripts (initial values) and meanings are:
VINTR
(003, ETX, Ctrl-C, or also 0177, DEL, rubout) Interrupt character. Sends SIGINT signal. Recognized when ISIG is set and is not passed as input.
VQUIT
(034, FS, Ctrl-\) Quit character. Sends SIGQUIT signal. Recognized when ISIG is set and is not passed as input.
VERASE
(0177, DEL, rubout, or 010, BS, Ctrl-H, or also #) Delete character. Deletes the last character that has not been deleted, but does not delete the last EOF or beginning of the line. Recognized when ICANON is set and is not passed as input.
VKILL
(025, NAK, Ctrl-U, or Ctrl-X, or also @) Terminate character. Deletes input since the last EOF or beginning of the line. Recognized when ICANON is set and is not passed as input.
VEOF
(004, EOT, Ctrl-D) End-of-file character. More precisely, this character causes the contents of the tty buffer to be sent to a user program waiting for input without waiting for EOL. If it is the first character of a line, a user program's read() will return 0, indicating that EOF was read. Recognized when ICANON is set, and not passed as input.
VMIN
Minimum number of characters to read in non-canonical mode.
VEOL
(0, NUL) Additional end-of-line character. Recognized when ICANON is set.
VTIME
Delay in non-canonical mode reading, in tenths of a second.
VEOL2
(not in POSIX; 0, NUL) Another end-of-line character. Recognized when ICANON is set.
VSWTCH
(not in POSIX; not supported under Linux; 0, NUL) Switch character. (Used only by shl.)
VSTART
(021, DC1, Ctrl-Q) Start character. Restarts output interrupted by the Stop character. Recognized when IXON is set, and no longer passed as input.
VSTOP
(023, DC3, Ctrl-S) Stop character. Stops output until the Start character is typed. Recognized when IXON is set, and no longer passed as input.
VSUSP
(032, SUB, Ctrl-Z) Suspend character. Sends SIGTSTP. Recognized when ISIG is set, and no longer passed as input.
VDSUSP
(not in POSIX; not supported under Linux; 031, EM, Ctrl-Y) Delayed suspend signal. When the user program reads this character, SIGTSTP is sent. Recognized when IEXTEN and ISIG are set and the system supports job management, and no longer passed as input.
VLNEXT
(not in POSIX; 026, SYN, Ctrl-V) Literal next. Quotes the next input character, canceling any special meaning for it. Recognized when IEXTEN is set, and no longer passed as input.
VWERASE
(not in POSIX; 027, ETB, Ctrl-W) Delete words. Recognized when ICANON and IEXTEN are set, and not passed as input.
VREPRINT
(not in POSIX; 022, DC2, Ctrl-R) Re-output unread characters. Recognized when ICANON and IEXTEN are set, and not passed as input.
VDISCARD
(not in POSIX; not supported under Linux; 017, SI, Ctrl-O) Switch: start/end discarding unfinished output. Recognized when IEXTEN is set, and not passed as input.
VSTATUS
(not in POSIX; not supported under Linux; status request: 024, DC4, Ctrl-T).
These symbolic subscript values are mutually exclusive, except that VTIME and VMIN may have the same value as VEOL and VEOF, respectively. (In non-canonical mode, the meaning of special characters is changed to delayed meanings. MIN is the minimum number of characters that should be read. TIME is a timer in tenths of a second. If both are set, read will wait until at least one character has been read, returning as soon as MIN characters have been read or TIME has elapsed since the last character was read. If only MIN is set, read will not return until MIN characters have been read. If only TIME is set, read will return as soon as at least one character has been read or the timer expires. If neither is set, read will return immediately with only the characters currently ready.) (?)
tcgetattr() gets the parameters associated with the object pointed to by fd, storing them in the termios structure referenced by termios_p. The function may be called from a background process; however, the terminal attributes may be changed by a subsequent foreground process.
tcsetattr() sets terminal-specific parameters (unless underlying support is required and not available), using the termios structure referenced by termios_p. optional_actions specifies when the changes take effect:
TCSANOW
changes take effect immediately
TCSADRAIN
changes take effect after all output written to fd has been transmitted. This function should be used to modify parameters that affect output.
TCSAFLUSH
changes take effect after all output written to the object referenced by fd has been transmitted, and any input accepted but not read is discarded before the change takes place.
tcsendbreak() transmits a continuous stream of zero-valued bits, lasting for a certain period of time, if the terminal uses asynchronous serial data transmission. If duration is 0, it is transmitted for at least 0.25 seconds and no more than 0.5 seconds. If duration is non-zero, it is sent for an implementation-defined length of time.
If the terminal does not use asynchronous serial data transmission, tcsendbreak() does nothing.
tcdrain() waits until all output written to the object referenced by fd has been transmitted.
tcflush() discards data that was to be written to the referenced object but not yet transmitted, or data that was received but not yet read, depending on the value of queue_selector:
TCIFLUSH
flushes received data but not reads it.
TCOFLUSH
flushes written data but not transmits it.
TCIOFLUSH
simultaneously flushes received data but not reads it, and flushes written data but not transmits it.
tcflow() suspends data transmission or reception on the object referenced by fd, depending on
TCOOFF
suspends output.
TCOON
restarts suspended output.
TCIOFF
sends a STOP character to stop the terminal device from transmitting data to the system.
TCION
sends a START character to enable the terminal device to transmit data to the system.
The default setting when opening a terminal device is that neither input nor output is suspended.
The baud rate functions are used to get and set the input and output baud rate values in the termios structure. The new values will not take effect immediately until the tcsetattr() function is successfully called.
Setting the speed to B0 puts the modem "on-hook". The actual bitrate corresponding to B38400 can be adjusted with setserial(8).
The input and output baud rates are stored in the termios structure.
cfmakeraw sets the terminal properties as follows:
termios_p->c_iflag &= ~(IGNBRK|BRKINT|PARMRK|ISTRIP
|INLCR|IGNCR|ICRNL|IXON);
termios_p->c_oflag &= ~OPOST;
termios_p->c_lflag &= ~(ECHO|ECHONL|ICANON|ISIG|IEXTEN);
termios_p->c_cflag &= ~(CSIZE|PARENB);
termios_p->c_cflag |= CS8;
cfgetospeed() Returns the output baud rate stored in the termios structure pointed to by termios_p.
cfsetospeed() sets the output baud rate stored in the termios structure pointed to by termios_p to speed. The value must be one of the following constants:
B0
B50
B75
B110
B134
B150
B200
B300
B600
B1200
B1800
B2400
B4800
B9600 B19200
B38400
B57600
B115200
B230400
The
value B0 is used to terminate the connection. If B0 is specified, no connection should be assumed. Normally, this will terminate the connection. CBAUDEX is a mask indicating speeds higher than those defined by POSIX.1 (57600 and above). Thus, B57600 & CBAUDEX is non-zero.
cfgetispeed() Returns the input baud rate stored in the termios structure.
cfsetispeed() sets the input baud rate stored in the termios structure to speed. If the input baud rate is set to 0, the actual input baud rate will be equal to the output baud rate.
RETURN VALUE
cfgetispeed() returns the input baud rate stored in the termios structure.
cfgetospeed() returns the output baud rate stored in the termios structure.
Other functions return:
0
on success
- 1
on failure, and errno is set to indicate the error.
Note that tcsetattr() returns success if any of the requested modifications can be made. Therefore, when making multiple modifications, tcgetattr() should be called again after this function to check whether all modifications were successfully made.
NOTES Note that
Unix V7 and many later systems have a list of baud rates, with the two constants EXTA, EXTB ("External A" and "External B") after the fourteen values B0, ..., B9600. Many systems extend this list for higher baud rates. A
nonzero duration in tcsendbreak has different effects. SunOS specifies a break of duration*N seconds, where N is at least 0.25 and not more than 0.5. Linux, AIX, DU, Tru64 send a break of duration microseconds. FreeBSD, NetBSD, HP-UX and MacOS ignore the value of duration. On Solaris and Unixware, tcsendbreak with a nonzero duration has the same effect as tcdrain.
SEE ALSO
stty(1), setserial(8)
Previous article:Arm instruction set addressing mode
Next article:ARM Interrupt Registers
Recommended ReadingLatest update time:2024-11-16 17:39
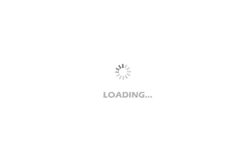
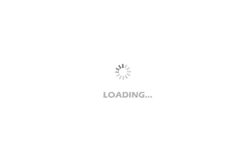
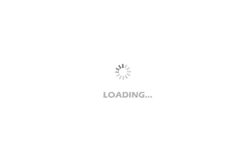
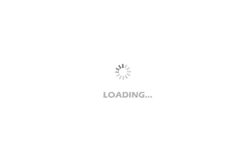
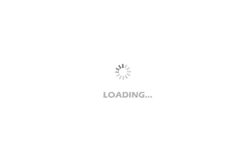
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Discussion: Why is the promotion and popularization of 5G not as fast as 4G and 3G?
- Flash - How to modify an application from RAM configuration to Flash configuration?
- BearPi-HM Nano 3 + Analysis of source code writing process under vscode
- STC15W104 power-off wake-up can not be done, please help
- Shift register based frequency divider
- DSP C6000 Architecture
- Application of millimeter wave radar technology in barriers
- (Bonus 3) GD32L233 Review - Unboxing and showing off the big box
- CPLD
- Help, after the load power supply has been working for a period of time, the microcontroller will shut down automatically