Today, when I was writing an ARM assembly code, I wanted to display string information in the program, but it didn't work. I thought it was because of the implementation of printf in the C library. I finally solved the problem in the afternoon. It turned out that the C library was not initialized in the assembly program.
When I encountered this problem, for the sake of simplicity, I directly used assembly to write a program that called the printf function to display the string hello, as follows:
area |hello$code|,code,readonly
code32
entry
import _printf
import ||LibRequest
armlib||,weak
hello
adr r0,strhello
bl _printf
strhello
dcb "hello!/n/0"
end
As a result, during debugging, although the _printf function can be called, the string cannot be output in the console. But later I rewrote this section of assembly code into a procedure (removed entry, added registers and lr protection at the entry, and restored the protected registers before returning), and then added a C original program (main), called this procedure in main, and found that the string can be output in the console.
So I checked some information (Google) and finally found the problem. It turned out that the C library was not initialized in the assembly code. So I changed the assembly code to the following:
area |.text|,code,readonly
code32
export main; Export main, main function,
import _printf
import __main; C Library Entry
import ||LibRequest
armlib||,weak
main
stmfd sp!,{lr}
adr r0,strhello
bl _printf
ldmfd sp!,{pc}
strhello
dcb "hello!/n/0"
end
The debugging is successful, and the string information can be successfully output to the console.
This program has a main function main, so the main symbol needs to be exported. In addition, the label __main (import __main) needs to be introduced to represent the beginning of C library initialization; because the _printf function is called in the program and the ARM C library is used, it is necessary to import |Lib$Request$armlib|. The weak at the end indicates that if the label of this line cannot be found, no connection error will be reported.
The meaning of |.text|: It indicates the code segment generated by the C compiler, or the code segment used to be associated with the C library in some way. Here it refers to the latter.
References:
1. ARM assembly optimization, http://blog.csdn.net/guanchanghui/archive/2007/06/27/1669513.aspx
2. RealView Compiler Tool 2.0 Assembler Guide, http://infocenter.arm.com/help/index.jsp
Keywords:arm
Reference address:Calling C library function printf in arm assembly language
When I encountered this problem, for the sake of simplicity, I directly used assembly to write a program that called the printf function to display the string hello, as follows:
area |hello$code|,code,readonly
code32
entry
import _printf
import ||Lib
hello
adr r0,strhello
bl _printf
strhello
dcb "hello!/n/0"
end
As a result, during debugging, although the _printf function can be called, the string cannot be output in the console. But later I rewrote this section of assembly code into a procedure (removed entry, added registers and lr protection at the entry, and restored the protected registers before returning), and then added a C original program (main), called this procedure in main, and found that the string can be output in the console.
So I checked some information (Google) and finally found the problem. It turned out that the C library was not initialized in the assembly code. So I changed the assembly code to the following:
area |.text|,code,readonly
code32
export main; Export main, main function,
import _printf
import __main; C Library Entry
import ||Lib
main
stmfd sp!,{lr}
adr r0,strhello
bl _printf
ldmfd sp!,{pc}
strhello
dcb "hello!/n/0"
end
The debugging is successful, and the string information can be successfully output to the console.
This program has a main function main, so the main symbol needs to be exported. In addition, the label __main (import __main) needs to be introduced to represent the beginning of C library initialization; because the _printf function is called in the program and the ARM C library is used, it is necessary to import |Lib$Request$armlib|. The weak at the end indicates that if the label of this line cannot be found, no connection error will be reported.
The meaning of |.text|: It indicates the code segment generated by the C compiler, or the code segment used to be associated with the C library in some way. Here it refers to the latter.
References:
1. ARM assembly optimization, http://blog.csdn.net/guanchanghui/archive/2007/06/27/1669513.aspx
2. RealView Compiler Tool 2.0 Assembler Guide, http://infocenter.arm.com/help/index.jsp
Previous article:arm-linux connection and connection script
Next article:ARM Procedure Call Standard
Recommended ReadingLatest update time:2024-11-16 07:56
[JZ2440] Install arm-linux-gcc cross-compiler
1. Acquisition of relevant information Personally, when I was learning the mini2440 development board, I followed teacher Wei Dongshan’s videos. The videos and information can be obtained from the Baiwen.com forum. Baiwen.com forum link: http://www.100ask.net/bbs/forum.php After downloading the information
[Microcontroller]
Intel's foundry business and Arm announce a collaboration involving multi-generation cutting-edge system chip design
This cooperation will bring chip designers a powerful combination of Arm cores and Intel's Ami-era process technology. Santa Clara, California and Cambridge, UK, April 12, 2023 – Intel Foundry Services (IFS) and Arm today announced the signing of a multi-generation cutting-edge system-on-chip design. The agreement a
[Embedded]
Getting the ARM instruction set right (Part 1) --- Data storage and loading instructions
ARM instructions belong to Reduced Scalability Computer Instructions (RISC), which are numerous and difficult to remember. However, the development of things is continuous, especially natural science, which is a kind of accumulation. Therefore, their development must be based on certain rules! After mastering and bein
[Microcontroller]
GigaDevice and Arm Technology deepen technical cooperation to jointly create opportunities for Arm MCU innovation
Beijing, China (August 15, 2024) -
GigaDevice Technology Group Co., Ltd. (hereinafter referred to as "GigaDevice"), an industry-leading semiconductor device supplier, and Arm Technology (China) Co., Ltd. (hereinafter referred to as "Arm Technology") jointly announced that the two parties will further strengthen
[Embedded]
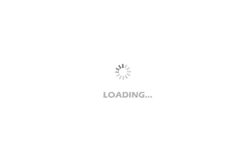
PC in ARM and PC in AXD
R15 (PC) always points to the instruction being "fetched", not the instruction being "executed" or "decoded". Generally speaking, people usually agree to use the "instruction being executed as the reference point", which is called the first instruction at the moment, so PC always points to the third instruction. W
[Microcontroller]
ARM chip development (S5PV210 chip) - timer, watchdog, RTC
1. Counter The counter increases its count value by one at fixed intervals, so we can calculate the time based on the count value: elapsed time = count value x counting time interval. 2. Timer 2.1、Timer Introduction The timer has a timing function, similar to the countdown function of our mobile phone. For example,
[Microcontroller]
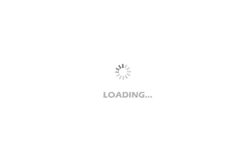
Japanese chip manufacturer Socionext announced that it will join forces with TSMC to develop 2nm ARM processors
According to news on October 27, Socionext is the only listed company in Japan responsible for customizing SoC chips. This company claims to "only develop and produce chips after receiving orders." Its operating model is essentially different from Nvidia and AMD. However, the company has now announced that it is teami
[Semiconductor design/manufacturing]
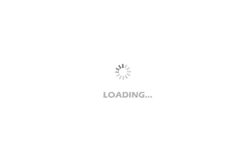
ARM AVI video playback based on 2440 freambuffer drawing
AVI file format diagram
tidy:
AVI is a container that can hold data in any format.
Video encoding: The format can be H264, MPEG-4, MJPEG. Since MJPEG is relatively simple, only MJPEG avi videos are supported.
Audio encoding: PCM16, mp3 Because PCM encoding, the file is too large, so onl
[Microcontroller]
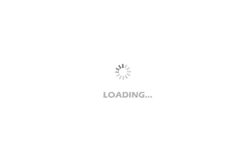
Recommended Content
Latest Microcontroller Articles
He Limin Column
Microcontroller and Embedded Systems Bible
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
MoreSelected Circuit Diagrams
MorePopular Articles
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
MoreDaily News
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
Guess you like
- LIS2HH12 MEMS Digital Output Motion Sensor Datasheet
- Three major misunderstandings about Bluetooth transmission
- The problem of not being able to sample the AD value using the official ADC routine based on the BlueNRG-LP chip
- [RVB2601 Creative Application Development] Environmental Monitoring Terminal 06-Synchronize Data to Alibaba Cloud
- One question per week, relax and broaden your mind
- Crowdfunding 3,000 yuan to buy batteries for needle penetration tests, BYD VS CATL technology game
- [Evaluation of EC-01F-Kit, the NB-IoT development board of Anxinke] + Alibaba Cloud connection to upload light illumination data
- Operate the lmx2582 registers through stm32, the project file is in the attachment
- 【ESP32-S2-Kaluga-1 Review】2. Update the firmware
- Speed sensor