**** AVR Analog Comparator Usage Example ***
**** Compiler: WINAVR20050214 ***
***********************************************/
/*
This program simply demonstrates how to use the analog comparator of ATMEGA16
Analog Comparator Settings
Interrupt mode --more commonly used
The query method is not commonly used, just detect ACO. It can be used as a DELTA-SIGMA A/D CONVERTER. After calibration, the accuracy is relatively high, but the speed is low. The speed can be slightly improved by using assembly.
In order to simplify the program, various data are not output externally. It is recommended to use the JTAG ICE hardware emulator when learning.
*/
#include
#include
#include
#include
/*
The usage of the macro INTERRUPT is similar to that of SIGNAL, except that:
When SIGNAL is executed, the global interrupt trigger bit is cleared and other interrupts are disabled.
When INTERRUPT is executed, the global interrupt trigger bit is set and other interrupts can be nested.
In addition, avr-libc provides two API functions for setting and clearing the global interrupt trigger bit, which are frequently used.
They are: void sei(void) and void cli(void) defined by interrupt.h
Note: The internal function _delay_ms() has a maximum delay of 262.144mS@1MHz
This function can achieve more accurate timing, but it is more troublesome when using JTAG simulation - it will enter the machine code window (Disassembeler). Be sure to jump out of this paragraph.
Once the JTAG simulation enters the internal function statement, it will become like a "freeze" (actually it is running). You can first [break], then set [breakpoint] in the following C statement, and [RUN] to skip
It is troublesome to calculate the delay time using for()/while() statements.
In order to make the delay of the _delay_ms() function correct, you must set F_CPU to the actual system clock frequency in the makefile.
This example uses a 1MHz internal RC oscillator, i.e. F_CPU = 1000000
*/
//Pin definition
#define LED0 0 //PB0
#define AIN_P 2 //PB2(AIN0)
#define AIN_N 3 //PB3(AIN1)
//Macro definition
#define LED0_ON() PORTB|= (1< //output high level, the light is on
#define LED0_OFF() PORTB&=~(1< //output low level, light off
//Constant definition
/*
The positive input of the analog comparator is determined by the ACBG bit. =0 selects the AIN0 pin, =1 selects the 1.23V internal bandgap reference source
Analog comparator multiplexing inputs (not commonly used as the ADC will not be able to be used)
Any one of ADC7..0 can be selected to replace the negative input of the analog comparator.
An ADC multiplexer can be used to accomplish this function.
Of course, in order to use this function, the ADC must first be turned off.
If the analog comparator multiplexer enable bit (ACME in SFIOR) is set and the ADC is turned off (ADEN in the ADCSRA register is 0), the pin that replaces the negative input of the analog comparator can be selected through MUX2..0 in the ADMUX register. If ACME is cleared or ADEN is set, the negative input of the analog comparator is AIN1.
*/
#define AC_ADC0 0x00 //ADC0
#define AC_ADC1 0x01 //ADC1
#define AC_ADC2 0x02 //ADC2
#define AC_ADC3 0x03 //ADC3
#define AC_ADC4 0x04 //ADC4
#define AC_ADC5 0x05 //ADC5
#define AC_ADC6 0x06 //ADC6
#define AC_ADC7 0x07 //ADC7
SIGNAL(SIG_COMPARATOR) //Analog comparator interrupt service routine
{
//Hardware automatically clears the ACI flag
_delay_us(10);
if ((ACSR&(1< //Detect ACO
LED0_ON(); //If AIN0 is on
else
LED0_OFF(); //Otherwise the LED turns off
_delay_ms(200); //When the voltage difference is close to 0V, the analog comparator will produce critical jitter, so the delay is 200ms so that the naked eye can see it.
}
int main(void)
{
//Power-on default DDRx=0x00, PORTx=0x00 input, no pull-up resistor
PORTA=0xFF;
PORTC=0xFF; // Enable internal pull-up resistors for unused pins.
PORTD=0xFF;
PORTB=~((1< //When used as analog comparator input, the internal pull-up resistor cannot be enabled.
DDRB= (1< //PB0 is output
/*
Analog Comparator Control and Status Register - ACSR
Bit 7 – ACD: Analog Comparator Disable
The analog comparator is already working by default when it is powered on, which is different from other modules.
When ACD is set, the power supply of the analog comparator is cut off. This bit can be set at any time to turn off the analog comparator.
This reduces power consumption in both active and idle modes of the device.
When changing the ACD bit, the ACIE bit in the ACSR register must be cleared to disable the analog comparator interrupt. Otherwise, an interrupt may be generated when the ACD changes.
Bit 6 – ACBG: Selects the bandgap reference source for the analog comparator
When ACBG is set, the positive input of the analog comparator is replaced by the 1.23V bandgap reference. Otherwise, AIN0 is connected to the positive input of the analog comparator.
Bit 5 – ACO: Analog Comparator Output
The analog comparator output is directly connected to ACO after synchronization. The synchronization mechanism introduces a delay of 1-2 clock cycles.
Bit 4 – ACI: Analog Comparator Interrupt Flag
ACI is set when the comparator output event triggers the interrupt mode defined by ACIS1 and ACIS0.
If the ACIE and SREG register global interrupt flags I are also set, the analog comparator interrupt service routine is executed and ACI is cleared by hardware.
ACI can also be cleared by writing "1".
Bit 3 – ACIE: Analog Comparator Interrupt Enable
When the ACIE bit is set to "1" and the global interrupt flag I in the status register is also set, the analog comparator interrupt is activated .
Otherwise the interrupt is disabled.
Bit2 – ACIC: Analog Comparator Input Capture Enable
This function is used to detect some weak trigger signal sources and save an external op amp.
When ACIC is set, it allows the analog comparator to trigger the input capture function of Timer/C1.
At this time, the output of the comparator is directly connected to the front-end logic of the input capture, so that the comparator can take advantage of the noise suppressor and trigger edge selection functions of the T/C1 input capture interrupt logic.
In order for the comparator to trigger the input capture interrupt of T/C1, the TICIE1 bit in the timer interrupt mask register TIMSK must be set.
When ACIC is "0", there is no connection between the analog comparator and the input capture function.
Bits 1, 0 – ACIS1, ACIS0: Analog comparator interrupt mode selection
These two bits determine the event that triggers the analog comparator interrupt.
ACIS1 ACIS0 Interrupt Mode
0 0 Comparator output changes can trigger an interrupt
0 1 Reserved
1 0 The falling edge of the comparator output generates an interrupt
1 1 A rising edge on the comparator output generates an interrupt
When changing ACIS1/ACIS0, the interrupt enable bit in the ACSR register must be cleared to disable the analog comparator interrupt. Otherwise, an interrupt may be generated when changing these two bits.
*/
ACSR=(1<
// Enable the analog comparator interrupt. The change of the comparator output can trigger the interrupt. AIN0 is the positive input terminal and AIN1 is the negative input terminal.
sei(); // Enable global interrupt
while (1); //The main program has no tasks. In any case, it must be an infinite loop.
}
/*
Program testing:
Two potentiometers, one end connected to VCC and the other end connected to ground, constitute a potentiometer voltage divider circuit.
AIN0 and AIN1 are connected to the center tap of the potentiometer respectively.
PBO output string resistor drives LED, high level is valid.
Then rotate the potentiometers to increase or decrease the tap voltage. You will find that the output of PB0 (LED0) changes according to the voltage relationship of AIN0/AIN1.
Due to the influence of power supply ripple, IO current and external interference, when the voltage difference is close to 0V, the analog comparator will produce critical jitter. Connecting a small capacitor to the ground of AIN0/AIN1 can improve this situation.
When using the AVR51 experiment board, since there is only one potentiometer, you need to make some adjustments:
1. Enable ACBG and use the 1.23V internal bandgap reference source instead of AIN0 as the positive input of the analog comparator.
ACSR=(1<
2. Enable the internal 2.56V voltage reference of the ADC, and then connect AIN0 or AIN1 to the pin32 AREF pin.
ADCSRA=(1< //ADC needs to be turned on
ADMUX=(1<
*/
Previous article:C language source program for connecting PC keyboard to AVR microcontroller
Next article:AVR microcontroller program examples based on 74HC595 74HC165
Recommended ReadingLatest update time:2024-11-16 22:53
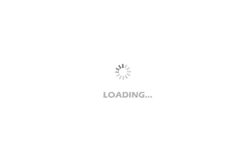
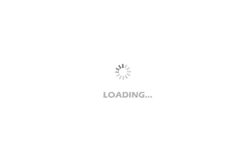
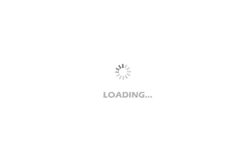
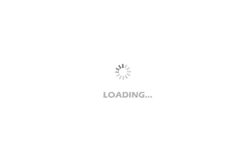
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Metronom Real-Time Operating System RTOS for AVR microcontrollers
-
Learn C language for AVR microcontrollers easily (with video tutorial) (Yan Yu, Li Jia, Qin Wenhai)
-
ATmega16 MCU C language programming classic example (Chen Zhongping)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 【IoT Development Notes】Gizwits Cloud Device Transplantation RT-Thread
- Help with op amp output sine wave signal analysis
- GigaDevice GD Series System Solution Evaluation
- Learn about IO-Link and its five advantages
- 【RT-Thread Reading Notes】Reflections on RT-Thread Chapters 9-12
- 【NXP Rapid IoT Review】+ Familiar with the hardware
- 【Qinheng RISC-V core CH582】BLE lighting
- EEWORLD University ---- Altium Designer 4-layer smart car complete PCB design video tutorial
- How to solve the problem of insufficient ports of 51 MCU? (without adding expansion chips)
- 【TI recommended course】#What is I2C design tool? #