So how to use OpenCV to read and write video files on the ARM board and perform video processing? How to port it?
This requires the installation of x264, xvid and ffmpeg, which is a bit different from the previous article's discussion of obtaining camera video through V4L2 underlying functions.
Here is a method.
Test environment: Ubuntu 12.04 LTS
OpenCV version: 1.0
Cross compiler: arm-none-linux-gnueabi-4.3.2
Proceed as follows:
1. Cross-compile libjpeg
In order to enable OpenCV to process jpeg images, we must cross-compile libjpeg in advance.
The version used here is jpegsrc.v6b
1 Download libjpeg source code: ftp://ftp.uu.net/graphics/jpeg/jpegsrc.v6b.tar.gz
2 Unzip and enter the directory
3 Configure
#./configure --prefix=/root/libjpeg-arm --exec-prefix=/root/libjpeg-arm --enable-shared
--enable-static
The following are the functions of these parameters:
--prefix=/root/libjpeg-arm: After executing make install, the system-independent files will be copied to this directory, as
follows:
/root/libjpeg-arm.....................................
|
+---include........................................
|
---jconfig.h
|
---jerror.h
|
---jmorecfg.h
|
---jpeglib.h
+---man............................................
|
+---man1.......................................
|
---cjeg.1
|
---djpeg.1
|
---jpegtran.1
|
---rdjpgcom.1
|
---wrjpgcom.1
--exec-prefix=/root/libjpeg-arm : After executing make install, system-independent files will be copied to this directory
, that is, some executable programs, dynamic link libraries and static link libraries will be copied to the corresponding directories of this directory, as follows:
/root/libjpeg-arm........................................
|
+---bin............................................
|
---cjeg
|
---djpeg
|
---jpegtran
|
---rdjpgcom
|
---wrjpgcom
+---lib...........................................
|
---libjpeg.la
|
---libjpeg.so
|
---libjpeg.so.62
|
---libjpeg.so.62.0.0
--enable-shared : Use GNU libtool to compile into a dynamic link library.
4 Modify the generated Makefile:
# The name of your C compiler:
CC= gcc should become CC= /root/arm-none-linux-gnueabi/bin/arm-none-linux-gnueabi-gcc
(modify according to the location of your own cross compiler)
# library (.a) file creation command
AR= ar rc should become AR= /root/arm-none-linux-gnueabi/bin/arm-none-linux-gnueabi-ar rc
(same as above)
# second step in .a creation (use "touch" if not needed)
AR2= ranlib should become AR2= /root/arm-none-linux-gnueabi/bin/arm-none-linux-gnueabiranlib
(same as above)
5 Create four directories in the /root/libjpeg-arm directory: man/man1, include, lib, bin6
# make
# make install
7 Copy the files in /root/libjpeg-arm/include/ (jconfig.h, jerror.h, Copy the four header files (jmorecfg.h, jpeglib.h)
to /root/arm-none-linux-gnueabi/arm-none-linux-gnueabi/include.
Copy the four library files (libjpeg.la, libjpeg.so, libjpeg.so.62, libjpeg.so.62.0.0) in /root/libjpeg-arm/lib
to /root/arm-none-linux-gnueabi/arm-none-linux-gnueabi/lib.
Note: Execute the following command to check whether the generated libjpeg.so is the ARM version:
# file libjpeg.so
The following is the correct output, otherwise check the cross compiler path and Makefile and recompile.
Note: After executing the above operations, execute the following command to check whether the library file has been correctly installed:
# arm-linux-gcc -print-file-name=libjpeg.so
If the output is "libjpeg.so", it means it is not installed correctly, repeat step 7.
If the output is "DIR/libjpeg.so", it means it is installed correctly.
At this point, libjpeg cross compilation is completed.
2. Cross compile x264, xvid, ffmpeg
In order to enable OpenCV to process videos, we must cross compile ffmpeg in advance, and ffmpeg depends on x264 and
xvid.
1 Download yasm:
Go to http://www.tortall.net/projects/yasm/wiki/Download Download yasm0.7.2 (x264 requires
the assembly compiler)
# ./configure --enable-shared --prefix=/root/arm-none-linux-gnueabi/arm-none-linuxgnueabi/
--host=arm-linux
# make
# make install
2 Cross-compile x264
to ftp://ftp.videolan.org/pub/videolan/x264/snapshots/ Download x264-snapshot-20060805-
2245.tar.bz2, unzip and enter the directory
(1). Configure
# ./configure --prefix=/root/arm-none-linux-gnueabi/arm-none-linux-gnueabi/ --enableshared
(2). Modify the configuration parameters
Modify config.mak:
prefix=/root/arm-none-linux-gnueabi/arm-none-linux-gnueabi/
exec_prefix=${prefix}
bindir=${exec_prefix}/bin
libdir=${exec_prefix}/lib
includedir=${prefix}/include
# Change to ARM here
ARCH=ARM
SYS=LINUX
# Change to arm-linux-gcc here
CC=arm-linux-gcc
# Remove -DHAVE_MMXEXT -DHAVE_SSE2 -DARCH_X86
CFLAGS=-Wall -I. -O4 -ffast-math -D__X264__ -DHAVE_MALLOC_H -DSYS_LINUX
-DHAVE_PTHREAD -s -fomit-frame-pointer
LDFLAGS= -lm -lpthread -s
AS=nasm
ASFLAGS=-O2 -f elf
VFW=no
GTK=no
EXE=
VIS=no
HAVE_GETOPT_LONG=1
DEVNULL=/dev/null
CONFIGURE_ARGS= '--enable-shared' '--prefix=/root/arm-none-linux-gnueabi/armnone-
linux-gnueabi/'
SONAME=libx264.so.49
default: $(SONAME)
Modify the Makefile and change the ar and ranlib in lines 66~68 to those under arm:
libx264.a: .depend $(OBJS) $(OBJASM)
arm-linux-ar rc libx264.a $(OBJS) $(OBJASM)
arm-linux-ranlib libx264.a
(3). Compile and install
# make
# make install Here you can
generate libx264.so
in the cross-compilation chain directory /root/arm-none-linux-gnueabi/arm-none-linux-gnueabi/ lib
3 Cross-compile xvid
to http://downloads.xvid.org/downloads/xvidcore-1.1.3.tar.gz Download xvid
Download xvid Unzip and enter build/generic
configuration
# ./configure --prefix=/root/arm-none-linux-gnueabi/arm-none-linux-gnueabi/ --disableassembly
[Explanation] --disable-assembly: Because xvid does not have assembly optimization for ARM, assembly must be turned off during compilation
Edit
Modify the platform.inc file referenced by Makefile, change CC=gcc to CC=arm-linux-gcc
# make
# make After install
is successful, enter the example folder
Test, enter
arm-linux-gcc -o xvid_encraw xvid_encraw.c -lc -lm -I../src/ -L../build/generic/=build
-lxvidcore
can generate xvid_encraw. Here you can
generate the corresponding header files and library files under include,lib
in the cross-compilation chain directory /root/arm-none-linux-gnueabi/arm-none-linux-gnueabi/
4 Cross-compile ffmpeg
to http://download.chinaunix.net/download.php?id=5532&ResourceID=2990 Download ffmpeg-0.4.9-p20051120.tar.bz2 from this website
, and then decompress it.
Modify the configure file. The following should be modified.
Since cc, ar, ranlib, and strip are all executable files in the cross-compilation environment, you can configure it like this
prefix="/root/arm-none-linux-gnueabi/arm-none-linux-gnueabi/"
cross_prefix="root/arm-none-linux-gnueabi/bin/arm-linux-"
cpu="arm"
configuration
# ./configure --cpu=arm --cc=arm-linux-gcc --enable-shared --disable-ffserver --enablexvid
--enable-x264 --enable-gpl --enable-pthreads --disable-strip
# make
# make install Here you can
generate the corresponding header files and library files under include,lib
in the cross-compilation chain directory /root/arm-none-linux-gnueabi/arm-none-linux-gnueabi/ So far, all the libraries that OpenCV depends on have been cross-compiled, and
there are corresponding files
under include,bin,share,lib in the cross-compilation chain
3. Cross-compile OpenCV
Download OpenCV-1.0.0 source code http://www.opencv.org.cn/download/opencv-1.0.0.tar.gz
Unzip and enter the directory configuration
# ./configure --host=arm-none-linux-gnueabi --without-gtk --without-carbon --withoutquicktime
--without-1394libs --with-ffmpeg --without-python --without-swig --enable-static
--enable-shared --disable-apps CXX=arm-none-linux-gnueabi-g++ CPPFLAGS=-
I/root/arm-none-linux-gnueabi/arm-none-linux-gnueabi/include LDFLAGS=-L/root/arm-
none-linux-gnueabi/arm-none-linux-gnueabi/lib --with-v4l --prefix=/root/opencv-arm
--libdir=/root/opencv-arm/lib --includedir=/root/opencv-arm/include
Description:
--host=arm-none-linux-gnueabi: indicates cross-compile arm platform
--without-gtk: ignore gtk+2.0 windows
--without-carbon: do not use X library on Mac OS
--without-quicktime
--without-1394libs
--without-ffmpeg
--without-python
--without-swig
--enable-static: generate static library
--enable-shared: generate dynamic library
CXX=arm-none-linux-gnueabi-g++: specify compilation tool
CPPFLAGS=-I/root/arm-none-linux-gnueabi/arm-none-linux-gnueabi/include:
OpenCV will use some dev packages, such as png.h, jpeglib.h, most header files are in /usr/include
--prefix=/root/opencv-arm: specify the installation directory
-libdir=/root/opencv-arm/lib: specify the library file installation location
--includedir=/root/opencv-arm/include: specify the include file installation location
If the configuration is correct, there will be the following informationGeneral
configuration ====================================================
Compiler: arm-none-linux-gnueabi-g++
CXXFLAGS: -Wall -fno-rtti -pipe -O3 -fomit-frame-pointer
Install path: /root/opencv-arm
HighGUI configuration ================================================
Windowing system --------------
Use Carbon / Mac OS X: no
Use gtk+ 2.x: no
Use gthread: no
Image I/O --------------------------
Use libjpeg: yes
Use zlib: yes
Use libpng: yes
Use libtiff: no
Use libjasper: no
Use libIlmImf: no
Video I/O --------------------------
Use QuickTime / Mac OS X: no
Use xine: no
Use ffmpeg: yes
Use dc1394 & raw1394: no
Use v4l: yes
Use v4l2: yes
Wrappers for other languages ===================== ====================
SWIG
Python no
Additional build settings ======================== ====================
Build demo apps no
Now run make...
================================================== ==================
===
# make
# make install
The libraries required to run OpenCV on arm:
1. Copy the library file
libcvaux. so.1.0.0
libcv.so.1.0.0
libcxcore.so.1.0.0
libhighgui.so.1.0.0
libml.so.1.0.0
Copy them and rename them to
*.so.1
2 Add the previous library files and
copy the libjpeg, xvid, x264, and ffmpeg libraries under /root/arm-none-linux-gnueabi/arm-none-linux-gnueabi/lib to the board's /usr/lib or /lib, or you can go to a
folder on the board, and then #export LD_LIBRARY_PATH=$LD_LIBRARY_PATH:/YOUR/lib/DIR
3 List of libraries required by OpenCV:
Compile the source file method
arm-none-linux-gnueabi-g++ demo.c -o demo -I/root/opencv-arm/include/opencv
-L/root/opencv-arm/lib -lcv -lcxcore -lpthread -lrt -lcvaux -lm -lpng -ljpeg -lz -lml -lhighgui
-ldlFinally
, copy the generated binary file to the board and run it
Previous article:Porting strace debugging tool to arm platform
Next article:How to port lua to the linux kernel of the arm platform
Recommended ReadingLatest update time:2024-11-17 15:59
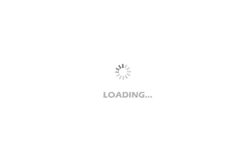
- Popular Resources
- Popular amplifiers
-
Deep Learning and Computer Vision: Core Algorithms and Applications (Xie Wenwei)
-
Python Computer Vision and Natural Language Processing: Developing Robotic Application Systems
-
imx93 opencv-4.8.0 and opencv-contrib-4.8.0 cross-compiled library files
-
Deep Learning and Computer Vision in Autonomous Driving
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- New breakthrough! Ultra-fast memory accelerates Intel Xeon 6-core processors
- New breakthrough! Ultra-fast memory accelerates Intel Xeon 6-core processors
- Consolidating vRAN sites onto a single server helps operators reduce total cost of ownership
- Consolidating vRAN sites onto a single server helps operators reduce total cost of ownership
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- STEVAL-MKI109V3 recent debugging experience and problem sharing
- Why are relays still used to drive automotive motors?
- [Rawpixel RVB2601 development board trial experience] 3. OLED display of temperature and humidity
- AD9 Application Help
- Dongguan Alda New Materials Technology Co., Ltd. recruits electronic engineers
- Sonoff WiFi Smart Socket Network Service
- How to convert a DC 5V power supply into ±12V
- Live broadcast at 10:00 am today: ADI energy storage system helps build electric vehicle fast charging stations
- Please tell me about the inductance in this boost circuit
- There is no better way to view and visualize your swept 3D spherical pattern results.