Chapter 4 Instruction System
Data Transfer Instructions
Register to Register Transfer: MOV Instruction, MVN Instruction
MOV R8, R3; R8 = R3
MVN R8, R3; R8 = -R3
Those who have studied microcomputer principles should remember that in x86, a MOV instruction can transfer data between memory and registers. This is not possible in ARM, which is also a more obvious difference between CISC and RISC cores.
Memory to Register Transfer: LDRx Instruction, LDMxy Instruction
Register to Memory: STRx Instruction, STMxy Instruction
The x in the LDRx instruction can be B (byte), H (half word), D (double word), or omitted (word). The specific usage is as follows:
Example |
Functional Description |
LDRB Rd, [Rn, #offset] |
Read a byte from address Rn+offset and send it to Rd |
LDRH Rd, [Rn, #offset] |
Read a half word from address Rn+offset and send it to Rd |
LDR Rd, [Rn, #offset] |
Read a word from address Rn+offset and send it to Rd |
LDRD Rd1, Rd2, [Rn, #offset] |
Read a double word (64-bit integer) from address Rn+offset and send it to Rd1 (low 32 bits) and Rd2 (high 32 bits). |
The x in the STRx instruction can also be B (byte), H (half word), D (double word), or omitted (word). The specific usage is as follows:
Example |
Functional Description |
STRB Rd, [Rn, #offset] |
Store the low byte in Rd to address Rn+offset |
STRH Rd, [Rn, #offset] |
Store the lower halfword in Rd to address Rn+offset |
STR Rd, [Rn, #offset] |
Store the low word in Rd to address Rn+offset |
STRD Rd1, Rd2, [Rn, #offset] |
Store the double word represented by Rd1 (low 32 bits) and Rd2 (high 32 bits) at address Rn+offset |
The LDRx and STRx instructions also have a pre-indexed format. Here is an example (note the "!" in the statement):
LDR.W R0,[R1, #20]! ; Pre-index
The above statement means to first load the value at address R1+offset into R0, then R1 ßR1+ 20
There is also a post-index form, note the difference from the pre-index above (also note that there is no "!" in the statement):
STR.W R0, [R1], #-12; store the value of R0 to address R1. After completion, R1ßR1+(-12)
The LDMxy and STMxy instructions can transfer more data at one time.
X can be I or D, I means increment, D means decrement.
Y can be A or B, indicating whether the timing of self-increment or self-decrement is before (Before) or after (After) each access.
In addition, an instruction with a ".W" suffix indicates that the instruction is a 32-bit Thumb-2 instruction, otherwise it is a 16-bit instruction.
Example |
Functional Description |
LDMIA Rd!, {register list} |
Read multiple words from Rd and send them to the registers in the register list in sequence. Rd increments once after each word is read. 16-bit instructions |
LDMIA.W Rd!, {register list} |
Read multiple words from Rd and send them to the registers in the register list in sequence. Rd increments once after each word is read. |
STMIA Rd!, {register list} |
Store the values of each register in the register list to the address given by Rd in sequence. Rd increments once after each word is stored. 16-bit instructions |
STMIA.W Rd!, {register list} |
Store the values of each register in the register list to the address given by Rd in sequence. Rd increments once after each word is stored. |
LDMDB.W Rd!, {register list} |
Read multiple words from Rd and send them to the registers in the register list in sequence. Rd is decremented before each word is read. |
STMDB.W Rd!, {register list} |
Store multiple words in Rd. Rd is decremented before each word is stored. |
Here we need to pay special attention to the meaning of ! It means to increment (Increment) or decrement (Decrement) the value of the base register Rd, before (Before) or after (After) each access. For example:
Assume R8 = 0x8000, then
STMIA.W R8!, {R0-R3} ; R8 value becomes 0x8010
STMIA.W R8, {R0-R3} ; R8 value remains unchanged
Both lines of code above store 16 bytes of data from R0 to R3 in the 16-byte space starting from 0x8000. The only difference is that after the first instruction is executed, R8 is updated to 0x8010, while the second instruction does not update R8.
Loading an immediate value
MOV supports 8-bit immediate loads, such as:
MOV R0, #0x12
The 32-bit instructions MOVW (load to the lower 16 bits of a register) and MOVT (load to the upper 16 bits of a register) can support 16-bit immediate value loading. If you want to load a 32-bit immediate value, you must use MOVW first and then MOVT, because MOVW will clear the upper 16 bits.
Differences between LDR and ADR
LDR and ADR are both pseudo instructions, and can be used to load an immediate value (or an address). If the program address is loaded, LDR will automatically set the LSB, but ADR will not:
LDR R0, =address1 ; R0= 0x4000 | 1
ADR R1, address1; R1 = 0x4000. Note: There is no "=" sign
…
address1
0x4000: MOV R0, R1
Special function registers can only be accessed using MSR/MRS instructions:
MRS
MSR
Here are two examples:
MRS R0, PRIMASK ; Read PRIMASK into R0
MSR BASEPRI, R0 ; write R0 to BASEPRI
However, it should be noted that most special function registers can only be accessed at the privileged level, and only APSR can be accessed at the non-privileged level.
Previous article:Linux driver--ADC driver
Next article:STM32F10x Study Notes 10 (Basic Timer)
Recommended ReadingLatest update time:2024-11-16 15:00
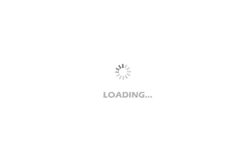
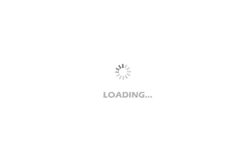
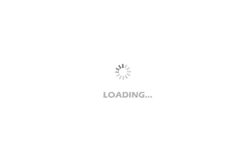
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Loss Analysis of MOSFET in Flyback Critical Operation Mode
- [SAMR21 new gameplay] 30. Pin definition
- In fact, the original "analog electronics" and "digital electronics" are incompatible!
- Learning ARM-LINUX embedded system based on LS_BBB development board Part 1: Platform Overview
- What is loop response?
- GD32E231 DIY Competition (3) GD32E231C driving OLED (128X32)
- Looking for domestic brushless motor control chip?
- A serial screen product with analog video signal usage sharing
- Useful information | Four major development skills for microcontrollers!
- 【TI mmWave Radar Review】+Configuration File Test (I)