In Keil C51, call the library function directly:
#include // declares void _nop_(void);
_nop_(); // Generate a NOP instruction
Function: For very short delays, which are required to be at the us level, use the "_nop_" function, which is equivalent to the assembly NOP instruction, with a delay of several microseconds. The NOP instruction is a single-cycle instruction, and the delay time can be calculated from the crystal frequency. For a 12M crystal, the delay is 1uS. For longer delays, which are required to be greater than 10us, use the loop statement in C51 to achieve it.
When choosing a loop statement in C51, pay attention to the following issues
First, when defining loop variables in C51, try to use unsigned character variables.
Second, in the FOR loop statement, try to use variable subtraction to loop.
Third, in the do...while and while statements, the variables in the loop body are also reduced.
This is because in the C51 compiler, different instructions are used to implement different loop methods.
Here are some examples:
unsigned char i;
for(i=0;i<255;i++);
unsigned char i;
for(i=255;i>0;i--);
Among them, after the second loop statement C51 is compiled, it is completed with the DJNZ instruction, which is equivalent to the following instruction:
MOV 09H, #0FFH
LOOP: DJNZ 09H, LOOP
The instructions are quite concise and it is also easy to calculate the precise delay time.
The same is true for do...while and while loop statements.
example:
unsigned char n;
n=255;
do{n--}
while(n);
or
n=255;
while(n)
{n--};
After these two loop statements are compiled by C51, they form the method completed by DJNZ.
Therefore, it is also very convenient to calculate the exact time.
Third: For those who require a longer delay time, the nested loop method should be used. Therefore, the nested loop method is often used to achieve a delay of ms. The loop statement can also be completed using the for, do...while, while structure, and the variables in each loop body are still unsigned character variables.
unsigned char i,j
for(i=255;i>0;i--)
for(j=255;j>0;j--);
or
unsigned char i,j
i=255;
do{j=255;
do{j--}
while(j);
i--;
}
while(i);
or
unsigned char i,j
i=255;
while(i)
{j=255;
while(j)
{j--};
i--;
}
All three methods use DJNZ instructions to nest loops, which are implemented by the C51 compiler using the following instruction combination:
MOV R7, #0FFH
LOOP2: MOV R6, #0FFH
LOOP1: DJNZ R6, LOOP1
DJNZ R7, LOOP2
The combination of these instructions uses the DJNZ instruction in assembly language to delay, so its accurate time calculation is also very simple. Assuming that the initial value of the variable i is m and the initial value of the variable j is n, the total delay time is: m×(n×T+T), where T is the execution time of the DJNZ instruction (DJNZ instruction is a two-cycle instruction). Here +T is the time used by the MOV instruction. Similarly, for longer delays, multiple cycles can be used to complete it.
Just pay attention to the above issues when programming loop statements.
The following are some issues to pay attention to when designing a delay subroutine in C51:
1. When designing precise delay subroutines in C51, try not to define local variables in the delay subroutines or define as few local variables as possible in the delay subroutines. All variables in the delay subroutines are passed through parameterized functions.
2. When designing a delay subroutine, it is better to use the do...while structure as the loop body rather than the for structure.
3. When designing a delay subroutine, if you want to nest the loop body, it is better to use the inner loop first and then reduce it rather than reduce it first and then the inner loop.
unsigned char delay(unsigned char i,unsigned char j,unsigned char k)
{unsigned char b,c;
b="j";
c="k";
do{
do{
do{k--};
while(k);
k="c";
j--;};
while(j);
j=b;
i--;};
while(i);
}
This precise delay subroutine is compiled by C51 into the following instruction combination
The delay subroutine is as follows:
MOV R6,05H
MOV R4,03H
C0012: DJNZ R3, C0012
MOV R3,04H
DJNZ R5, C0012
MOV R5,06H
DJNZ R7, C0012
RET
Assuming that the initial value of parameter variable i is m, the initial value of parameter variable j is n, and the initial value of parameter variable k is l, the total delay time is: l×(n×(m×T+2T)+2T)+3T, where T is the execution time of DJNZ and MOV instructions. When m=n=l, the precise delay is 9T, the shortest; when m=n=l=256, the precise delay is 16908803T, the longest.
Previous article:Summary of single chip microcomputer delay method
Next article:How to write efficient and beautiful C language code for microcontrollers?
Recommended ReadingLatest update time:2024-11-17 07:17
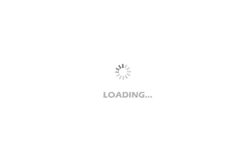
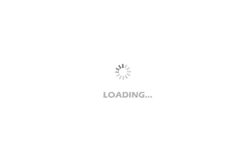
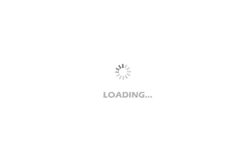
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- PCB issues for switching power supplies
- [Zero-knowledge ESP8266 tutorial] Quick start 21 world clock demo
- A question about the schematic
- National College Student Electronic Design Competition Open Source Flight Control Data Album: APM Learning Materials
- How to modify this PCB
- Annual review: 2019 TI training course highlights, good reviews and gifts!
- Which development environment do I use for C8051F58x/F59x?
- C2000 CLA FAQ: Accessing Peripherals
- Signal isolator selection problem
- [15th Anniversary] Let's DIY an electronic toolbox together, I want the tool software - DFlash (tentative)