* File name: main.c
* Program author: kidcao1987
* Program version: V1.0
* Function description: Install LCD1602 and use it to display on LCD1602. It is recommended to connect an external power supply.
"This a LCD demo!
www.CEPARK.com
Keep moving!!
By:kicao1987"
* Compiler: WinAVR-20090313
* Chip: ATmega16, external 11.0592MHZ crystal oscillator
* Technical support: http://bbs.cepark.com
***********************************************************************/
#include
#include
#define SETLCD1602RS PORTA |= (1<
unsigned char const ucString1[]={"This a LCD demo!"};
unsigned char const ucString2[]={" www.CEPARK.com "};
unsigned char const ucString3[]={" Keep moving!! "};
unsigned char const ucString4[]={" By:kicao1987 "};
void LCD1602BusyCheck(void); //Busy detection
void LCD1602WriteOperation(unsigned char,unsigned char); //Write operation
void LCD1602Init(void); //Initialization function
void LCD1602MoveToPosition(unsigned char,unsigned char); //Locate to the specified position
void LCD1602PrintString(unsigned char const * str); //Output a string of characters
int main(void)
{
PORTA = 0xFF;
DDRA = 0xFF;
PORTB = 0xFF;
DDRB = 0xFF;
LCD1602Init();
while(1)
{
LCD1602MoveToPosition(0,0);
LCD1602PrintString(ucString1);
LCD1602MoveToPosition(1,0);
LCD1602PrintString(ucString2);
_delay_ms(5000); //Delay 5 milliseconds
LCD1602MoveToPosition(0,0);
LCD1602PrintString(ucString3);
LCD1602MoveToPosition(1,0); //Delay 5 milliseconds
LCD1602PrintString(ucString4);
_delay_ms(5000);
}
}
//Busy detection
void LCD1602BusyCheck(void)
{
unsigned char i = 254;
DDRB = 0x00; //Set the highest bit to input
CLRLCD1602RS;
SETLCD1602RW;
SETLCD1602EN;
_delay_us(5);
while((i--) && (PINB & 0x80));
DDRB = 0xff;
_delay_us(5);
CLRLCD1602EN;
_delay_us(5);
}
//Write operation
void LCD1602WriteOperation(unsigned char Data,unsigned char ComOrData)
{
LCD1602BusyCheck(); //ComOrData=0 is command, 1 is data
if(0 == ComOrData)
CLRLCD1602RS;
else if(1 == ComOrData)
SETLCD1602RS;
CLRLCD1602RW;
SETLCD1602EN;
_delay_us(5);
PORTB = Data;
_delay_us(5);
CLRLCD1602EN;
_delay_us(100);
}
void LCD1602Init(void) //Initialization
{
LCD1602WriteOperation(0x38,0);
_delay_ms(15);
LCD1602WriteOperation(0x38,0);
_delay_ms(5);
LCD1602WriteOperation(0x38,0);
_delay_ms(5);
LCD1602WriteOperation(0x38,0);
_delay_ms(5);
LCD1602WriteOperation( 0x0c,0);
_delay_ms(1);
LCD1602WriteOperation(0x06,0);
_delay_ms(1);
LCD1602WriteOperation(0x01,0);
_delay_ms(1);
}
void LCD1602MoveToPosition(unsigned char ucx,unsigned char ucy)
{
if(0 == ucx) //First line
{
LCD1602WriteOperation(0x80 + ucy,0); //Yth column
}
if(1 == ucx) //Second row
{
LCD1602WriteOperation(0xC0 + ucy,0); //第Y列
}
}
void LCD1602PrintString(unsigned char const * str)
{
while(*str != '')
{
LCD1602WriteOperation(*str,1);
str++;
}
}
Video address: http://v.youku.com/v_show/id_XMTYxNDg1MDQ0.html
Previous article:AVR M16 Experiment 3 PWM Experiment
Next article:AVR M16 Experiment 1: LED Flashing
Recommended ReadingLatest update time:2024-11-17 00:44
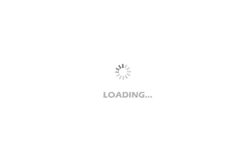
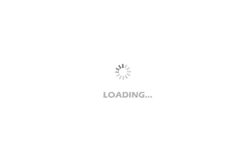
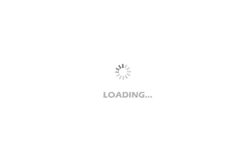
- Popular Resources
- Popular amplifiers
-
Learning PLC is easy - PLC ladder diagram and statement table with pictures (Li Changjun, Zhou Hua)
-
A Complete Illustrated Guide to Operational Amplifier Applications (Written by Wang Zhenhong)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Retiming in Vivado Synthesis Operations
- Short circuit, help needed
- A brief description of the stack structure in the ZigBee standard
- Digital voltmeter based on ADC0832
- Does anyone know how to use a can recognition device?
- Low power radio frequency wireless data acquisition node circuit
- Analysis of Capacitance and Resistance Changes Caused by PCB Layout
- LSM6DS3 calibration issue
- F28335 ADC learning process
- Development board directly for AI scenarios: phyBOARD-i.MX 8M Plus development board free application in progress!