1. GND
2. DQ
3. VCC
DQ → P1.7
K1 → P3.2
K2 → P3.4
K3 → P3.6
K4 → P3.7
The temperature is displayed using a four-digit digital tube. When the temperature is >= 100 degrees, the fourth digit is displayed.
The fifth digit displays the status symbol.
Power on and detect DS18B20 status:
DS18B20 normal display:
O 18.8 ← Display real-time temperature and heating mark "O"
DS18B20 displays abnormally:
black screen and buzzer keeps beeping.
At this time, you need to check whether the DS18B20 is connected properly and correctly, otherwise you need to replace it with a new DS18B20 chip.
The program will always test the DS18B20 during the whole process.
You can unplug the DS18B20 from the 51hei-5 to test this function.
1. Check the temperature alarm value:
K1 → Enter to view the temperature alarm value TL status:
L--20
TL: 20 low alarm value
K1 → Enter to view the temperature alarm value TH status:
H--28
TH: 28 high alarm value
K1 → Exit to view the temperature alarm value status.
2. Set the temperature alarm value:
1. K3 → Enter the set temperature alarm value TL state:
L--20
2. K3 → Enter the set temperature alarm value TH state:
H--28
3. K3 → Return
4. Setting process: K1 → UP key, K2 → DOWN key, for quick adjustment.
The set temperature alarm value is automatically stored in the EEROM of DS18B20 and can be permanently saved.
The temperature alarm value is automatically read from the EEROM of DS18B20 every time the power is turned on.
3. Display of alarm status:
1. When the actual temperature is greater than the set value of TH,
H 38.8 H → flashes
Closes the relay, indicating overtemperature.
2. When the actual temperature is lower than the set value of TL,
L 18.8 L → flashes
Indicates that the heating section is faulty.
3. When the actual temperature is less than the set value of TH, the relay is energized and heating begins.
The heating mark is "O"
4. Other functions
1. Display the markers “H”, “L” and “O” in a flashing manner.
2. When the hundreds digit of the real-time temperature is "0", it will not be displayed.
3. When the hundreds digit of the alarm temperature value is "0", "-" is displayed.
Complete program code download address: http://www.51hei.com/f/DS18B20 temperature control LED display_asm.rar
;****************************************************** **************** ;* Title: 51Hei MCU Development System Demonstration Program - DS18B20 Temperature Control Digital Tube Display * ;* Crystal: 12M * ;* Jumper settings: short-circuit J5's 12th pin with a jumper cap, connect J5's 3rd pin to the top P1.0 extension with a Dupont line, and set the others to default* * ;* Website: http://www.51HEI.com * ;****************************************************** ***************** ;* describe: * ;* DS18B20 temperature control digital tube display * ;* 1. K3 → Enter the set temperature alarm value TL state: * ;* L--20 * ;* 2. K3 → Enter the set temperature alarm value TH state: * ;* H--28 * ;* 3. K3 → Return * ;* 4. Setting process: K1 → UP key, K2 → DOWN key, can be adjusted quickly. * ;* * ;****************************************************** ******************************* TIMER_L DATA 23H TIMER_H DATA 24H TIMER_COUN DATA 25H TEMPL DATA 26H TEMPH DATA 27H TEMP_TH DATA 28H TEMP_TL DATA 29H TEMPHC DATA 2AH TEMPLC DATA 2BH TEMP_ZH DATA 2CH BEEP EQU P1.0 DATA_LINE EQU P1.7 RELAY EQU P2.2 FLAG1 EQU 20H.0 FLAG2 EQU 20H.1 ;------------------------------------------------- K1 EQU P3.2 K2 EQU P3.4 K3 EQU P3.6 K4 EQU P3.7 ;================================================== ORG 0000H JMP MAIN ORG 000BH AJMP INT_T0 ;------------------------------------------------- - MAIN: MOV SP,#30H ; The function of the following 5 lines is to turn off the output of the 8*8 dot matrix, that is, to set all the lines to 0, so that no matter what level the dot matrix is, it will not light up. MOV R2,#8 ; Output data 8 bits hei: CLR P2.4; Pull SCL low so that the next time it is pulled high, a rising edge will be generated CLR P2.5; data is first sent to 74hc164, waiting for the rising edge of clk SETB P2.4; Low level to high level edge trigger arrives, parallel data output to Q0-Q7 of 164 DJNZ R2,hei ; Determine whether the output is completed MOV TMOD,#01H ;T0,mode 1 MOV TIMER_L,#00H; 50ms timing value MOV TIMER_H,#4CH MOV TIMER_COUN,#00H ; interrupt count MOV IE,#82H ;EA=1,ET0=1 LCALL READ_E2 ;LCALL RE_18B20 MOV 20H,#00H SETB BEEP SETB RELAY MOV 7FH,#0AH ; Extinguish symbol CALL RESET; reset and detect DS18B20 JNB FLAG1, MAIN1; FLAG1 = 0, DS18B20 does not exist JMP START MAIN1: CALL RESET JB FLAG1, START LCALL BEEP_BL ;DS18B20 error, alarm JMP MAIN1 START: MOV A,#0CCH ; Skip ROM matching CALL WRITE MOV A,#044H ; Issue temperature conversion command CALL WRITE CALL RESET MOV A,#0CCH ; Skip ROM matching CALL WRITE MOV A,#0BEH ; Issue a temperature read command CALL WRITE CALL READ ; Read temperature data CALL CONVTEMP CALL DISPBCD CALL DISP1 CALL SCANKEY LCALL TEMP_COMP JMP MAIN1 ;================================================== ==== ;DS18B20 reset and detection subroutine ;FLAG1=1 OK, FLAG1=0 ERROR ;================================================== ===== RESET: SETB DATA_LINE NOP CLR DATA_LINE MOV R0,#64H; The host sends a reset low pulse with a delay of 600 microseconds MOV R1,#03H RESET1: DJNZ R0,$ MOV R0,#64H DJNZ R1,RESET1 SETB DATA_LINE; then pull the data line high NOP MOV R0,#25H RESET2: JNB DATA_LINE,RESET3; Wait for DS18B20 to respond DJNZ R0,RESET2 JMP RESET4 ; Delay RESET3: SETB FLAG1; Set the flag to indicate that DS1820 exists JMP RESET5 RESET4: CLR FLAG1; Clear the flag, indicating that DS1820 does not exist JMP RESET6 RESET5: MOV R0,#064H DJNZ R0,$ ; Timing requires a delay of some time RESET6: SETB DATA_LINE RET ;================================================== ========== ; ;================================================== ========== WRITE: MOV R2,#8; 8 bits of data in total CLR CY WR1: CLR DATA_LINE ; Start writing to the DS18B20 bus and put it in the reset (low) state MOV R3,#09 DJNZ R3,$ ; Bus reset is maintained for more than 18 microseconds RRC A ; divide a byte DATA into 8 BIT rings and transfers them to C MOV DATA_LINE,C ; write a BIT MOV R3,#23 DJNZ R3,$ ; Wait 46 microseconds SETB DATA_LINE ;Release the bus NOP DJNZ R2,WR1 ;Write the next BIT SETB DATA_LINE RET ;================================================== =========== ; Read the low temperature, high temperature and alarm values TH and TL from DS18B20 ; Store in 26H, 27H, 28H, 29H ;================================================== =========== READ: MOV R4,#4 ; Read the high and low bits of temperature from DS18B20 MOV R1,#26H ; store 26H, 27H, 28H, 29H RE00: MOV R2,#8 RE01: CLR C SETB DATA_LINE NOP NOP CLR DATA_LINE ; bus line is kept low before reading NOP NOP NOP SETB DATA_LINE ; Start reading bus release MOV R3,#09 ;Delay 18 microseconds DJNZ R3,$ MOV C, DATA_LINE; read a BIT from the DS18B20 bus MOV R3,#23 DJNZ R3,$ ; Wait 46 microseconds RRC A; Circularly shift the read bit value to A DJNZ R2,RE01 ;Read next BIT MOV @R1,A INC R1 DJNZ R4,RE00 RET [page] ;-------------------------------------------------- ; Invert the flashing mark once every 200ms ;-------------------------------------------------- INT_T0: PUSH ACC PUSH PSW MOV TL0,TIMER_L MOV TH0,TIMER_H INC TIMER_COUN MOV A,TIMER_COUN CJNE A,#04H,INT_END MOV TIMER_COUN,#00H CPL FLAG2 INT_END: POP PSW POP ACC RETI ;================================================== ========= ; Reinitialize DS18B20 ;Write the set temperature alarm value into DS18B20 ;================================================== ========= RE_18B20: JB FLAG1,RE_18B20A RET RE_18B20A: CALL RESET MOV A,#0CCH ; Skip ROM matching LCALL WRITE MOV A,#4EH ; write to temporary register LCALL WRITE MOV A,TEMP_TH; TH (alarm upper limit) LCALL WRITE MOV A,TEMP_TL; TL (lower limit of alarm) LCALL WRITE MOV A,#7FH ;12-bit precision LCALL WRITE RET ;================================================== === ; Function key scanning subroutine ;================================================== === SCANKEY: ;MOV P1,#0F0H JB K1,SCAN_K2 CALL BEEP_BL SCAN_K1: CALL ALERT_TL CALL ALERT_PLAY JB K1,SCAN_K1 CALL BEEP_BL SCAN_K11: CALL ALERT_TH CALL ALERT_PLAY JB K1,SCAN_K11 CALL BEEP_BL SCAN_K2: JB K2,SCAN_K3 CALL BEEP_BL SCAN_K3: JB K3,SCAN_K4 CALL BEEP_BL LCALL RESET_ALERT LCALL RE_18B20 LCALL WRITE_E2 SCAN_K4: JB K4,SCAN_END CALL BEEP_BL SCAN_END: RET ;================================================ ;Set temperature alarm value ;================================================ RESET_ALERT: CALL ALERT_TL CALL ALERT_PLAY JNB K3,$; K3 is the shift key SETB TR0 RESET_TL: CALL ALERT_PLAY JNB FLAG2,R_TL01 mov 75H,7fh ; send the extinguishing symbol mov 76H,7fh CALL ALERT_PLAY JMP R_TL02 R_TL01: CALL ALERT_TL mov 75h,7Eh ; send set value mov 76h,7Dh CALL ALERT_PLAY ; Display the setting value R_TL02: JNB K1,K011A JNB K2,K011B JNB K3,RESET_TH JMP RESET_TL K011A: INC TEMP_TL MOV A,TEMP_TL CJNE A,#120,K012A; Not reaching the upper limit, turn MOV TEMP_TL,#0 K012A: CALL TL_DEL JMP RESET_TL K011B: DEC TEMP_TL MOV A,TEMP_TL CJNE A,#00H,K012B; If the lower limit is not reached, turn MOV TEMP_TL,#119 K012B: CALL TL_DEL JMP RESET_TL ;------------------------------------------------- ------ RESET_TH: CALL BEEP_BL JNB K3,$ RESET_TH1: CALL ALERT_PLAY JNB FLAG2,R_TH01 mov 75H,7fh ; send the extinguishing symbol mov 76H,7fh CALL ALERT_PLAY JMP R_TH02 R_TH01: CALL ALERT_TH mov 75h,7Eh ; mov 76h,7Dh CALL ALERT_PLAY R_TH02: JNB K1,K021A JNB K2,K021B JNB K3,K002 JMP RESET_TH1 K021A: INC TEMP_TH MOV A,TEMP_TH CJNE A,#120,K022A; Not reaching the upper limit, turn MOV TEMP_TH,#0 K022A: CALL TH_DEL JMP RESET_TH1 K021B: DEC TEMP_TH ; decrement 1 MOV A,TEMP_TH CJNE A,#00H,K022B; If the lower limit is not reached, turn MOV TEMP_TH,#119 K022B: CALL TH_DEL JMP RESET_TH1 K002: CALL BEEP_BL CLR TR0 ; turn off interrupt RET ;------------------------------------------------- ---- ;Key delay subroutine ; Call the alarm value display program multiple times to delay ;------------------------------------------------- ---- TL_DEL: ; Alarm low value delay MOV R2,#0AH TL_DEL1: CALL ALERT_TL CALL ALERT_PLAY DJNZ R2,TL_DEL1 RET TH_DEL: ; Alarm high value delay MOV R2,#0AH TH_DEL1: CALL ALERT_TH CALL ALERT_PLAY DJNZ R2,TH_DEL1 RET ;================================================== === ; Subroutine to compare the real-time temperature value with the set alarm temperature value TH, TL ;When the actual temperature is greater than the set value of TH, "H" is displayed and the relay is closed. ;When the actual temperature is lower than the set value of TH, “O” is displayed and the relay is energized. ;When the actual temperature is less than the set value of TL, “L” is displayed. ; Flashing display markers H, L, O ;================================================== === TEMP_COMP: SETB TR0 ; Start interrupt MOV A,TEMP_TH SUBB A,TEMP_ZH ; if subtrahend > minuend, then JC CHULI1 ; Borrow flag C=1, transfer MOV A,TEMP_ZH SUBB A,TEMP_TL; if subtrahend > minuend, then JC CHULI2 ; Borrow flag C = 1, transfer JNB FLAG2,T_COMP1; FLAG2=0, display mark character MOV 74H,#0AH ; Extinguish symbol LCALL DISP1 JMP T_COMP2 T_COMP1: MOV 74H,#00H LCALL DISP1 ;Display "O" T_COMP2: CLR RELAY; relay energized CLR TR0 ; turn off interrupt RET ;------------------------------------------------ ;Overheating treatment ;------------------------------------------------ CHULI1: SETB RELAY ;Relay off JNB FLAG2,CHULI10 MOV 74H,#0AH ; Extinguish symbol LCALL DISP1 JMP CHULI11 CHULI10: MOV 74H,#0DH LCALL DISP1 ;Display "H" ;CALL BEEP_BL ;Buzzer sounds CHULI11: CLR TR0 ; turn off interrupt RET ;------------------------------------------------ ;Undertemperature treatment ;------------------------------------------------ CHULI2: ;Under-temperature treatment JNB FLAG2,CHULI20 MOV 74H,#0AH ; Extinguish symbol LCALL DISP1 JMP CHULI21 CHULI20: MOV 74H,#0CH LCALL DISP1 ;Display "L" ;CALL BEEP_BL ;Buzzer sounds CHULI21: CLR TR0 ; Disable interrupt RET ;------------------------------------------------- ---------- ;Copy the temperature alarm value in the DS18B20 register to the EEROM ;------------------------------------------------- ---------- WRITE_E2: CALL RESET MOV A,#0CCH ; Skip ROM matching LCALL WRITE MOV A,#48H ;Copy the temperature alarm value to EEROM LCALL WRITE RET ;------------------------------------------------- ------------- ;Copy the temperature alarm value in DS18B20 EEROM back to the register ;------------------------------------------------- ---------- READ_E2: CALL RESET MOV A,#0CCH ; Skip ROM matching LCALL WRITE MOV A,#0B8H ;Copy the temperature alarm value back to the register CALL WRITE RET [page] ;****************************************************** **** ; Processing temperature BCD code subroutine ;****************************************************** *** CONVTEMP: MOV A,TEMPH; Determine whether the temperature is below zero ANL A,#80H JZ TEMPC1 ; Temperature zero upward CLR C MOV A,TEMPL ; Binary complement (double byte) CPL A ; invert and add 1 ADD A,#01H MOV TEMPL,A MOV A,TEMPH ;- CPL A ADDC A,#00H MOV TEMPH,A ;TEMPHC HI = sign bit MOV TEMPHC,#0BH SJMP TEMPC11 TEMPC1: MOV TEMPHC,#0AH; TEMPC11: MOV A,TEMPHC SWAP A MOV TEMPHC,A MOV A,TEMPL ANL A,#0FH ; multiply by 0.0625 MOV DPTR,#TEMPDOTTAB MOVC A,@A+DPTR MOV TEMPLC,A ;TEMPLC LOW = decimal part BCD MOV A,TEMPL ; integer part ANL A,#0F0H SWAP A MOV TEMPL,A MOV A,TEMPH ANL A,#0FH SWAP A ORL A,TEMPL MOV TEMP_ZH,A ; Store the combined value into TEMP_ZH LCALL HEX2BCD1 MOV TEMPL,A ANL A,#0F0H SWAP A ORL A,TEMPHC ;TEMPHC LOW = Tens digit BCD MOV TEMPHC,A MOV A,TEMPL ANL A,#0FH SWAP A ;TEMPLC HI = single digit BCD ORL A,TEMPLC MOV TEMPLC,A MOV A,R7 JZ TEMPC12 ANL A,#0FH SWAP A MOV R7,A MOV A,TEMPHC ;TEMPHC HI = hundreds digit BCD ANL A,#0FH ORL A,R7 MOV TEMPHC,A TEMPC12: RET ;------------------------------------------------- ---------- ; Decimal code table ;------------------------------------------------- ---------- TEMPDOTTAB: DB 00H,01H,01H,02H,03H,03H,04H,04H,05H,06H DB 06H,07H,08H,08H,09H,09H ;================================================== ========== ; Display area BCD code temperature value refresh subroutine ;================================================== ========== DISPBCD: MOV A,TEMPLC ANL A,#0FH MOV 70H,A ; decimal place MOV A,TEMPLC SWAP A ANL A,#0FH MOV 71H,A ; units digit MOV A,TEMPHC ANL A,#0FH MOV 72H,A ; Tens digit MOV A,TEMPHC SWAP A ANL A,#0FH MOV 73H,A ; Hundreds place MOV A,TEMPHC ANL A,#0F0H CJNE A,#010H,DISPBCD0 SJMP DISPBCD2 DISPBCD0: MOV A,TEMPHC ANL A,#0FH JNZ DISPBCD2; the tens digit is 0 MOV A,TEMPHC SWAP A ANL A,#0FH MOV 73H,#0AH ;Sign bit is not displayed MOV 72H,A ; Tens digit display sign DISPBCD2: RET ;****************************************************** **************** ; Temperature display subroutine ;****************************************************** **************** ; Display data in 70H - 73H unit, using 4-bit common anode digital tube display, P0 port output segment code data, ; Port P0 is used for scanning control, each LED digital tube lights up for 2MS and then cycles bit by bit. DISP1: MOV R1,#70H ; point to the display data first address MOV R5,#7FH ; Scan control word initial value PLAY: ; MOV P0,#0FFH MOV A,R5 ; scan word and put it into A MOV P0,A SETB P2.7 CLR P2.7 MOV A,@R1; Get display data to A MOV DPTR,#TAB ; Get the segment code table address MOVC A,@A+DPTR; Check the corresponding segment code of the displayed data MOV P0,A; put the segment code into port P0 SETB P2.6 CLR P2.6 MOV A,R5 JB ACC.6, LOOP5; decimal point processing SETB P0.7 SETB P2.6 CLR P2.6 LOOP5: LCALL DL_MS ; Display 2MS INC R1 ; point to the next address MOV A,R5; put back into R5 JNB ACC.3,ENDOUT; When ACC.3=0, the display ends once. RR A ; data in A is rotated left MOV R5,A ; put into R5 AJMP PLAY ; Jump back to PLAY loop ENDOUT: ; MOV P0,#0FFH ; One display ends, P0 port reset ; MOV P2,#0FFH ;P2 port reset RET TAB: ; Define segment code corresponding to 0 1 2 3 4 5 6 7 8 9 - LH DB 3FH,06H,5BH,4FH,66H,6DH,7DH,07H,7FH,6FH,00H,40H,38H,76H DL_MS: MOV R6,#0AH; 2MS delay program, LED display program DL1: MOV R7,#64H DL2: DJNZ R7,DL2 DJNZ R6,DL1 MOV P0,#00H ;Blanking SETB P2.6 CLR P2.6 RET ;****************************************************** ***** ; Single byte hexadecimal to BCD ;****************************************************** ***** HEX2BCD1: MOV B,#064H DIV AB MOV R7,A MOV A,#0AH XCH A,B DIV AB SWAP A ORL A,B RET ;================================================ ; Alarm value TH, TL data conversion ;================================================ ALERT_TL: MOV 79H,#0CH MOV 78H,#0BH MOV A,TEMP_TL MOV R0,#77H MOV B,#064H DIV AB CJNE A,#01H,ALERT_TL1 MOV @R0,A JMP ALERT_TL2 ALERT_TL1: MOV A,#0BH ; Display “-” MOV @R0,A ALERT_TL2: MOV A,#0AH XCH A,B DIV AB DEC R0 MOV @R0,A MOV 7DH,A DEC R0 MOV @R0,B MOV 7EH,B RET ;-------------------------------------------------- ALERT_TH: MOV 79H,#0DH MOV 78H,#0BH MOV A,TEMP_TH MOV R0,#77H MOV B,#064H DIV AB CJNE A,#01H,ALERT_TH1 MOV @R0,A JMP ALERT_TH2 ALERT_TH1: MOV A,#0BH ; Display “-” MOV @R0,A ALERT_TH2: MOV A,#0AH XCH A,B DIV AB DEC R0 MOV @R0,A MOV 7DH,A DEC R0 MOV @R0,B MOV 7EH,B RET ;================================================ ; Alarm value display subroutine ;================================================ ALERT_PLAY: MOV R1,#75H ; point to the first address of display data MOV R5,#7FH ; Scan control word initial value A_PLAY: ; MOV P0,#0FFH MOV A,R5 ; scan word and put it into A MOV P0,A SETB P2.7 CLR P2.7 MOV A,@R1; Get display data to A MOV DPTR,#ALERT_TAB ; Get the segment code table address MOVC A,@A+DPTR; Check the corresponding segment code of the displayed data MOV P0,A; put the segment code into port P0 SETB P2.6 CLR P2.6 LCALL DL_MS1 ; Display 2MS INC R1 ; point to the next address MOV A,R5 JNB ACC.3,ENDOUT1 RR A ; data in A is rotated left MOV R5,A ; put into R5 AJMP A_PLAY ; Jump back to PLAY loop ENDOUT1: ; MOV P0,#0FFH ; One display ends, P0 port reset ; MOV P2,#0FFH ;P2 port reset RET ALERT_TAB: ; Define segment code corresponding to 0 1 2 3 4 5 6 7 8 9 - LH DB 3FH,06H,5BH,4FH,66H,6DH,7DH,07H,7FH,6FH,00H,40H,38H,76H DL_MS1: MOV R6,#0AH; 2MS delay program, LED display program ADL1: MOV R7,#64H ADL2: DJNZ R7,ADL2 DJNZ R6,ADL1 MOV P0,#00H ;Blanking SETB P2.6 CLR P2.6 RET ;================================================ ; Buzzer sounds once subroutine ;P3.7=0, buzzer sounds ;================================================ BEEP_BL: MOV R6,#100 BL2: CALL DEX1 CPL BEEP ; negate beep DJNZ R6,BL2 MOV R5,#10 CALL DELAY RET DEX1: MOV R7,#180 DE2: NOP DJNZ R7,DE2 RET DELAY: ;(R5)*Delay 10MS MOV R6,#50 DEL1: MOV R7,#100 DJNZ R7,$ DJNZ R6,DEL1 DJNZ R5,DELAY RET ;================================================== = END
Previous article:STC89C52 MCU drives CC1101 wireless module receiving C language program
Next article:Summary of some common mistakes that novices make in 51 MCU programming
Recommended ReadingLatest update time:2024-11-16 19:29
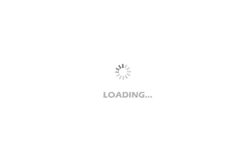
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Single-chip microcomputer technology and application - electronic circuit design, simulation and production (edited by Zhou Runjing)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- National Undergraduate Electronic Design Competition Commonly Used Modules and Related Devices Data Album
- TOP223 chip
- Effects of Improper Use of Derived Clocks on Logic Timing
- Consulting on segment LCD issues
- Oscilloscope measurement of automotive LIN bus signal and waveform analysis
- Southchip SC8905 evaluation board free evaluation, supports charging and discharging bidirectional operation
- Analysis of the design steps of RS-485 bus interface circuit
- How to remotely control a two-wheeled balancing vehicle via the Internet?
- How to get the most out of your low noise amplifier solution?
- JHIHAI APM32E103VET6 Review: External Interrupt (EINT)