#include
//-----------------1602 library function-----------------------
void writecmd(uchar cmd)
{
wait();
RS=0;
RW=0;
__no_operation();
DATA=cmd;
__no_operation();
EN=1;
__no_operation();
__no_operation();
EN=0;
}
void displayonechar(uchar x,uchar y,uchar dda)
{
y&=0x01;
x&=0x0f;
if(y)x|=0x40;
x|=0x80;
writecmd(x);
writedata(dda);
}
Keywords:AVR
Reference address:AVR 1602 LCD character movement
#include
#define uchar unsigned char
#define uint unsigned int
#define uint unsigned int
uchar str1[16]="This is a ASCII";
uchar str2[16]=" Test ";
uchar str2[16]=" Test ";
#include "1602.h"
void port_init()
{
PORTA=0xff;
DDRA=0xff;
PORTB=0xff;
DDRB=0xff;
PORTD=0xff;
DDRD=0xff;
}
{
PORTA=0xff;
DDRA=0xff;
PORTB=0xff;
DDRB=0xff;
PORTD=0xff;
DDRD=0xff;
}
void init()
{
MCUCR=0x0a;
GICR=0xc0;
SREG=0x80;
}
{
MCUCR=0x0a;
GICR=0xc0;
SREG=0x80;
}
void disline(uchar w) //Character movement function
{
uchar i;
for(i=0;i<16;i++)
{
displayonechar(i,1,w); //Characters are displayed on the second line, i.e. letters A--Z
w++;
if(w>90)w=65; //Character display is limited to A--Z
}
delay(500);
}
{
uchar i;
for(i=0;i<16;i++)
{
displayonechar(i,1,w); //Characters are displayed on the second line, i.e. letters A--Z
w++;
if(w>90)w=65; //Character display is limited to A--Z
}
delay(500);
}
void main()
{
uchar w=65;
port_init();
init();
//1602 device initialization
delay(15);
writecmd(0x38);
delay(5);
writecmd(0x38);
delay(5);
writecmd(0x38);
writecmd(0x80);
writecmd(0x01);
writecmd(0x06);
writecmd(0x0c);
delay(500);
//End of initialization
{
uchar w=65;
port_init();
init();
//1602 device initialization
delay(15);
writecmd(0x38);
delay(5);
writecmd(0x38);
delay(5);
writecmd(0x38);
writecmd(0x80);
writecmd(0x01);
writecmd(0x06);
writecmd(0x0c);
delay(500);
//End of initialization
writecmd(0x01);
writecmd(0x0c);
displaychar(0,0,str1);
delay(1000);
writecmd(0x01);
writecmd(0x0c);
displaychar(0,1,str2);
delay(1000);
writecmd(0x01);
writecmd(0x0c);
while(1)
{
disline(w++); //字符移动模式
if(w>90)w=65;
}
}
writecmd(0x0c);
displaychar(0,0,str1);
delay(1000);
writecmd(0x01);
writecmd(0x0c);
displaychar(0,1,str2);
delay(1000);
writecmd(0x01);
writecmd(0x0c);
while(1)
{
disline(w++); //字符移动模式
if(w>90)w=65;
}
}
//-----------------1602 library function-----------------------
#define RS PORTB_Bit0
#define RW PORTB_Bit1
#define EN PORTB_Bit2
#define DATA PORTA
#define busy 0x80
#define RW PORTB_Bit1
#define EN PORTB_Bit2
#define DATA PORTA
#define busy 0x80
[page]
void delay(int k)
{
int i,j;
for(i=0;i
{
for(j=0;j<1140;j++);
}
}
void delay(int k)
{
int i,j;
for(i=0;i
for(j=0;j<1140;j++);
}
}
void wait()
{
uchar val;
DATA=0xff;
RS=0;
RW=1;
__no_operation();
__no_operation();
EN=1;
__no_operation();
__no_operation();
DDRA=0x00;
val=PINA;
while(val&busy)
{
val=PINA;
}
EN=0;
DDRA=0xff;
}
{
uchar val;
DATA=0xff;
RS=0;
RW=1;
__no_operation();
__no_operation();
EN=1;
__no_operation();
__no_operation();
DDRA=0x00;
val=PINA;
while(val&busy)
{
val=PINA;
}
EN=0;
DDRA=0xff;
}
void writecmd(uchar cmd)
{
wait();
RS=0;
RW=0;
__no_operation();
DATA=cmd;
__no_operation();
EN=1;
__no_operation();
__no_operation();
EN=0;
}
void writedata(uchar data)
{
wait();
RS=1;
RW=0;
__no_operation();
DATA=data;
__no_operation();
EN=1;
__no_operation();
__no_operation();
EN=0;
}
{
wait();
RS=1;
RW=0;
__no_operation();
DATA=data;
__no_operation();
EN=1;
__no_operation();
__no_operation();
EN=0;
}
void displayonechar(uchar x,uchar y,uchar dda)
{
y&=0x01;
x&=0x0f;
if(y)x|=0x40;
x|=0x80;
writecmd(x);
writedata(dda);
}
void displaychar(uchar x,uchar y,uchar *p)
{
y&=0x01;
x&=0x0f;
while(*p!='')
{
if(x<=0x0f)
{
displayonechar(x,y,*p);
p++;
x++;
}
}
}
{
y&=0x01;
x&=0x0f;
while(*p!='')
{
if(x<=0x0f)
{
displayonechar(x,y,*p);
p++;
x++;
}
}
}
Previous article:AVR CTC mode waveform output experiment
Next article:AVR EEPROM simple experiment
Recommended ReadingLatest update time:2024-11-16 19:44
Main features of AVR microcontrollers
1) It has its own cheap program memory (FLASH) and non-volatile data memory (EEPROM). These memories can be electrically erased and rewritten many times, making program development experiments more convenient and working more reliable.
2) High speed and low power consumption. Under the same crystal oscillator conditi
[Microcontroller]
AVR MCU Tutorial - Button Status
Today we are going to talk about buttons. There are 4 buttons on the lower right corner of the development board, and there will be a noticeable "click" sound when pressed. How to detect whether the button is pressed? First, the button must be connected to the microcontroller directly or indirectly. Unlike the 4 LEDs
[Microcontroller]
Electronic engineers pass on their experience: classic experience in using AVR microcontrollers
Using better devices only creates a good foundation and possibility for designing and implementing a good system. If you still use and follow the traditional hardware and software design ideas and methods, you can't use AVR well, and you can't even really understand the characteristics and strengths of AVR.
[Analog Electronics]
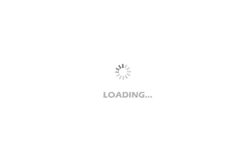
Design of power management system based on AVR
Preface Nowadays, the wide application of aerial robot technology in many fields such as civil and national defense has been increasingly valued by people and has attracted the attention of experts and scholars from various countries. The small rotor robot is based on a model helicopter and is equipped with a
[Microcontroller]
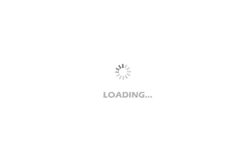
AVR mega16 SPI dual-machine communication example that has been debugged
//ICC-AVR application builder : 2007-7-18 13:01:11
// Target : M16
// Crystal: 7.3728Mhz
// 作者:古欣
// AVR与虚拟仪器 http://www.avrvi.com
// 功能:SPI主机模式,循环发送从1~255
#include iom16v.h
#include macros.h
void port_init(void)
{
PORTA = 0x00;
DDRA = 0x00;
PORTB = 0x00;
DDRB = 0x00;
PORTC = 0x00; //m103 output only
[Microcontroller]
Implementation of AVR single button state machine
#include
#include macros.h
#include "1602.h"
#define key_state_0 0
#define key_state_1 1
#define key_state_2 2
#pragma interrupt_handler timer0:20
flash unsigned char led_7 ={0x3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d,0x07,0x7f,0x6f};
unsigned char time ;
unsigned char time_counter,key_stime_counter;
char po
[Microcontroller]
AVR AD conversion interrupt
System functions Most AVRs have internal AD. This section uses the internal AD of ATMEGA16 as an example to give the AD conversion interrupt program. hardware design AVR main control circuit schematic diagram AD conversion value low, LED control circuit schematic AD conversion value high, LED control c
[Microcontroller]
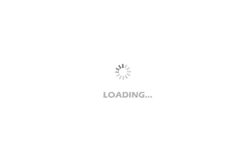
PIC microcontroller LCD1602 display
P IC single chip LCD 1602 displays the website address and phone number ;************************************ PCL EQU 2H ;Define the low byte address of program memory STATUS EQU 3H ;Define the status register address PORTD EQU 8H ;Define the RD port data register address PORTC EQU 7H ;define the address of RC po
[Microcontroller]
- Popular Resources
- Popular amplifiers
-
Single-chip microcomputer C language programming and simulation
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
Recommended Content
Latest Microcontroller Articles
He Limin Column
Microcontroller and Embedded Systems Bible
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
MoreSelected Circuit Diagrams
MorePopular Articles
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
MoreDaily News
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
Guess you like
- tree Command
- What are the categories of edge computing devices?
- How to make a simple calculator using STM8L151K4
- A question about transformers
- Application skills of watchdog reset
- Anyone know what connector this is?
- [Evaluation of the popular N32G45XVL model of the National Technology M4 core] Part 6 Preparation for motor application development
- Design method of adding soft start to DCDC chip
- The stm32 board runs normally online, but does not function normally when it is powered on again
- Four points of detailed description of field effect tube application