Code
#include
sbit led=P2^0;
sbit led2=P2^1;
void DelayUs2x(unsigned char t)
{
while(--t);
}
void DelayMs(unsigned char t)
{
while(t--)
{
//roughly delay 1mS
DelayUs2x(245);
DelayUs2x(245);
}
}
/*The following function is the essence of reverse scanning*/
unsigned char jpsm() //Matrix keyboard reverse scanning
{
unsigned char i=0; //Used to receive key value
P0 = 0x0f; //Detect the lower 4 bits
if(0x0f != P0) //Detect whether the key is pressed
{
DelayMs(10); //Debounce
if(0x0f != P0) //Judge whether the key is really pressed instead of other interference
{
i = P0; //Assign the lower 4 bits to i
P0 = 0xf0; //Check the upper 4 bits
DelayUs2x(5); //Slight delay. This statement can be removed
i = i | P0; //Combine the lower 4 bits and the upper 4 bits into a complete key value
while( 0xf0 != P0 ) //Check if the key is released
{
;
}
return i; //Return the key value
}
}
return 0; //Return 0 if the key is not pressed
}
void main()
{
unsigned char i=0xaa;
while(1)
{
if(126 ==jpsm()) //Judge whether button 1 is pressed
{
/*i <<= 1; //Note: In the Keil compiler, no matter whether it is left shift or right shift, the shifted data is placed in CY
led = CY;*/
i >>=1;
led = CY;
}
}
}
Summary
1. What is a carry digit?
Answer: It is used to store the carry digit.
Previous article:Communication method between single chip microcomputer and human-machine interface
Next article:How many bytes does a pointer occupy?
Recommended ReadingLatest update time:2024-11-17 00:25
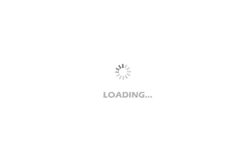
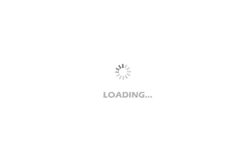
- Popular Resources
- Popular amplifiers
-
Virtualization Technology Practice Guide - High-efficiency and low-cost solutions for small and medium-sized enterprises (Wang Chunhai)
-
Detailed explanation of big data technology system: principles, architecture and practice (Dong Xicheng)
-
Handbook of Multisensor Data Fusion: Theory and Practice
-
Multi-Sensor Data Fusion: An Introduction
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- PT6312 driver Samsung segment screen hardware design driver source code
- Verilog_HDL_Implementation of Common Logic
- TI CC2530 button controls the light on and off
- Could you please tell me what component this is?
- Why does the STM series MCU use SMD T card instead of traditional T card?
- The problem that the main function cannot be run after downloading the program with IAR compiler
- Stick to your job
- [Hua Diao Experience] 07 Building a Xingkong board development environment for VSCode programming
- 【GD32307E-START】Solve the last problem + routine test
- Added multiple ST sensor drivers in MakeCode