Based on the MSP430F149 microcontroller driving the DS2762 read and write operation C language program, it can respond to keystrokes or other signals, read current and voltage, and perform corresponding processing.
Keywords:MSP430F149
Reference address:MSP430F149 microcontroller drives DS2762 to read and write C language program
//#include
#define uchar unsigned char
#define uint unsigned int
uint A,B,C,D,E,F,G,H,I,J; //The macro definition C in io430x14x is commented here, please note
#define IO_OUT P3DIR |= BIT0;
#define IO_INP P3DIR &= ~BIT0;
uint data;
/****************************************************** *****************************
Normalize read and write times
Initial debugging uses the parameter standard=1
// Pause for exactly 'tick' number of ticks = 0.25us
The actual measured A delay is 14Us
B125US
*************************************************** ****************************/
void SetSpeed(uint standard)
{
// Adjust tick values depending on speed
if (standard)
{
// Standard Speed
A = 6 * 4 / 2;
B = 64 * 4 / 2;
C = 60 * 4 / 2;
D = 10 * 4 / 2;
E = 9 * 4 / 2;
F = 55 * 4 / 2;
G = 0 / 2;
H = 480 * 4 / 2;
I = 70 * 4 / 2;
J = 410 * 4 / 2;
}
else
{
// Overdrive Speed
A = 1.5 * 4;
B = 7.5 * 4;
C = 7.5 * 4;
D = 2.5 * 4;
E = 0.75 * 4;
F = 7 * 4;
G = 2.5 * 4;
H = 70 * 4;
I = 8.5 * 4;
J = 40 * 4;
}
}
/****************************************************** *****************************
Delay Program
Note that you need to use an 8M crystal oscillator with a clock period of 125ns
*************************************************** ****************************/
void tickDelay(uint tick) // Implementation is platform specific
{
for(;tick>0;tick--);
}
/****************************************************** *****************************
Host reset pulse
When the receiving result is 0, it indicates that the slave responds
*************************************************** ****************************/
uchar OWTouchReset(void)
{
uchar result;
IO_OUT;
tickDelay(G);
P30 = 0; // Drives DQ low
tickDelay(H);
P30 = 1; // Releases the bus
tickDelay(I);
IO_INP;
result = P3IN & 0X01; // Sample for presence pulse from slave
tickDelay(J); // Complete the reset sequence recovery
return result; // Return sample presence pulse result
}
/****************************************************** *****************************
Like writing a DS2762 write a bit
Send a 1-Wire write bit. Provide 10us recovery time.
*************************************************** ****************************/
void OWWriteBit(uchar bit)
{
if (bit)
{
// Write '1' bit
P30 = 0; // Drives DQ low
tickDelay(A);
P30 = 1; // Releases the bus
tickDelay(B); // Complete the time slot and 10us recovery
}
else
{
// Write '0' bit
P30 = 0; // Drives DQ low
tickDelay(C);
P30 = 1; // Releases the bus
tickDelay(D);
}
}[page]
/****************************************************** *****************************
Reading a bit from the DS2762
Read a bit from the 1-Wire bus and return it. Provide 10us recovery time.
*************************************************** ****************************/
uchar OWReadBit(void)
{
uchar result;
P30 = 0; // Drives DQ low
tickDelay(A);
P30 = 1; // Releases the bus
tickDelay(E);
result = P3IN & 0X01; // Sample the bit value from the slave
tickDelay(F); // Complete the time slot and 10us recovery
return result;
}
/****************************************************** *****************************
Like writing a byte to the DS2762
Send a 1-Wire write. Provide 10us recovery time.
The DS2762 feature sends all commands and data with the low-order bit of the byte first, which is the opposite of most serial communication formats.
*************************************************** ****************************/
void OWWriteByte(uchar data)
{
uchar loop;
// Loop to write each bit in the byte, LS-bit first
for (loop = 0; loop < 8; loop++)
{
OWWriteBit(data & 0x01);
// shift the data byte for the next bit
data >>= 1;
}
}
/****************************************************** *****************************
Read a byte from the DS2762
*************************************************** ****************************/
uchar OWReadByte(void)
{
uchar loop, result=0;
for (loop = 0; loop < 8; loop++)
{
// shift the result to get it ready for the next bit
result >>= 1;
// if result is one, then set MS bit
if (OWReadBit())
result |= 0x80;
}
return result;
}
/****************************************************** *****************************
Send character function
Operations performed according to the time sequence of the paper diagram
It is not clear that sequential reads need to be optimized through testing.
Solution 1: Issue a read command and read one address twice. This should be correct.
Solution 2: Issue a read command and send two addresses to read twice
*************************************************** ****************************/
uint readvoltage(void)
{
uchar j = 1;
unsigned char volhigh,vollow;
while(j) //Check if 2762 responds
{
j = OWTouchReset();
}
OWWriteByte(0xcc); //
OWWriteByte(0x69);
OWWriteByte(0x0c);
vollow = OWReadByte();
volhigh = OWReadByte();
return ((volhigh<<8)|(vollow)); //Merge two bytes
}
/****************************************************** *****************************
Clock initialization
Note that you need to use an 8M crystal oscillator with a clock period of 125ns
*************************************************** ****************************/
void Init_clk(void)
{unsigned char i;
//Clock settings for the time base module
//When the MCU is powered on, the source of the MCLK master clock is selected by default as provided by DCO. The default DCO of the F1 series is 800KHZ.
//ACLK auxiliary clock defaults to XT1, XT1 is usually connected to 32768HZ crystal.
//The default SMCLK sub-clock is DCO, which is also 800KHZ.
//XT2 needs to be manually turned on and tested to see if it is turned on successfully.
BCSCTL1 &= ~(XT2OFF + XTS); //Start XT2 high-speed clock module
BCSCTL2 |= SELM1; //MCLK master clock selects XT2 as the clock source. TX2 input is not divided.
BCSCTL2 &= ~SELS; //SMCLK is selected as DCO as the clock source. (reference)
//Just turned on XT2, it takes a while for XT2 to enter a stable state. So you need to wait and detect the stable state.
//Usually use do...for syntax, which is the program writing method recommended by TI
do
{
IFG1 &=~OFIFG; //Clear OSCFault flag
for(i=0xff;i>0;i--) //Delay and wait for it to start and stabilize
;
}
while((IFG1 & OFIFG) !=0); //Check if the OSCFault flag is 0. If it is 0, it means XT2 is stable.
//Otherwise keep waiting...
}
/****************************************************** *****************************
Main function
Note that you need to use an 8M crystal oscillator with a clock period of 125ns
*************************************************** ****************************/
void main()
{
WDTCTL = WDTPW | WDTHOLD; // Stop watchdog
Init_clk();
SetSpeed(1);
while(1)
{
data = readvoltage();
}
}
Previous article:Chapter 2 Some Data Units (X86 Assembly Tutorial)
Next article:Backtracking algorithm 1
Recommended ReadingLatest update time:2024-11-16 16:47
LCD1602 low-level driver subroutine
Crystal oscillator 11.0592M
/**********************LCD1602 bottom driver program***************************/
/********************************************************************/
//delay: delay xms
void delay(uint xms)
{
uchar i, j;
for(i = 0; i xms; i++)
for(j = 0; j 110; j++);
}
//wait: busy waiting
v
[Microcontroller]
[MCU Notes] OLED controller SSD1306 and driver code
Preface: I have rarely used OLED for a long time, and even if I did, I would refer to the code of experts. Recently, I had to study it again carefully. Write it down so that I don't forget it. The 0.61-inch OLED I have on hand has a resolution of 96*16, which means there are 96 dots horizontally and 16 dots vertic
[Microcontroller]
![[MCU Notes] OLED controller SSD1306 and driver code](https://6.eewimg.cn/news/statics/images/loading.gif)
Using mixed signal high voltage microcontroller to realize LED buck-boost drive circuit
LED Background Knowledge
In recent years, LEDs have become a viable new light source that is no longer just used as a "status indicator" for electronic devices. Technological advances have made LEDs typically more than three times more efficient than incandescent bulbs, and they are also very durable, with a li
[Microcontroller]
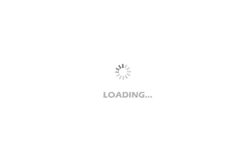
51 MCU IO port driving capability, sink current, pull current, and pull-up resistor selection
The pins of a microcontroller can be controlled by a program to output high or low levels, which can be considered the output voltage of the microcontroller. However, the program cannot control the output current of the microcontroller. The output current of the microcontroller depends largely on the external devices o
[Microcontroller]
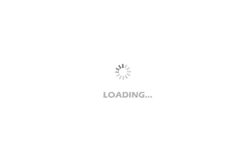
51 MCU drives I2C (24C02) assembly language program
;Program description: Write the contents of 55h~58h inside the MPU to 24c02 via the I2C bus from external interrupt 0, and then read the written contents to
;MPU internal data memory 60H~~63H
SCL EQU P1.1
SDA EQU P1.0
org 0000h
jmp begin
org 0003H
ljmp write
org 0013H
ljmp read
[Microcontroller]
51 MCU driver for proximity switch
Today I made a little thing: the digital tube displays the change value of the proximity switch. The proximity switch is connected to the microcontroller through external interrupt 0; the digital tube reading increases to 50 and then clears to zero; #include reg51.h // Reference the header file of the standard libra
[Microcontroller]
Design of driving circuit for multi-channel PZT based on single chip microcomputer
Abstract: A driving circuit for controlling multiple PZTs (piezoelectric ceramics) based on C8051F005 single-chip microcomputer is designed. The serial data transmission method is adopted, and the characteristics of the new digital-to-analog converter AD5308 with 8-channel DAC output are utilized to greatly simplify
[Industrial Control]
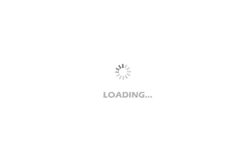
PIC microcontroller drives LED digital tube display program
;*****This program is used to drive the LED digital tube display, and display the numbers 1, 2, 3, 4, 5, 6, 7, 8 on the 8 LED digital tubes in sequence*******
;****http://www.51hei.com The classic program of the microcontroller learning network has been tested. If the common cathode and common anode of the LED
[Microcontroller]
- Popular Resources
- Popular amplifiers
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
Recommended Content
Latest Microcontroller Articles
He Limin Column
Microcontroller and Embedded Systems Bible
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
MoreSelected Circuit Diagrams
MorePopular Articles
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
MoreDaily News
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
Guess you like
- SparkRoad Review (7) - FPGA Serial Port Test
- Disable AD auto-start JLink
- Seeking guidance - stc microcontroller remote upgrade program
- Problems with creating sheet symbols for multi-page schematics
- Zigbee Z-Stack 3.0.1 Modify channels using broadcasting
- SHT31 Review + My Review Summary
- Using AT89S series microcontroller
- 【McQueen Trial】Use IPAD to program McQueen's car
- The STM32 FFT library calculates the amplitude normally, but the phase is different each time. Has anyone encountered this problem?
- EEWORLD University----UCD3138 Analog Front End (AFE) Module