Today I got LPC1766, and compared it with STM32, LPC is more suitable for direct register operation. Today's learning results are as follows (based on Zhou Ligong's LPC1766 board):
/****************************************Copyright (c)***** ***********************************************
** Guangzhou ZLGMCU Development Co., LTD
**--------------File Info-------------------------- -------------------------------------------------- -----
** File name: main.c
** Last modified Date: 2010-09-28
** Last Version: V1.0
** Descriptions: The main() function example template
**
**--- -------------------------------------------------- -------------------------------------------------- -
** Created by: Lan wuqiang
** Created date: 2010-09-28
** Version: V1.00
** Descriptions: Organize templates, add user applications
**
**---------- -------------------------------------------------- -----------------------------------------------
** Modified by:
* * Modified date:
** Version:
** Descriptions:
**
**---------------------------------- -------------------------------------------------- --------------------
** Modified by:
** Modified date:
** Version:
** Descriptions:
**
** Rechecked by:
***** *************************************************** *************************************************** /
#include "LPC17xx.h" /* LPC17xx peripheral registers */
/*************************************************************************************************************
Variables and macro definitions
****************************************************************************************/
#define BEEP (1 << 11) /* P0.11 is connected to the buzzer */
#define KEY1 (LPC_GPIO2->FIOPIN & (1 << 10)) /* P2.10 is connected to KEY1 */
#define KEY2 (LPC_GPIO2->FIOPIN & (1 << 11)) /* P2.11 is connected to KEY2 */
#define KEY3 (LPC_GPIO2->FIOPIN & (1 << 12)) /* P2.12 is connected to KEY3 */
#define KEY4 (LPC_GPIO2->FIOPIN & (1 << 13)) /* P2.13 is connected to KEY4 */
#define BEEPOFF() LPC_GPIO0->FIODIR |= BEEP;LPC_GPIO0->FIOSET |= BEEP /* Buzzer off */
#define BEEPON() LPC_GPIO0->FIODIR |= BEEP;LPC_GPIO0->FIOCLR |= BEEP /* Buzzer on */
#define LED1 (1 << 0) /* P2.0 connects to LED1 */
#define LED2 (1 << 1) /* P2.1 connects to LED2 */
#define LED3 (1 << 2) /* P2.2 connects to LED3 */
#define LED4 (1 << 3) /* P2.3 connects to LED4 */
#define LED1OFF() LPC_GPIO2->FIODIR |= LED1;LPC_GPIO2->FIOSET |= LED1 /* LED1 off */
#define LED1ON() LPC_GPIO2->FIODIR |= LED1;LPC_GPIO2->FIOCLR |= LED1 /* LED1 on */
#define LED2OFF() LPC_GPIO2->FIODIR |= LED2;LPC_GPIO2->FIOSET |= LED2 /* LED2 off */
#define LED2ON() LPC_GPIO2->FIODIR |= LED2;LPC_GPIO2->FIOCLR |= LED 2 /* LED2 on */
#define LED3OFF() LPC_GPIO2->FIODIR |= LED3;LPC_GPIO2->FIOSET |= LED3 /* LED1 off */
#define LED3ON() LPC_GPIO2->FIODIR |= LED3;LPC_GPIO2->FIOCLR |= LED3 /* LED1 on */
#define LED4OFF() LPC_GPIO2->FIODIR |= LED 4;LPC_GPIO2->FIOSET |= LED4 /* LED2 is off */
#define LED4ON() LPC_GPIO2->FIODIR |= LED4;LPC_GPIO2->FIOCLR |= LED4 /* LED2 is on */
/*************************************************************************************************************
** Function name: GPIOInit
** Descriptions: GPIO initialization
** Input parameters: None
** Output parameters: None
** Returned value: None
**************************************************************************************************/
void GPIOInit (void)
{
LPC_PINCON->PINSEL0 &= ~(0x03 << 22); /* Configure P0.11 as GPIO */
LPC_PINCON->PINSEL4 &= 0XF00FFF00; /* Configure P2.0~P2.3 and P2.10~P2.13 as GPIO*/
LPC_GPIO0->FIODIR |= BEEP; /* Configure P0.11 (BEEP) as output 1 */
LPC_GPIO2->FIODIR |= 0X000000FF; /* Configure P2.0~P2.3 as output 1 */
LPC_GPIO2->FIODIR &= 0XFFC3FFFF; /* Configure P2.10~P2.13 as input 0 */
}
/*************************************************************************************************************
** Function name: main
** Descriptions: User program entry function, short JP17, P0.11 pin controls the buzzer,
** Short JP4's KEY1 and P2_10, each time KEY1 is pressed, the buzzer sounds
** input parameters: None
** output parameters: None
** Returned value: None
******************************************************************************************************/
int main (void)
{
SystemInit(); /* System initialization */
GPIOInit();
while (1) {
if (KEY1 == 0) { /* If KEY1 is pressed, the buzzer will sound */
BEEPON();
LED1ON();
} else { /* If KEY1 is released, the buzzer will stop */
BEEPOFF();
LED1OFF();
}
if (KEY2 == 0) { /* If KEY2 is pressed, LED2 will turn on */
LED2ON();
} else { /* If KEY2 is released, LED2 will turn off */
LED2OFF();
}
if (KEY3 == 0) { /* If KEY3 is pressed, LED3 will turn on */
LED3ON();
} else { /* If KEY3 is released, LED3 will turn off */
LED3OFF();
}
if (KEY4 == 0) { /* If KEY4 is pressed, LED4 will turn on */
LED4ON();
} else { /* If KEY4 is released, LED4 will turn off */
LED4OFF();
}
}
}
/****************************************************** *************************************************** ******
End Of File
***************************************** *************************************************** ******************/
Previous article:Stm32 TFT LCD display control study notes
Next article:STM32 LED light based on 3.5 library 2
Recommended ReadingLatest update time:2024-11-16 17:57
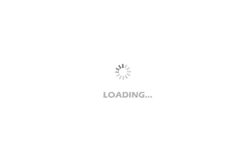
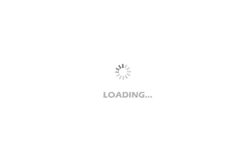
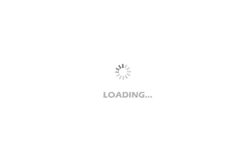
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- What types of anti-wear hydraulic oil are there? What are their characteristics?
- [STM32WB55 Review] What are the differences between dual-core MCUs?
- Where can I buy a multi-channel RF front-end development board with integrated digital attenuator and phase shifter?
- Question: What is the maximum communication rate of AT32F421?
- Why are there so few microcontrollers with integrated 16-bit ADC?
- micropython update: 2020.10
- Reply to receive a gift! It’s Chinese Valentine’s Day, let’s talk about how you and your significant other met?
- Theoretical speed calculation of WiFi protocols
- Does ARM have an instruction to directly obtain the overflow bit?
- MSP430F6638 three system reset