Example of using other MCUs to perform serial port ISP download on STC15 series microcontrollers (equivalent to opening the STC ISP download protocol)
Example of using other MCUs to perform serial port ISP download on STC15 series microcontrollers (equivalent to opening the ISP download protocol of STC microcontrollers)
//In this example, please select Intel's 8058 chip model for compilation in the Keil development environment
//Assume that the operating frequency of the test chip is 11.0592MHz
//Note: When using this code to download the STC15 series MCU, you must execute the Download code first.
//To power on the target chip, otherwise the target chip will not be able to download correctly
#include "reg51.h"
typedef bit BOOL;
typedef unsigned char BYTE;
typedef unsigned short WORD;
typedef unsigned long DWORD;
//Macro and constant definitions
#define FALSE 0
#define TRUE 1
#define LOBYTE(in) ((BYTE)(WORD)(in))
#define HIBYTE(w) ((BYTE)((WORD)(w) >> 8))
#define MINBAUD 2400L
#define MAXBAUD 115200L
#define FOSC 11059200L //main control chip operating frequency
#define BR(n) (65536 - FOSC/4/(n)) // Calculation formula for the baud rate of the main control chip serial port
#define T1MS (65536 - FOSC/1000) // 1ms timing initial value of the main control chip
#define FUSER 24000000L //15 series target chip operating frequency
#define RL(n) (65536 - FUSER/4/(n)) //15 series target chip serial port baud rate calculation formula
//SFR definition
sfr AUXR = 0x8e;
//Variable definitions
BOOL f1ms; //1ms flag
BOOL UartBusy; //Serial port sending busy flag
BOOL UartReceived; //Serial port data reception completed flag
BYTE UartRecvStep; //Serial port data receiving control
BYTE TimeOut; //Serial communication timeout counter
BYTE xdata TxBuffer[256]; //Serial port data sending buffer
BYTE xdata RxBuffer[256]; //Serial port data receiving buffer
char code DEMO[256]; //demonstration code data
// Function declaration
void Initial(void);
void DelayXms(WORD x);
BYTE UartSend(BYTE dat);
void CommInit(void);
void CommSend(BYTE size);
BOOL Download(BYTE *pdat, long size);
//Main function entry
void main(void)
{
while (1)
{
Initial();
if (Download(DEMO, 0x0100))
{
//download successful
P3 = 0xff;
DelayXms(500);
P3 = 0x00;
DelayXms(500);
P3 = 0xff;
DelayXms(500);
P3 = 0x00;
DelayXms(500);
P3 = 0xff;
DelayXms(500);
P3 = 0x00;
DelayXms(500);
P3 = 0xff;
}
else
{
//download failed
P3 = 0xff;
DelayXms(500);
P3 = 0xf3;
DelayXms(500);
P3 = 0xff;
DelayXms(500);
P3 = 0xf3;
DelayXms(500);
P3 = 0xff;
DelayXms(500);
P3 = 0xf3;
DelayXms(500);
P3 = 0xff;
}
}
}
//1ms timer interrupt service routine
void tm0(void) interrupt 1 using 1
{
static BYTE Counter100;
f1ms = TRUE;
if (Counter100-- == 0)
{
Counter100 = 100;
if (TimeOut) TimeOut--;
}
}
//Serial port interrupt service routine
void uart(void) interrupt 4 using 1
{
static WORD RecvSum;
static BYTE RecvIndex;
static BYTE RecvCount;
BITE that;
if (TI)
{
IF = 0;
UartBusy = FALSE;
}
if (RI)
{
RI = 0;
dat = SBUF;
switch (UartRecvStep)
{
case 1:
if (dat != 0xb9) goto L_CheckFirst;
UartRecvStep++;
break;
case 2:
if (dat != 0x68) goto L_CheckFirst;
UartRecvStep++;
break;
case 3:
if (dat != 0x00) goto L_CheckFirst;
UartRecvStep++;
break;
case 4:
RecvSum = 0x68 + dat;
RecvCount = dat - 6;
RecvIndex = 0;
UartRecvStep++;
break;
case 5:
RecvSum += that;
RxBuffer[RecvIndex++] = dat;
if (RecvIndex == RecvCount) UartRecvStep++;
break;
case 6:
if (dat != HIBYTE(RecvSum)) goto L_CheckFirst;
UartRecvStep++;
break;
case 7:
if (dat != LOBYTE(RecvSum)) goto L_CheckFirst;
UartRecvStep++;
break;
case 8:
if (dat != 0x16) goto L_CheckFirst;
UartReceived = TRUE;
UartRecvStep++;
break;
L_CheckFirst:
case 0:
default:
CommInit();
UartRecvStep = (dat == 0x46 ? 1 : 0);
break;
}
}
}
//system initialization
void Initial(void)
{
UartBusy = FALSE;
SCON = 0xd0; //Serial port data mode must be 8-bit data + 1-bit even check
AUXR = 0xc0;
TMOD = 0x00;
TH0 = HIBYTE(T1MS);
TL0 = LOBYTE(T1MS);
TR0 = 1;
TH1 = HIBYTE(BR(MINBAUD));
TL1 = LOBYTE(BR(MINBAUD));
TR1 = 1;
ET0 = 1;
EN = 1;
EA = 1;
}
//Xms delay program
void DelayXms(WORD x)
{
do
{
f1ms = FALSE;
while (!f1ms);
} while (x--);
}
//Serial data sending program
BYTE UartSend(BYTE dat)
{
while (UartBusy);
UartBusy = TRUE;
ACC = that;
TB8 = P;
SBUF = ACC;
return that;
}
//Serial communication initialization
void CommInit(void)
{
UartRecvStep = 0;
TimeOut = 20;
UartReceived = FALSE;
}
//Send serial communication data packet
void CommSend(BYTE size)
{
WORD sum;
BYTE i;
UartSend(0x46);
UartSend(0xb9);
UartSend(0x6a);
UartSend(0x00);
sum = size + 6 + 0x6a;
UartSend(size + 6);
for (i=0; i
{
sum += UartSend(TxBuffer[i]);
}
UartSend(HIBYTE(sum));
UartSend(LOBYTE(sum));
UartSend(0x16);
while (UartBusy);
CommInit();
}
//Data download program for STC15 series chips
BOOL Download(BYTE *pdat, long size)
{
BYTE angry;
BYTE cnt;
WORD addr;
//Handshake
CommInit();
while (1)
{
if (UartRecvStep == 0)
{
UartSend(0x7f);
DelayXms(10);
}
if (UartReceived)
{
arg = RxBuffer[4];
if (RxBuffer[0] == 0x50) break;
return FALSE;
}
}
//Setting parameters
TxBuffer[0] = 0x01;
TxBuffer[1] = arg;
TxBuffer[2] = 0x40;
TxBuffer[3] = HIBYTE(RL(MAXBAUD));
TxBuffer[4] = LOBYTE(RL(MAXBAUD));
TxBuffer[5] = 0x00;
TxBuffer[6] = 0x00;
TxBuffer[7] = 0xc3;
CommSend(8);
while (1)
{
if (TimeOut == 0) return FALSE;
if (UartReceived)
{
if (RxBuffer[0] == 0x01) break;
return FALSE;
}
}
//Prepare
TH1 = HIBYTE(BR(MAXBAUD));
TL1 = LOBYTE(BR(MAXBAUD));
DelayXms(10);
TxBuffer[0] = 0x05;
CommSend(1);
while (1)
{
if (TimeOut == 0) return FALSE;
if (UartReceived)
{
if (RxBuffer[0] == 0x05) break;
return FALSE;
}
}
// Erase
DelayXms(10);
TxBuffer[0] = 0x03;
TxBuffer[1] = 0x00;
CommSend(2);
TimeOut = 100;
while (1)
{
if (TimeOut == 0) return FALSE;
if (UartReceived)
{
if (RxBuffer[0] == 0x03) break;
return FALSE;
}
}
//Write user code
DelayXms(10);
addr = 0;
TxBuffer[0] = 0x22;
while (addr < size)
{
TxBuffer[1] = HIBYTE(addr);
TxBuffer[2] = LOBYTE(addr);
cnt = 0;
while (addr < size)
{
TxBuffer[cnt+3] = pdat[addr];
addr++;
cnt++;
if (cnt >= 128) break;
}
CommSend(cnt + 3);
while (1)
{
if (TimeOut == 0) return FALSE;
if (UartReceived)
{
if ((RxBuffer[0] == 0x02) && (RxBuffer[1] == 'T')) break;
return FALSE;
}
}
TxBuffer[0] = 0x02;
}
//Write hardware options (if you do not need to modify hardware options, this step can be skipped)
DelayXms(10);
for (cnt=0; cnt<128; cnt++)
{
TxBuffer[cnt] = 0xff;
}
TxBuffer[0] = 0x04;
TxBuffer[1] = 0x00;
TxBuffer[2] = 0x00;
TxBuffer[34] = 0xfd;
TxBuffer[62] = arg;
TxBuffer[63] = 0x7f;
TxBuffer[64] = 0xf7;
TxBuffer[65] = 0x7b;
TxBuffer[66] = 0x1f;
CommSend(67);
while (1)
{
if (TimeOut == 0) return FALSE;
if (UartReceived)
{
if ((RxBuffer[0] == 0x04) && (RxBuffer[1] == 'T')) break;
return FALSE;
}
}
//Download completed
return TRUE;
}
char code DEMO[256] =
{
0x02,0x00,0x5E,0x12,0x00,0x4B,0x75,0xB0,
0xEF,0x12,0x00,0x2C,0x75,0xB0,0xDF,0x12,
0x00,0x2C,0x75,0xB0,0xFE,0x12,0x00,0x2C,
0x75,0xB0,0xFD,0x12,0x00,0x2C,0x75,0xB0,
0xFB,0x12,0x00,0x2C,0x75,0xB0,0xF7,0x12,
0x00,0x2C,0x80,0xDA,0xE4,0xFF,0xFE,0xE4,
0xFD,0xFC,0x0D,0xBD,0x00,0x01,0x0C,0xBC,
0x01,0xF8,0xBD,0xF4,0xF5,0x0F,0xBF,0x00,
0x01,0x0E,0xBE,0x03,0xEA,0xBF,0xE8,0xE7,
0x02,0x00,0x4B,0x75,0x80,0xFF,0x75,0x90,
0xFF,0x75,0xA0,0xFF,0x75,0xB0,0xFF,0x75,
0xC0,0xFF,0x75,0xC8,0xFF,0x22,0x78,0x7F,
0xE4,0xF6,0xD8,0xFD,0x75,0x81,0x07,0x02,
0x00,0x03,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00,
};
Previous article:LCD12864 with Chinese character library graphic display-MCU program
Next article:STC MCU PCA generates PWM program
Recommended ReadingLatest update time:2024-11-16 15:01
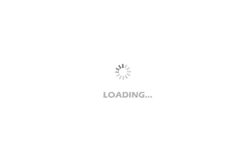
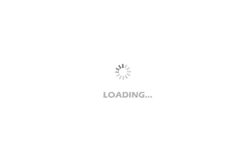
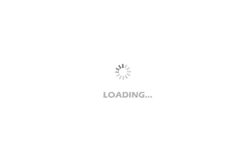
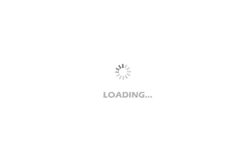
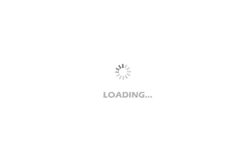
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Is there anyone who is good at inverter power supply?
- EEWORLD University Hall----Live Replay: ADI - Noise Generation in Switching Power Supplies and How to Reduce Power Supply Noise
- Battery power collection
- How to set up PCB routing like this?
- Dear bachelors, do you cook your dinner yourself or eat out?
- Share an ARM OS project package (based on STM32F103 on-chip resources).
- MSP-EXP430F5529LP Development Board 005-PWM Library Function + Clock Configuration
- EEWORLD University ---- RF Circuit Basics
- EEWORLD University ---- ARM Programming
- C6678 shared memory problem