/*****************************************************************************
Read LCD status word to STA
Function: Used to judge busy/idle before writing data or commands to the LCD
******************************************************************************/
void read_sta(void)
{
P4DIR = 0x00; //P4 data input
P3OUT = P3_2_DATAIO; //4245 ( x1 ) data transmission direction selection: from LCD to 430
P3OUT = P3_1_CD; // Command mode
P3OUT &= ~P3_0_RD; //read
STA = P4IN ; // Read back status
P3OUT = P3_0_RD; //cancel read
P4DIR = 0xff; //P4 port is set to data output mode
P3OUT &= ~P3_2_DATAIO; //4245 ( x1 ) data transmission direction selection: from 430 to LCD
}
/*****************************************************************************
Judge the status bit S1, S0 function ( read and write instructions and read and write data status )
******************************************************************************/
void ST1( void )
{
do
{
read_sta();
}
while( (STA & 0x03) != 0x03 );
}
/*****************************************************************************
Status bit S2 function ( data automatic reading status )
******************************************************************************/
void ST2( void )
{
do
{
read_sta();
}
while( (STA & 0x04) != 0x04);
}
/*****************************************************************************
- Status bit S3 function ( data automatic write status ) -
******************************************************************************/
void ST3( void )
{
do
{
read_sta();
}
while( (STA & 0x08) != 0x08 );
}
/*****************************************************************************
Write data byte
******************************************************************************/
void write_data(unsigned char Byte )
{
ST1();
P4OUT = Byte;
P3OUT &= ~P3_1_CD; // Data mode
P2OUT &= ~P2_7_WR ; // write
P2OUT = P2_7_WR ;
}
/*****************************************************************************
Automatically write data bytes
******************************************************************************/
void autowrite_data(unsigned char Byte )
{
P4OUT = Byte;
P3OUT &= ~P3_1_CD; // Data mode
P2OUT &= ~P2_7_WR ; // write
P2OUT = P2_7_WR ;
}
/*****************************************************************************
Write command word
******************************************************************************/
void write_cmd(unsigned char cmd )
{
ST1();
P4OUT = cmd ;
P3OUT = P3_1_CD ; // Command mode
P2OUT &= ~P2_7_WR ; // write
P2OUT = P2_7_WR ;
}
/*****************************************************************************
Writing commands with only one argument
******************************************************************************/
void write_onepara(unsigned char dat1,unsigned char command )
{
write_data( dat1 );
write_cmd( command );
}
/*****************************************************************************
Write a command with two parameters
******************************************************************************/
void write_doublepara(unsigned char dat1,unsigned char dat2,unsigned char command )
{
write_data( dat1 );
write_data( dat2 );
write_cmd( command );
}
/*****************************************************************************
Function to clear the display buffer
******************************************************************************/
void CLEAR_RAM( void )
{
int i;
write_doublepara( 0x00,0x00,0x24 ); /* Set the first address of display RAM */
write_cmd( 0xb0 ); /* Set automatic write mode */
for(i=0;i<8200;i++) /* Clear 8K memory */
{
ST3(); /* Judge status bit S3 */
write_data(0x00); /* write data */
}
write_cmd( 0xb2 ); /* Set automatic write end instruction */
}
/*****************************************************************************
Some initial setting functions for the LCD screen
******************************************************************************/
void LcdIni( void )
{
write_doublepara(0x00,0x00,0x40); /* Set the first address of the text display area */
write_doublepara(0x28,0x00,0x41); /* Set the text display area width */
write_doublepara(0x03,0x00,0x22); /* Set CGRAM bias address */
write_doublepara(0x00,0x08,0x42); /* Set the first address of the graphics display area */
write_doublepara(0x1e,0x00,0x43); /* Set the width of the graphics display area */
// write_cmd(0xa2); /* Cursor shape setting */
// write_doublepara(0x08,0x08,0x21); /* Set the cursor position */
write_cmd(0x80); /* Display mode settings logical " or " synthesis , internal character generator is effective */
write_cmd(0x98); /* Display switch setting: turn off text and turn on graphic display */
CLEAR_RAM();
}
Previous article:Detailed explanation of DS18B20 temperature sensor
Next article:Timed interruption controls the on and off of the light
Recommended ReadingLatest update time:2024-11-16 15:46
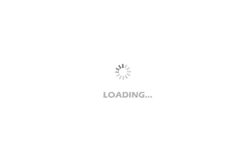
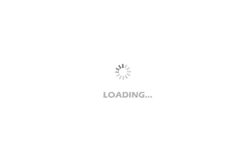
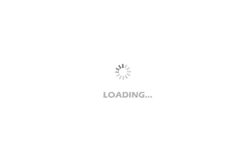
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications