////////////////////////////////////////////////////////////////////////////////////////
Function implemented: Complete the test of independent keys. When one of the twenty keys, key5-key20, is pressed, a
specific value will be displayed on the first digital tube of the development board. The displayed value is
defined as follows: when key5 is pressed, 0 is displayed; when key6 is pressed, 2 is displayed; and so on,
until the corresponding value is displayed when the corresponding value is pressed.
Experiment board model: BS-XYD-C52
Experiment name: Static digital tube
Author: TabLee
Date of writing: 2014-3-21
////////////////////////////////////////////////////////////////////////////////
#include
#include
#define uchar unsigned char //Define unsigned char as uchar
#define uint unsigned int
sbit Duan = P2^6; //Define the segment selection enable terminal of the digital tube
sbit Wei = P2^7; //Define the bit selection enable terminal of the digital tube
#define Digital_tube_Wei_Enable Wei=1; //Turn on the bit select enable terminal for controlling the digital tube
#define Digital_tube_Wei_Disable Wei=0; //Turn off the bit select enable terminal for controlling the digital tube
#define Digital_tube_Duan_Enable Duan=1; //Turn on the segment selection enable terminal for controlling the digital tube
#define Digital_tube_Duan_Disable Duan=0; //Turn off the segment selection enable terminal for controlling the digital tube
#define Digital_tube_Duan P0 //Define the digital tube data port
#define KEY_DOWN 0
#define Keyport P3
uchar code Dis_table[]= //Convert BCD code into array of digital tube scan code
{0x3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d,0x07,0x7f,0x6f,0x77,0x7c,0x39,0x5e,
0x79,0x71};
uchar code Dis_Position[]= //Define array of digital tube position selection
{0xfe,0xfd,0xfb,0xf7,0xef,0xdf};
///
...
No
precautions: This experiment realizes millisecond delay under the premise that the crystal oscillator used is 12M. This function is completed in
the form , so if the frequency of the crystal oscillator is changed, please make corresponding changes
/ ... ////////////////////////////////////////////////////////////////////////////////
Function name: One_DigitalTube_display
Function function: Complete the specified display of the digital tube on the experimental board, that is, display a specific number on a specific digital tube, than
displaying 0 on the first digital tube
Parameter introduction: uData: BCD code array of the number to be displayed
uNumber: Select which digital tube to display, that is, let a specific digital tube display
Return value: None
Notes: The digital tube on the experimental board is a common cathode digital tube. If you use a common anode digital tube, be careful
not to get it reversed
/ ...
void One_DigitalTube_display(uchar uData,uchar uNumber)
{
Digital_tube_Duan=Dis_Position[uNumber]; //Light up a specific digital tube
Digital_tube_Wei_Enable; //Enable the bit selection of the digital tube
Digital_tube_Wei_Disable; //Disable the bit selection of the digital tube
DelayMs(5); //Adjust the timing to achieve stable display
Digital_tube_Duan_Enable; //Enable the segment selection of the digital tube
Digital_tube_Duan=Dis_table[uData]; //Enter the value to be displayed
Digital_tube_Duan_Disable; //Disable the segment selection of the digital tube
}
///
...
void Scan_Keyboard()
{
uchar cTemp_Value;
//Scan the first column of the matrix keyboardKeyport
=0xef; //Assign a specific value to the keyboard port to achieve the following detectioncTemp_Value
=Keyport; //Assign the keyboard port value to a temporary variableif
(cTemp_Value!=0xef)
{
DelayMs(10); //Eliminate jitter, that is, eliminate interferencecTemp_Value
=Keyport; //Reassign to temporary valueif
(cTemp_Value!=0xef) //Check againwhile
((Keyport&0x0F)!=0x0F) //Judge again whether a key is pressed
{
switch(cTemp_Value)
{
case 0xee:
One_DigitalTube_display(0,0);break; //The fifth key is pressed, display 0
case 0xed:
One_DigitalTube_display(4,0);break; //The ninth key is pressed, display 4
case 0xeb:
One_DigitalTube_display(8,0);break; //The thirteenth button is pressed, and 8 is displayed
case 0xe7:
One_DigitalTube_display(12,0);break; //The seventeenth button is pressed, and C is displayed
}
}
}
//Scan the second column of the matrix keyboard
Keyport=0xdf; //Assign a specific value to the keyboard port to achieve the following detection
cTemp_Value=Keyport; //Assign the keyboard port value to a temporary variable
if(cTemp_Value!=0xdf)
{
DelayMs(10); //Eliminate jitter, that is, eliminate interference
cTemp_Value=Keyport; //Reassign to the temporary value
if(cTemp_Value!=0xdf) //Check again
while((Keyport&0x0F)!=0x0F) //Judge again whether a button is pressed
{
switch(cTemp_Value)
{
case 0xde:
One_DigitalTube_display(1,0);break; //The sixth button is pressed, and 1 is displayed
case 0xdd:
One_DigitalTube_display(5,0);break; //The tenth key is pressed, and 5 is displayed
case 0xdb:
One_DigitalTube_display(9,0);break; //The fourteenth key is pressed, and 9 is displayed
case 0xd7:
One_DigitalTube_display(13,0);break; //The eighteenth key is pressed, and D is displayed
}
}
}
//Scan the third column of the matrix
keyboardKeyport=0xbf; //Assign a specific value to the keyboard port to achieve the following detection
cTemp_Value=Keyport; //Assign the keyboard port value to a temporary variableif
(cTemp_Value!=0xbf)
{
DelayMs(10); //Eliminate jitter, that is, eliminate interferencecTemp_Value
=Keyport; //Reassign to the temporary valueif
(cTemp_Value!=0xbf) //Check againwhile
((Keyport&0x0F)!=0x0F) //Judge whether a key is pressed again
{
switch(cTemp_Value)
{
case 0xbe:
One_DigitalTube_display(2,0);break; //The seventh button is pressed, and the display shows 2
case 0xbd:
One_DigitalTube_display(6,0);break; //The eleventh button is pressed, and the display shows 6
case 0xbb:
One_DigitalTube_display(10,0);break; //The fifteenth button is pressed, and the display shows A
case 0xb7:
One_DigitalTube_display(14,0);break; //The nineteenth button is pressed, and the display shows E
}
}
}
//Scan the fourth column of the matrix keyboard
Keyport=0x7f; //Assign specific values to the keyboard port to implement subsequent detection
cTemp_Value=Keyport; //Assign the keyboard port value to the temporary variable
if(cTemp_Value!=0x7f)
{
DelayMs(10); //Eliminate jitter, that is, eliminate interference
cTemp_Value=Keyport; //Reassign to the temporary value
if(cTemp_Value!=0x7f) //Check again
while((Keyport&0x0F)!=0x0F) //Judge again whether a key is pressed
{
switch(cTemp_Value)
{
case 0x7e:
One_DigitalTube_display(3,0);break; //The eighth key is pressed, and 3 is displayed
case 0x7d:
One_DigitalTube_display(7,0);break; //The twelfth key is pressed, and 7 is displayed
case 0x7b:
One_DigitalTube_display(11,0);break; //The sixteenth key is pressed, and B is displayed
case 0x77:
One_DigitalTube_display(15,0);break; //The 20th button is pressed, and F is displayed
}
}
}
}
/// ...
Function name: mainFunction
function: Continuously scan the keyboard in a loop. When a key is pressed, the corresponding value will be displayed on the digital tube.
Parameter introduction: None
Return value: None
Notes: None
/ ...
void main()
{
DelayMs(50);
while(1)
{
Scan_Keyboard(); //Call keyboard scanning function
}
}
Previous article:Modular program of LCD12232 liquid crystal
Next article:The difference between open-drain output and push-pull output of microcontroller I/O port
Recommended ReadingLatest update time:2024-11-16 16:35
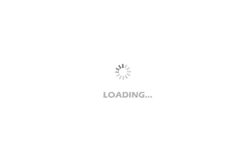
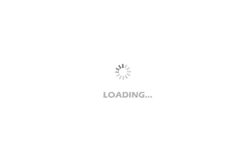
![[51 microcontroller STC89C52] Timer (interrupt) controls LED](https://6.eewimg.cn/news/statics/images/loading.gif)
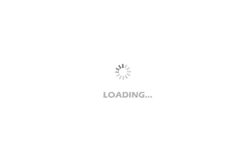
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Traffic Light Control System Based on Fuzzy Control (Single-Chip Microcomputer Implementation)
-
Principles and Applications of Single Chip Microcomputers (Second Edition) (Wanlong)
-
MCU Principles and C51 Programming Tutorial (2nd Edition)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- LTC2325-16 Sampling Issues
- Design considerations for single-chip microcomputer key scanning program
- Free application: Domestic FPGA Gaoyunjia Little Bee Family GW1N Series Development Board
- Taking stock of the college entrance examination experience of Internet tycoons! How many points did you get in the exam?
- Without American EDA software, we can’t make chips?
- 【Share】Flash management tools: FAL (Flash Abstraction Layer) library
- [Awards awarded] Grab the post! Download the TWS headset white paper, write a wonderful review, and win a JD card!
- Constant voltage circuit and constant current circuit composed of operational amplifier and triode
- [RVB2601 Creative Application Development] Record the startup process of the hello world system
- How to initialize the key port of the power button in C language