This assembly program is only suitable for the connection between a single DS18B20 and a 51 microcontroller, and the crystal oscillator is about 12MHZ
DQ: DS18B20 data bus pin
FLAG1: flag bit, when "1" means that DS18B20 is detected
TEMPER_NUM: save the read temperature data
TEMPER_LEQU36H
TEMPER_HEQU35H
DQBITP1.7
; DS18B20 initialization assembly program
; //********************************************//
INIT_1820:
SETBDQ
NOP
CLRDQ
MOVR0,#06BH
TSR1:
DJNZR0,TSR1; Delay
SETBDQ
MOVR0,#25H
TSR2:
JNBDQ,TSR3
DJNZR0,TSR2
LJMPTSR4; Delay
TSR3:
SETBFLAG1; Set the flag to indicate that DS1820 exists
LJMPTSR5
TSR4:
CLRFLAG1; Clear the flag to indicate that DS1820 does not exist
LJMPTSR7
TSR5:
MOVR0,#06BH
TSR6:
DJNZR0,TSR6; Delay
TSR7:
SETBDQ
RET
;//*********************************************//
; Rewrite the setting value of DS18B20 temporary memory
; //********************************************//
RE_CONFIG:
JBFLAG1,RE_CONFIG1; If DS18B20 exists, go to RE_CONFIG1
RET
RE_CONFIG1:
MOVA,#0CCH; Send SKIP ROM command
LCALLWRITE_1820
MOVA,#4EH; Send write temporary memory command
LCALLWRITE_1820
MOVA,#00H; Write 00H to TH (alarm upper limit)
LCALLWRITE_1820
MOVA,#00H; Write 00H to TL (alarm lower limit)
LCALLWRITE_1820
MOVA,#1FH; Select 9-bit temperature resolution
LCALLWRITE_1820
RET
;//********************************************//
; Read the converted temperature value
; //********************************************//
GET_TEMPER:
SETBDQ; Timing entry
LCALLINIT_1820
JBFLAG1,TSS2
RET; If DS18B20 does not exist, return to
TSS2:
MOVA,#0CCH; Skip ROM matching
LCALLWRITE_1820
MOVA,#44H; Issue temperature conversion command
LCALLWRITE_1820
LCALLINIT_1820
MOVA,#0CCH; Skip ROM matching
LCALLWRITE_1820
MOVA,#0BEH; Issue a temperature read command
LCALLWRITE_1820
LCALLREAD_1820
MOVTEMPER_NUM,A; Save the read temperature data
RET
;//************************************************//
; Read DS18B20 program, read one byte of data from DS18B20
; //********************************************//
READ_1820:
MOVR2,#8
RE1:
CLRC
SETBDQ
NOP
NOP
CLRDQ
NOP
NOP
NOP
SETBDQ
MOVR3,#7
DJNZR3,$
MOVC,DQ
MOVR3,#23
DJNZR3,$
RRCA
DJNZR2,RE1
RET
;//************************************************//
; Write the program for DS18B20
; //********************************************//
WRITE_1820:
MOVR2,#8
CLRC
WR1:
CLRDQ
MOVR3,#6
DJNZR3,$
RRCA
MOVDQ,C
MOVR3,#23
DJNZR3,$
SETBDQ
NOP
DJNZR2,WR1
SETBDQ
RET
; //************************************************//
; Read DS18B20 program, read two bytes of temperature data from DS18B20
; //********************************************//
READ_18200:
MOVR4,#2; Read the high and low bits of temperature from DS18B20
MOVR1,#36H; Store the low bit in 36H (TEMPER_L) and the high bit in 35H (TEMPER_H)
RE00:
MOVR2,#8
RE01:
CLRC
SETBDQ
NOP
NOP
CLRDQ
NOP
NOP
NOP
SETBDQ
MOVR3,#7
DJNZR3,$
MOVC,DQ
MOVR3,#23
DJNZR3,$
RRCA
DJNZR2,RE01
MOV@R1,A
DECR1
DJNZR4,RE00
RET
;//************************************************//
; Convert the temperature data read from DS18B20
; //********************************************//
TEMPER_COV:
MOVA,#0F0H
ANLA,TEMPER_L; Discard the four decimal places of the low temperature
SWAPA
MOVTEMPER_NUM,A
MOVA,TEMPER_L
JNBACC.3,TEMPER_COV1; Round off the temperature value
INCTEMPER_NUM
TEMPER_COV1:
MOVA,TEMPER_H
ANLA,#07H
SWAPA
ORLA,TEMPER_NUM
MOVTEMPER_NUM,A; Save the converted temperature data
LCALLBIN_BCD
RET
;//************************************************//
; Convert the hexadecimal temperature data into compressed BCD code
; //********************************************//
BIN_BCD:
MOVDPTR,#TEMP_TAB
MOVA,TEMPER_NUM
MOVCA,@A+DPTR
MOVTEMPER_NUM,A
RET[page]
TEMP_TAB:
DB00H,01H,02H,03H,04H,05H,06H,07H
DB08H,09H,10H,11H,12H,13H,14H,15H
DB16H,17H,18H,19H,20H,21H,22H,23H
DB24H,25H ,26H,27H,28H,29H,30H,31H
DB32H,33H,34H,35H,36H,37H,38H,39H
DB40H,41H,42H,43H,44H,45H,46H,47H
DB48H,49H,50H,51H, 52H,53H,54H,55H
DB56H,57H,58H,59H,60H,61H,62H,63H
DB64H,65H,66H,67H,68H,69H,70H
;//************************ ************************//
The following is a ds18b20 assembler program
; *************************************
FLAG1 BIT F0 ;DS18B20 exists flag bit
DQ BIT P1.7
TEMPER_L EQU 29H
TEMPER_H EQU 28H
A_BIT EQU 35H
B_BIT EQU 36H
;************ds18b20 assembler program starts********************
ORG 0000H
AJMP MAIN
ORG 0100H
;**************Main program starts************
MAIN:
LCALL INIT_18B20
;LCALL RE_CONFIG
LCALL GET_TEMPER
AJMP CHANGE
;**********DS18B20 reset procedure********************
INIT_18B20: SETB DQ
NOP
CLR DQ
MOV R0,#0FBH
TSR1: DJNZ R0,TSR1 ;Delay
SETB DQ
MOV R0,#25H
TSR2: JNB DQ ,TSR3
DJNZ R0,TSR2
TSR3: SETB FLAG1 ;Set the flag, indicating that DS18B20 exists
CLR P2.0 ;Diode indication
AJMP TSR5
TSR4: CLR FLAG1
LJMP TSR7
TSR5: MOV R0,#06BH
TSR6: DJNZ R0,TSR6
TSR7:SETB DQ ;Indicates that it does not exist
RET
;********************Set DS18B20 register setting value**************
;RE_CONFIG:
;JB FLAG1,RE_CONFIG1
;RET
;RE_CONFIG1: MOV A,#0CCH ; Skip ROM command
; LCALL WRITE_18B20
;MOV A,#4EH
;LCALL WRITE_18B20 ; Write register command
; MOV A,#00H ; Write 00H in the upper limit of alarm
; LCALL WRITE_18B20
;MOV A,#00H ; Write 00H in the lower limit of alarm
; LCALL WRITE_18B20
;MOV A,#1FH ; Select nine-bit temperature resolution
; LCALL WRITE_18B20
; RET
;********************Read the converted temperature value****************
GET_TEMPER:
SETB DQ
LCALL INIT_18B20
JB FLAG1,TSS2
RET ; Return to TSS2 if it does not exist
: MOV A,#0CCH ; Skip ROM
LCALL WRITE_18B20
MOV A,#44H ; Issue temperature conversion command
LCALL WRITE_18B20
LCALL DISPLAY ; Delay
LCALL INIT_18B20
MOV A,#0CCH ; Skip ROM
LCALL WRITE_18B20
MOV A,#0BEH ; Issue a temperature read command
LCALL WRITE_18B20
LCALL READ2_18B20 ; Read two bytes of temperature
RET
; ****************** Write ds18b20 assembly program **********
WRITE_18B20:
MOV R2,#8
CLR C
WR1:
CLR DQ
MOV R3,#6
DJNZ R3,$
RRC A
MOV DQ,C
MOV R3,#23
DJNZ R3,$
SETB DQ
NOP
DJNZ R2,WR1
SETB DQ
RET
;***********Read 18B20 program, read two bytes of temperature*********
READ2_18B20:
MOV R4,#2 ;The low bit is 29H, the high bit is 28H
MOV R1,#29H
RE00: MOV R2,#8
RE01: CLR C
SETB C
NOP
NOP
CLR DQ
NOP
NOP
NOP
SETB DQ
MOV R3,#7
DJNZ R3,$
MOV C,DQ
MOV R3,#23
DJNZ R3,$
RRC A
DJNZ R2,RE01
MOV @R1,A
DEC R1
DJNZ R4,RE00
RET
;************Read the temperature and convert it into data**************
CHANGE: MOV A,29H
MOV C,28H.0 ; Move the lowest bit in 28H into C
RRC A
MOV C,28H.1
RRC A
MOV C,28H.2
RRC A
MOV C,28H.3
RRC A
MOV 29H,A
; setb p2.0
LCALL DISPLAY ; Call the digital tube display subroutine
; setb P2.0
LJMP MAIN
;*******************DISPLAY******
DISPLAY: mov a,29H;Convert the hexadecimal number in 29H to decimal
mov b,#10 ;Decimal/10=decimal
div ab
mov b_bit,a ;Tens digit is in a
mov a_bit,b ;Ones digit is in b
mov dptr,#TAB ;Specify the start address of table lookup
mov r0,#4
dpl1: mov r1,#250 ;Display 1000 times
dplop: mov a,a_bit ;Get the ones digit
MOVC A,@A+DPTR ;Check the 7-segment code of the ones digit
mov p0,a ;Send the 7-segment code of the ones digit
clr p2.5;Open the ones digit to display
acall d1ms ;Display 1ms
setb p2.5
mov a,b_bit ;Get the tens digit
MOVC A,@A+DPTR ; Check the 7-segment code of the ten-digit
mov p0,a ; Send the 7-segment code of the ten-digit
clr p2.4 ; Open the ten-digit display
acall d1ms ; Display 1ms
setb p2.4
djnz r1,dplop ; 100 times endless loop
djnz r0,dpl1 ; 4 100 times endless loop
ret
;***********************************
D1MS: MOV R7,#80 ;1MS delay (calculated as 12MHZ)
DJNZ R7,$
RET
;*****************************
TAB: DB 0C0H,0F9H,0A4H,0B0H,99H,92H,82H,0F8H,80H,90H
Previous article:Introduction to the Principle of 51 Single Chip Microcomputer
Next article:Range-auto-switching frequency meter based on AT89C51 single-chip microcomputer
Recommended ReadingLatest update time:2024-11-16 23:42
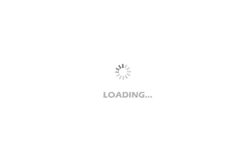
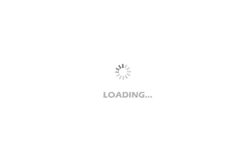
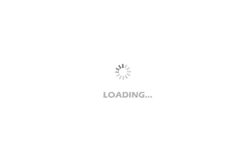
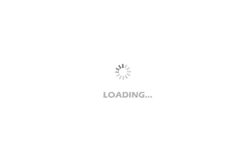
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Fundamentals and Applications of Single Chip Microcomputers (Edited by Zhang Liguang and Chen Zhongxiao)
-
Single-chip microcomputer technology and application - electronic circuit design, simulation and production (edited by Zhou Runjing)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Welding problem or SI problem? You decide!
- Issues that should be paid attention to when using CMOS circuits
- Is Python worth learning? Is it easy to learn?
- TMP112 read timing is incorrect, can produce results, 9 more clocks
- High salary recruitment of hardware maintenance engineers
- Audio Power Amplifier Design Handbook (6th Edition)
- Power supply voltage drop problem
- This circuit is for audio amplification. Please explain it to me.
- Modification of 4-20mA two-wire passive digital display meter Part 10 (Small task completed)
- Looking for AD component integrated library package library