This program uses 51 single chip microcomputer to decode pt2262 code. It is a wireless service pager code that has been successfully applied in products with LED display.
There are schematic diagrams, pcb files and complete code for download:
http://www.51hei.com/ziliao/file/37724122PT2272.rar
; ================================================= ======== ; ================================================= =============== ; PT2272 analog decoder (receives 2262 format serial data stream from RF decoding module) ; Manage three-digit digital tube display ; Use AT24C32 to implement stack-type power-off cache protection, and clear the stack through a special clear transmitter ; The display has two levels of brightness variation ; With buzzer, after receiving the new code, the buzzer will sound 2/3 times, the display will be highlighted, and it will return to normal after three seconds ; ; CPU: AT89C2051@12MHz ; ; COPYRIGHT yanggt@163.net SEP 9, 2004 ; ================================================= ============== ; ================================================= ================== EESIZE EQU 32768; EEPROM capacity (32768/16384/8192/4096/2048/1024/512) PWDF EQU 50H; used in EEPROM to identify the historical record is valid MYA0_3 EQU 00010101B ; Valid identification code, no response if it does not match MYCLRL EQU 00H; Master mobile phone code value (currently 0000H) MYCLRH EQU 00H; calculated from A4-A11 (00 D7 D6 D5 D4 D3 D2 D1) (D0) BELONT EQU 40 ;Sound duration BELOFT EQU 20 ;Silence time SCL BIT P3.0 ;AT24C32 (4K bytes) SDA BIT P3.1 SIGPIN BIT P3.2 ;Receive signal input BELL BIT P3.3 ;Buzzer control VSEL BIT P1.0 ;LED brightness selection, H = high brightness U0E BIT P3.5 ; LED position selection U1E BIT P3.4; Tens LED bit selection U2E BIT P3.7; Hundreds digit LED bit selection BELLSW BIT 78H ;Buzzer operation period = 1 EEEMPTY BIT 79H ;EEPROM empty flag BELLON BIT 7AH ; Notification buzzer sounds CODEOK BIT 7BH ;The received code is correct TMK250 BIT 7CH; 250us flag, set once every 250us SIGING BIT 7DH; Mobile phone signal transmission is continuing = 1, no signal = 0 LEDHIGH BIT 7EH ;Display brightness flag = 0: normal; = 1: high brightness TOUTMK BIT 7FH; The time for the display to reduce brightness has expired = 1 RBUF81 DATA 20H ; The last 8 bits are the first sampling result A4-A11 RBUF82 DATA 21H ; The second sampling result of the last 8 bits A4-A11 RBUF44 DATA 22H ; The first 4 bits of the 12th sampling result A0-A3 SIGPOT DATA 23H; Receive the last level of the pin and directly save all the contents of P3 port SIGMSK EQU 04H ;SIG----P3.2 TCNTL DATA 24H; Timing counter (4ms time base) TCNTH DATA 25H TMX16 DATA 26H ;16*250=4000us=4ms BELLTM DATA 27H ; Ringing time DBUF0 DATA 28H ; Ones display buffer DBUF1 DATA 29H ; Tens digit DBUF2 DATA 2AH ; Hundreds digit EEPTRL DATA 2BH ; EEPROM current position pointer EEPTRH DATA 2CH EEPOL DATA 2DH ; Save and clear pointer EEPOH DATA 2EH ; RES2F DATA 2FH ; Use the flag bit EEBUF DATA 30H ;30-37H, I2C buffer BELLCNT DATA 38H ;Bell count counter (3/2/1) BELLTMK DATA 39H CODEL DATA 40H ;Received code low bit CODEH DATA 41H ; High CODELK DATA 42H ;Save the previous code CODEHK DATA 43H ; CODE1L DATA 44H CODE1H DATA 45H ; ================================================= ====== ; ================================================= ============ ORG 0000H LJMP START ; ================================================= ================== ; Enter once every 250us, switch the display position every 16 times (4ms) ; ================================================= ================= ORG 000BH ;Timer 0 interrupt entry, exclusive R7 TM0SUB: SETB TMK250 TM0_4MS:DJNZ TMX16, TM0_E MOV TMX16, #10H JNB BELLSW, TM0_CLY DJNZ BELLTM, TM0_CLY SETB BELLON TM0_CLY: JB TOUTMK, TM0_0 ; executed every 4ms DJNZ TCNTL, TM0_0 DJNZ TCNTH, TM0_0 SETB TOUTMK TM0_0: CJNE R7, #0, TM0_1; Current display bit CLR U2E MOV P1, DBUF0 INC R7 UE RETI TM0_1: CJNE R7, #1, TM0_2 U0E MOV P1, DBUF1 INC R7 SETB U1E RETI TM0_2: CLR U1E MOV P1, DBUF2 MOV R7, #0 SETB U2E RETI TM0_E: RETI ; ================================================= ============== ; ================================================= ====== START: MOV P1, #00H ; Turn off the display, normal brightness CLR BELLON CLR BELL ACALL EECHK ; Check EEPROM, determine the pointer, and extract the last history record number ACALL TOBUF MOV TMX16, #10H; Set the interrupt count register to the initial value 16 SETB TOUTMK ; Delay timer is in stop state U0E CLR U1E CLR U2E MOV R7, #00H ; Display from the first bit MOV TMOD, #12H ;TIMER0, MODE 2, TIMER1, MODE 1 MOV TL0, #06H MOV TH0, #06H ; time = 250us SETB TR0 SETB ET0 SETB EA MOV A, #6-1 ;Buzzer sounds automatically when power on ACALL BELL_S MOV BELLTM, #BELONT-20 ACALL LED OFF CLR LEDHIGH ; turn off high brightness MOV SIGPOT, #00H ;Assume receiving pin = 0 at the beginning CLR SIGING SETB F0 ;=--=--=--=--=--=--=--=--=--=--==--=--=--=--=--=-- MAIN: JNB BELLSW, MAIN_S0 ACALL BELL_M MAIN_S0:JNB LEDHIGH, MAIN_00; Display normal brightness, do not check the flag JNB TOUTMK, MAIN_0 ACALL LEDOFF; Display returns to normal brightness CLR LED HIGH JB EEEMPTY, MAIN_0 ; No unconfirmed code AJMP MAIN_01 MAIN_00:JB EEEMPTY, MAIN_0 ; No unconfirmed code JNB TOUTMK, MAIN_0 MOV A, #2-1 ACALL BELL_S ; ringing MAIN_01:MOV TCNTL, #LOW(5000/4); high brightness for 3000ms = 3s before recovery MOV TCNTH, #HIGH(5000/4) CLR TOUTMK MOV BELLTM, #30 MAIN_0: MOV A, P3; monitor SIG pin level changes XCH A, SIGPOT XRL A, SIGPOT ANL A, #SIGMSK JZ MAIN ;A=0 means the pin level does not change SETB F0 ACALL GETCOD ; Start receiving, return directly if an error occurs JC MAIN ; The received code is invalid and discarded. ACALL FIND JC MAIN ;C=1, indicating that the code is invalid and nothing happens MOV CODE1L, CODEL MOV CODE1H, CODEH CLR F0 ACALL GETCOD ; Start receiving, return directly if an error occurs SETB F0 JC MAIN ; The received code is invalid and discarded. ACALL FIND JC MAIN ;C=1, indicating that the code is invalid and nothing happens MOV B, A MOV A, CODE1L XRL A, CODEL JNZ MAIN_0D MOV A, CODE1H XRL A, CODEH JZ MAIN_0C MAIN_0D:MOV CODE1L, CODEL MOV CODE1H, CODEH CLR F0 ACALL GETCOD ; Start receiving, return directly if an error occurs SETB F0 JC MAIN ; The received code is invalid and discarded. ACALL FIND JC MAIN ;C=1, indicating that the code is invalid and nothing happens MOV B, A MOV A, CODE1L XRL A, CODEL JNZ MAIN_E MOV A, CODE1H XRL A, CODEH JNZ MAIN_E MAIN_0C:MOV A, B JNZ MAIN_1 ;A=1: master mobile phone, transfer to unstack MOV BELLCNT, #4-1 MOV A, CODEL XRL A, CODELK JNZ MAIN_0A MOV A, CODEH XRL A, CODEHK JZ MAIN_0B ;Encoding filtering can be performed here=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- MAIN_0A:MOV BELLCNT, #6-1 ACALL EEPUSH ; Other phones, save and display ACALL TOBUF MAIN_0B:SETB TOUTMK MOV TCNTL, #LOW(3000/4); high brightness lasts for 3000ms = 3s before recovery MOV TCNTH, #HIGH(3000/4) CLR TOUTMK SETB LEDHIGH; The display enters the high brightness state MOV A, BELLCNT ACALL BELL_S ; ringing MOV BELLTM, #BELONT AJMP MAIN MAIN_1: MOV A, CODEL; check mobile phone number XRL A, #MYCLRL JNZ MAIN_E MOV A, CODEH XRL A, #MYCLRH JNZ MAIN_E ACALL EEPOP ; Execute stack popping operation MAIN_E: AJMP MAIN ; ================================================= ============ ; End of main program ; ================================================= =========== BELL_S: MOV BELLCNT, A SETB BELLSW SETB BELL RET ; ================================================= ============== BELL_M: JBC BELLON, BELLM RET BELLM: MOV A, BELLCNT BELLM0: JNB ACC.0, BELLM1 MOV BELLTM, #BELOFT ;A.0=1, it is ringing, it should be turned off CLR BELL AJMP BELLM9 BELLM1: MOV BELLTM, #BELONT ; mute state, should be turned on SETB BELL BELLM9: DJNZ BELLCNT, BELLME CLR BELLSW CLR BELL BELLME: RET ; ================================================= ================== LEDOFF: ANL DBUF0, #0FEH ANL DBUF1, #0FEH ANL DBUF2, #0FEH RET ; ================================================= =============== ; ================================================= =========== TM1SET: CLR TR1 ;1,ACALL=2 CLR TF1 ;1 MOV TL1, DPL ;2 MOV TH1, DPH ;2 SETB TR1 ;1 RET ;2, TOTAL=2+1+1+2+2+1+2=11us ; ================================================= ==================== ; Receive code, and it is recognized if two identical codes are received. ; Receive the next one after the transmission is completed. ; ================================================= ============ GETCOD: NOP GETC_S: MOV DPTR, #65535-50000 ; Search for a low level that lasts at least 4ms within 50ms [***] ACALL TM1SET GETC_S0:MOV R6, #16 ;16*250us=4000us=4ms GETC_S1:CLR TMK250 GETC_S2:JB TF1, GETC_E; Here the timeout means that there is no transmitter working or the last transmission has ended. JB SIGPIN, GETC_S0 JNB TMK250, GETC_S2 DJNZ R6, GETC_S1 MOV R5, #4*2 ; First receive A0-A3, 4 bits in total, 8 sampling points MOV DPTR, #65535-8000 ; A rising edge must occur within the next 8ms [***] ACALL TM1SET GETC_0: JB TF1, GETC_E; Here the timeout means that there is no transmitter working or the last transmission has ended. JNB SIGPIN, GETC_0 ; JB SIGING, GETC_F ; The last transmission has not yet ended, return directly without receiving the code. ; AJMP GETC_20 JNB SIGING, GETC_20 ; The last transmission has not yet ended, return directly without receiving the code. JNB F0, GETC_20 AJMP GETC_F GETC_1P:MOV DPTR, #65535-1250+11+2; A low level must appear within the next 1250us [***] ACALL TM1SET GETC_1: JB TF1, GETC_F; Timeout JB SIGPIN, GETC_1 MOV DPTR, #65535-1250+11+2; A rising edge must appear within the next 1250us [***] ACALL TM1SET GETC_2: JB TF1, GETC_F; Timeout JNB SIGPIN, GETC_2 GETC_20:MOV DPTR, #65535-500+11+2; fixed delay 500us before sampling ACALL TM1SET JNB TF1, $ MOV C, SIGPIN; Sample received signal MOV A, RBUF44 RLC A MOV RBUF44, A DJNZ R5, GETC_1P ; Receive 4 data bits in a loop and perform 8 samplings MOV R5, #8*2 ; Receive A4-A11, 8 data bits in total, 16 sampling points MOV R0, #RBUF81; RBUF81 stores the first sampling value GETC_3P:MOV DPTR, #65535-1250+11+2; A low level must appear within the next 1250us [***] ACALL TM1SET GETC_3: JB TF1, GETC_F; Timeout JB SIGPIN, GETC_3 MOV DPTR, #65535-1250+11+2; A rising edge must appear within the next 1250us [***] ACALL TM1SET GETC_4: JB TF1, GETC_F; Timeout JNB SIGPIN, GETC_4 GETC_40:MOV DPTR, #65535-500+11+2; fixed delay 500us before sampling ACALL TM1SET JNB TF1, $ MOV C, SIGPIN; Sample received signal MOV A, @R0 RLC A MOV @R0, A XRL 00H, #RBUF81 ; Switch between RBUF81 and RBUF82 XRL 00H, #RBUF82 DJNZ R5, GETC_3P ; Receive 8 data bits in a loop and perform 16 samples SETB SIGING CLR C RET GETC_E: CLR SIGING GETC_F: SETB C RET ; ================================================= ========== ; ================================================= ============== FIND: MOV A, RBUF44 ;Analyze code CJNE A, #MYA0_3, FIND_E MOV R4, RBUF81 MOV R5, RBUF82 MOV R6, #7 MOV R0, #00H MOV R1, #00H FIND_L: MOV A, R0 ; multiply the result by 3 CLR C RLC A MOV B, A MOV A, R1 RLC A XCH A, B ADD A, R0 MOV R0, A MOV A, B ADDC A, R1 MOV R1, A ;Multiply the result by 3 and end MOV A, R4 RLC A MOV R4, A ;D7-->C MOV A, R5 RL A MOV R5, A ANL A, #01H ;D7-->A.0 ADDC A, R0 ;A.0+C+R0 MOV R0, A MOV A, R1 ADDC A, #00H MOV R1, A DJNZ R6, FIND_L ; Determine the encoding result of A4-A10 CLR A MOV C, RBUF81.0 ; Analyze the code to determine whether it is the main control mobile phone MOV ACC.0, C MOV C, RBUF82.0 ADDC A, #00H; A=(0,1,2). A=0: non-master mobile phone; A=1: master mobile phone; A=2: invalid mobile phone JB ACC.1, FIND_E ;A=2! MOV CODEL, R0 MOV CODEH, R1 CLR C RET ;A=0/1 FIND_E: SETB C RET ; ================================================= ============== ; ================================================= ===================== EECHK: MOV EEPTRL, #00H; 0000H record is not used, because 0000H is used to determine whether the EEPROM is empty MOV EEPTRH, #00H MOV EEBUF, #00H MOV EEBUF+1, #00H EECHK0: MOV CODEL, EEBUF; put into encoding buffer MOV CODEH, EEBUF+1 ANL CODEH, #0FH MOV DPL, EEPTRL ; read the two bytes at the bottom of the stack MOV DPH, EEPTRH INC DPTR INC DPTR; Point to the next record, but do not modify the pointer for now MOV A, DPH ADD A, #HIGH(65536-EESIZE) ;??? JC EECHK9 ;All record spaces have been checked. MOV EEPOL, DPL MOV EEPOH, DPH ; Temporarily save here MOV R0, #EEBUF MOV B, #02H ACALL I2CD_R MOV A, EEBUF+1 ANL A, #0F0H XRL A, #PWDF JNZ EECHK9 MOV EEPTRL, EEPOL MOV EEPTRH, EEPOH AJMP EECHK0 EECHK9: CLR EEEMPTY ;Assume not empty MOV A, EEPTRL ORL A, EEPTRH JNZ EECHKE SETB EEEMPTY; declare EEPROM empty EECHKE: RET ; ================================================= ====================== ; ================================================= ============ EEPUSH: MOV EEBUF, CODEL; write to EEPROM stack, use real stack top MOV EEBUF+1, CODEH ORL EEBUF+1, #PWDF ; Flag, 50H MOV DPL, EEPTRL MOV DPH, EEPTRH INC DPTR ; Each record is entered, the pointer increases by 2 INC DPTR MOV A, DPH ; Perform stack overflow check ADD A, #HIGH(65536-EESIZE) JC EEPUSHE ANL DPL, #0FEH MOV EEPTRL, DPL MOV EEPTRH, DPH MOV R0, #EEBUF MOV B, #02H ACALL I2CD_W CLR EEEMPTY ; declare EEPROM is not empty EEPUSHE:RET ; ================================================= =========== ; ================================================= =============== EEPOP: MOV A, EEPTRL; EEPROM exit operation ORL A, EEPTRH JZ EEPOPB EEPOP0: MOV EEPOL, EEPTRL MOV EEPOH, EEPTRH CLR C ; pointer minus 2, pointing to the previous data MOV A, EEPTRL SUBB A, #02H MOV EEPTRL, A MOV A, EEPTRH SUBB A, #00H MOV EEPTRH, A ; pointer has been updated MOV A, EEPTRL ; Check if the stack becomes empty after exiting a record? ORL A, EEPTRH JNZ EEPOP1 MOV CODEL, A MOV CODEH, A SETB EEEMPTY; declare EEPROM empty AJMP EEPOP9 EEPOP1: MOV DPL, EEPTRL; read the top record of the stack MOV DPH, EEPTRH MOV R0, #EEBUF MOV B, #02H ACALL I2CD_R MOV CODEL, EEBUF ; put into encoding buffer MOV CODEH, EEBUF+1 ANL CODEH, #0FH ; shield the sign EEPOP9: MOV EEBUF, #00H ; Clear the record that has just been popped off the stack MOV EEBUF+1, #00H MOV DPL, EEPOL MOV DPH, EEPOH MOV R0, #EEBUF MOV B, #02H ACALL I2CD_W ACALL TOBUF ; send to display buffer ACALL LED OFF EEPOPB: MOV A, #2-1 ACALL BELL_S ; ringing ; MOV BELLTM, #30 EEPOPE: RET ; ================================================= ===== ; ================================================= ==================== TOBUF: MOV A, CODEL ; fill in the display buffer MOV B, CODEH ;/100 MOV R2, #00H TOBUF_0:CLR C SUBB A, #100 XCH A, B SUBB A, #00H XCH A, B JC TOBUF_8 INC R2 AJMP TOBUF_0 TOBUF_8:ADD A, #100 MOV B, #10 DIV AB MOV DPTR, #FONT MOVC A, @A+DPTR MOV DBUF1, A MOV A, B MOVC A, @A+DPTR MOV DBUF0, A MOV A, R2 MOVC A, @A+DPTR MOV DBUF2, A MOV CODELK, CODEL MOV CODEHK, CODEH RET ; ================================================= ================ ; ================================================= ========== DELAY: PUSH ACC; delay time = A*10ms MOV A, #20 DLY1: PUSH ACC MOV A, #250 DJNZ ACC, $ ;500us POP ACC DJNZ ACC, DLY1 POP ACC DJNZ ACC, DELAY RET ;======= I2C subroutine ============================================ ; I2CD_W, I2CD_R ;LAYER 1 ; I2C_O, I2C_I ;LAYER 2 ; I2C_BG, I2C_ED ; LAYER 3 ;================================================== ========= ; Write several bytes to memory, up to 8 bytes. ; Entry: DPTR EEPROM storage unit address to be written. ; R0 points to the first address of the byte to be written in RAM. ; B Number of bytes written. ; Exit: If C=1, it means a writing error. I2CD_W: ACALL I2C_BG MOV A, #10100000B ; write command ACALL I2C_O JC I2CD_WE ;C=1, ACK bit not received, error, no further processing, return directly MOV A, DPH ACALL I2C_O JC I2CD_WE MOV A, DPL ACALL I2C_O JC I2CD_WE I2CD_WL:MOV A, @R0 ACALL I2C_O JC I2CD_WE INC R0 DJNZ B, I2CD_WL ACALL I2C_ED CLR C RET I2CD_WE:ACALL I2C_ED SETB C RET ; ================================================= ======= ; Read several bytes from EEPROM ; Entry: DPTR storage unit address to be read. ; R0 points to the first address of the buffer to be read in RAM. ; B The number of bytes read. ; Exit: The read content is in the buffer. If C=1, it means a read error. I2CD_R: ACALL I2C_BG MOV A, #10100000B ; send the [write] command first ACALL I2C_O JC I2CD_RE ;C=1, ACK bit not received, error, no further processing, return directly MOV A, DPH ; send address low bit ACALL I2C_O JC I2CD_RE MOV A, DPL ; send address low bit ACALL I2C_O JC I2CD_RE ACALL I2C_BG MOV A, #10100001B ;Send read command ACALL I2C_O JC I2CD_RE I2CD_IB:MOV DPL, #08H ;Get a byte SETB SDA I2CD_IL:SETB SCL ;SCL=1 NOP MOV C, SDA; MCU samples SDA and sends it to C RLC A ;C->ACC.0 CLR SCL ;SCL=0 DJNZ DPL, I2CD_IL; get a byte in ACC MOV @R0, A INC R0 MOV A, B XRL A, #01H JZ I2CD_GO CLR SDA NOP I2CD_GO:SETB SCL NOP CLR SCL DJNZ B, I2CD_IB ; Get more than one byte ACALL I2C_ED CLR C RET I2CD_RE:ACALL I2C_ED SETB C RET ; ================================================= ================ ;Send a byte to the IIC bus I2C_O: PUSH B MOV B, #08H I2C_OLP:RLC A ;ACC.7 -> C MOV SDA, C SETB SCL ;SCL=1 NOP CLR SCL ;SCL=0 DJNZ B, I2C_OLP SETB SDA ; ready to receive ACK bit SETB SCL ;SCL=1, [SDA=1] NOP MOV C, SDA CLR SCL POP B RET ;C=1, ACK bit not received, error, C=0, ACK bit received, normal ; ================================================= ==== I2C_BG: SETB SCL ; Make sure SCL=HIGH CLR SDA ;Send start bit to IIC bus NOP CLR SCL RET ; ================================================= ======================================== I2C_ED: CLR SDA SETB SCL NOP SETB SDA ;Send stop bit to IIC bus RET ; ================================================= =============== ; It is best to display 000-999=1000, otherwise it is easy to cause misunderstanding. Here all 2187 are displayed, 0-9, AF, H, L, P, U, Y ; ================================================= ============= FONT: DB 0FDH ;"0" DB 061H ;"1" DB 0DBH ;"2" DB 0F3H ;"3" DB 067H ;"4" DB 0B7H ;"5" DB 0BFH ;"6" DB 0E1H ;"7" DB 0FFH ;"8" DB 0F7H ;"9" DB 0EFH ;"A" DB 03FH ;"B" DB 09DH ;"C" DB 07BH ;"D" DB 09FH ;"E" DB 08FH ;"F" DB 06FH ;"H" DB 071H ;"J" DB 01DH ;"L" DB 0CFH ;"P" DB 07DH ;"U" DB 077H ;"Y" ; ================================================= = END
Previous article:Design of a water bath thermostat controller with switch quantity control
Next article:Infrared data communication experiment
Recommended ReadingLatest update time:2024-11-16 15:55
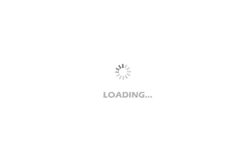
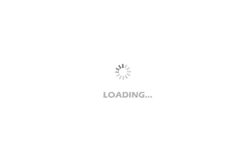
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- EEWORLD University ---- WPI-NXP demo
- Synchronous Rectification
- ESP32-Audio-Kit (8388 version) development environment setup video tutorial
- I'm going to make some IC chips. Please contact me if you need them.
- 6 steps to become an FPGA design expert
- Key Design Points for L-Band GaN Power Amplifiers
- What instruments do hardware engineers need?
- Stayed up late to sort out, and here comes the information about these components on the list~
- Which IDE is more suitable for Python?
- [Tutorial] Teach you how to set the probe ratio on different brands of oscilloscopes (Tektronix + Keysight + Puyuan)