1. Introduction
BSP is the abbreviation of Board Support Package. This term is usually used in the embedded field, mainly referring to the various support libraries provided by system developers when developing embedded applications. Since each embedded system provider has a different understanding of BSP based on its own operating system, when it comes to the specific meaning of BSP, it must be based on the specific embedded system. This article will take the wireless communication product based on SEP3203 as an example to explain the content and implementation of its BSP. The hardware structure of the wireless communication product is as follows (Figure 1 Wireless Communication Product Hardware Structure Diagram). SEP3203 is connected to Nor flash and SDRAM, and communicates with the wireless module and instrument through the serial port. The software execution flow chart is as follows (Figure 2 Wireless Communication Product Software Flow Chart). The BSP of the wireless communication product will provide application developers with a hardware-independent development platform.
2. Overview Design
2.1. Contents of the wireless communication platform BSP
According to the hardware platform of the wireless data terminal, the content of BSP should include the configuration of EMI (memory interface), the configuration of PMU (power management module), the code transfer and the serial port driver.
2.2 Overall Framework
In order to complete the content of BSP, after power-on, EMI and PMU should be configured first, because EMI determines the address allocation of memory, and PMU is the premise of configuring other hardware. Then, considering the efficiency of code execution, the code is moved to the memory for execution and the pointer is relocated. Finally, the configuration of the serial port is completed in the main program as shown in (Figure 3 BSP process). The code that runs from power-on to the end of the Remap operation is the startup code of the wireless communication platform.
3. Design of startup code
3.1. Writing startup code
After power-on, the pc pointer points to address 0x00000000, and at this time the address 0x00000000 coincides with the first address of NOR FLASH. At this time, NOR FLASH has two addresses at the same time: 0x00000000 and 0x20000000. Therefore, the pc pointer actually points to the first address of NOR FALSH, which is the starting address of the startup code. The startup code is written as follows:
//Configure PMU and EMI
ldr r1, =0x1000100c //Configure the control register for the internal module clock source supply
ldr r2, =0x0ffff;
str r2,[r1]
ldr r1, =0x10001014 //Configure chip working mode register
ldr r2, =0x1
str r2,[r1]
…
ldr r1, =0x11000000 //Configure memory parameter configuration register
ldr r2, =0xB91331FF
str r2, [ r1 ]
ldr r1, =0x11000014 //Configure SDRAM timing configuration register
ldr r2, =0x01004077
str r2, [ r1 ] [page]
//Move all code in Nor flash (including bootloader) to SDRAM
ldr r3, =0x00000000 //SDRAM start address
ldr r1, =0x30002000 //Transfer code SDRAM target address
ldr r2, =0x20001000 //The starting address of the code in flash
LOOP // Each loop carries 32 bits of code
ldr r4, [r2], #4 //Move code from flash to SDRAM
str r4, [r1], #4
add r3, r3, #1
cmp r3, #0x2C000 //0x2C000 is the code amount
bne LOOP
//REMAP operation
ldr pc, =0x20000080 //locate pc pointer
mov r0, r0 //empty statement
mov r0, r0
mov r0, r0
mov r0, r0
ldr r1, =0x11000010 //Configure REMAP register
ldr r2, =0x0000000b
str r2, [ r1 ]
// Make the pc pointer point to the starting address of the main program
ldr pc, =0x30002000 //locate pc pointer
mov r0, r0
mov r0, r0
mov r0, r0
mov r0, r0
3.2. Difficulty analysis of startup code
ARM7 executes instructions in a three-stage pipeline structure, that is, when the first instruction is executed, the second instruction is being decoded and the third instruction is being fetched (Figure 4: Three-stage pipeline).
In the Remap operation, the statement ldr pc, =0x20000080 points the pc pointer to the following four mov statements. These four mov statements are meaningless and are only used to fill the three-stage pipeline to avoid unnecessary errors when executing the Remap operation.
After the remap operation, NOR FLASH will lose the zero address and only have the actual address 0x20000000, while SDRAM has two starting addresses, namely 0x00000000 and 0x30000000. Accessing these two addresses is actually accessing SDRAM. Therefore, after the code transfer is completed, the pc pointer should be relocated to the actual address of Nor Flash (the address starting from 0x20000000) before continuing to run the rest of the startup code, otherwise the pc will take the wrong address after the remap, resulting in an error.
The statement ldr pc, =0x30002000 positions the pc pointer to the entry of the main program. The following four mov statements are used to fill the three-stage pipeline. Otherwise, the pc pointer will continue to move downward after reading the instruction to reposition the pc pointer, and the wrong instruction will be fetched.
After the pc pointer jumps to the entry of the main program, the entire startup code ends.
4. Serial port driver
The serial port driver includes two parts: serial port configuration and data transmission and reception. The receiving data uses the structure of do (get fifo data) while (fifo is not empty) to read the data in the fifo; the sending data directly fills the data into the sending fifo, and its code is not repeated here. The serial port configuration is performed after the main function is run. The code is as follows:
int init_uart(unsigned long sysclk, unsigned long baudrate, unsigned long databit, unsigned long trigerlevel)
{
unsigned long baud, bit, triger, baudh, baudl;
baud = sysclk/16/baudrate
baudh = baud >> 8 // Separate the high 8 bits and low 8 bits of the baud rate
baudl = baud & 0xff
write_reg(UART0_LCR, bit); //Select to access the baud rate setting register
write_reg(UART0_DLH, baudh) //Configure the baud rate for high and low 8 bits respectively
write_reg(UART0_DLL, baudl)
read_reg(UART0_LCR) &= (~(0x1 << 7)) //Disable baud rate configuration register access
write_reg(UART0_FCR, triger) //Configure fifo trigger level
write_reg(UART0_IER, 0x00) //Enable serial port related interrupt sources
irq_enable(INT_UART0); //Enable serial port interrupt
}
Since the serial port baud rate configuration register address of the SEP3203 processor is multiplexed with other register addresses, the following operations must be performed when configuring the baud rate: The 7th bit of the bit parameter in the statement: write_reg(UART0_LCR, bit) determines the access to the baud rate configuration register, and the subsequent statement: read_reg(UART0_LCR) &= (~(0x1 << 7)) closes the access to the baud rate configuration register.
The author's innovation points:
According to the test of wireless communication platform and the actual application in instrument monitoring system, this BSP has completed the scheduled content and runs stably. The successful writing of BSP provides guarantee for more complex underlying development in the future, and also helps the transplantation and development of related embedded operating systems.
References
1 Du Chunlei. ARM Architecture and Programming[M]. Beijing: Tsinghua University Press, 2003.1-160
2 Andrew N.Sloss, Dominic Symes, Chris Wright. ARM Embedded System Development - Software Design and Optimization [M]. Beijing: Beijing University of Aeronautics and Astronautics Press, 2005.1-92
3 Dong Ce, Yang Zhijia. High-speed and low-power ASIC design of AES encryption algorithm [J]. Microcomputer Information-2005 Issue 09X, 36-37
Previous article:Design of RFID reader based on ARM
Next article:Design and Implementation of S3C44B0 Boot Loader Based on U-BOOT
Recommended ReadingLatest update time:2024-11-17 02:52
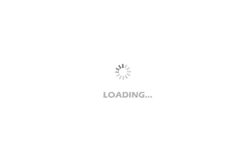
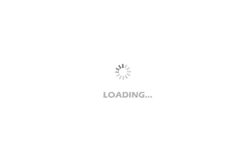
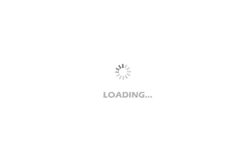
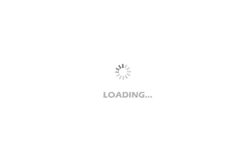
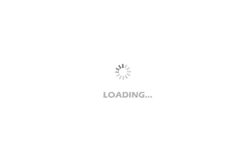
- Popular Resources
- Popular amplifiers
-
Practical Deep Neural Networks on Mobile Platforms: Principles, Architecture, and Optimization
-
ARM Embedded System Principles and Applications (Wang Xiaofeng)
-
ARM Cortex-M4+Wi-Fi MCU Application Guide (Embedded Technology and Application Series) (Guo Shujun)
-
An ARM7-based automotive gateway solution
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- Many choices in life are your destiny.
- Experience using the bootloader of TMS320VC33
- Please help me. When the circuit board motor is working, the LED light controlled by the IO port of the main control IC will flash.
- [Qinheng RISC-V core CH582] serial port routine sending and receiving test
- Understanding of analog IC
- msp430f149 microcontroller serial port C program
- How do we distinguish the difference between bypass capacitors and decoupling capacitors?
- [Distributed temperature and humidity acquisition system] + WIFI module expansion board V2
- Basic knowledge of power amplifiers - the original feedforward - is still in use today
- LMH1982 signal lock is unstable