Introduction
With the development of computer technology, especially single-chip microcomputer technology, people have increasingly adopted single-chip microcomputers to detect and control parameters such as temperature, flow and pressure in some industrial control systems. PC has powerful monitoring and management functions, while single-chip microcomputer has fast and flexible control characteristics. Communicating with external devices through the RS-232 serial interface of PC is a common communication solution in many measurement and control systems. Therefore, how to realize the communication between PC and single-chip microcomputer has very important practical significance. This article will discuss the software and hardware solutions for realizing serial communication between PC and single-chip microcomputer in VB environment. The communication program of PC is written in Visual Basic. VB is a Windows application development tool launched by Microsoft. It is widely used because of its user-friendly interface and simple programming. In addition, Visual Basic 6.0 version comes with MSCOMM control that is specially used to realize serial communication.
1. Hardware Principle
Current PCs have at least one serial communication port RS-232. RS-232 ports can be used for communication between two computers. The logic level of RS-232 is represented by positive and negative voltages, and the signal uses negative logic. The voltage range of logic 0 is +5V~+15V, and the voltage range of logic 1 is -5V~-15V. RS-232 port is the most commonly used interface for computers to communicate with other devices. It is not only simple to implement, but also cheap. There is a full-duplex asynchronous serial I/O port inside the 8051 microcontroller. Its input and output use 5V logic instead of RS-232 voltage. How to convert the two signals is a problem that needs to be solved when connecting. In fact, the solution is very simple. Just use a dedicated chip such as MAX232. The circuit
structure is shown in the figure below.
MAX232 contains two drivers that convert TTL input into RS-232 output, and two receivers that translate RS-232 input into CMOS output. These drivers and receivers have reverse functions. The size of the 4 external capacitors is 1uF. If polarized capacitors are used, pay attention to the polarity. The voltage of pin 6 is negative, so the positive pole of its capacitor is connected to the ground. The capacitor level is at least 15V.
2. Software Design
In this communication, we take the communication between the host and slave computers of a measurement and control system as the background, and give the communication program examples of the microcontroller part and the VB environment. The microcontroller in the system is responsible for data acquisition, processing and control, and the host computer performs on-site visual detection. The communication protocol adopts half-duplex asynchronous serial communication mode, and the RTS signal of RS232 is used for transceiver conversion. The transmission data uses binary data, and the master-slave communication is adopted between the host and the slave.
(1) Serial communication program of the microcontroller
A group of data (30) collected by the 8051 microcontroller from the outside is stored in the RAM20H~3DH area on the chip. It is required to transmit this data block to the PC. In order to ensure the correct transmission, the microcontroller first sends data #55H (communication signal) to the PC before transmission. After receiving it, the PC sends data #0AAH (response signal) back to the microcontroller. After verification, the microcontroller starts to transmit data to the PC.
The operation of the serial port can be divided into the following steps: ① Initialize the serial port; ② Send contact signal; ③ Receive contact signal; ④ Send data. The following program is written in assembly language:
ORG 0030H
START:MOV SP,#60H
MOV TMOD, #20H ;T1 working mode 2
MOV TH1, #72H
MOV TL1, #72H ;Baud rate 110HZ
SETB TR1
MOV PCON, #00H ;SMOD=0
MOV SCON, #50H ;Serial mode 1
MOV R0,#20H
MOV R2,#30 ;Number of data
XX1: MOV A,#55H
MOV SBUF,A ;Send contact signal
WAIT1: JBC TI,WAIT2 ;Wait for sending to be completed
AJMP WAIT1
WAIT2: JBC RI,READ ;Wait for receiving to be completed
AJMP WAIT2
READ: MOV A,SBUF ;Receive contact signal
CJNE A,# 0AAH,XX1 ;Received data is incorrect, restart
LOOP: MOV A,@R0
MOV SBUF, A
WAIT: JBC TI, LOOP1
AJMP WAIT; Wait for the transmission to be completed
LOOP1: INC R0
DJNZ R2, LOOP
END [page]
(2) Computer serial communication program
In order to more conveniently understand the data acquisition situation in real time, we assign the data read from the single-chip microcomputer to the array respectively, and then draw the waveform curve.
First, open a VB project and arrange an MSCOMM control on the form as a serial communication channel. Press F4 to call out the property window. The variable COMPORT property is 2. In addition, the Rthreshold property is set to 1, which means that as long as any string is transmitted from the outside, an event will be triggered immediately. Arrange a timer as the execution control of the continuous action. Once a bit of data is sent, an interrupt will be triggered and the next data will be sent. Set up a picturebox object and draw the data transmitted by the single-chip microcomputer in the form of lines on this picture box control. Press F4 to call out the property window and change its Name property to "Graph1".
The MSCOMM control supports data transmission in text and binary formats. Since the lower computer is a single-chip microcomputer, it is more convenient to process binary data. Therefore, in this example, data is sent and received in binary format. VB supports byte variable type to store binary data. The bytes read from the serial port are stored in byte array variables. Therefore, two BYTE type dynamic arrays are defined to store and receive data. When receiving data, the event-driven method is used. When there are Rthreshold data in the receiving buffer, the ONCOMM event is triggered.
This program receives 30 data sent from the microcontroller. When the data enters the input buffer of the PC, the program will continuously read the data in the input buffer, collect these read data together, and assign them to the array.
Because VB supports the coordinate format, we use the SCALE function to make coordinates, define the X axis from 0 to 30, indicating that there are 30 data, and the Y axis from -20 to +20, indicating that the range of collected data is -20 to +20. The expression is SCALE (0, +20) - (30, -20). In order to collect data in real time, the timer can be used to continuously update the curve. In this way, the collected data can be displayed dynamically.
Private sub form_l oad()
Comm1.portopen=true
Timer.interval=1000 'The timer interval is 1000s
Timer.enabled=true
Mscomm1.compore=1 'Select communication port 1
Mscomm1.settings="110,n,8,1" 'Baud rate 110, no parity, 8 data bits, one stop bit
Mscomm1. inputmode=1 'Retrieve data in binary form through the input attribute
Mscomm1.inputlen=0 'Read the entire content of the receive buffer
Mscomm1.rthreshold=1 'Triggers an oncomm event for each character received
Graph1.scale(0,10)-(20,0) 'Customize coordinates
Grahp1.forecoclr=rgb(0,0,255)
End sub
Private Sub Command1_Click()
Dim a!(30), b!(30)
num = MSComm1.InBufferCount 'Get the number of bytes in the input buffer
instring = MSComm1.Input 'Send the input buffer data to the array
For i = 0 To num - 1
a(i) = i
b(i) = instring(i)
GRAPH1.Cls
If i = 1 Then
GRAPH1.PSet (a(i), b(i))
Else
GRAPH1.Line -(a(i), b(i)) ' Give the array data to the drawing function
End If
Next i
End Sub
3. Conclusion
The event-driven method of VB6.0 can be used to easily develop data acquisition and monitoring systems. A single PC can be used to measure and monitor multiple control signals. The entire control system is easy to design and has great practicality for the design of small measurement and control systems.
References:
1. He Limin, Single Chip Microcomputer Application System Design. Beijing: University of Aeronautics and Astronautics Press, 1992
2. Jan Axelson, Serial Port Encyclopedia, Elite Technology Translation, 2001.5
Previous article:Design of a single chip microcomputer controlled instruction converter
Next article:Design Method of Driving Stepper Motor Using Bit Display Interface
Recommended ReadingLatest update time:2024-11-17 07:21
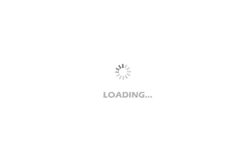
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- Does anyone know about the Xtensa core of ESP32?
- Application of Four-way Two-Tube Forward Series-parallel Output Method in Maglev Train DC-DC Converter
- Comparison of the internal structures of TI's three major series of DSPs
- This is good, you can try it
- Looking for the Chinese user manual for MSP430FR6989
- [RVB2601 creative development and application] 1. RGB three-color breathing light and key anti-shake
- Urgent recruitment: C# host computer development engineer
- Download the Maxim Basic Analog IC APP to help you innovate analog design! Comments and posts are all rewarded!
- Raspberry Pi 4B Review Summary
- How to Analyze a Transformer Circuit with Taps