Most ARM-based chips are complex on-chip systems. Most hardware modules in such complex systems are configurable and need to be set by software to their required working states. Therefore, before the user's application program, a special piece of code is required to complete the initialization of the system. Since this type of code directly programs the processor core and hardware controller, it is generally written in assembly language. General common content includes:
Interrupt vector table
Initialize the memory system
Initialize the stack
Initialize the interface and equipment with special requirements
Initialize the user program execution environment
Changing the processor mode
Calling the main application
Interrupt vector table
ARM requires that the interrupt vector table must be placed in a continuous 8X4 byte space starting from address 0.
Whenever an interrupt occurs, the ARM processor forces the PC pointer to be set to the address value of the corresponding interrupt type in the vector table. Because each interrupt only occupies 1 word of storage space in the vector table, only one ARM instruction can be placed to make the program jump to other places in the memory and then execute the interrupt processing.
The program implementation of the interrupt vector table is usually expressed as follows:
AREA Boot ,CODE, READONLY
ENTRY
B ResetHandler
B UndefHandler
B SWIHandler
B PreAbortHandler
B DataAbortHandler
B IRQHandler
B FIQHandler
The keyword ENTRY specifies that the compiler should keep this code, because the compiler may consider it as a redundant code and optimize it. When linking, make sure that this code is linked at address 0 and serves as the entry point for the entire program.
Initialize the memory system
Memory Type and Timing Configuration
Usually Flash and SRAM are both static memory types and can share the same memory port; however, DRAM is usually equipped with a dedicated memory port because of its characteristics such as dynamic refresh and address line multiplexing.
The interface timing optimization of the memory port is very important, which will affect the performance of the entire system. Because the speed bottleneck of the general system operation exists in memory access, the memory access timing should be as fast as possible; at the same time, the resulting stability issues must be taken into account.
Memory address distribution
A typical case is address remapping of the boot ROM.
Initialize the stack
Because ARM has 7 execution states, the stack pointer register (SP) of each state is independent. Therefore, a stack address must be defined for SP for each mode needed in the program. The method is to change the status bit in the status register to switch the processor to a different state, and then assign a value to SP. Note: Do not switch to User mode to set the stack of User mode, because after entering User mode, you can no longer operate CPSR to return to other modes, which may affect the execution of the next program.
This is a code example of stack initialization, in which only three modes of SP pointer are defined:
MRS R0,CPSR
BIC R0,R0,#MODEMASK For safety reasons, mask all bits except the mode bit
ORR R1,R0,#IRQMODE
MSR CPSR_cxfs,R1
LDR SP,=UndefStack
ORR R1,R0,#FIQMODE
MSR CPSR_cxsf,R1
LDR SP,=FIQStack
ORR R1,R0,#SVCMODE
MSR CPSR_cxsf,R1
LDR SP,=SVCStack
Initialize ports and devices with special requirements
Initialize the application execution environment. An ARM image file consists of three segments: RO, RW, and ZI. RO is the code segment, RW is the initialized global variable, and ZI is the uninitialized global variable. The image is always stored in ROM/Flash at the beginning. The RO part can be executed in ROM/Flash or transferred to the faster RAM for execution; while the RW and ZI parts must be transferred to the writable RAM. The so-called initialization of the application execution environment is to complete the necessary data transfer from ROM to RAM and clear the content.
The following is a direct implementation of a commonly used memory model in ADS:
The compiler uses the following symbols to record the start and end addresses of sections:
|Image$$RO$$Base| : RO segment starting address
|Image$$RO$$Limit| : RO segment end address plus 1
|Image$$RW$$Base| : RW segment starting address
|Image$$RW$$Limit| : ZI segment end address plus 1
|Image$$ZI$$Base| : ZI segment start address
|Image$$ZI$$Limit| : ZI segment end address plus 1
The values of these labels are calculated based on the ro-base and rw-base settings set in the linker.
Initializing the user execution environment mainly involves copying the RO, RW, and ZI segments to the specified location.
LDR r0,=|Image$$RO$$Limit| Get the starting address of the RW data source
LDR r1,=|Image$$RW$$Base| The starting address of the execution area of the RW area in RAM
LDR r2,=|Image$$ZI$$Base| The starting address of the ZI area in RAM
CMP r0,r1 compares them to see if they are equal
BEQ %F1
0 CMP r1,r3
LDRCC r2,[r0],#4
STRCC r2,[r1],#4
BCC %B0
1 LDR r1,=|Image$$ZI$$Limit|
MOV r2,#0
2 CMP r3,r1
STRCC r2,[r3],#4
BCC %B2
Jump directly from the startup code to the main function entry of the application. Of course, the main function name can be defined by the user.
In the ARM ADS environment, a system-level calling mechanism is also provided.
IMPORT __main
B __main
__main() is a function provided by the compilation system, which is responsible for initializing the library functions and initializing the application execution environment, and finally automatically jumps to the main() function.
Previous article:ARM chip model selection, for beginners' reference
Next article:22 common concepts about ARM
Recommended ReadingLatest update time:2024-11-16 20:44
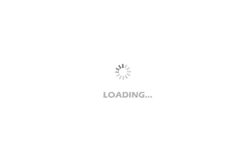
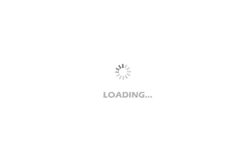
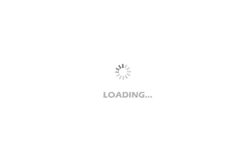
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [RVB2601 Creative Application Development] Zero-based Part 1: Play with RVB2601 (based on RISC-V MCU) Getting Started Board Power On
- Free Download with Prizes | Tektronix Automotive Electronics Test Solutions Collection
- 【Construction Monitoring and Security System】12. Kaluga parses the parameters recorded in the SD card file and connects to OneNET
- Modification of 4-20mA two-wire passive digital display meter (Part 9) (hardware photos)
- I bought a polymer lithium battery (3.7V10A, 1C) on TB. Can I use TP4056 or IP5305, IP5306 module to charge and discharge it?
- Courseware Collection|Tektronix Semiconductor Materials and Devices Seminar (2019-2018)
- RaspberryPi Pico To-Do Board using Micropython
- TTP223_BA6 uses non-isolated power supply + inverter and the lower button fails
- 【TI Recommended Course】#TI.com Online Purchasing Special: Smart Buildings#
- Filtering and Signal Processing Reference Design for MSP430 FRAM Microcontrollers