1 Characteristics of NAND Flash
Non-volatile flash memory Flash has the characteristics of fast speed, low cost and high density, and is widely used in embedded systems. There are two main types of Flash memory: NOR and NAND. The NOR type is more suitable for storing program code; the NAND type can be used for large-capacity data storage. The storage units of NAND flash memory are blocks and pages. The Samsung K9F5608 used in this article includes 2,048 blocks, each block includes 32 pages, and the page size is 528 bytes, which is divided into two 256-byte data areas, and finally a 16-byte spare space.
K9F5608 has the following characteristics: Read/write operations are performed in page units, while erase operations are performed in block units. Read, write, and erase operations are all completed through commands; byte erasure is not possible and needs to be performed before each rewrite operation. Erase a whole block; there is a certain proportion of bad blocks when leaving the factory; the number of erases for each block is limited, about 100,000 times [1].
2 Introduction to YAFFS file system
YAFFS is the first embedded file system specifically designed for NAND Flash memory, suitable for large-capacity storage devices; and is released under the GPL (General Public License) agreement, and the source code is available for free on its website.
In YAFFS, files are stored in fixed-size data blocks, and the block size can be 512 bytes, 1024 bytes, or 2048 bytes. This implementation relies on its ability to associate a data block header with each data block. Each file (including directories) has a data block header corresponding to it. The data block header stores ECC (Error Correction Code) and file system organization information for error detection and bad block processing. Taking full consideration of the characteristics of NAND Flash, YAFFS stores this data block header in the 16-byte spare space of Flash. When the file system is mounted, the file system information can be read into the memory by simply scanning the spare space of the memory, and resides in the memory. This not only speeds up the loading speed of the file system, but also increases the speed of file access. Increased memory consumption.
In order to save memory while improving the search speed of file data blocks, YAFFS uses a more efficient mapping structure to map file locations to physical locations. The data segments of the file are organized into a tree structure. This tree structure has 32-byte nodes. Each internal node includes 8 pointers to other nodes. The leaf nodes include 16 2-byte pointers to physical addresses. . When YAFFS rewrites a file, it always writes new data blocks first, and then deletes the old data blocks from the file. In this way, even if the power fails unexpectedly when modifying the file, only the smallest writing unit of the modified data will be lost, thereby achieving power failure protection and ensuring data integrity.
Combining the efficiency of the greedy algorithm and the averageness of random selection, YAFFS achieves the purpose of balancing loss average and reducing system overhead. When certain small probability conditions are met, an attempt is made to randomly select a recyclable page; in other cases, a greedy algorithm is used to recycle the "dirties" blocks [2].
The YAFFS file system is designed according to the hierarchical structure and is divided into the following four parts: yaffs_guts.c, the main algorithm of the file system. This part of the code is completely written in portable C language; yaffs_fs.c, the interface of the Linux VFS layer; NAND Interface, packaging layer between yaffs_guts and NAND memory access functions, such as calling the Linux mtd layer or RAM simulation layer; portable functions, packaging functions of services. The most important point is that in order to obtain better portability, YAFFS provides a direct call mode, which gives us the opportunity to transplant the YAFFS file system on the C51 system.
3 Transplantation process
The direct source code can be obtained at http://www.aleph1.co.uk/cgi-bin/viewcvs.cgi/ , including the following files and their header files.
◆ yaffscfg.c: Set various device parameters and system parameters.
◆ yaffsfs.c: Mainly implements directly called interface functions, such as opening files, writing files, and closing files, etc. Just include its header file in the application when using it.
◆ yaffs_flashif.c: NAND Flash operation function interface, which is the underlying function that directly operates the memory. For testing, this file uses RAMDISK simulation method to realize the operation of Flash memory. In practical applications, it is necessary to modify the definition of Flash hardware operation functions, including yflash_EraseBlockInNAND(), yflash_WriteChunkToNAND(), yflash_ReadChunkFromNAND() and yflash_InitialiseNAND().
◆ yaffs_guts.c: The main implementation algorithm of YAFFS file system.
◆ nand_ecc.c:: ECC algorithm.
◆ yaffs_ramdisk.c:: RAMDISK support code.
◆ yaffs_fileem.c: Use a file on the host to simulate Flash memory, only for testing.
◆ dtest.c:: Directly call the test function of the file system.
After obtaining the source code, the transplantation process can be divided into two steps: ①? Cut according to your own needs; ②? Convert the code to C51 style.
3.1 Downsizing
YAFFS is a powerful file system. Considering that the program code memory and RAM resources of the C51 system are very limited, and certain file operation functions may not be needed in the application, it is necessary to reduce the size of this file system. Cutting includes code cutting and data structure modifications.
First, remove the files yaffs_ramdisk.c, yaffs_ramdisk.h, yaffs_fileem.c and interface.h used for testing, and add #include yaffs_flashif.h to yaffscfg.c.
In this system, only three database files in K9F5608 are read/written. The first-level directory is sufficient. There is no operation permission problem for a single user. Simple file storage does not involve connection (relationship between Linux-like operating system files) issues. Therefore, operation functions related to directory operations, operation permissions, and file connections can be deleted from the system.
Include in yaffsfs.c and its header files (omitting the yaffs_ prefix): readlink(), DumpDir(), readdir(), opendir(), lstat(), stat(), freespace(), chmod(), mkdir (), rename(), link(), closedir(), FollowLink(), fstat(), listclear(), fchmod(), sylink() and mknod().
Include in yaffs_guts.c and its header file (omitting the yaffs_ prefix): Renameobject(), mknodedirectoty(), mknodSymLink(), mknodSpecial(), Link(), GetAttributes(), GetSymLinkAlias(), root(), LostNFound (), GutsTest(), DumpObject(), GetNumberofFreeChunk(), GetObjectLInkCount() and GetEquivalentObject().
Then modify the data structure according to your own needs. The data structures related to the directory operations, operation permissions and file connections mentioned above (such as Uid, Gid, nlink, etc.) are meaningless to us, so we need to modify the relevant data structure. In order to save memory, some macro-defined data constants need to be modified, such as the number of handles running at the same time and the maximum length of the file name.
The cutting work is best carried out on a machine equipped with a Linux operating system. You can use simulation while cutting to check whether the functions you need can be achieved.
3.2 Conversion to C51 style
The YAFFS file system is developed in the Linux environment using the C language for developing user programs. It has some differences from C51, mainly including:
◇ C51 does not support the __inline__ function modifier, and its macro can be defined as empty;
◇ u8, u16, and u32 all need to be re-macro-defined as C51 data types unsigned char, unsigned int, and unsigned long;
◇ off_t is defined as long.
In the YAFFS source code, data and bit are used as variables, but in C51 these are keywords and must be replaced.
In the definition of the yaffs_Device structure in the YAFFS source code, a function pointer with parameters is used to operate the Flash hardware by calling the function pointed to by the pointer. However, in C51, calling the function through the register function pointer cannot pass the actual parameters unless the obtained Parameters can be passed between registers. Therefore, the function pointer in the yaffs_Device structure definition is removed here, and the Flash interface function in yaffs_if.c is directly called.
When converting to C51 style, it is best to modify and compile in the Keil integrated development environment, and then modify after errors are found. When the compiler prompts that there are multiple errors, start correcting the first error; it may be that after the previous error is corrected, the following errors will no longer be errors.
4 YAFFS usage examples under C51 system
The following program code is the key code for operating DBF database files in the design.
yaffs_StartUp(); //Set some parameters, including the addresses of the start block and end block of each partition in Flash, the number of reserved blocks, etc.
yaffs_mount("/flash"); //YAFFS supports multiple partitions, choose to mount here Load the /flash partition and create a new file db1.dbf
f=yaffs_open("/flash/db1.dbf", O_CREAT,S_IREAD | S_IWRITE);
yaffs_close(f);//Close the file db1.dbf
f=yaffs_open("/flash/db1 .dbf", O_RDWR,0);//Open the file db1.dbf in read/write mode
r=yaffs_write(f,"hello",5);//Write data to the file
yaffs_lseek(f,2,SEEK_SET); //Move the file read/write pointer
r=yaffs_write(f,"world",5);
r=yaffs_lseek(f,0,SEEK_SET);
r=yaffs_read(f,buffer,10);//Read data from the file
r=yaffs_close(f);//Close the file db1.dbf
r=yaffs_unlink("/flash/db1.dbf");//Delete the file db1.dbf
It can be seen that the use of the YAFFS interface function is very similar to the file operation function in the standard C language, which is simple and easy to use.
5 Summary
The YAFFS file system is the first embedded file system written specifically for NAND Flash memory. It implements power-down protection, fatigue balancing and effective garbage collection. Compared with JFFS, it takes up less resources and runs faster. Compared with FAT, it is more suitable for managing NAND Flash data memory. If you need to implement a Flash file system in a C51 system, transplanting YAFFS is a good choice, but after all, it is developed under Linux on a 32-bit machine. To make it perfectly combine with the C51 style of an 8-bit machine, it is also a good choice. Further efforts are needed.
References
[1] Samsung Corporation.? K9F5608DataSheet. 20031217.
[2] Mao Yongqiang, Huang Guangming. Implementation of YAFFS file system on embedded Linux. Electronic Design Applications, 2006(3).
Previous article:Implementing YAFFS file system on C51 system
Next article:Application of AT89C51 microcontroller in wireless data transmission
Recommended ReadingLatest update time:2024-11-16 16:43
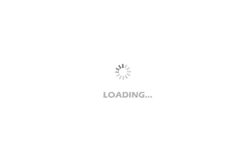
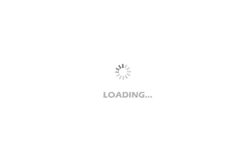
- Popular Resources
- Popular amplifiers
-
Semantic Segmentation for Autonomous Driving: Model Evaluation, Dataset Generation, Viewpoint Comparison, and Real-time Performance
-
Monocular semantic map localization for autonomous vehicles
-
Improving 3D NAND performance, reliability and yield
-
Machine Learning and Embedded Computing in Advanced Driver Assistance Systems (ADAS)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- nF capacitor and 1M resistor in parallel between the metal case and GND
- Do you know about Allegro's latest solution for vehicle electrification and emission control 48V system?
- 【CH579M-R1】+ Bluetooth function key preliminary test
- Angle estimation problem of micro-core sliding film observer SMO
- BPM shortwave receiver module
- DAC8552 of MSP430F5529 microcontroller
- Several important questions about Zigbee
- Where can I change the component description in the AD printed circuit board BOM?
- CY8CKIT-149 PSoC 4100S PLUS PROTOTYPING KIT Resources
- [ESP32-Audio-Kit Audio Development Board] - 1: Blink (esp-idf)