In the programming of Siemens S7-300 and S7-400, it is often necessary to call some system functions or function blocks. When inputting parameters, you often encounter pointer type parameters. Do you know the pointer type? The first time I came into contact with the term pointer was when I was learning C language. Pointers and linked lists are a key point in C language. In C language, pointers are memory addresses, and pointers in Siemens PLC also refer to addresses.
Let's take a look at the structure of the Siemens POINTER type:
The parameter type POINTER stores the following information:
DB number (or 0 if the data is not stored in a DB)
· Memory area in the CPU (the following table gives the hexadecimal code for the memory area of parameter type POINTER)
Hexadecimal code | Storage Area | describe |
b#16#81 | I | Input area |
b#16#82 | Q | Output area |
b#16#83 | M | Bit storage area |
b#16#84 | DB | Data Block |
b#16#85 | FROM | Instance data block |
b#16#86 | L | Local data (L stack) |
b#16#87 | V | Previous local data |
The address of the data (in byte.bit format)
STEP 7 provides the pointer format: p#memory_area byte.bit_address. (If the formal parameter is declared as parameter type POINTER, you only need to indicate the memory area and address. STEP 7 will automatically reformat the input pointer.) The following example shows how to enter the parameter type POINTER for data starting with M50.0: P#M50.0
Memory Indirect Addressing:
A program statement using memory indirect addressing consists of an instruction followed by an [address] identifier and finally an (address must be enclosed in square brackets). Depending on the address identifier used, the instruction interprets the data stored at the specified address as a word or double word pointer. The complete data address consists of the address identifier and the pointer, as shown in the following example. The advantage of indirect addressing is that the data address of an instruction can be modified dynamically during program execution.
Memory indirect addressing uses the following two parts of the address:
1. Address Identifier
To bits addressed by bit logic operations, the address identifiers I, Q, M, L, DIX or DBX can be assigned.
For bytes, words, and double words addressed by load instructions, storage areas I, Q, M, L, D, and PI can be used, and address identifiers in the form of IB, IW, ID, DBB, DBW, DBD, DIB, DIW, DID, PIB, PIW, PID, etc. can be assigned.
For bytes, words, and double words addressed by transfer instructions, storage areas I, Q, M, L, DB, DI, and PQ can be used, and address identifiers in the form of IB, IW, ID, DBB, DBW, DBD, DIB, DIW, DID, PQB, PQW, PQD, etc. can be assigned.
To address timers, counters or blocks, use area identifiers of the form T, C, DB, DI, FB, FC.
2. The address of a word or double word pointer enclosed in square brackets "[ ]"
Word pointer - A word that contains the identification number of a timer (T), counter (C), data block (DB, DI) or logic block (FC, FB). A word pointer is a decimal integer.
Dword pointer - Refers to a double word that contains the exact location of a bit, byte, word, or double word. The format of a double word pointer is: P#byte.bit. The pointer must be stored in one of the following areas to perform memory indirect addressing:
M - Bit Memory
L - Local Data
D - Data Block (DB or DI)
STAT Static data (not for blocks with multiple instance capability)
Note If you want to address a byte, word, or double word using memory indirect addressing, make sure the pointer bit number is double word format 0.
Word pointer example:
L 5 //Load pointer value into ACCU 1. T MW2 //Transfer pointer to MW2.
LT[MW2] //Load the current time value of timer 5 into ACCU 1.
LC[MW2] //Load the current count value of counter 5 into ACCU 1.
OPN DB[MW2] //Open data block DB5 as a shared data block.
OPN DI[MW2] //Open data block DB5 as background data block.
Double word pointer example:
LP#8.7 //Load pointer value into ACCU 1. T MD2 //Transfer pointer to MD2. AI [MD2] //Scan the state of input bit 8.7 and assign it = Q [MD2] //Signal state to output bit Q 8.7.
Intra-region register indirect addressing:
A program statement using indirect addressing with a local register contains an instruction and the following components: address identifier [address register identifier, address]. Indirect addressing with a local register uses the following two parts of the address:
1. Address Identifier
To bits addressed by bitwise logic operations, the address identifiers I, Q, M, L, DIX or DBX can be assigned.
For bytes, words, and double words addressed by load instructions, storage areas I, Q, M, L, D, and PI can be used, and address identifiers in the form of IB, IW, ID, DBB, DBW, DBD, DIB, DIW, DID, PIB, PIW, PID, etc. can be assigned.
For bytes, words, and double words addressed by transfer instructions, storage areas I, Q, M, L, DB, DI, and PQ can be used, and address identifiers in the form of IB, IW, ID, DBB, DBW, DBD, DIB, DIW, DID, PQB, PQW, PQD, etc. can be assigned.
2. The contents enclosed in square brackets "[ ]" include the address register reference (AR1 or AR2), the comma separator "," and the double-word pointer.
Dword pointer - Refers to a double word containing a bit, byte, word, or partial address of a double word. The format of a double word pointer is: P#byte.bit.
Note Remember that you are now using two pointers of the format "P#byte.bit". One pointer is already specified. The other pointer is determined by referencing the address register AR1 or AR2. If you want to address a byte, word, or double word, make sure the pointer bit number is 0. Statements using register indirect addressing do not change the contents of the address register.
Pointer example:
LP#8.7 //Load pointer value into ACCU 1. LAR1 //Load AR1 with pointer in ACCU 1. AI [AR1, P#0.0] //Check input bit I 8.7 and assign signed state to Q 10.0. = Q [AR1, P#1.1] //The exact address 8.7 is in AR1. The offset does not affect it. The exact location 10.0 is calculated by adding 8.7 (AR1) to 1.1 (offset), which is 10.0 instead of 9.8.
Indirect addressing example of registers within a region:
A I [AR1,P#4.3] Performs a logical AND operation on the input bit whose position is calculated by adding 4 bytes to the contents of AR1, plus 3 bits. = DIX [AR2, P#0.0] Assigns the RLO bit state to the instance data bit located in AR2. L IB [AR1,P#10.0] Loads the input byte into ACCU 1. The address is calculated by adding ten bytes to the contents of AR1. T LD [AR2,P#53.0] Transfers the contents of ACCU 1 to a local double word located by adding 53 bytes to the contents of AR2.
The characteristics of indirect addressing of registers within the area are: the address identifier is determined before the square brackets, and the pointers in the square brackets do not contain storage area information (such as AR1=P#8.7 in [AR1, P#4.3], and both pointers do not contain storage area information), otherwise it will conflict with the storage area represented by the address identifier before the square brackets.
Cross-region register indirect addressing:
A program statement using cross-region register indirect addressing consists of an instruction and the following components: address identifier [address register identifier, address].
Cross-region register indirect addressing uses the following two parts of the address:
1. Specification of the size of the addressed data object (address identifier) Data object size specification Bit (no specification means one bit) B Byte W Word D Double word
2. The content within the square brackets "[ ]" includes the address register reference (AR1 or AR2), the comma separator "," and the double word pointer. Double word pointer - refers to a double word containing a partial address of a bit, byte, word, or double word. Pointers have the following in-area format: P#byte.bit.
Note that the cross-area doubleword pointer must have been loaded into the address register referenced by the register indirect address beforehand. Cross-area doubleword pointer - A doubleword containing a partial address of a bit (for bit logic instructions) or a partial address of a byte, word or doubleword (for load and transfer instructions). The area identifier preceding the address is used to specify bytes and bits. The format of a cross-area doubleword pointer is: P#area identifier byte.bit.
To the bits addressed by the bit logic instructions, you can assign the cross-area pointer area identifiers I, Q, M, DIX or DBX.
For bytes, words and double words addressed by load or transfer instructions, you can assign cross-area pointers the area identifiers I, Q, M, DIX, DBX or P. Note To specify a peripheral input or PI area in a pointer, enter the pointer in the form P#Px.y. The area is specified as P. You cannot use a peripheral output PQ area in a cross-area pointer. Remember that you are using two pointers:
As an offset, a double word pointer directly into the area indicated in the address, for example P#4.0.
A cross-region double-word pointer stored in an address register (AR1 or AR2), for example, P#Q4.0.
If you want to access a byte, word, or double word addressed by direct addressing, make sure that both pointers have bit numbers 0. Statements using register indirect addressing do not change the contents of the address register.
The first example of cross-region register indirect addressing:
LP# I8.7 //Load the pointer value and area identifier into ACCU 1. LAR1 //Store bank I and address 8.7 into AR1. LP# Q8.7 //Load the pointer offset and area identifier into ACCU 1. LAR2 //Store bank Q and address 8.7 into AR2. A [AR1, P#0.0] //Check input bit I 8.7 and assign its signal state to output bit Q 10.0. = [AR2, P#1.1] //Offset 0.0 has no effect. Output bit 10.0 is calculated by adding 8.7 (AR2) to 1.1 (offset), which is 10.0 instead of 9.8. Second example of cross-bank register indirect addressing:
Previous article:What are the PLC industrial control configuration software?
Next article:Main anti-interference measures for PLC controller
Recommended ReadingLatest update time:2024-11-16 09:49
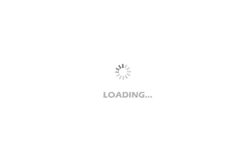
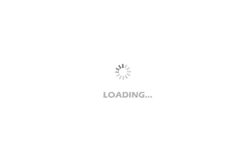
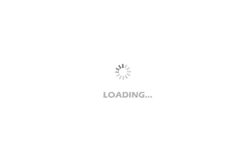
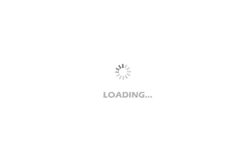
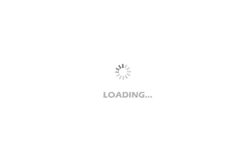
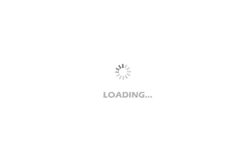
- Popular Resources
- Popular amplifiers
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- July 21 Live Replay: Microchip Timberwolf Audio Processor Online Seminar (including video, PPT, QA)
- MSP430 FAQ: FLASH storage
- [RISC-V MCU CH32V103 Review] Driving OLED Display
- Experience in using TI DSP/BIOS and CCS
- Four-layer PCB proofing matters needing attention
- Why your 4.7uF capacitor becomes 0.33uF, it's incredible!
- Industry 4.0 has a bright future, but the road is tortuous. Download the "Fluke Industrial Automation Industry Application Product Manual" to learn more!
- The relationship between capacitance and frequency
- Help: "There are so many types of push button switches. Will choosing one have any impact on the physical hardware design?"
- About the efficiency and memory usage of CCS9 floating point operations