1. Measurement Schematic Diagram
Description K1 K2 K3 are the settings of the LCD module. K1 is the WDT selection switch. When the switch is closed, WDT is turned on. Otherwise, WDT is turned off
. K2 is the TONE selection switch. When the switch is closed, audio output is turned on. Otherwise, it is turned off. K3 is the LCD module oscillator selection switch. When K3 is closed, external 32.768K crystal oscillator is selected. Otherwise, internal RC oscillator 256K is selected. KEY is the confirmation key used to loop the measurement process. LED is the indicator light for the measured value to be readable. When the MCU has finished setting the LCD module, the IO port P0.1 P0.3 P0.5 of the interface will be set to high impedance state, and then the LED will be lit to indicate that the measured value is readable.
Measurement method: Select the working mode of the LCD module by K1 K2 K3, and then press the KEY key and wait for the LED to light up. Observe
the LCD. If the LCD is fully displayed, the current measured by the ammeter is the current of the current setting working mode. Press the KEY key again, and the LCD will be completely off. At this time, the current measurement value is the current in the power-off mode.
2 Source program
/********************************************************
DLCD.c
This program is used to debug and measure the parameters of the water meter LCD module of our company. It can measure
the power consumption of the LCD module in full display mode, the power consumption in power-off mode WDT and the power consumption during audio output.........
**********************************************************/
#include
#define uint unsigned int
/*
Set LCD module interface port parameters
for
SPI.h
*/
sbit CS_PORT=P0^1; /*CS port*/
sbit CLK_PORT=P0^3; /*WR port*/
sbit SDA_PORT=P0^5; /*DATA port*/
#include /*uchar has been defined in the SPI.h file*/
/*Mode selection switch definition*/
sbit K1=P0^0; /*WDT on/off selection*/
sbit K2=P1^6; /*Audio output on/off selection*/
sbit K3=P1^4; /*Internal or external crystal selection*/
sbit KEY=P1^1; /*'Confirm' button*/
sbit LED=P0^7; /*Readable indicator light*/
/*Command word definition The command word is the lower 8 bits of 'command mode'*/
#define BIAS 0X52 /*Define 1 3 Bias 4 Back Pole*/
#define XTAL32 0X28 /*Use external crystal*/
#define RC256 0X30 /*Use internal 256KRC oscillator*/
#define SYSEN 0X02 /*Turn on oscillator generator*/
#define LCDON 0X06 /*Turn on LCD*/
#define SY
SDI
S 0X00 /*Turn off oscillator generator*/
#define LCDOFF 0X04 /*Display off*/
#define TONE4 0X80 /*Set BZ output frequency to 4K*/
#define TONEON 0X12 /*Turn on BZ audio output*/
#define TONEOFF 0X10 /*Turn off BZ audio output*/
#define CLRWDT 0X1c /*Clear WDT*/
#define F1 0X40 /*WDT set to 4 seconds overflow*/
#define IRQEN 0X10 /*IRQ output disabled*/
#define IRQDIS 0X00 /*IRQ output enabled*/
#define WD
TE
N 0X0e /*Turn on WDT*/
#define WDTDIS 0X0a /*Turn off WDT*/
#define
TI
MERDIS 0X08 /*Turn off time base output*/
/****************************************************************
Delay function
Function prototype: void Delay()
Usage: Delay of SPI operation
**************************************************************/
void Delay()
{
uchar i;
for(i=0;i<10;i++); /*Used to adjust CLK pulse width*/
}
/*********************************************************
Long delay function
Function prototype: void Delay1s()
Usage: long delay.....
******************************************************/
void Delay1s()
{
uchar i;
uint j;
for(i=0;i<10;i++)
for(j=0;j<1300;j++);
}
/*********************************************************
Send command function class A
Function prototype: void SEN
DC
OMA(uchar com)
Use: When sending a command to HT1621, you must first send the ID value, and the command word is used to set the HT1621.
*********************************************************/
void SENDCOMA(uchar com)
{
Start_spi();
SendBit(0X80,4); /*Send setting command ID=100 0*/
SendByte(com); /*Send command word*/
}
/**********************************************************
Send command function class B
Function prototype: void SENDCOMB(uchar
ad
r)
Use: When sending a command to HT1621, you must first send the ID value Value, then send the starting address of the data to be written,
used for
RAM
write operation (data can be sent after calling this function) adr is the high 5 bits valid
**********************************************************/
void SENDCOMB(uchar adr)
{
Start_spi();
SendBit(0XA0,4); /*Send write display RAM command ID=101 0*/
SendBit(adr,5); /*Specify write address*/
}
/************************************************************
Send command function (C class)
Function prototype: void SENDCOMC(uchar com)
Usage: When sending a command to HT1621, you must first send the ID value. Then send the C class command word
*********************************************************/
void SENDCOMC(uchar com)
{
Start_spi();
SendBit(0X90,4); /*Send command ID=100 1*/
SendByte(com); /*Send command word*/
}
/********************************************************
Fill the display buffer
Prototype: void disp(ucahr dat)
Function: Fill the display buffer and display data in the simplest form
*******************************************************/
void disp(uchar dat)
{
uchar i;
SENDCOMB(0x00); /*Set the data pointer back to 0 and then write data*/
for(i=0;i<16;i++) /*Write 16 bytes of data*/
{
SendByte(dat); /*Write data*/
}
}
/**********************************************************
Full display current measurement
Optional components WDT Audio output Internal RC External crystal selection
Time base output closed
**********************************************************/
void DISP_ALL()
{
/*Display
Chip
Initialization is also the LCD module power-down wake-up procedure*/
SENDCOMA(BIAS); /*Set bias voltage, back pole number*/
/*Select crystal type*/
K3=1;
if(K3==1)SENDCOMA(RC256); /*Set to internal crystal 256K*/
else SENDCOMA(XTAL32);
/*Start LCD oscillator*/
SENDCOMA(SYSEN); /*Start oscillator*/
Delay1s();
SENDCOMA(LCDON); /*Display enable*/
SENDCOMA(TIMERDIS); /*Disable time base output*/
/*
Watchdog
selection*/
K1=1;
if(K1==0)
{
SENDCOMC(F1); /*WDT set to 4S*/
SENDCOMA(WDTEN); /*Open WDT*/
SENDCOMA(CLRWDT); /*Clear WDT*/
SENDCOMC(IRQEN); /*Turn on IRQ*/
}
else
{
SENDCOMC(IRQDIS); /*Disable IRQ*/
SENDCOMA(WDTDIS); /*Disable WDT overflow flag output*/
}
/*Audio output selection*/
K2=1;
if(K2==0)
{
SENDCOMA(TONE4); /*Audio output is set to 4KHz*/
SENDCOMA(TONEON); /*Turn on audio output*/
}
else SENDCOMA(TONEOFF); /*Otherwise turn off audio output*/
disp(0xff); /*Output full display data*/
PT0AD=0x3e; /*SPI interface digital input function disabled*/
P0M1=P0M1|0x3e; /*Set SPI port to input only P0M2 corresponding bit is already 0*/
LED=1; /*Indicates that the measured value is readable*/
KEY=1; /*Wait for the 'confirm' button*/
while(KEY==1);
P0M1=P0M1&0xc1; /*Set SPI port as bidirectional port*/
PT0AD=0x00;
LED=0; /*Turn off LED indication*/
while(KEY==0);
}
/************************************************************
Power-off measurement part
First turn off the audio output and then enter the power-off mode
After power-off, the LCD interface is set to high-impedance state. If the IO port is not in high-impedance mode, it should be set to 1
********************************************************/
void DISP_OFF()
{
SENDCOMA(TONEOFF); /*Audio output disabled*/
Delay1s();
SENDCOMA(LCDOFF); /*Display disabled*/
Delay1s();
SENDCOMA(SYSDIS); /*Stop oscillator*/
P0M1=P0M1|0x3e; /*Set SPI port as input only*/
PT0AD=0x3e; /*SPI interface digital input function disabled*/
LED=1; /*Indicates that the power-off current measurement value can be read*/
KEY=1;
while(KEY==1);
P0M1=P0M1&0xc1; /*Set the SPI port to a bidirectional port*/
PT0AD=0x00;
LED=0;
while(KEY==0);
}
/****************************************************
Main function
Prototype: void main()
Function: Continuously query KEY. If there is a key, perform full display measurement state. If the key is pressed again, enter
Power-off measurement state and cycle measurement in sequence
**********************************************************/
void main()
{
P0M1=P0M1&0x7f; /*Set the LED port to pull-up output*/
P0M2=P0M2|0x80;
while(1)
{
LED=0; /*Turn off the indicator light*/
DISP_ALL(); /*Full display measurement*/
DISP_OFF(); /*Power-off measurement*/
}
}
Header file SPI.h
/************************************************************
SPI.h
This header file is some basic functions for reading and writing SPI. It is used for the LCD module of our company's water meter
Driver debugging
********************************************************/
#define uchar unsigned char
extern void Delay(); /*Delay program of device driver*/
/*************************************************************/
Name: Send data bit
Prototype: void SendBit(uchar dat,uchar bitcnt)
Purpose: Send the bitcnt data of dat to SPI starting from the high bit. (bitcnt cannot be greater than 8)
/***************************************************************/
Previous article:LCD TV System Solutions
Next article:Design of plasma color TV based on Genesis gm5020 chip
Recommended ReadingLatest update time:2024-11-16 11:33
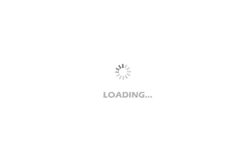
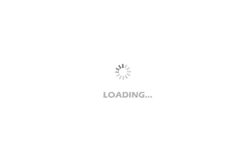
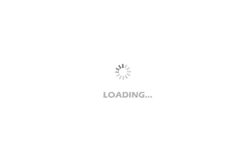
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
- Sn-doped CuO nanostructure-based ethanol gas sensor for real-time drunk driving detection in vehicles
- Design considerations for automotive battery wiring harness
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 【RT-Thread Reading Notes】——The harder you work, the luckier you will be
- DSP2812 FIFO usage
- Getting Started with I2C on MSP MCUs
- Question sharing: What is the difference between AFE and battery monitor in battery management chip?
- Qorvo's Response to WiFi 6
- The best video to introduce you to Matter is here! Check it out now.
- Design summary of RGB to VGA module
- About connecting Raspberry Pi to SensorTile.box via Bluetooth
- Last day! ST live broadcast with prizes | ST60 contactless connector and application exploration
- Playing with Zynq Serial 10 - Using GIT for Project Backup and Version Management 2