Introduction
They are all 8-bit MCUs derived from 8051. STC MCUs have several major series: 89/90/10/11/12/15. The characteristics of each series are as follows
The 89 series is a traditional 8051 microcontroller. The burning method is different, but the function is compatible with the AT89 series. It belongs to the 12T microcontroller.
The 90 series is an improved version of the 89 series, 12T single chip microcomputer
The 10 and 11 series are 1T MCUs with PWM, 4-state IO interface, EEPROM and other functions, but they do not have ADC
The 12 series is a 1T MCU with enhanced functions. The model with the suffix AD has ADC, and the model with the suffix S2 has ADC and dual serial ports.
The 15 series is the latest product, with a high-precision R/C clock integrated inside, and no external crystal oscillator is required.
STC89C52 Parameters
The difference between STC89C52 and STC89C51 is the Flash size, C51 is 4K and C52 is 8K. Both have multiple packages, the most common one is the larger DIP40 wide body dual inline
Flash: 8K bytes
RAM: 512 bytes
Built-in 4KB EEPROM
32-bit I/O
Watchdog timer, MAX810 reset circuit
Three 16-bit timers
4 external interrupts, a 7-vector 4-level interrupt structure and full-duplex serial port
Maximum operating frequency 35MHz, 6T/12T optional
VCC is 5V, do not exceed this voltage.
If you need ADC, you can choose STC12C5A60S2 series. The instruction clock speed of 1T type is faster than that of ordinary STC89 series (12T). Please pay attention to it when doing timing.
Hardware Preparation
Use the common C51 minimum development board
A USB2TTL adapter card
LED + 1K current limiting resistor for viewing output
Development under Ubuntu 20.04
Software
Compilation tool SDCC http://sdcc.sourceforge.net/
Burning tool stcgal https://github.com/grigorig/stcgal
(Optional) Package library HML_FwLib_STC89 https://github.com/MCU-ZHISHAN-IoT/HML_FwLib_STC89
Install sdcc
The sdcc version that comes with Ubuntu 20.04 is 3.8.0, so I plan to compile and install it myself. First read the installation instructions of sdcc at http://sdcc.sourceforge.net/doc/sdccman.pdf
Go to https://sourceforge.net/projects/sdcc/files/sdcc/4.1.0/ to download the latest 4.1.0 source code.
Unzip tar -xvjf sdcc-src-4.1.0.tar.bz2
During the configuration ./configure, it prompted that gputils needed to be installed. I installed it through apt install gputils. There was no error in the ./configure again, but it prompted that this gputils was relatively old and not supported by some devices. Ignore it for now
Compile make, and it prompts that texinfo is missing. Install it through apt install texinfo, and then make again. It should be said that the compilation time is very long. If you only need to compile C51, you can disable some other unnecessary chips during configuration.
Installsudo make install
Check and test the installed sdcc
Run sdcc --version to see if the output is correct, and run sdcc --print-search-dirs to see the location of the library file. The default location of the 8051 library file is /usr/local/share/sdcc/include/mcs51/
Write a piece of code test001.c,
char test;
void main(void) {
test = 0;
}
Compile with sdcc. If the generated result file is normal and there is no error, it means that the installation is fine.
# This step will generate test001.asm and other files
sdcc -c test001.c
# This step will generate test001.ihx and other files
sdcc test001.c
Install stcgal
According to the instructions on the project homepage on Github, install it directly using pip3 install stcgal, and then execute stcgal -h to view the output
Write code and compile with sdcc
Write a test002.c, and control the duty cycle to make the LED on P0.7 produce a breathing light effect.
8052.h is the generic C52 header file that comes with sdcc, not the custom header file for STC89Cxx
Need STC header file, corresponding to STC89C52, you can use stc89.h; corresponding to STC12C5A60S2, you can use stc12.h
#include <8052.h>
#define Led10 P0_7
typedef unsigned int u16;
int atime = 64;
void delay(u16 pms) {
u16 x, y;
for (x=pms; x>0; x--) {
for (y=11; y>0; y--);
}
}
void ledfade(u16 i) {
Led10 = 0;
delay(i);
Led10 = 1;
delay(atime-i);
}
int main(void) {
u16 a, b;
while(1) {
for (a=0; a ledfade(a); } } for (a=atime; a>0; a--) { for (b=0; b < (atime - a)/4; b++) { ledfade(a); } } } } There are two ways to compile Add -m Without parameters, specify the path in the header file, for example #include This is how you use the parameters sdcc -mmcs51 test002.c Burn Burn using stcgal, this page has detailed instructions For STC89C52 use -P stc89, for STC12C5A60S2 series use -P stc12 stcgal -P stc89 test002.ihx Waiting for MCU, please cycle power: done # Here you need to turn off and then on the power Target model: Name: STC89C52RC/LE52R Magic: F002 Code flash: 8.0 KB EEPROM flash: 6.0 KB Target frequency: 11.010 MHz Target BSL version: 3.2C Target options: cpu_6t_enabled=False bsl_pindetect_enabled=False eeprom_erase_enabled=False clock_gain=high ale_enabled=True xram_enabled=True watchdog_por_enabled=False Loading flash: 243 bytes (Intel HEX) Switching to 19200 baud: checking setting testing done Erasing 2 blocks: done Writing flash: 640 Bytes [00:00, 1844.10 Bytes/s] Setting options: done Disconnected! It will automatically run after burning. The default baud rate is 19200, which is slow to write. For STC89C52RC, you can directly use the baud rate of 115200, specify it with the -b parameter stcgal -P stc89 -b 115200 test002.ihx If there are multiple com ports at the same time, use -p to specify the port stcgal -P stc89 -b 115200 dist/89/89.hex -p /dev/ttyUSB1 Use the -D parameter to display the interactive information of the serial port. stcgal -D -P stc89 -b 115200 test002.ihx Waiting for MCU, please cycle power: <- Packet data: 46 B9 68 00 1C 00 0A 76 0A 72 0A 76 0A 72 0A 76 0A 72 0A 76 0A 72 32 43 FD F0 02 82 5A 16 done Target model: Name: STC89C52RC/LE52R Magic: F002 Code flash: 8.0 KB EEPROM flash: 6.0 KB Target frequency: 11.010 MHz Target BSL version: 3.2C Target options: cpu_6t_enabled=False bsl_pindetect_enabled=False eeprom_erase_enabled=False clock_gain=high ale_enabled=True xram_enabled=True watchdog_por_enabled=False Loading flash: 337 bytes (Intel HEX) Switching to 115200 baud: checking -> Packet data: 46 B9 6A 00 0C 8F FF FD 00 06 A0 81 28 16 <- Packet data: 46 B9 68 00 0C 8F FF FD 00 06 A0 81 26 16 setting -> Packet data: 46 B9 6A 00 0B 8E FF FD 00 06 A0 A5 16 <- Packet data: 46 B9 68 00 0B 8E FF FD 00 06 A0 A3 16 testing -> Packet data: 46 B9 6A 00 0C 80 00 00 36 01 F0 02 1F 16 <- Packet data: 46 B9 68 00 06 80 EE 16 -> Packet data: 46 B9 6A 00 0C 80 00 00 36 01 F0 02 1F 16 <- Packet data: 46 B9 68 00 06 80 EE 16 -> Packet data: 46 B9 6A 00 0C 80 00 00 36 01 F0 02 1F 16 <- Packet data: 46 B9 68 00 06 80 EE 16 -> Packet data: 46 B9 6A 00 0C 80 00 00 36 01 F0 02 1F 16 <- Packet data: 46 B9 68 00 06 80 EE 16 done Erasing 2 blocks: -> Packet data: 46 B9 6A 00 0D 84 02 33 33 33 33 33 33 2F 16 <- Packet data: 46 B9 68 00 06 80 EE 16 done -> Packet data: 46 B9 6A 00 8C 00 00 00 00 00 00 80 02 00 06 02 00 AC 75 81 09 12 01 4D E5 82 60 03 02 00 03 79 00 E9 44 00 60 1B 7A 00 90 01 51 78 01 75 A0 00 E4 93 F2 A3 08 B8 00 02 05 A0 D9 F4 DA F2 75 A0 FF E4 78 FF F6 D8 FD 78 00 E8 44 00 60 0A 79 01 75 A0 00 E4 F3 09 D8 FC 78 00 E8 44 00 60 0C 79 00 90 00 01 E4 F0 A3 D8 FC D9 FA 75 08 40 75 09 00 02 00 03 AE 82 AF 83 EE 4F 60 14 7C 0B 7D 00 1C BC FF 01 1D EC 4D 70 F7 1E BE FF FB 16 <- Packet data: 46 B9 68 00 07 80 85 74 16 Writing flash: 0%| | 0/512 [00:00, ? Bytes/s]-> Packet data: 46 B9 6A 00 8C 00 00 00 00 80 00 80 01 1F 80 E8 22 AE 82 AF 83 C2 87 8E 82 8F 83 C0 07 C0 06 12 00 68 D0 06 D0 07 D2 87 AC 08 AD 09 EC C3 9E F5 82 ED 9F F5 83 02 00 68 7E 00 7F 00 AC 08 AD 09 C3 EE 9C EF 9D 50 45 7C 00 7D 00 AA 08 AB 09 EA C3 9E FA EB 9F C3 13 CA 13 CA C3 13 CA 13 CA FB C3 EC 9A ED 9B 50 1E 8E 82 8F 83 C0 07 C0 06 C0 05 C0 04 12 00 85 D0 04 D0 05 D0 06 D0 07 0C BC 00 C9 0D 80 C6 0E BE 00 B3 0F 80 B0 14 16 <- Packet data: 46 B9 68 00 07 80 1E 0D 16 -> Packet data: 46 B9 6A 00 8C 00 00 00 01 00 00 80 AE 08 AF 09 EE 4F 60 A4 7C 00 7D 00 AA 08 AB 09 EA C3 9E FA EB 9F C3 13 CA 13 CA C3 13 CA 13 CA FB C3 EC 9A ED 9B 50 1E 8E 82 8F 83 C0 07 C0 06 C0 05 C0 04 12 00 85 D0 04 D0 05 D0 06 D0 07 0C BC 00 C9 0D 80 C6 1E BE FF 01 1F 80 B7 75 82 00 22 FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF AD 16 <- Packet data: 46 B9 68 00 07 80 36 25 16 Writing flash: 75%|█████████████████████████▌ | 384/512 [00:00<00:00, 3814.67 Bytes/s]-> Packet data: 46 B9 6A 00 8C 00 00 00 01 80 00 80 FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF FF 77 16 <- Packet data: 46 B9 68 00 07 80 80 6F 16 Writing flash: 640 Bytes [00:00, 4207.06 Bytes/s] Setting options: -> Packet data: 46 B9 6A 00 0A 8D FD FF FF FF FB 16 <- Packet data: 46 B9 68 00 0A 8D FD FF FF FF F9 16 done -> Packet data: 46 B9 6A 00 06 82 F2 16 Disconnected! Serial port under SDCC Open the serial port and redirect putchar() to the serial port For STC89C52 using 11.0592 crystal When using T1, the maximum baud rate can only reach 28800 without doubling, and 57600 after doubling When using T2 as a baud rate generator, the increment frequency is 2 times the crystal frequency, which can reach 115200.
Previous article:51 MCU package library HML_FwLib_STC89/STC11
Next article:Multi-channel PWM pulse width modulation output of 51 single chip microcomputer (STC89C52)
Recommended ReadingLatest update time:2024-11-16 17:42
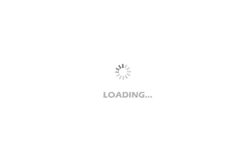
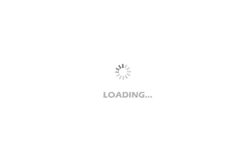
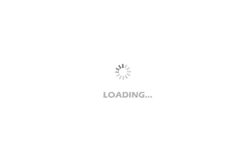
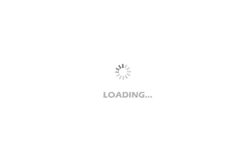
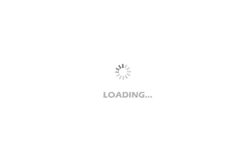
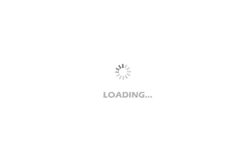
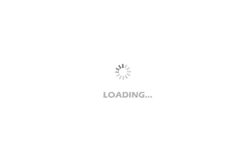
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Fundamentals and Applications of Single Chip Microcomputers (Edited by Zhang Liguang and Chen Zhongxiao)
-
Single chip microcomputer control technology (Li Shuping, Wang Yan, Zhu Yu, Zhang Xiaoyun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Maxim's MAX15066 high-efficiency DC-DC solution
- Frequency converter, inverter circuit
- 【RPi PICO】Soil Moisture Indicator
- Gaoyun Yunyuan software edits multiple lines at the same time, and the problem of Chinese input method
- Raspberry Pi Windows IoT Development (Part 3) Flashing LED
- If you are doing technology, you must learn to read datasheets!
- Programming the pyboard with Arduino
- 【Silicon Labs Development Kit Review】+ Try running the light-up demo
- [GD32E231 DIY Contest] (II): USART0
- The microcontroller reads PT1000 temperature. Please recommend a more stable conversion chip