Introduction to timers, static digital tube displays, logical operators and logic circuit symbols
1. Logical operations and logic circuits
Introducing the concept of logic: In life, logic has two logical values, 'true' and 'false', and logic corresponds to C language and digital circuits. 'True' corresponds to all 'non-zero values' in C language or digital circuits, and 'false' corresponds to 'zero value'.
1.1 C language logical operators
Assume two byte variables A and B, and the result of a certain logical operation between them is F.
The following are logical operators (operating on the overall value of the variable)
&& Logical AND: F = A && B. When the values of A and B are both true (non-zero values, the same below), the operation result is F.
is true (the specific value is 1, the same below); when any one of the values of A and B is false (that is, 0, the same below), the result F is false (the specific value is 0, the same below).
|| Logical OR: F = A || B, if either A or B is true, F is true; if both A and B are false, F is false.
! Logical NOT: F = !A, if the value of A is false, F is true; if the value of A is true, F is false.
The following are bitwise operators (operating on each bit of the variable)
& Bitwise AND: F = A & B, perform AND operation on each bit in the two bytes A and B, and combine each bit result into the total result F. For example, A = 0b00111100, B = 0b11011101, then the result F is equal to 0b00011100.
| Bitwise OR: F = A | B, for example, A = 0b00111100, B = 0b11011101, F is equal to 0b11111101.
~ Bitwise inversion: F = ~A, which means negating each bit in byte A (i.e. inverting it). For example, if A = 0b00111100, F equals 0b11000011.
^ Bitwise XOR: XOR means that if the values of the two sides of the operation are different (that is, different), the result is true.
If they are the same, the result is false. F = A ^ B, A = 0b00111100, B = 0b11011101, the result F is equal to 0b11100001.
1.2 Logic Circuit Symbols
The following figure shows the commonly used circuit symbols for digital circuits. Focus on recognizing popular symbols abroad and remember them by looking them up.
2. Timer learning (key points, not difficult points)
2.1 Introduction to Clock Cycle and Machine Cycle
Clock cycle: It is the smallest time unit in the timing. The calculation method is: Clock cycle = 1 / clock source frequency. (Note: 1. The unit is seconds 2. The time source frequency is the crystal oscillator of the microcontroller. The crystal oscillator of the blogger's STC89C52RC crystal oscillator circuit is: 11.0592M)
Machine cycle: The shortest time for a microcontroller to complete an operation. A machine cycle of a 51 microcontroller under the standard architecture is equal to 12 clock cycles (12 / clock cycle S). For some enhanced 51 microcontrollers, a machine cycle is equal to 4 clock cycles or 1 clock cycle. The following explains the 51 microcontroller under the standard framework.
2.2 Introduction to timers
The timer and counter in the microcontroller are the same internal module. Readers can understand the counter function by themselves. Let us introduce the timer function.
Timer: used for timing. The standard 51 single-chip microcomputer has two timers, T0 and T1. There is a register inside the timer. The value of the register automatically increases by 1 every time a machine cycle passes. The machine cycle can be understood as the counting cycle of the timer. Just like a clock, the number automatically increases by 1 every second, and the register automatically increases by 1 every 12/11059200 seconds. When the clock adds 60 seconds, the second automatically jumps to 0. This situation is called overflow in single-chip microcomputers and computers. Different working modes of the timer have different overflow situations. If a 16-bit timer is added to 65535, adding 1 will overflow, and the value will directly become 0 after the overflow.
2.2.1 Timer register introduction
The timer module has 6 registers, which are introduced one by one below. Readers can check the manual for the use of these registers.
Timing value storage register
There are four registers in Table 5-1 for storing the count value of the timer, where TH0 and TL0 are used for timer 0, and TH1 and TL1 are used for timer 1.
TCON – Timer Control Register
(Note: Bit addressability means that each bit of the register can be operated separately. For example, one bit TR1 of the register can be directly operated as TR1 = 1 by the program.)
(Note: When the hardware is set to 1 or cleared to 0, it means that once the conditions are met, the microcontroller will automatically complete the action. When the software is set to 1 or cleared to 0, it means that we must use the program to complete the action.)
Table 5-2 is the bit allocation of the timer control register TCON, and Table 5-3 is a description of the specific meaning of each bit. In the TCON register, TF0 and TR0 are used for timer 0, and TF1 and TR1 are used for timer 1. Let's take timer 1 as an example. The same is true for timer 0. TR1 is equal to 1. The timer value increases by 1 every machine cycle. If it is equal to 0, it stops increasing by 1. The function of TF1 is to tell us that the timer has overflowed. For example, in the 16-bit mode of the timer, TL1 will increase by 1 every machine cycle. After adding to 255, TL1 will become 0 after adding 1 again, and TH1 will increase by 1. This cycle repeats. When TL1 and TH1 are 255, adding 1 will overflow. At this time, TL1 and TH1 will become 0, and TF1 will automatically become 1, providing readers with an overflow signal.
TMOD – Timer Mode Register
(Note: Non-bit addressability means that each bit of the register cannot be operated individually. For example, the operation of M1 (T1) = 1 in TMOD is wrong. If we want to operate, we must operate the entire byte at a time, that is, we must operate all the bits of TMOD at once)
The above table shows the bit allocation of TMOD.
Table 5-5 is the bit description of TMOD. The GATE bit is for readers to understand. The C/T bit is used to select the timer or counter function. C/T=0 selects the timer function, and C/T=1 selects the counter function.
Table 5-6 introduces the modes in TMOD4. Modes 0 and 3 are rarely used now. Readers can learn about them on their own. Modes 1 and 2 are introduced below.
Mode 1: THn and TLn form a 16-bit timer with a counting range of 0 to 65535. After overflow, as long as THn and TLn are not reassigned, the counting starts from 0.
Mode 2: It is an 8-bit automatic reload mode. Only TLn counts by adding 1. The counting range is 0 to 255. The value of THn does not change but keeps the original value. After TLn overflows, TFn is directly set to 1, and the original value of THn is directly assigned to TLn, and then TLn starts counting from the newly assigned number. For example, in this mode, TH1 = 0x66, TL1 = 0x00, TL1 adds 1 every machine cycle. When TL1 = 0xFF, TF1 will become 1 after adding 1, and TL1 will be assigned the value of TH1 and then count, that is, TL1 starts counting from 0x66, and the value of TH1 remains unchanged.
2.2.2 Timer Mode Working Circuit Logic Diagram
Timer Mode 1 Working Circuit Logic Diagram
Explanation: Take timer 1 as an example, and the same goes for timer 0. OSC is the clock source frequency, and d is the multiple of the microcontroller machine cycle to the clock cycle.
When C/T = 0, the timer function is enabled, and when C/T = 1, the counter function is enabled.
To achieve the control effect, the circuit composed of TR1 and the OR gate must be ANDed. The AND operation is true only when both are true. Therefore, TR1 must be 1, and the result of the OR operation of the circuit composed of the OR gate must be 1.
There are two ways for the OR gate to output 1: (1) When the INT1 pin is 1, no matter what the GATA bit is, the result of the OR operation is 1, and Timer 1 Mode 1 is enabled. (2) When GATA is 0, it becomes 1 after the NOT operation. No matter what the INT1 bit is, the result of the OR operation is 1, and Timer 1 Mode 1 is enabled.
Timer Mode 2 Working Circuit Logic Diagram
Explanation: The circuit logic diagram of mode 2 is similar to that of mode 1, so it will not be explained in detail. In this mode, the timer acts as an 8-bit counter (TLn) that can be automatically reloaded. When TLn overflows, it is not only set to TFn, but also the content of THn is reloaded into TLn. The content of THn is preset by software and remains unchanged during reloading.
2.3 Application of timer
2.3.1 Steps to use the timer
The following uses timer 0 as an example, and the same applies to timer 1.
Set the timer mode register TMOD and select the timer operating mode.
Set the initial value of the counting registers TH0 and TL0.
Set the timer control register TCON and set TR0 to 1 to start the timer counting.
By judging the TF0 bit in TCON, the overflow of the timer is monitored.
2.3.2 Calculate how to use a timer to set time
We know that the machine cycle of the standard 51 microcontroller is 12 clock cycles. Taking the STC89C52RC microcontroller as an example, its machine cycle is: 12/11059200 seconds. If the timing is 0.02s, it is assumed that x machine cycles are required, then x* (12/11059200) = 0.02. By calculating, x is equal to 18432. Since the overflow value of the 16-bit timer is 65536, then let TH0 and TL0 be equal to the initial value y. After 18432 machine cycles, the overflow occurs, thus achieving the timing of 0.02s. The y value is calculated as 47104 through 65536-18432, which is converted to hexadecimal 0xB800 (link: hexadecimal conversion method), that is, TH0 = 0xB8, TL0 = 0x00.
2.3.3 Control the flashing of the small light through the timer
Program to control the small light to flash at 0.5HZ frequency
#include sbit LED = P0^7; sbit ADDR3 = P1^3; sbit ADDR2 = P1^2; sbit ADDR1 = P1^1; sbit ADDR0 = P1^0; sbit ENLED = P1^4; void main() { unsigned char cnt = 0; //Define a counting variable to record the number of overflows of T1 ENLED = 0; ADDR3 = 1;
Previous article:[Self-study 51 single-chip microcomputer] 6 --- Introduction to digital tube dynamic display and interrupt system
Next article:[Self-study 51 single-chip microcomputer] 4---base conversion, C language variable types and operators
Recommended ReadingLatest update time:2024-11-16 12:51
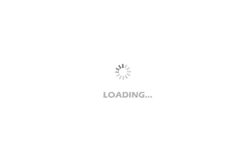
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications