Basic Applications of UART Serial Communication
Three basic types of communication:
Simplex communication: allows one party to send information to the other party, but the other party cannot send information back. Example: TV remote control, radio broadcast
Half-duplex communication: Data can be transmitted between two parties, but only one party can transmit to the other party at the same time. Example: Walkie-talkie
Full-duplex communication: data can be sent and received simultaneously. Example: telephone
UART Module Introduction
Usually, we care about the result of communication rather than the process. There is a UART module inside the 51 microcontroller, which can automatically receive data and send a notification signal after receiving. To use this module, you need to configure the corresponding register with special functions.
The UART serial port structure of the 51 single-chip microcomputer consists of three parts: the serial port control register SCON, the sending and receiving circuits.
First, let's understand the serial port control register SCON
SCON - bit allocation of serial control register (address 0X98, bit addressable)
SCON - Serial Control Register Bit Description
Of the four modes of the serial port, mode 1 is the most commonly used, which is the 1 start bit, 8 data bits and 1 stop bit mentioned above. The following is a detailed introduction to the working details and usage of mode 1 (the other 3 modes are similar to mode 1, and you can refer to the information when you need to use them).
In the hardware module, there is a dedicated baud rate generator to control the speed of sending and receiving data. For the STC89C52 microcontroller, this baud rate can only be generated by timer T1 or timer T2. This is completely different from the simulated communication concept. Timer T1 is used by default (timer T2 requires additional configuration registers).
The following mainly explains the use of T1 as a baud rate generator. The baud rate generator in mode 1 must use mode 2 of T1, which is the automatic reload mode.
The timer reload value calculation formula is: TH1 = TL1 = 256-crystal value/12/2/16/baud rate
Here is another calculator related to baud rate: the power management register PCON, its highest bit can double the baud rate, that is, if you write PCON |= 0X80, the calculation formula becomes: TH1 = TL1 = 256-crystal value/12/16/baud rate
The meanings of the numbers in the formula are explained as follows:
256——8-bit timer overflow value, 1TL1 overflow value.
Crystal value - 11059200 on the development board
12——1 machine cycle equals 12 clock cycles
16——collect one bit of signal 16 times (when the IO port simulates serial communication to receive data, the middle position of the data is collected, and the actual serial port module is more complex and accurate than the simulation. It collects one bit of signal 16 times, and takes out 7, 8, and 9 times. If two of these three times are high level, the data is considered to be 1, and if two are low level, the data is considered to be 0. In this way, even if the data is read incorrectly due to interference, the correctness of the final data can still be guaranteed)
After understanding the serial port acquisition mode, we can think about a question. Is it possible that the value calculated by "crystal value/12/2/16/baud rate" is a decimal? Understanding this also explains why the crystal oscillator uses 11059200. Because 11059200 can be divided by the common baud rate.
The sending and receiving circuits of the serial communication have two SBUF registers with the same name physically, and their addresses are the same, both 0X99. One of these two registers is used for sending buffer and the other for receiving buffer, which realizes the full-duplex communication of UART without interfering with each other. When operating SBUF, the microcontroller automatically determines and decides whether to select receiving SBUF or sending SBUF.
UART serial port program
In general, the steps for writing a serial communication program are as follows:
1) Configure the serial port to mode 1
2) Configure timer T1 to mode 2 (auto-reload mode)
3) Calculate the initial values of TH1 and TL1 according to the baud rate. If necessary, use PCON to double the baud rate.
4) Open the timer control register TR1 to start the timer running.
Note: When using T1, that is, when using T1 as a baud rate generator, do not enable the T1 interrupt.
The following is a demonstration code
#include void ConfigUART(unsigned int baud); //Serial port configuration function, baud——communication baud rate void main() { ConfigUART(9600); //Configure the baud rate to 9600 while(1) { while(!RI); //Wait for receiving to complete RI = 0; // Clear the receive interrupt flag SBUF = SBUF + 1; //Received data + 1, sent back while(!TI); //Wait for sending to complete TI = 0; // Clear the transmit interrupt flag } } void ConfigUART(unsigned int baud) { SCON = 0X50; //Configure the serial port to mode 1 TMOD &= 0X0F; // Clear the control bit of T1 TMOD |= 0X20; //Configure T1 to mode 2 TH1 = 256 - (11059200/12/32)/baud; //Calculate T1 reload value TL1 = TH1; //initial value equals reload value ES = 0; //Disable T1 interrupt TR1 = 1; //Start T1 } Note: In actual project development, this code is not written like this, because this code waits for the receive interrupt flag and the send interrupt flag in the main loop, which occupies a large amount of program memory. Serial port interrupts are often used when actually using the serial port. The following is the demonstration code for serial port interrupt #include void ConfigUART(unsigned int baud); //Serial port configuration function, baud——communication baud rate void main() { EA = 1; // Enable general interrupt ConfigUART(9600); //Configure the baud rate to 9600 while(1); } void ConfigUART(unsigned int baud) { SCON = 0X50; //Configure the serial port to mode 1 TMOD &= 0X0F; // Clear the control bit of T1 TMOD |= 0X20; //Configure T1 to mode 2 TH1 = 256 - (11059200/12/32)/baud; //Calculate T1 reload value TL1 = TH1; //initial value equals reload value ET1 = 1; //Disable T1 interrupt ES = 1; // Enable serial port interrupt TR1 = 1; //Start T1 } //UART interrupt service function void InterruptUART() interrupt 4 { if(RI) // Byte received { RI = 0; //Manually clear the receive interrupt flag SBUF = SBUF + 1; //The received data is +1 and then sent back. The left side is the sending SBUF and the right side is the receiving SBUF } if(TI) //Bytes sent { TI = 0; //Manually clear the transmit interrupt flag } }
Previous article:51 MCU serial communication uart notes
Next article:[Self-study 51 single-chip microcomputer] 11 -- UART serial communication
Recommended ReadingLatest update time:2024-11-17 00:04
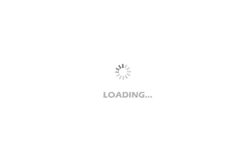
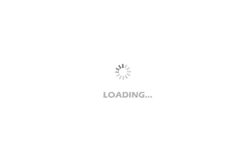
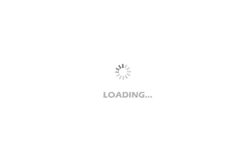
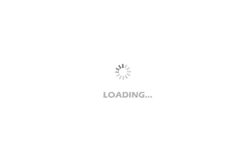
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Share a plug-in mpfshell that can run MicroPyhton code on VSCODE.
- 「Feedback」I found a very interesting front-end bug
- No matter what, in the end it will turn into people fighting people.
- 【Project source code】Timer based on FPGA TFT implementation
- [Modelsim FAQ] Error deleting "msim_transcript": permission denied...
- Please recommend a boost boot chip
- Interpolation filter design
- Embedded system reliability design technology and case analysis
- Broadband millimeter-wave digital-analog hybrid beamforming
- [ATmega4809 Curiosity Nano Review] Development Environment Setup